栈(后进先出 Last In First Out)
一种特殊的线性表,其只允许在固定的一端进行插入和删除元素操作。
进行数据插入和删除操作的一端
称为栈顶,另一端称为栈底。
栈中的数据元素遵守后进先出
LIFO
(
Last In First Out
)的原则。
压栈:栈的插入操作叫做进栈
/
压栈
/
入栈,
入数据在栈顶
。
出栈:栈的删除操作叫做出栈。
出数据也在栈顶
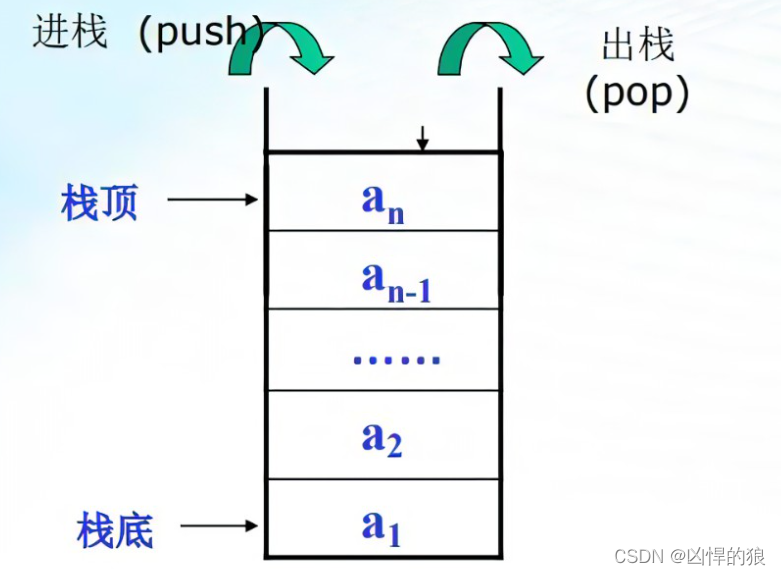
代码实现
stack.h
#pragma once
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
#include <string.h>
typedef int STDataType;
typedef struct Stack{
STDataType* _a;
int _top; // 栈顶
int _capacity; // 容量
}Stack;
// 初始化栈
void StackInit(Stack* ps);
// 入栈
void StackPush(Stack* ps, STDataType data);
// 出栈
void StackPop(Stack* ps);
// 获取栈顶元素
STDataType StackTop(Stack* ps);
// 获取栈中有效元素个数
int StackSize(Stack* ps);
// 检测栈是否为空,如果为空返回非零结果,如果不为空返回0
int StackEmpty(Stack* ps);
// 销毁栈
void StackDestroy(Stack* ps);
stack.c
#pragma once
#include "stack.h"
//初始化
void StackInit(Stack* ps){
assert(ps);
ps->_top = 0;
ps->_capacity = 0;
ps->_a = NULL;
}
//检查容量
static void Checkcapacity(Stack* ps){
assert(ps);
if (ps->_capacity == ps->_top){
int newCap = (ps->_capacity == 0 ? 2 : (ps->_capacity * 2));
ps->_a = (STDataType*)realloc(ps->_a,newCap*(ps->_capacity)*sizeof(STDataType));
assert(ps->_a);
ps->_capacity = newCap;
//printf("add capcity\n");//可以注释
}
}
void StackPush(Stack* ps,STDataType x){
Checkcapacity(ps);
ps->_a[ps->_top] = x;
ps->_top++;
}
void StackPop(Stack* ps){
if (ps->_top == 0){
//printf("top is 0\n");//可注释,方便调试
return;
}
--(ps->_top);
}
int StackEmpty(Stack* ps){
assert(ps);
return ps->_top==0;
}
void StackDestory(Stack* ps){
assert(ps);
if (ps->_a){
free(ps->_a);
ps->_a = NULL;
ps->_capacity = 0;
ps->_top = 0;
}
}
队列(先进先出 FIFO)
队列:只允许在一端进行插入数据操作,在另一端进行删除数据操作的特殊线性表,队列具有先进先出
FIFO(First In First Out)
入队列:进行插入操作的一端称为
队尾
出队列:进行删除操作的一端称为
队头
?代码实现
?queue.h
#pragma once
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <assert.h>
typedef int QDataType;
typedef struct QListNode{
QDataType _data;
struct QListNode* _next;
}QNode;
// 队列的结构
typedef struct Queue{
QNode* _front;
QNode* _rear;
}Queue;
// 初始化队列
void QueueInit(Queue* q);
// 队尾入队列
void QueuePush(Queue* q, QDataType data);
// 队头出队列
void QueuePop(Queue* q);
// 获取队列头部元素
QDataType QueueFront(Queue* q);
// 获取队列队尾元素
QDataType QueueBack(Queue* q);
// 获取队列中有效元素个数
int QueueSize(Queue* q);
// 检测队列是否为空,如果为空返回非零结果,如果非空返回0
int QueueEmpty(Queue* q);
// 销毁队列
void QueueDestroy(Queue* q);
?queue.c
#include "queue.h"
void QueueInit(Queue* q){
assert(q);
q->_front = NULL;
q->_rear = NULL;
}
void QueuePush(Queue* q,QDataType x){
assert(q);
QNode* Node = (QNode*)calloc(1,sizeof(QNode));
assert(Node);
Node->_data = x;
if (q->_front == NULL){
q->_front = q->_rear = Node;
}
else{
q->_rear->_next = Node;
q->_rear = Node;
}
}
void QueuePop(Queue* q){
assert(q);
Queue* head = q->_front;
if (head == NULL)return;
if (head == q->_rear){ //判断是否为一个节点
q->_rear=q->_front = q->_front->_next;
free(head);
}
else{
q->_front = q->_front->_next;
free(head);
}
}
QDataType QueueFront(Queue* q){
return q->_front->_data;
}
QDataType QueueBack(Queue* q){
return q->_rear->_data;
}
int QueueEmpty(Queue* q){
return q->_front == NULL;
}
int QueueSize(Queue* q){
QNode* cur;
int count = 0;
for (cur = q->_front; cur; cur = cur->_next){
count++;
}
return count;
}
void QueueDestory(Queue* q){
if (q->_front == NULL){
return;
}
while (q->_front){
QueuePop(q);
}
}
习题自测:
本次习题较为简单啦,这里就不作赘述.?
|