1 什么是LRU
LRU是Least Recently Used 的缩写,即最近最少使用,是一种常用的页面置换算法,选择最近最久未使用的页面予以淘汰。
2 实现思路
开始时,内存中没有页面。 每次访问页面时,先检测内存中是否存在该页面,若不存在则将该页面加载到内存“末尾”,若存在则直接访问该页面,并将该页面移到内存“末尾”。 如果访问某个内存中不存在的页面时,内存已满,则将内存“开头”的页面移出,并将新的页面加载到内存“末尾”。 这样就可以始终保持着最近访问的页面在不经常访问的页面的后面了。
3数据结构的选择
3.1 数组
- 页面命中时,需要将其移动到数组末尾。
- 页面命中失败时,需要删除数组头部元素,并将所有元素向前移动一步,然后在末尾添加元素。
这两个操作都涉及大量元素的移动,时间复杂度为O(n),效率较低。
3.2 链表
虽然不涉及到元素的移动,但是检查页面是否命中需要遍历链表,时间复杂度也为O(n)
3.3 双向链表 + 哈希表
双向链表维护最近使用的和很久没有使用页面的先后顺序,哈希表用于定位页面在双向链表中的位置,方便查找。 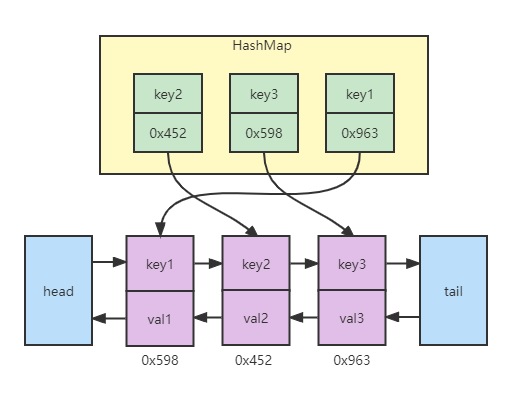 用双向链表是因为删除链表节点时,需要知道该节点的前驱节点。 双向链表两边特意加上虚拟头节点和虚拟伪节点,以使得链表两端元素的插入和删除操作与链表中间的元素保持一致。
有点类似于Java提供的集合LinkedHashMap 。借助LinkedHashMap 很容易实现LRU算法,我这里就不展示了。不借助LinkedHashMap 的代码实现:
import java.util.HashMap;
import java.util.Map;
public class LRUCache<K, V> {
static class Entry<K, V> {
public Entry<K, V> prev;
public Entry<K, V> next;
public K key;
public V val;
public Entry() {}
public Entry(K key, V val) { this.key = key; this.val = val; }
}
private Entry<K, V> head, tail;
private final int capacity;
private int size;
Map<K, Entry<K, V>> cache;
public LRUCache(int capacity) {
this.capacity = capacity;
initCache();
}
private void initCache() {
head = new Entry<>();
tail = new Entry<>();
head.next = tail;
tail.prev = head;
size = 0;
cache = new HashMap<>(this.capacity);
}
private V get(K key) {
Entry<K, V> entry = cache.get(key);
if(entry != null) {
moveToTail(entry);
return entry.val;
} else {
return null;
}
}
private void put(K key, V val) {
Entry<K, V> entry = cache.get(key);
if(entry != null) {
entry.val = val;
moveToTail(entry);
} else {
if(size == capacity) {
Entry<K, V> h = head.next;
deleteEntry(h);
cache.remove(h.key);
size--;
}
Entry<K, V> newEntry = new Entry<>(key, val);
addToTail(newEntry);
cache.put(key, newEntry);
size++;
}
}
private void moveToTail(Entry<K, V> entry) {
deleteEntry(entry);
addToTail(entry);
}
private void addToTail(Entry<K, V> entry) {
if(entry != null) {
entry.next = tail;
entry.prev = tail.prev;
tail.prev.next = entry;
tail.prev = entry;
}
}
private void deleteEntry(Entry<K, V> entry) {
if(entry != null) {
entry.prev.next = entry.next;
entry.next.prev = entry.prev;
}
}
public static void main(String[] args) {
LRUCache<Integer, String> cache = new LRUCache<>(2);
cache.put(1,"可口可乐");
cache.put(2,"雪碧");
System.out.println("页面1的内容:" + cache.get(1));
cache.put(3,"果粒橙");
System.out.println("页面2的内容:" + cache.get(2));
}
}
运行结果:
页面1的内容:可口可乐
页面2的内容:null
|