对于新定义的串,一定要初始化,否则做基本操作时容易出错(指针不为空)
比如赋值时,会判断str.ch是否为空,若非空,则用free函数将其内存空间释放掉
当没有初始化直接调用该函数赋值时,会在if(str.ch) free(str.ch)出错
出错原因:free函数释放的是malloc函数申请的空间
如下图所示,声明变量是会分配地址空间的,此时若直接为s2赋值的话,free(str.ch)会出错,因为此时的s2不是malloc函数申请的空间,是系统自动分配的
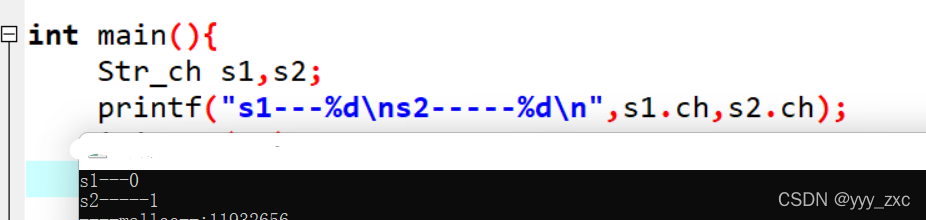
#include<stdio.h>
#include<stdlib.h>
#include<malloc.h>
#define maxSize 10
//-------串-----
//定长顺序存储
typedef struct{
char str[maxSize+1];
int length;
}Str;
//变长分配存储
typedef struct{
char *ch; //指向动态分配存储区首地址的字符指针
int length; //串长度
}Str_ch;
//初始化
void initstr(Str_ch & str){
str.ch = NULL;
str.length = 0;
}
//给串赋值
int strassign(Str_ch & str,char *arr){
int i;
if(str.ch){ //当str不为空时,释放其首地址指针
free(str.ch);
}
int len = 0;
char *c = arr; //c指针指向字符串首地址
//求字符串长度
while(*c){ //当c指向空,也就是遍历完arr时,退出循环
++len;
++c; //c的值加1,也就是地址值加一
}
if(len == 0){ //如果传入字符串为空,则将str置为空
str.ch = NULL;
str.length = 0;
return 1;
} else{
str.ch=(char*)malloc(sizeof(char)*(len+1)); //多分配一个空间给结束符
if(str.ch == NULL)
return 0; //分配失败
else{
c=arr;
//给str赋值
for(i=0;i<=len;++i,++c){
str.ch[i]=*c; //c是所指向的地址值,*c是指所向地址的值
}
str.length = len;
return 1;
}
}
}
//清空串
void clearstring(Str_ch &str){
if (str.ch){
free(str.ch);
str.ch = NULL;
}
str.length = 0;
printf("清理完成\n");
}
int main(){
Str_ch s1,s2;
printf("s1---%d\ns2-----%d\n",s1.ch,s2.ch);
initstr(s1);
initstr(s2);
int i,a,b;
a=strassign(s1,"input");
a=strassign(s2,"inooo");
for(i=0;i<=5;++i){
printf("---%c\n",s1.ch[i]);
}
for(i=0;i<=5;++i){
printf("---%c\n",s2.ch[i]);
}
clearstring(s1);
clearstring(s2);
return 1;
}
?
|