- delete方法里面的while (head != null)判断的相关示意图
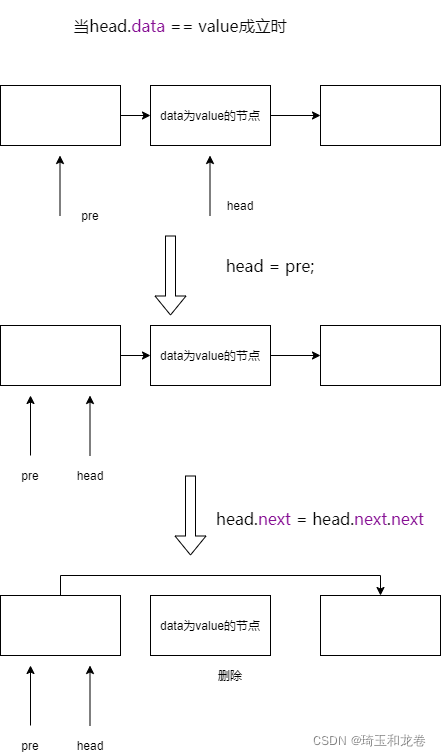 - 代码
public class LinkedNode {
Node head;
int data;
public void add(int value) {
Node newNode = new Node(value);
if (this.head == null) {
this.head = newNode;
return;
}
newNode.next = this.head;
this.head = newNode;
}
public void delete(int value) {
if (this.head == null) {
throw new RuntimeException("无法对空链表进行删除");
}
Node pre = this.head;
Node head = this.head;
while (head != null) {
if (head.data == value) {
head = pre;
head.next = head.next.next;
} else {
pre = head;
head = head.next;
}
}
}
public void display() {
if (this.head == null) {
System.out.println("The List is empty.");
return;
}
Node cur = this.head;
StringBuilder stringBuilder = new StringBuilder();
while (cur != null) {
stringBuilder.append(cur.data + " -> ");
cur = cur.next;
}
System.out.println(stringBuilder.append("NULL").toString());
}
public static void main(String[] args) {
LinkedNode linkedNode = new LinkedNode();
linkedNode.add(1);
linkedNode.add(2);
linkedNode.add(3);
linkedNode.add(3);
linkedNode.add(4);
System.out.println("初始模样");
linkedNode.display();
linkedNode.delete(3);
System.out.println("删除3");
linkedNode.display();
System.out.println("删除1");
linkedNode.delete(1);
linkedNode.display();
}
class Node {
public int data;
public Node next;
public Node(int data) {
this.data = data;
}
}
}
- 结果
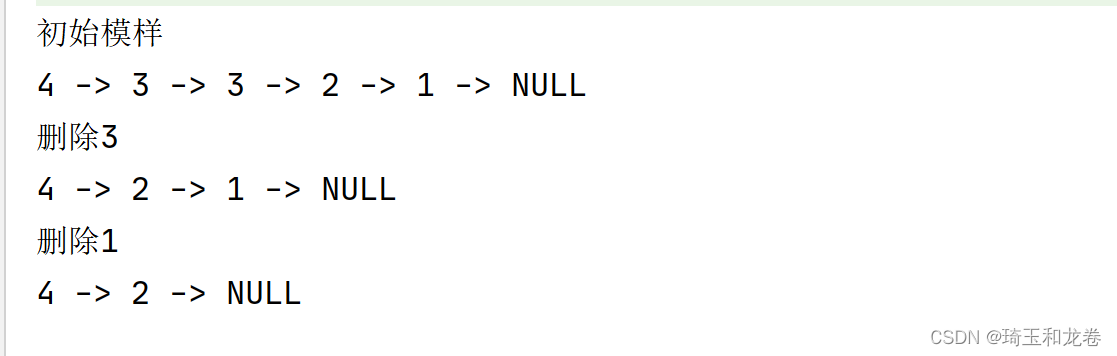
|