Collection
基本体系结构
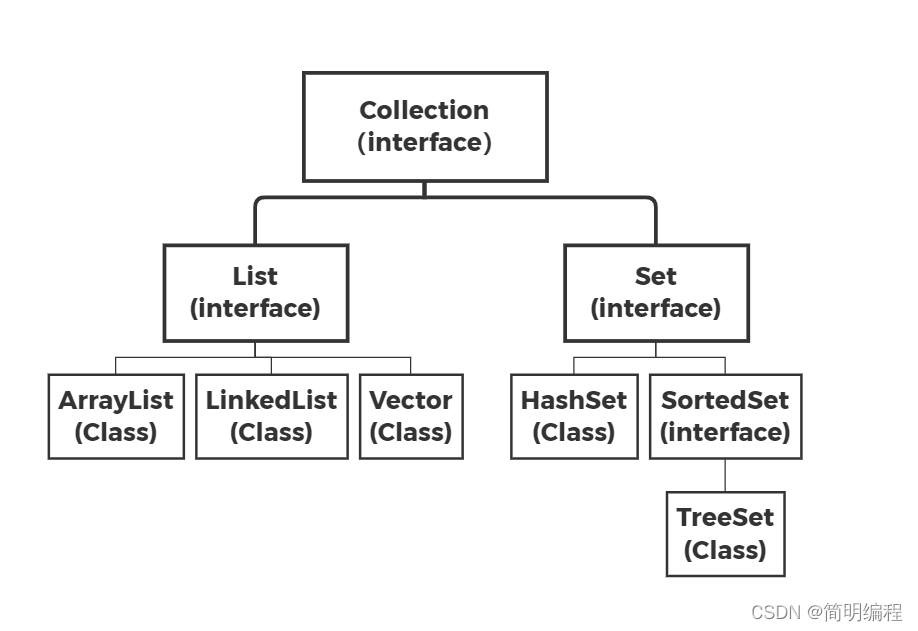
Collection的特点
代表一组任意类型的对象,无序,无下标,不能重复
方法(基于源码)
- boolean add(E e);
向容器中添加一个对象 - int size();
返回此集合中元素的个数 - boolean remove(Object o);
移除集合中的指定对象 - boolean containsAll(Collection<?> c);
判断集合中是否有参数集合的所有元素 - boolean addAll(Collection<? extends E> c);
向集合中添加参数集合中的所有元素 - boolean removeAll(Collection<?> c);
将集合中所有参数集合的元素移除 - boolean retainAll(Collection<?> c);
在集合中仅保留参数集合中的元素,删除其他元素 - void clear();
清除集合中所有的对象 - boolean equals(Object o);
比较此集合是否与指定对象相等 - Object[] toArray();
将集合转化为数组 - boolean contains(Object o);
判断集合中是否含有参数对象 - boolean isEmpty();
判断集合是否为空 - Iterator iterator();
返回此集合中元素的迭代器。对于元素的返回顺序没有任何保证(除非此集合是提供保证的某个类的实例) - int hashCode();
返回此集合的哈希值
案例
我们知道Collection是个接口具体实现还需要使用到ArrayList,LinkedList,HashSet等 所以案例中我使用ArrayList进行演示
案例1
1中我们使用了add,remove,removeAll,forEach,size,isEmpty,hashCode
package collection;
import java.util.ArrayList;
import java.util.Collection;
public class demo1 {
public static void printCollection(Collection<String> val) {
System.out.println("---------------------");
System.out.println("collection中的元素:");
val.forEach(System.out::println);
System.out.println("collection的长度:" + val.size());
System.out.println("collection是否为空:" + val.isEmpty());
System.out.println("collection的哈希值:" + val.hashCode());
System.out.println("---------------------");
}
public static void main(String[] args) {
Collection<String> collection = new ArrayList<>();
collection.add("元素1");
collection.add("元素2");
collection.add("元素3");
collection.add("元素4");
printCollection(collection);
collection.remove("元素2");
printCollection(collection);
collection.removeAll(collection);
printCollection(collection);
}
}
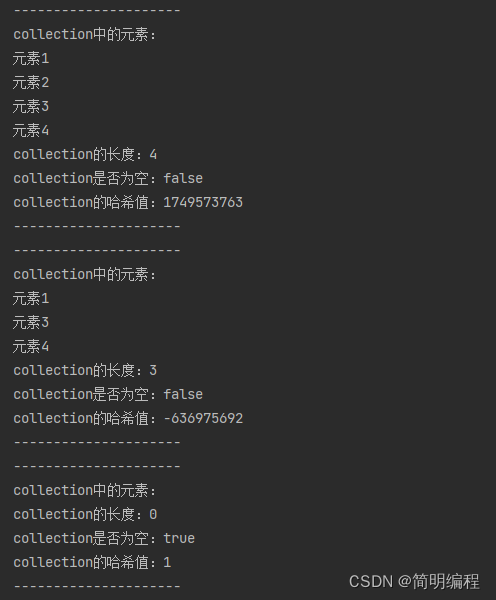
案例2
2中我们使用了将removeAll换成了clear,forEach换成iterator迭代器
package collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class demo2 {
public static void printCollection(Collection<String> val) {
System.out.println("---------------------");
System.out.println("collection中的元素:");
Iterator<String> iterator = val.iterator();
while (iterator.hasNext()){
String str = iterator.next();
System.out.println(str);
}
System.out.println("collection的长度:" + val.size());
System.out.println("collection是否为空:" + val.isEmpty());
System.out.println("collection的哈希值:" + val.hashCode());
System.out.println("---------------------");
}
public static void main(String[] args) {
Collection<String> collection = new ArrayList<>();
collection.add("元素1");
collection.add("元素2");
collection.add("元素3");
collection.add("元素4");
printCollection(collection);
collection.remove("元素2");
printCollection(collection);
collection.clear();
printCollection(collection);
}
}
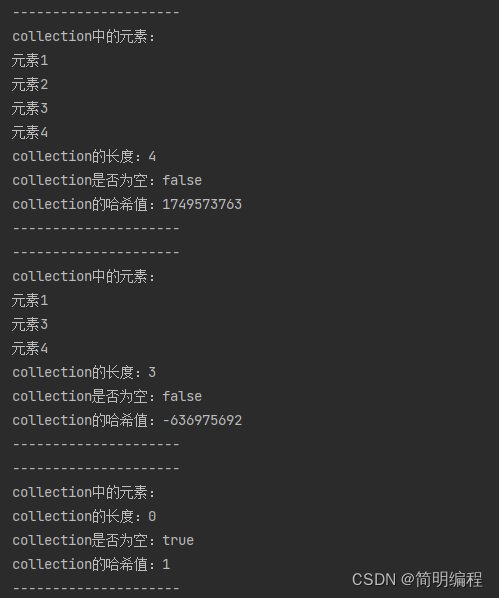
案例3
使用自定的类进行集合操作
user类
package collection.entity;
public class User {
private Integer id;
private String username;
private String password;
public User(Integer id, String username, String password) {
this.id = id;
this.username = username;
this.password = password;
}
public User() {
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", username='" + username + '\'' +
", password='" + password + '\'' +
'}';
}
}
实体类
package collection;
import collection.entity.User;
import java.util.ArrayList;
import java.util.Collection;
public class demo3 {
public static void printCollection(Collection<User> val) {
System.out.println("---------------------");
System.out.println("collection中的元素:");
val.forEach(System.out::println);
System.out.println("collection的长度:" + val.size());
System.out.println("collection是否为空:" + val.isEmpty());
System.out.println("collection的哈希值:" + val.hashCode());
System.out.println("---------------------");
}
public static void main(String[] args) {
User user1 = new User(1, "张三", "1234566");
User user2 = new User(2, "李四", "1234567");
User user3 = new User(3, "王五", "1234568");
User user4 = new User(4, "老六", "1234569");
Collection<User> collection = new ArrayList<>();
collection.add(user1);
collection.add(user2);
collection.add(user3);
collection.add(user4);
printCollection(collection);
collection.remove(user3);
printCollection(collection);
collection.clear();
printCollection(collection);
}
}
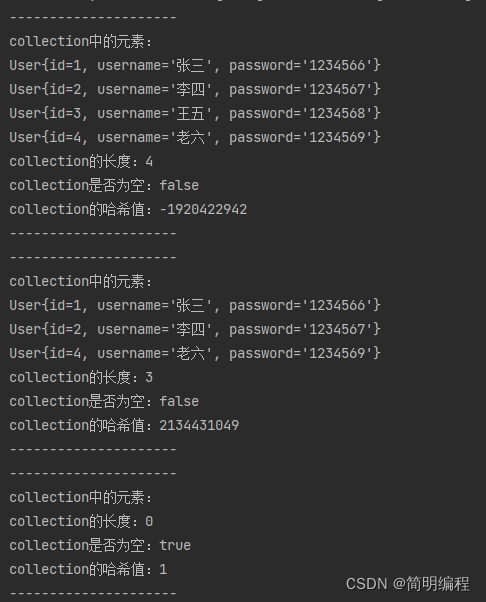
|