霍夫曼树
一、介绍

二、重要概念
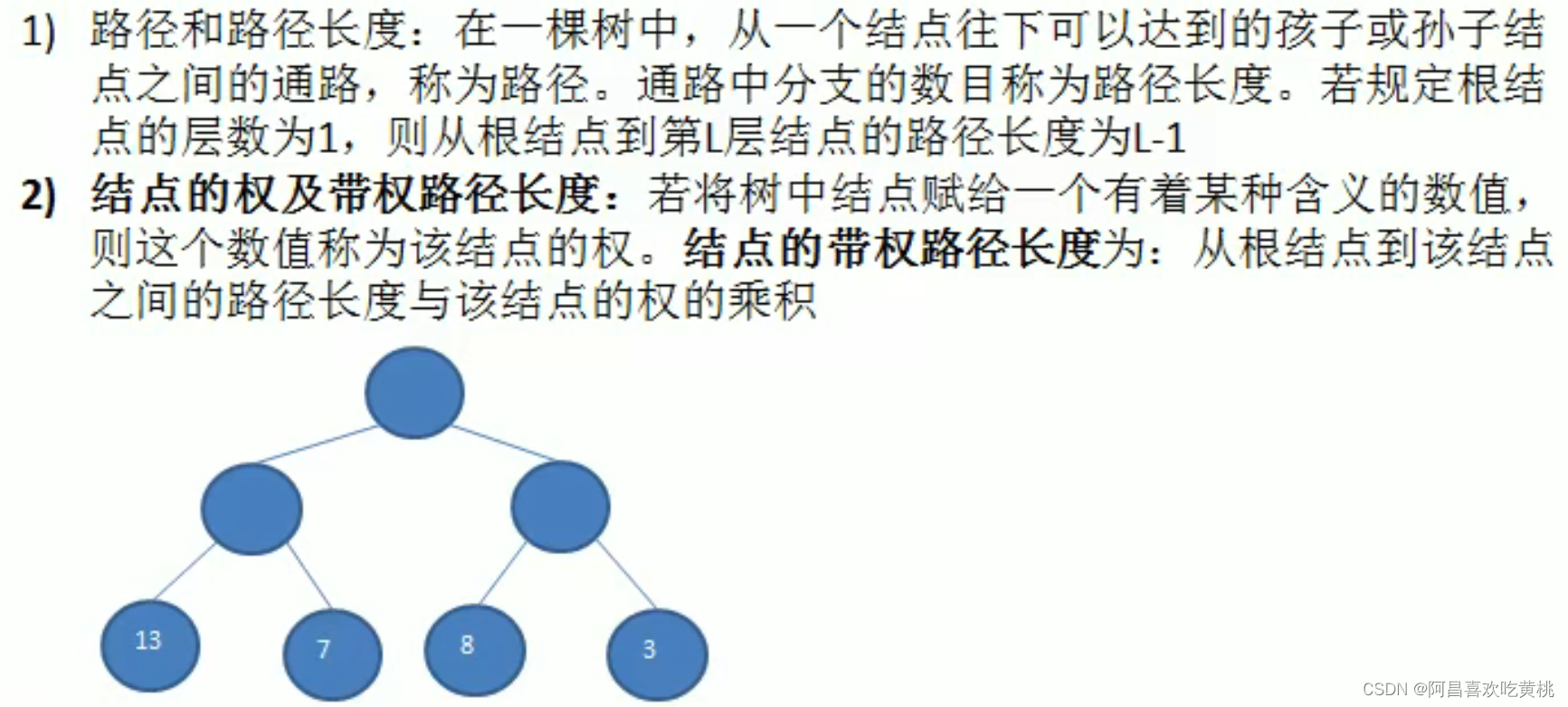
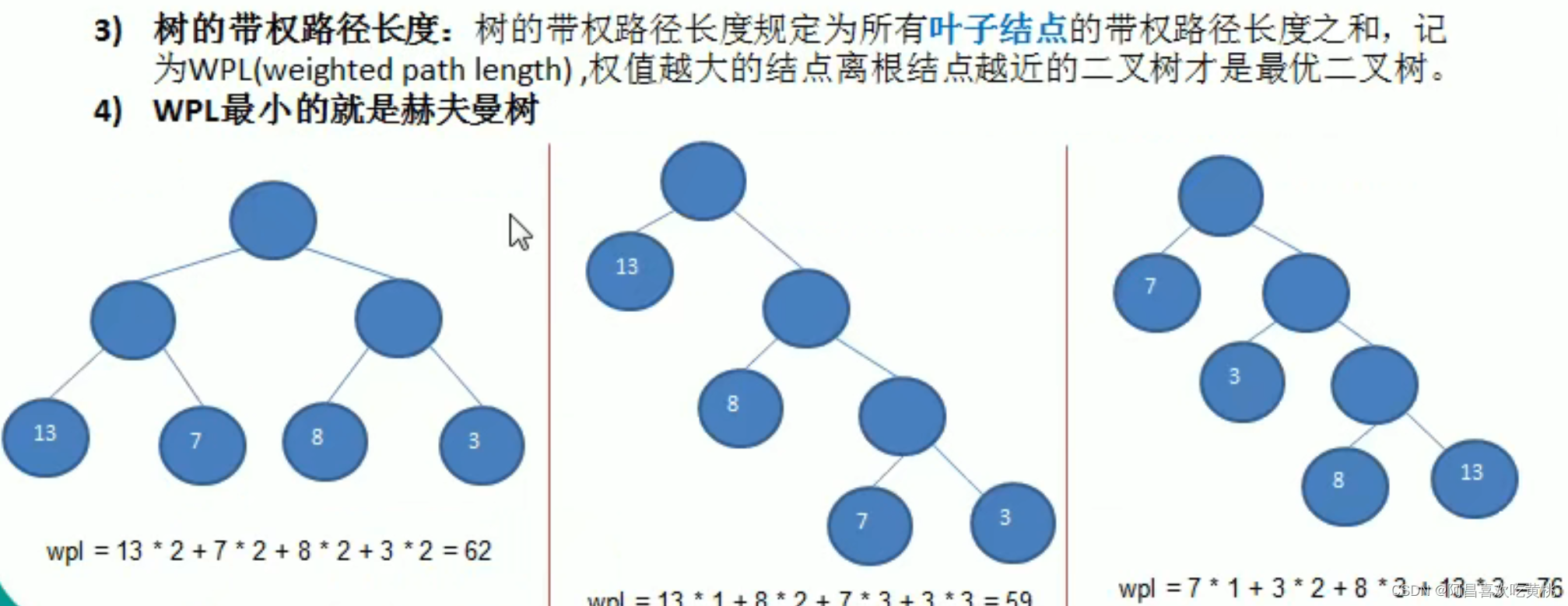
三、图解思路
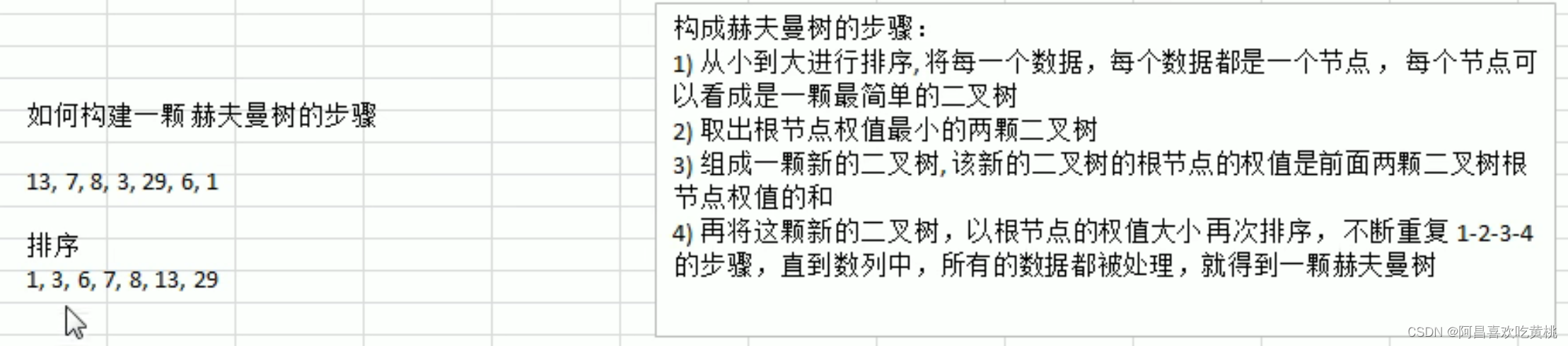
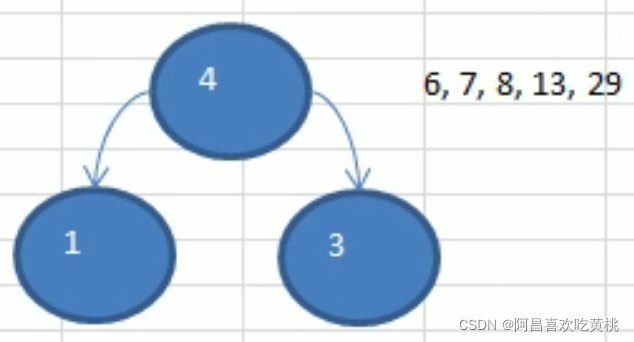
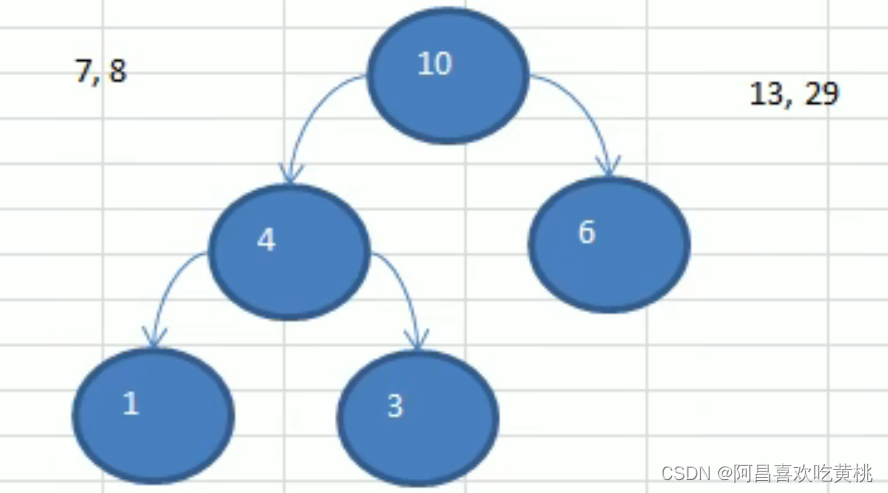
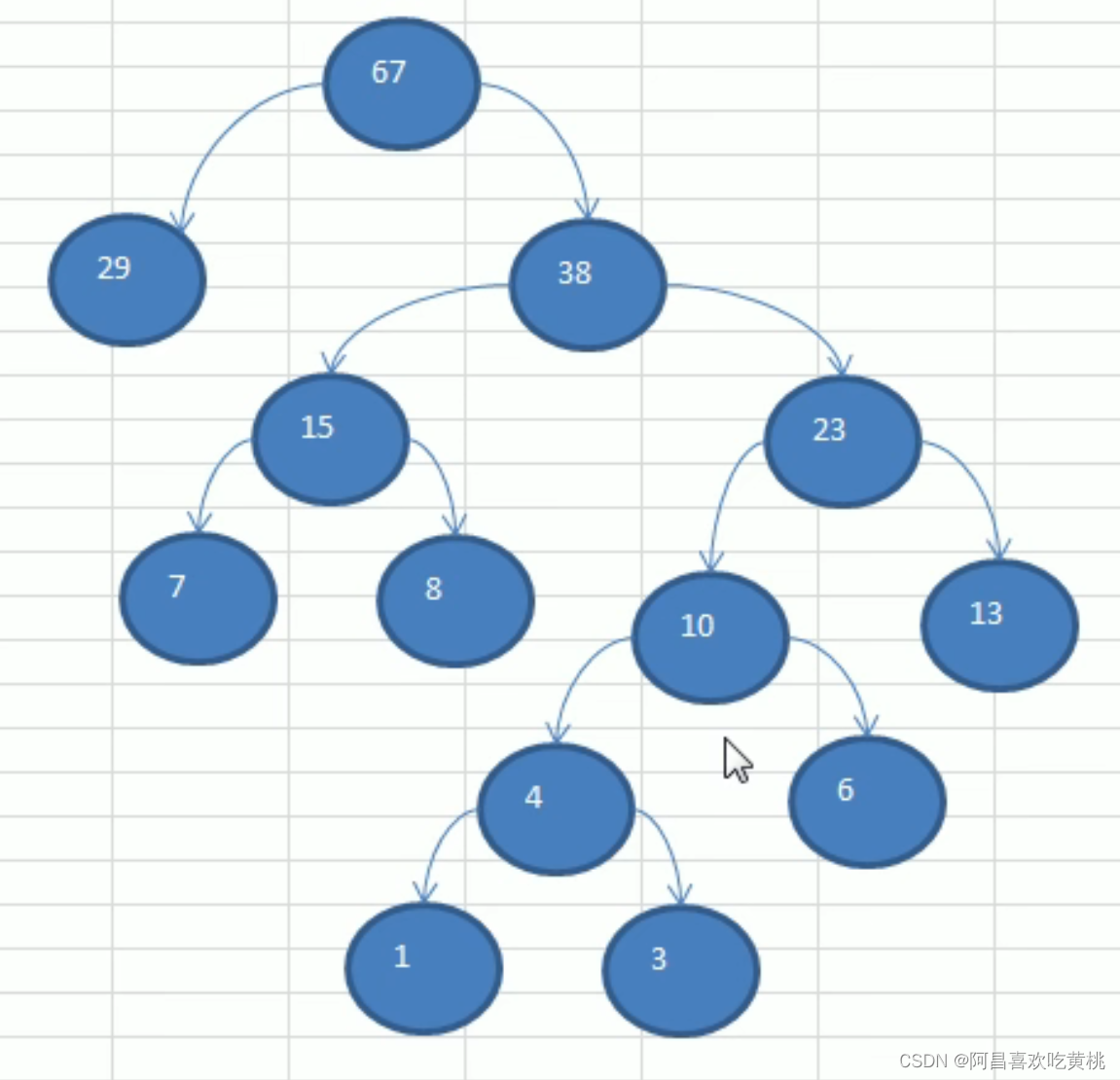
四、代码实现
package com.achang.tree;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class HufumanTree {
public static void main(String[] args) {
int[] arr = {13, 7, 8, 3, 29, 6, 1};
Node2 hufuManTree = buildHufuManTree(arr);
hufuManTree.preList();
}
public static Node2 buildHufuManTree(int[] arr) {
List<Node2> list = buildListSort(arr);
System.out.println("未处理:"+list);
while (list.size() != 1){
Node2 leftNode = list.get(0);
Node2 rightNode = list.get(1);
Node2 parent = new Node2(rightNode.value + leftNode.value);
parent.left = leftNode;
parent.right = rightNode;
list.add(parent);
list.remove(leftNode);
list.remove(rightNode);
Collections.sort(list);
}
System.out.println("处理结束:"+list);
return list.get(0);
}
private static List<Node2> buildListSort(int[] arr) {
ArrayList<Node2> list = new ArrayList<>();
for (int i : arr) {
list.add(new Node2(i));
}
Collections.sort(list);
return list;
}
}
class Node2 implements Comparable<Node2> {
public Node2 left;
public Node2 right;
public int value;
public Node2(int value) {
this.value = value;
}
@Override
public String toString() {
return "Node2[value=" + value + "]";
}
@Override
public int compareTo(Node2 o) {
return this.value - o.value;
}
public void preList(){
System.out.println(this);
if (this.left != null) {
this.left.preList();
}
if (this.right != null) {
this.right.preList();
}
}
}
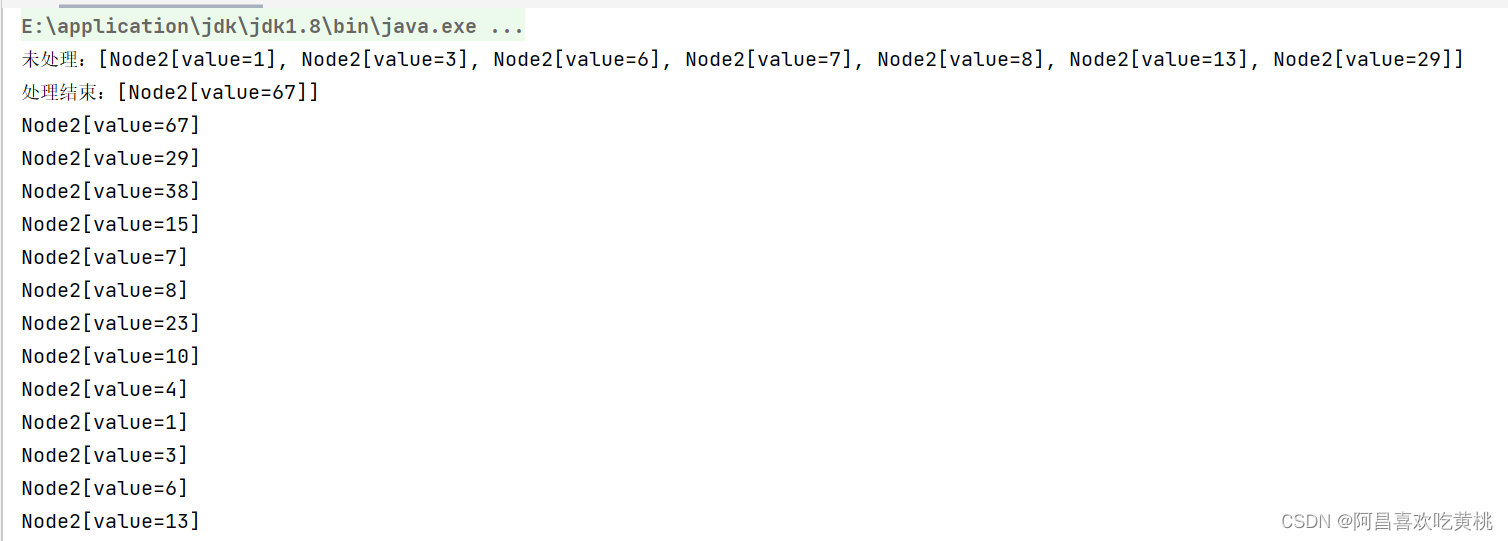
|