Map接口及其实现
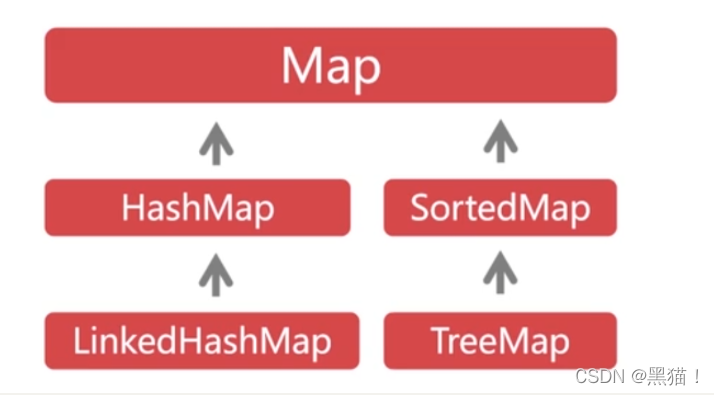
Map的遍历一共有四种
1、keySet
public static void showMap1(Map<String,Integer> userMap){
Long start = System.currentTimeMillis();
for(String key : userMap.keySet()){
System.out.println(key + "***********" + userMap.get(key));
}
Long end = System.currentTimeMillis();
System.out.println("time" +"===============" + (end - start));
}
2、values
public static void showMap2(Map<String,Integer> userMap){
Long start = System.currentTimeMillis();
for(Integer v : userMap.values()){
//System.out.println(v);
}
Long end = System.currentTimeMillis();
System.out.println("time" +"===============" + (end - start));
}
3、entrySet
public static void showMap3(Map<String,Integer> userMap){
Long start = System.currentTimeMillis();
for (Map.Entry<String, Integer> entry : userMap.entrySet()) {
System.out.println(entry.getKey() + "===========" + entry.getValue());
}
Long end = System.currentTimeMillis();
System.out.println("time" +"===============" + (end - start));
}
4、Iterator
public static void showMap4(Map<String,Integer> userMap){
Long start = System.currentTimeMillis();
Iterator<Map.Entry<String, Integer>> it = userMap.entrySet().iterator();
while(it.hasNext()){
Map.Entry<String,Integer> entry = it.next();
System.out.println(entry.getKey() + "*****" + entry.getValue());
}
Long end = System.currentTimeMillis();
System.out.println("time" +"===============" + (end - start));
}
其中entrySet比较常用,其次为iterator,除了keySet性能相比来说较慢,其他三个性能都差不多。 下面为entry在HashMap中的机构
static class Node<K,V> implements Map.Entry<K,V> {
final int hash;
final K key;
V value;
Node<K,V> next;
Node(int hash, K key, V value, Node<K,V> next) {
this.hash = hash;
this.key = key;
this.value = value;
this.next = next;
}
HashMap底层原理
Map map = new HashMap()
HashMap不带参的构造方法,默认长度为16,默认的负载因子是0.75 等同于
Map map = new HashMap(16,0.75f)
HashMap带参的构造方法
HashMap(int initialCapacity) : 例如 new HashMap(3)
这里的initialCapacity传入的值,初始化HashMap的时候会创建一个大于initialCapacity的最小的2的n次方的值,比如传入3,就创建大小为4,传入5,就创建大小为8。
HashMap(int initialCapacity,float loadFactor)
在HashMap创建的时候,自身的大小要根据自身的业务去进行创建,因为如果初始值小了,扩容会影响效率,会重新计算map中hashcode()的值,寻找对应的位置。
new HashMap(10000,0.75f)
如果录入的数据有10000条,会产生扩容吗?
首选会找到大于10000的最小的2的n次方的值,为2的14次方.
2^14 = 16384 * 0.75 = 12288 所以不会产生扩容。
LinkedHashMap在插入数据的时候比HashMap慢,在遍历的时候比HashMap快。
|