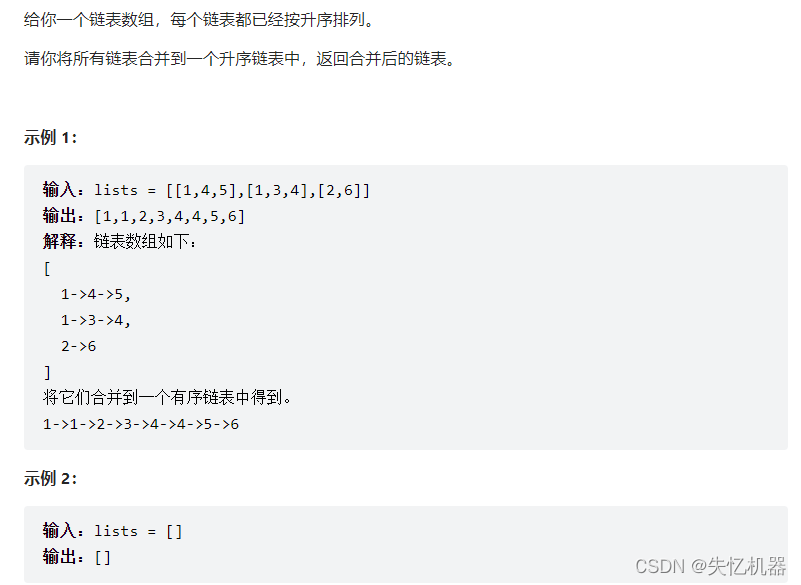
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode mergeKLists(ListNode[] lists) {
if(lists.length == 0){
return null;
}
PriorityQueue<ListNode> queue = new PriorityQueue<ListNode>(lists.length,(o1,o2)->(o1.val - o2.val));
ListNode tail = new ListNode(0);
ListNode head = tail;
for(ListNode node : lists){
if(node != null) queue.add(node);
}
while(!queue.isEmpty()){
ListNode cur = queue.poll();
head.next = cur;
head = head.next;
if(cur.next != null){
queue.add(cur.next);
}
}
return tail.next;
}
}
优先队列来做,?O(Nlogk)? k是链表数。
|