给定一个链表,两两交换其中相邻的节点,并返回交换后的链表。
你不能只是单纯的改变节点内部的值,而是需要实际的进行节点交换。
-1为输入结束标志
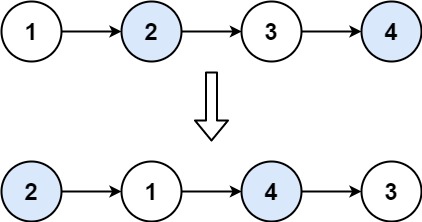
输入:2 3 4 5 6 7 8 -1
输出:3 2 5 4 7 6 8
//
// main.cpp
// link
//
// Created by chococolate on 2022/4/12.
//
#include <iostream>
using namespace std;
struct Node
{
int value;
Node *next;
};
Node * initList(){
Node *p=(Node *)malloc(sizeof(Node));
Node *head=p;
int n;
while(cin>>n && n!=-1)
{
Node *a=(Node *)malloc(sizeof(Node));
a->value=n;
a->next=NULL;
p->next=a;
p=p->next;
}
return head;
}
Node* reverse(Node *head){
Node *orign_head=head;//用于指向链表
Node *prehead=head;//prehead为交换左结点的前驱
head=head->next;
if(head==NULL||head->next==NULL)return head;//如果没有/只有一个节点,直接返回
Node *left;
Node *right;
Node *aft;
left=head;//left为交换左结点
right=head->next;//right为交换右结点
aft=right->next;//aft为交换结点的后驱
while(right!=NULL)
{
right->next=left;
prehead->next=right;
prehead=left;
if(aft==NULL)//后驱节点为空
{
prehead->next=NULL;
break;
}
left=aft;//后驱节点变成下次交换的左结点
right=aft->next;//下次交换的右结点
if(right == NULL)//链表交换结束
{
prehead->next = left;
}else{//获得下次交换右结点的后驱
aft = right->next;
}
}
return orign_head;
}
int main()
{
Node* list;
Node* slist;
list=initList();//创建头结点为空的链表
slist=reverse(list);//交换相邻的结点
slist=slist->next;//跳过头结点
//打印链表
do{
printf("%d ",slist->value);
slist=slist->next;
} while(slist!=NULL);
}