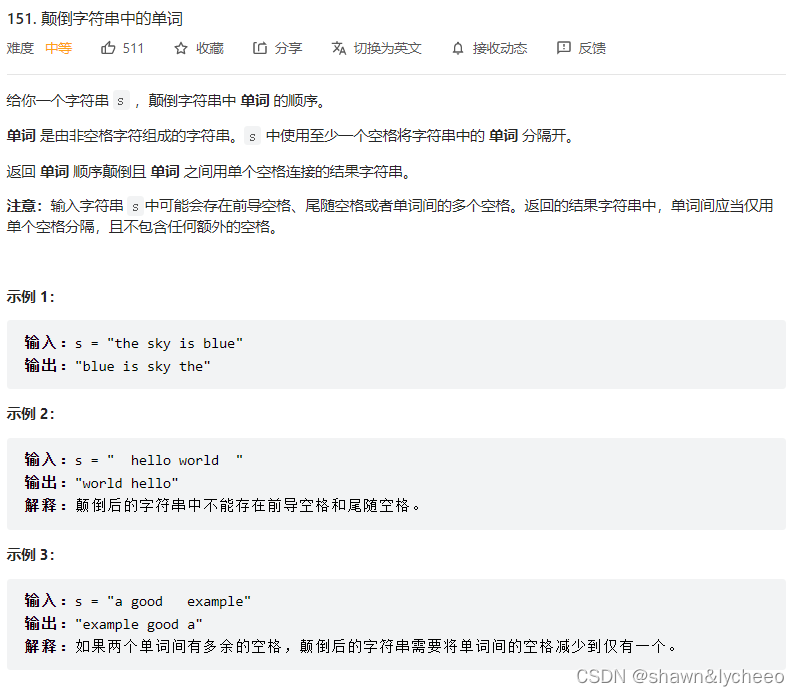
?
class Solution {
public:
//移除多余空格
void removespace(string& s){
int fastindex = 0, slowindex = 0;
//查找前面的多余空格
while (s.size() > 0 && fastindex < s.size() && s[fastindex] == ' '){
fastindex++;
}
for (; fastindex < s.size(); fastindex++){
//去掉前面和中间的空格
if (fastindex - 1 > 0 && s[fastindex - 1] == s[fastindex] && s[fastindex] == ' '){
continue;
}
else {
s[slowindex++] = s[fastindex]; //注意,这里是fast指针,
}
}
//去掉后面空格,调整字符串长度
if (slowindex - 1 > 0 && s[slowindex - 1] == ' '){
s.resize(slowindex - 1);
}
else {
s.resize(slowindex);
}
}
//字符串翻转
void reversestring(string& s, int start, int end){
for (int i = start, j = end;i < j; i++, j--){ //终止条件
swap(s[i], s[j]);
}
}
string reverseWords(string s) {
removespace(s);
int len = s.size();
reversestring(s, 0, len - 1);
for(int i = 0; i < s.size(); i++) {
int j = i;
// 查找单词间的空格,翻转单词
while(j < s.size() && s[j] != ' ') j++;
reversestring(s, i, j - 1);
i = j;
}
return s;
}
};
|