一、字符与整数之间的关系
字符其实是以ASCII的形式在计算机中展现的。 每个常用字符都对应一个-128 ~ 127的数字,二者之间可以相互转化: (但是ASCII为负数的情况一般用不到)
#include <iostream>
using namespace std;
int main()
{
char c = 'a';
cout << (int)c << endl;
int a = 66;
cout << (char)a << endl;
return 0;
}
常用ASCII值:'A'- 'Z' 是65 ~ 90,'a' - 'z' 是97 - 122,0 - 9 是 48 - 57。 字符可以参与运算,运算时会将其当做整数:
#include <iostream>
using namespace std;
int main()
{
int a = 'B' - 'A';
int b = 'A' * 'B';
char c = 'A' + 2;
cout << a << endl;
cout << b << endl;
cout << c << endl;
return 0;
}
二、字符串数组
字符串就是字符数组加上结束符'\0' 。 可以使用字符串来初始化字符数组,但此时要注意,每个字符串结尾会暗含一个'\0' 字符,因此字符数组的长度至少要比字符串的长度1!
#include <iostream>
using namespace std;
int main()
{
char a1[] = {'C', '+', '+'};
char a2[] = {'C', '+', '+', '\0'};
char a3[] = "C++";
char a4[6] = "Daniel";
return 0;
}
2.1 字符数组的输入输出:
#include <iostream>
using namespace std;
int main()
{
char str[100];
cin >> str;
cout << str << endl;
printf("%s\n", str);
puts(str);
return 0;
}
读入一行字符串,包括空格:
#include <iostream>
using namespace std;
int main()
{
char str[100];
fgets(str, 100, stdin);
string s;
getline(cin,s);
char a[100];
cin.getline(a,100);
cout << str << endl;
return 0;
}
2.2 字符数组的常用操作
下面几个函数需要引入头文件:
#include <string.h>
(1) strlen(str) ,求字符串的长度 (2) strcmp(a, b) ,比较两个字符串的大小,a < b 返回-1 ,a == b 返回0 ,a > b 返回1 。这里的比较方式是字典序! (3) strcpy(a, b) ,将字符串b 复制给从a 开始的字符数组。(将后面一个复制到前面的字符串上面)
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
char a[100] = "hello world!", b[100];
cout << strlen(a) << endl;
strcpy(b, a);
cout << strcmp(a, b) << endl;
return 0;
}
2.3 遍历字符数组中的字符:
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
char a[100] = "hello world!";
for (int i = 0; i < strlen(a); i ++ )
cout << a[i] << endl;
return 0;
}
题目:只出现一次的字符
题目链接 练习:给定一个只包含小写字母的字符串,请你找到第一个仅出现一次的字符。如果没有,输出no。 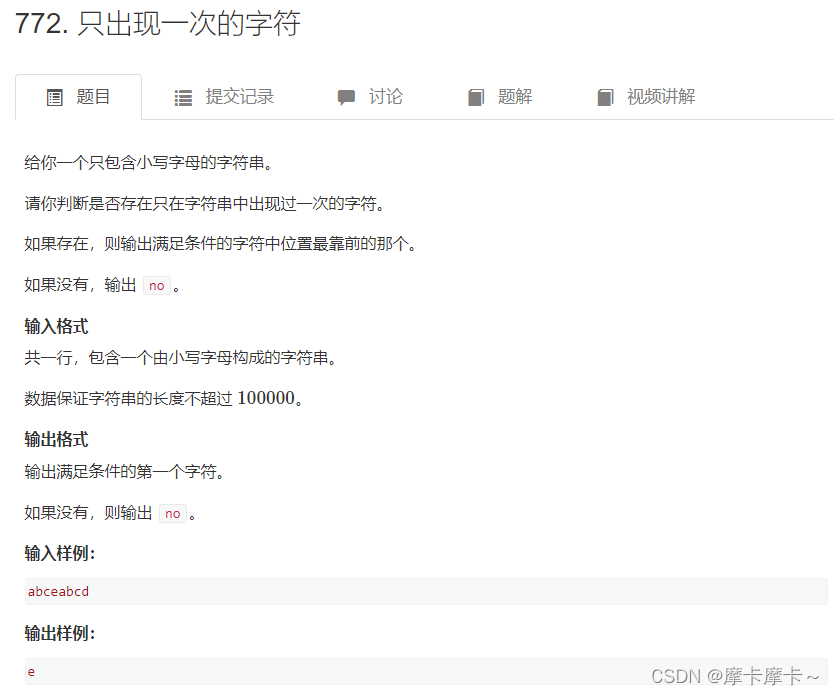 考点: 1、怎么读入这个字符串。 2、如何遍历这个字符串当中的每一个字符。 3、如何统计每个字符串的出现字符的次数。 写法一:27ms
#include<bits/stdc++.h>
using namespace std;
int a[30];
int main()
{
string s;
cin>>s;
for(int i=0,len=s.size();i<len;i++)
a[s[i]-'a']++;
bool f=1;
for(int i=0,len=s.size();i<len;i++)
if(a[s[i]-'a']==1)
{
f=0;
printf("%c",s[i]);
break;
}
if(f)printf("no");
return 0;
}
写法二:127ms
#include<iostream>
#include<cstring>
#include<map>
using namespace std;
int main()
{
string s;
cin>>s;
map<char,int>m;
for(int i=0;i<s.length();i++)
m[s[i]]++;
for(int i=0;i<s.length();i++)
if(m[s[i]]==1){cout<<s[i];return 0;}
cout<<"no";
return 0;
}
可知,上面的两个写法都是用一个思想,但是用的是两个容器储存的,一个是map,一个是数组,可知,数组运行的速度比map(STL)会快很多!
题目:替换字符
题目链接 练习:把一个字符串中特定的字符全部用给定的字符替换,得到一个新的字符串。 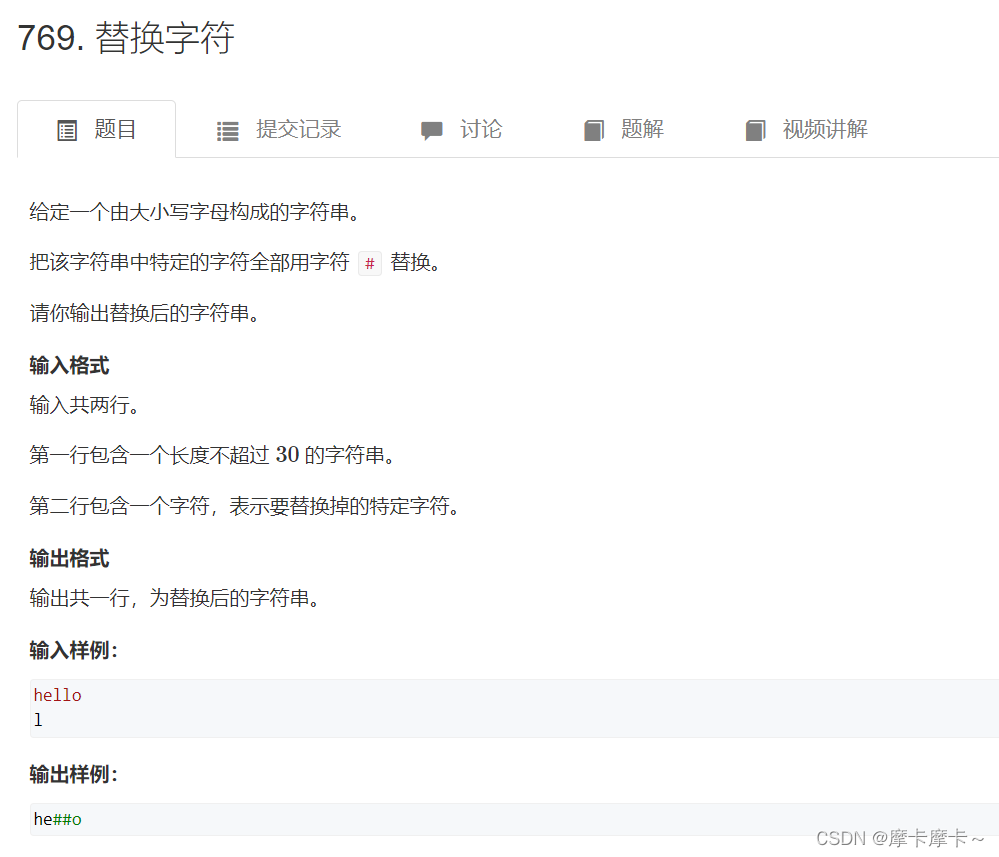
写法一:
#include<bits/stdc++.h>
using namespace std;
int main()
{
string s,k;
cin>>s>>k;
while(s.find(k)!=-1)
{
s=s.replace(s.find(k),1,"#");
}
cout<<s<<endl;
return 0;
}
写法二:
#include<bits/stdc++.h>
using namespace std;
int main()
{
char str[31];
scanf("%s",str);
char c;
scanf("\n%c",&c);
for(int i=0;str[i];i++)
if(str[i]==c)
str[i]='#';
puts(str);
return 0;
}
关于replace()函数
参考了这篇博客 用法一:用str替换指定字符串从起始位置pos开始长度为len的字符
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
str=str.replace(str.find("a"),2,"#");
cout<<str<<endl;
return 0;
}
运行结果: 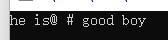
用法二: 用str替换 迭代器起始位置 和 结束位置 的字符
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
str=str.replace(str.begin(),str.begin()+5,"#");
cout<<str<<endl;
return 0;
}
 用法三: 用substr的指定子串(给定起始位置和长度)替换从指定位置上的字符串
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
string substr = "12345";
str=str.replace(0,5,substr,substr.find("1"),4);
cout << str << endl;
return 0;
}

用法四:string转char*时编译器可能会报出警告,不建议这样做 用str替换从指定位置0开始长度为5的字符串
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char * str1 = "12345";
str=str.replace(0,5,str1);
cout<<str<<endl;
return 0;
}
 用法五:string转char*时编译器可能会报出警告,不建议这样做 用str替换从指定迭代器位置的字符串
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char * str1 = "12345";
str=str.replace(str.begin(),str.begin()+6,str1);
cout<<str<<endl;
return 0;
}
 用法六:string转char*时编译器可能会报出警告,不建议这样做 用s的前n个字符替换从开始位置pos长度为len的字符串
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char * str1 = "12345";
str=str.replace(0,6,str1,4);
cout<<str<<endl;
return 0;
}
 用法七:string转char*时编译器可能会报出警告,不建议这样做 用s的前n个字符替换指定迭代器位置(从i1到i2)的字符串
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char * str1 = "12345";
str = str.replace(str.begin(),str.begin()+6,str1,4);
cout<<str<<endl;
return 0;
}
 用法八: 用重复n次的c字符替换从指定位置pos长度为len的内容
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char str1 = '#';
str = str.replace(0,6,3,str1);
cout<<str<<endl;
return 0;
}
 用法九: 用重复n次的c字符替换从指定迭代器位置(从i1开始到结束)的内容
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "he is@ a@ good boy";
char str1 = '#';
str = str.replace(str.begin(),str.begin()+6,3,str1);
cout<<str<<endl;
return 0;
}
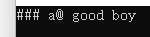
3. 标准库类型string
可变长的字符序列,比字符数组更加好用。需要引入头文件:
#include <string>
3.1 定义和初始化
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s1;
string s2 = s1;
string s3 = "hiya";
string s4(10, 'c');
return 0;
}
3.2 string上的操作
(1) string的读写:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s1, s2;
cin >> s1 >> s2;
cout << s1 << s2 << endl;
return 0;
}
注意:不能用printf直接输出string,需要写成:printf(“%s”, s.c_str());
(2) 使用getline读取一整行
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s;
getline(cin, s);
cout << s << endl;
return 0;
}
(3) string的empty和size操作(注意size是无符号整数,因此 s.size() <= -1一定成立):
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s1, s2 = "abc";
cout << s1.empty() << endl;
cout << s2.empty() << endl;
cout << s2.size() << endl;
return 0;
}
(4) string的比较:
支持 >, <, >=, <=, ==, !=等所有比较操作,按字典序进行比较。
(5) 为string对象赋值:
string s1(10, 'c'), s2;
s1 = s2;
(6) 两个string对象相加:
string s1 = "hello, "", s2 = "world\n";
string s3 = s1 + s2;
s1 += s2;
(7) 字面值和string对象相加:
做加法运算时,字面值和字符都会被转化成string对象,因此直接相加就是将这些字面值串联起来:
string s1 = "hello", s2 = "world";
string s3 = s1 + ", " + s2 + '\n';
当把string对象和字符字面值及字符串字面值混在一条语句中使用时,必须确保每个加法运算符的两侧的运算对象至少有一个是string:
string s4 = s1 + ", ";
string s5 = "hello" + ", ";
string s6 = s1 + ", " + "world";
string s7 = "hello" + ", " + s2;
3.3 处理string对象中的字符
可以将string对象当成字符数组来处理:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s = "hello world";
for (int i = 0; i < s.size(); i ++ )
cout << s[i] << endl;
return 0;
}
或者使用基于范围的for语句:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string s = "hello world";
for (char c: s) cout << c << endl;
for (char& c: s) c = 'a';
cout << s << endl;
return 0;
}
由于string里面的每一个字符都是char类型,所以我们就可以用auto,但是auto一个字符串的话,可能就会有问题。
题目:信息加密
题目链接 练习:密码翻译,输入一个只包含小写字母的字符串,将其中的每个字母替换成它的后继字母,如果原字母是’z’,则替换成’a’。 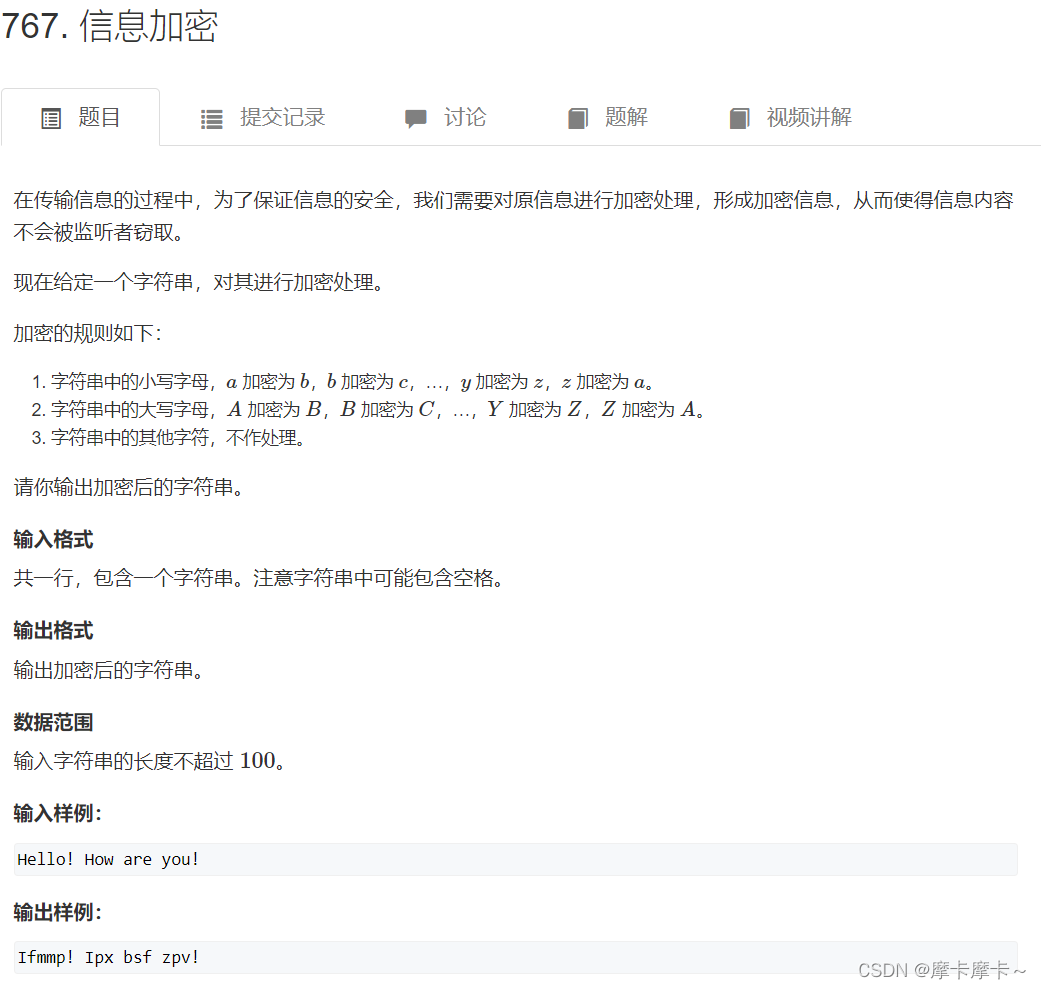 写法一:
#include<bits/stdc++.h>
using namespace std;
int main()
{
string s;
getline(cin,s);
for(int i=0;i<s.size();i++)
if(s[i]<'Z'&&s[i]>='A')
s[i]=s[i]+1;
else if(s[i]<'z'&&s[i]>='a')
s[i]=s[i]+1;
else if(s[i]=='Z')s[i]='A';
else if(s[i]=='z')s[i]='a';
cout<<s<<endl;
return 0;
}
写法二:
#include<bits/stdc++.h>
using namespace std;
int main()
{
string s;
getline(cin,s);
for(auto &c:s)
if(c>='a'&&c<='z')c=(c-'a'+1)%26+'a';
else if(c>='A'&&c<='Z')c=(c-'A'+1)%26+'A';
cout<<s<<endl;
return 0;
}
练习:输入两个字符串,验证其中一个串是否为另一个串的子串。
题目:字符串中的数字个数
题目链接 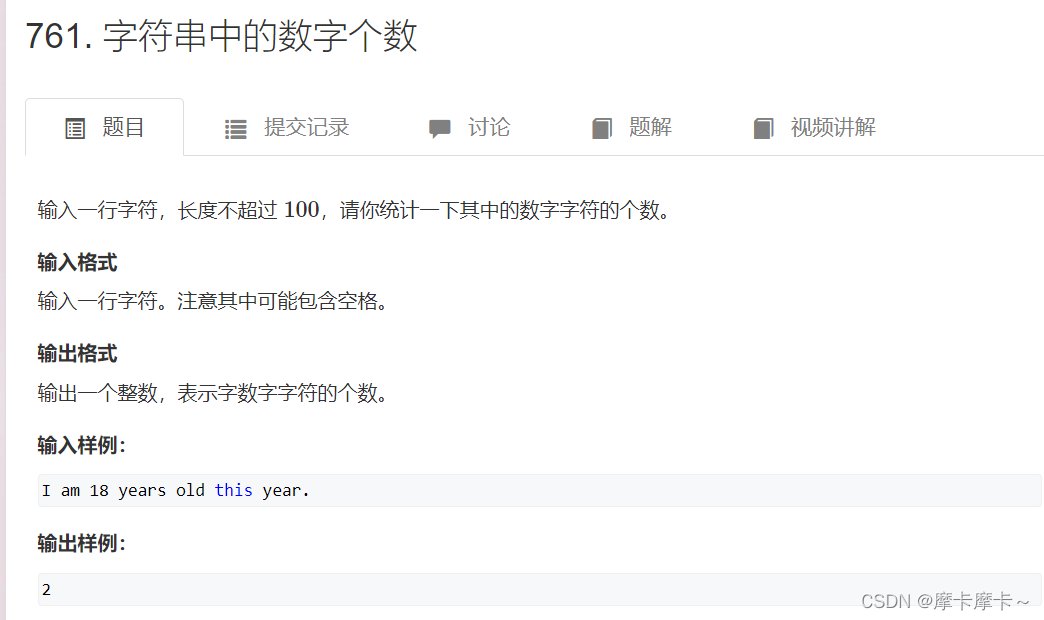 写着这道题其实是为了记录一下fgets()这个函数的用法。
#include<bits/stdc++.h>
using namespace std;
int main()
{
char str[101];
fgets(str,101,stdin);
int cnt=0;
for(int i=0;str[i];i++)
if(str[i]>='0'&&str[i]<='9')
cnt++;
printf("%d\n",cnt);
return 0;
}
题目:循环相克令(找规律,狗熊版石头剪刀布)
链接
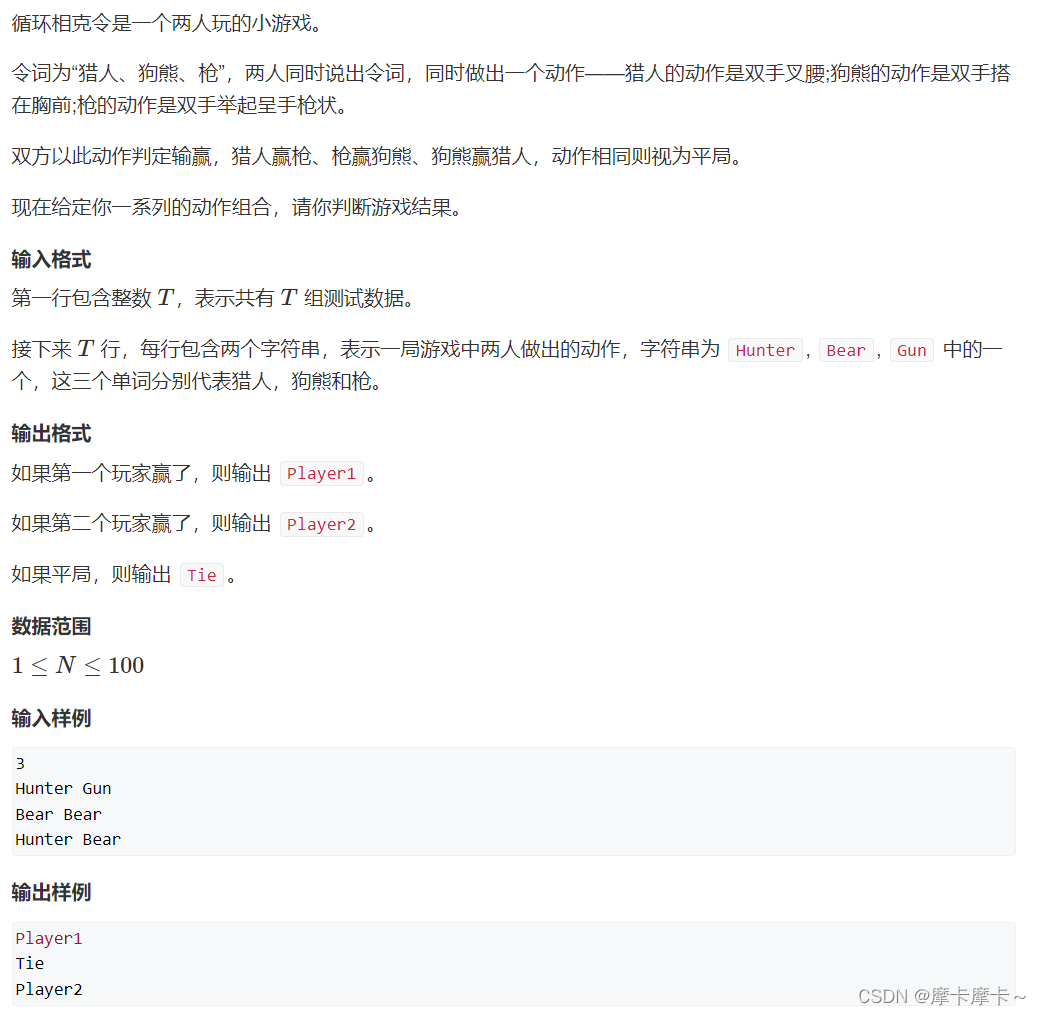 狗熊版的石头剪刀布 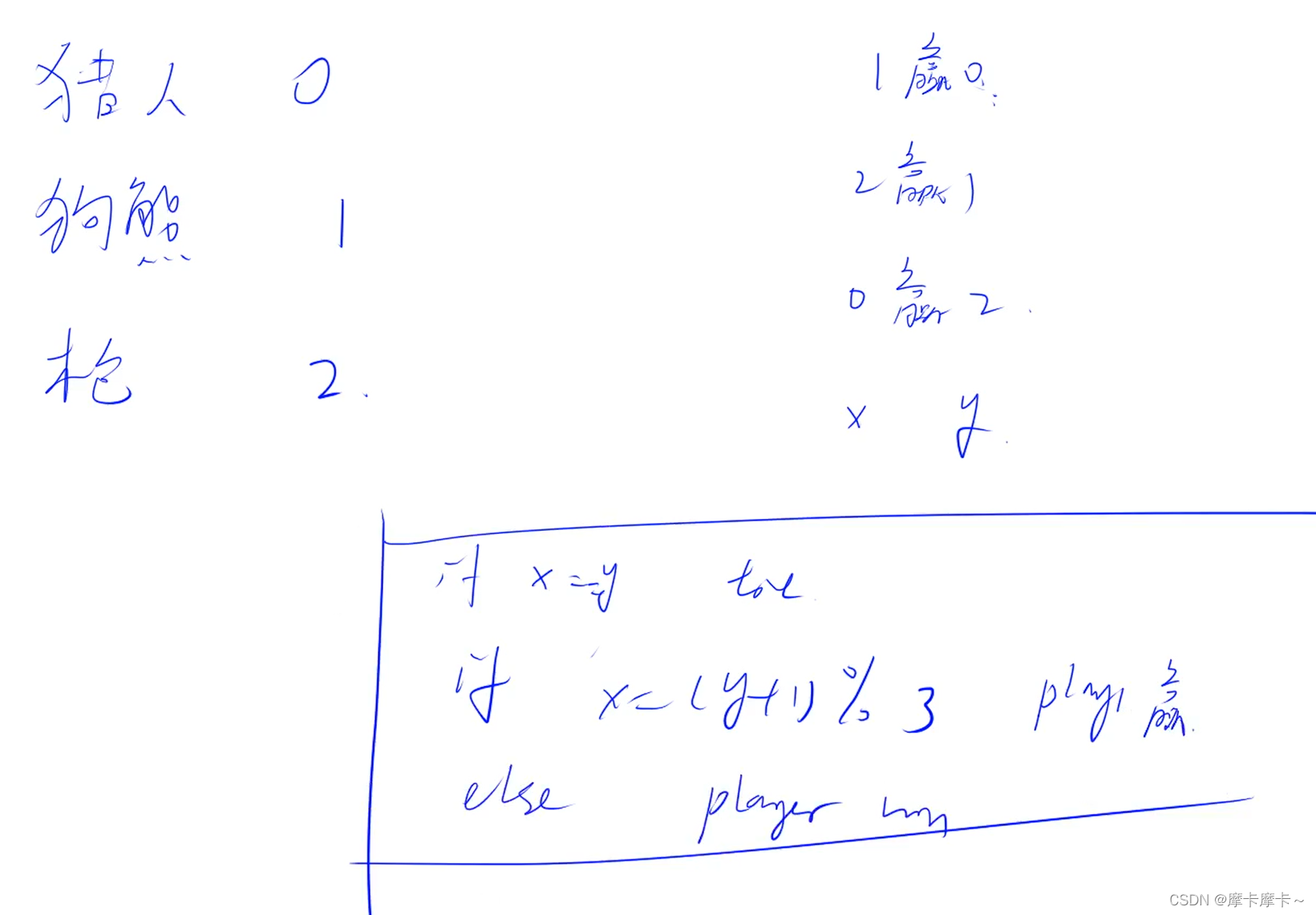
#include <cstdio>
#include <iostream>
using namespace std;
int main()
{
int n;
cin >> n;
while (n -- )
{
string a, b;
cin >> a >> b;
int x, y;
if (a == "Hunter") x = 0;
else if (a == "Bear") x = 1;
else x = 2;
if (b == "Hunter") y = 0;
else if (b == "Bear") y = 1;
else y = 2;
if (x == y) puts("Tie");
else if (x == (y + 1) % 3) puts("Player1");
else puts("Player2");
}
return 0;
}
若要删除字符串的最后一个字符,就要用s.pop_back() 若要将字符串中大写字符转化为小写字符,我们可以用函数tolower()
题目:字符串插入(substr()的应用)
题目链接 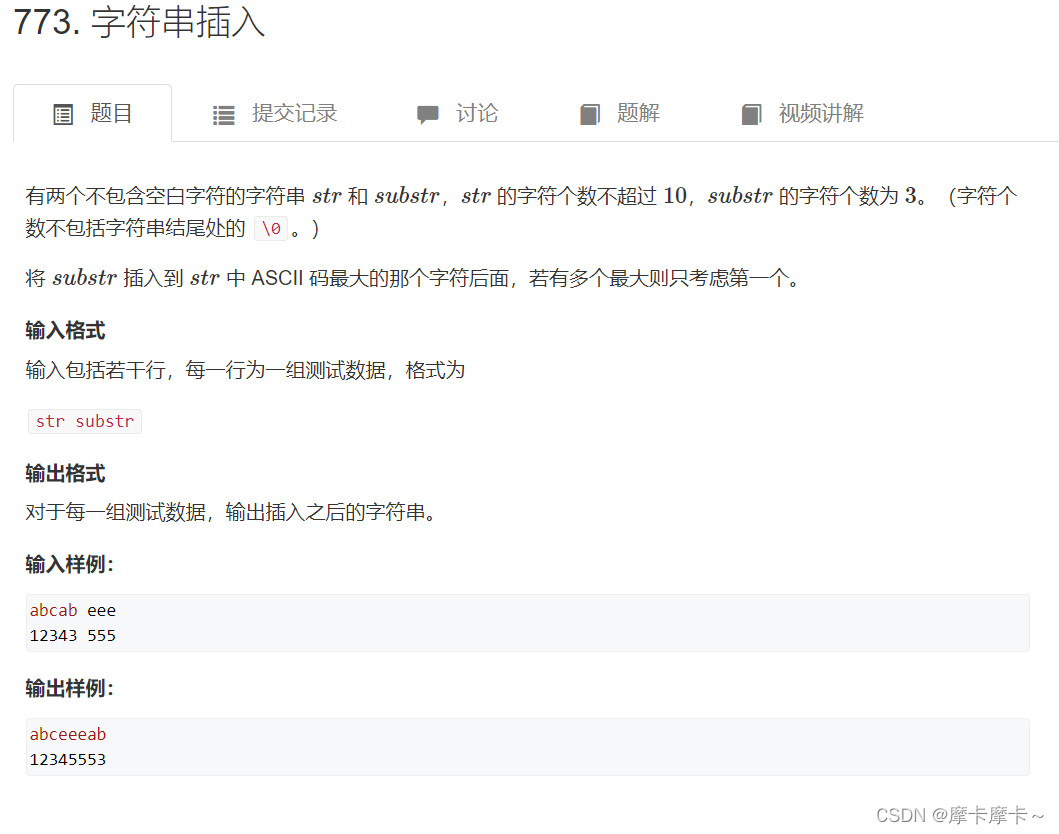
#include <iostream>
using namespace std;
int main()
{
string a, b;
while (cin >> a >> b)
{
int p = 0;
for (int i = 1; i < a.size(); i ++ )
if (a[i] > a[p])
p = i;
cout << a.substr(0, p + 1) + b + a.substr(p + 1) << endl;
}
return 0;
}
将字符串转化为全小写后,进行比较
#include<bits/stdc++.h>
using namespace std;
int main()
{
string a,b;
getine(cin,a);
getline(cin,b);
for(auto &c:a)c=tolower(c);
for(auto &c:b)c=tolower(c);
if(a==b)cout<<'='<<endl;
else if(a>b)cout<<'>'<<endl;
else cout<<'<'<<endl;
return 0;
}
题目:去掉多余的空格
题目链接 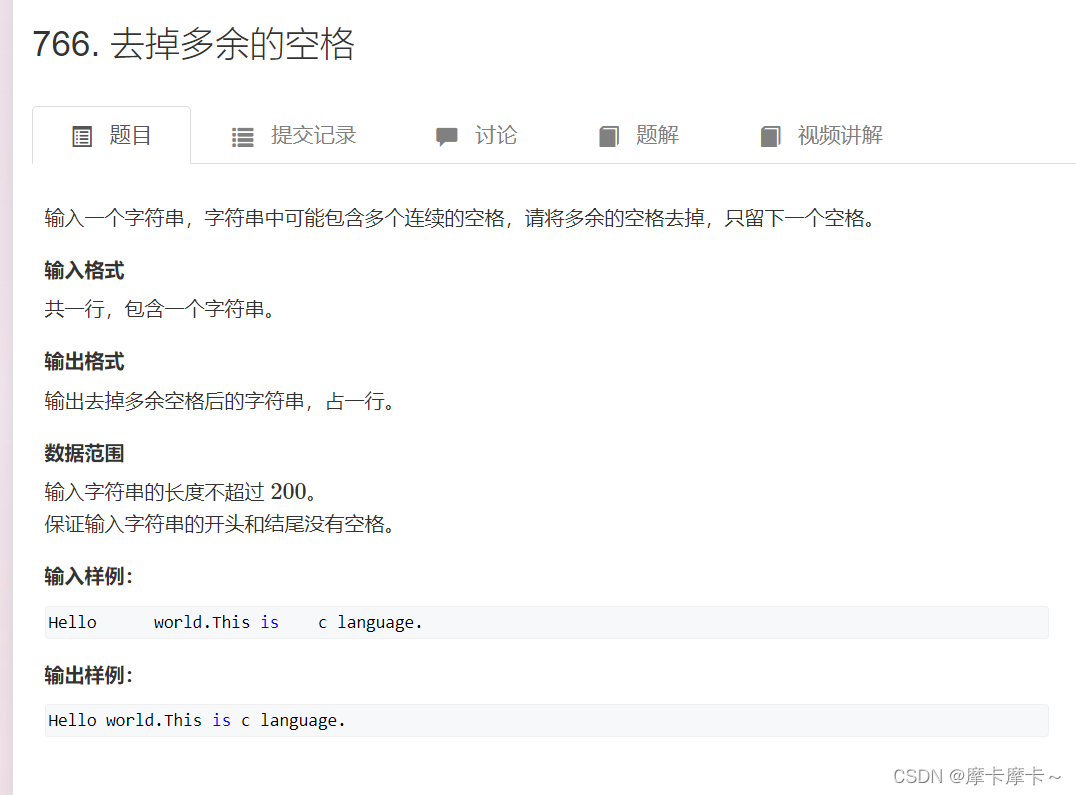
方法一:
#include <iostream>
using namespace std;
int main()
{
string s;
while (cin >> s) cout << s << ' ' ;
return 0;
}
方法二:第一类双指针算法
#include <iostream>
using namespace std;
int main()
{
string s;
getline(cin, s);
string r;
for (int i = 0; i < s.size(); i ++ )
if (s[i] != ' ') r += s[i];
else
{
r += ' ';
int j = i;
while (j < s.size() && s[j] == ' ') j ++ ;
i = j - 1;
}
cout << r << endl;
return 0;
}
写法三:局部性判断方法
#include <iostream>
using namespace std;
int main()
{
string s;
getline(cin, s);
string r;
for (int i = 0; i < s.size(); i ++ )
if (s[i] != ' ') r += s[i];
else
{
if (!i || s[i - 1] != ' ') r += ' ';
}
cout << r << endl;
return 0;
}
题目:单词替换
任意门 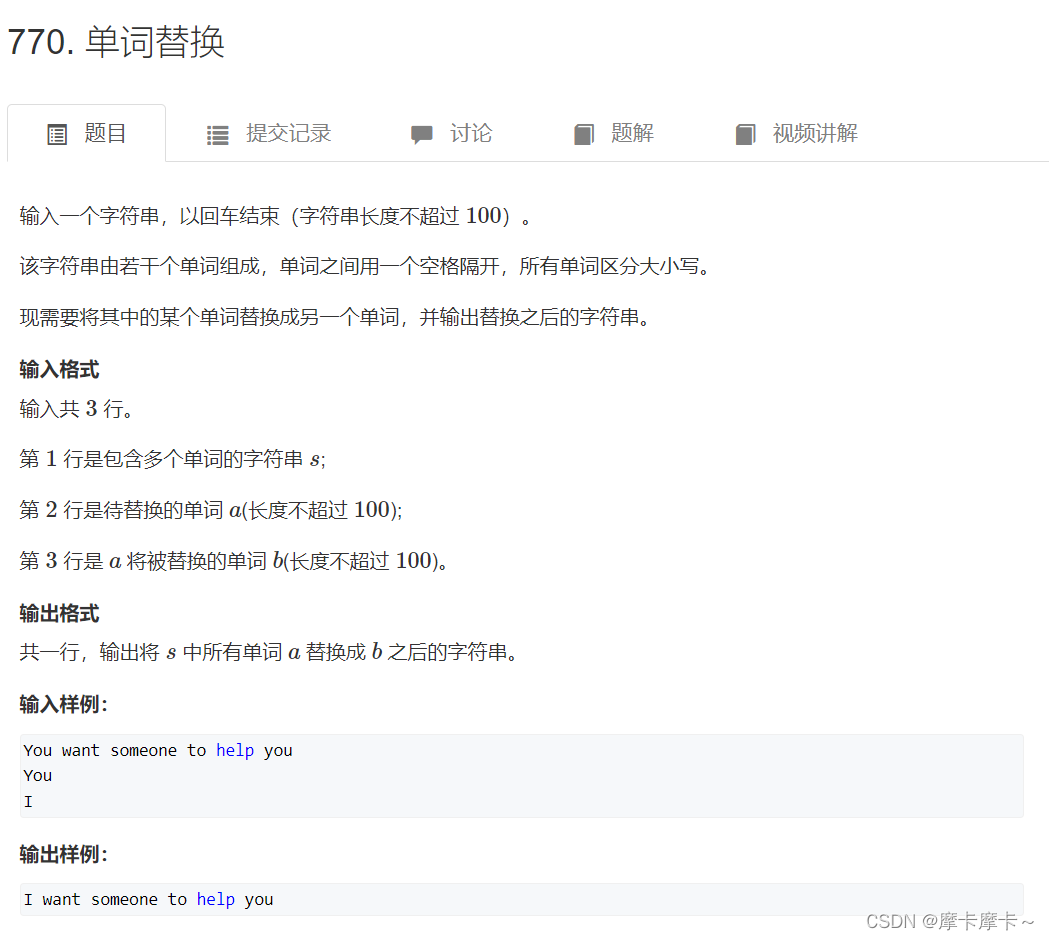
#include <iostream>
#include <sstream>
using namespace std;
int main()
{
string s, a, b;
getline(cin, s);
cin >> a >> b;
stringstream ssin(s);
string str;
while (ssin >> str)
if (str == a) cout << b << ' ';
else cout << str << ' ';
return 0;
}
题目:字符串中最长的连续出现的字符
题目链接 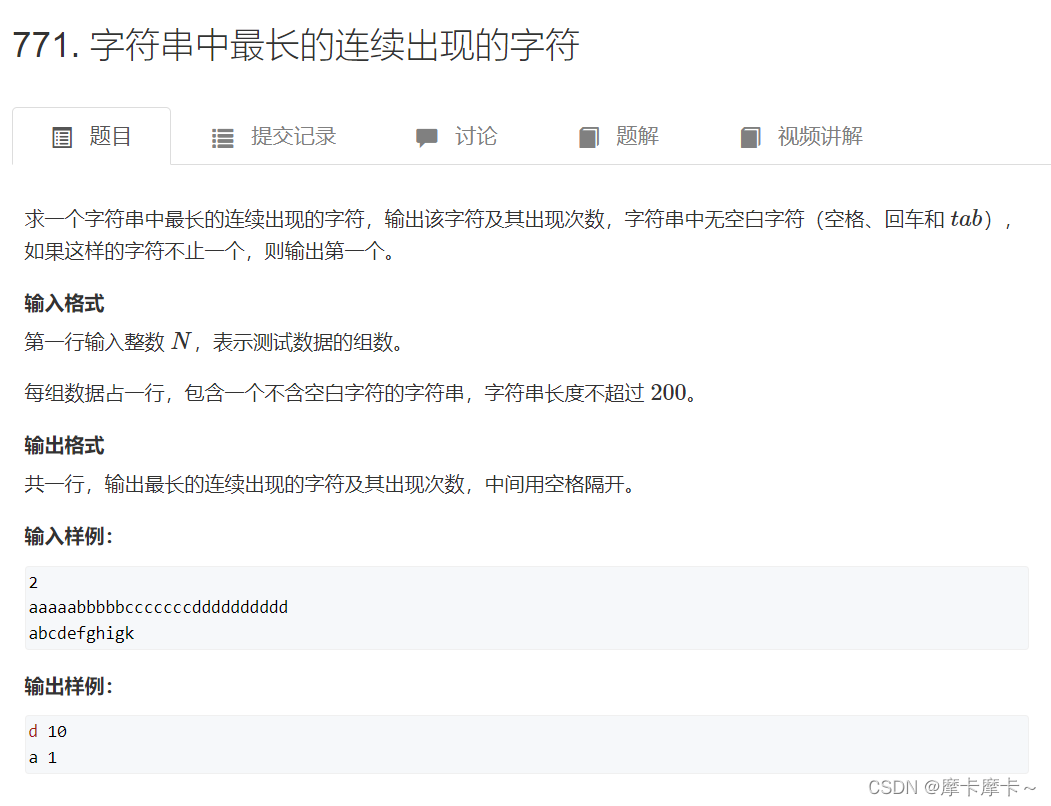 双指针算法!
#include <iostream>
using namespace std;
int main()
{
int n;
cin >> n;
while (n -- )
{
string str;
cin >> str;
int cnt = 0;
char c;
for (int i = 0; i < str.size(); i ++ )
{
int j = i;
while (j < str.size() && str[j] == str[i]) j ++ ;
if (j - i > cnt) cnt = j - i, c = str[i];
i = j - 1;
}
cout << c << ' ' << cnt << endl;
}
return 0;
}
题目:最长单词
题目链接 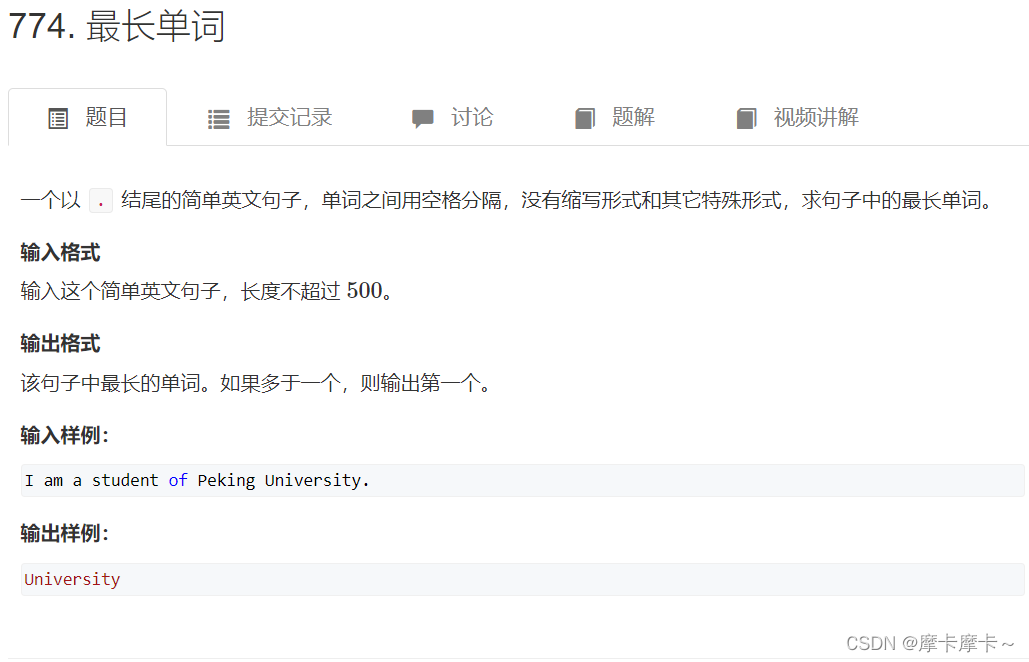
#include <iostream>
using namespace std;
int main()
{
string res, str;
while (cin >> str)
{
if (str.back() == '.') str.pop_back();
if (str.size() > res.size()) res = str;
}
cout << res << endl;
return 0;
}
题目:字符串移位包含问题
题目链接 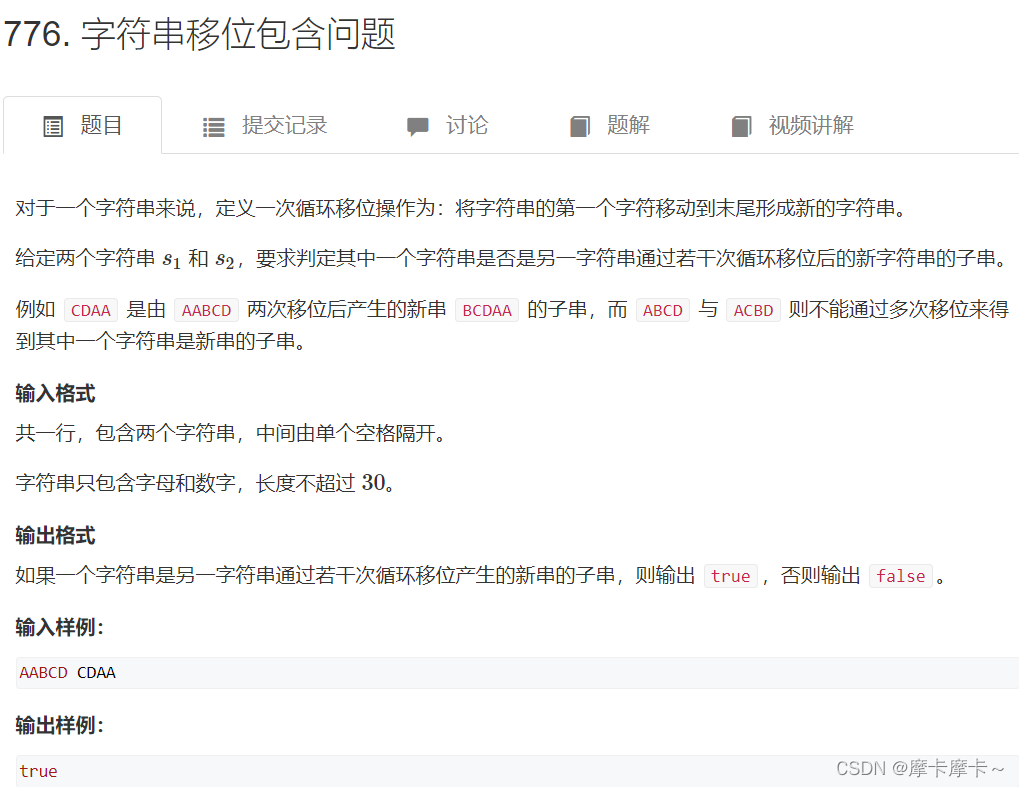
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
string a, b;
cin >> a >> b;
if (a.size() < b.size()) swap(a, b);
for (int i = 0; i < a.size(); i ++ )
{
a = a.substr(1) + a[0];
for (int j = 0; j + b.size() <= a.size(); j ++ )
{
int k = 0;
for (; k < b.size(); k ++ )
if (a[j + k] != b[k])
break;
if (k == b.size())
{
puts("true");
return 0;
}
}
}
puts("false");
return 0;
}
题目:字符串乘方(这道题目的思维超级赞)
任意门
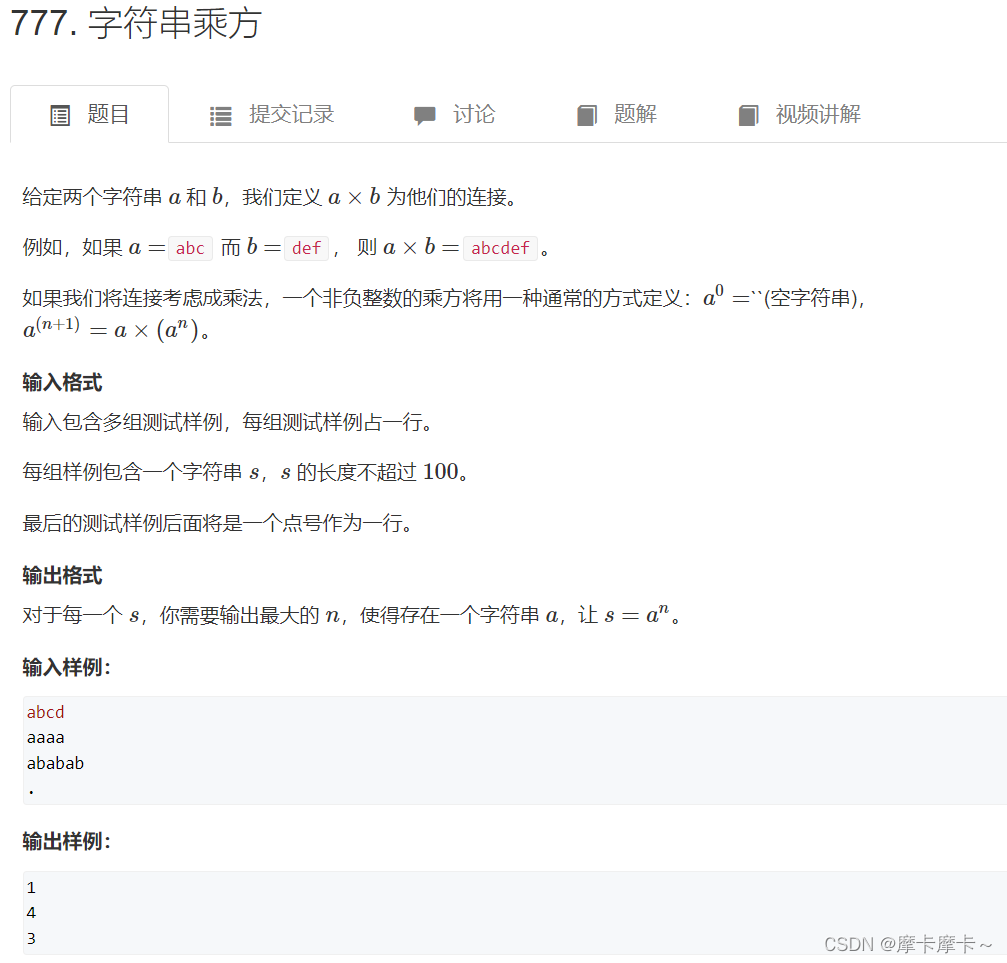
#include <iostream>
using namespace std;
int main()
{
string str;
while (cin >> str, str != ".")
{
int len = str.size();
for (int n = len; n; n -- )
if (len % n == 0)
{
int m = len / n;
string s = str.substr(0, m);
string r;
for (int i = 0; i < n; i ++ ) r += s;
if (r == str)
{
cout << n << endl;
break;
}
}
}
return 0;
}
题目:最长公共字符串后缀
任意门 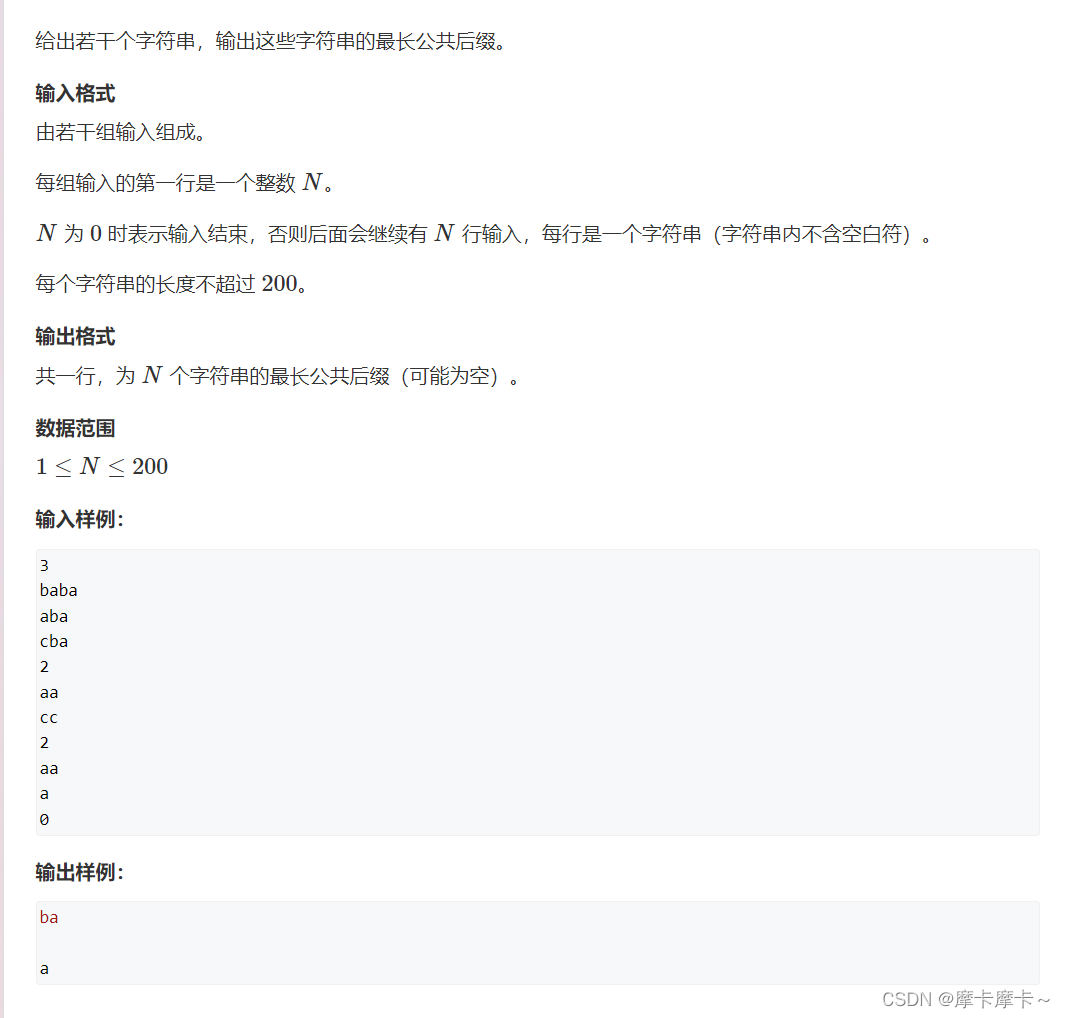
#include <iostream>
using namespace std;
const int N = 200;
int n;
string str[N];
int main()
{
while (cin >> n, n)
{
int len = 1000;
for (int i = 0; i < n; i ++ )
{
cin >> str[i];
if (len > str[i].size()) len = str[i].size();
}
while (len)
{
bool success = true;
for (int i = 1; i < n; i ++ )
{
bool is_same = true;
for (int j = 1; j <= len; j ++ )
if (str[0][str[0].size() - j] != str[i][str[i].size() - j])
{
is_same = false;
break;
}
if (!is_same)
{
success = false;
break;
}
}
if (success) break;
len -- ;
}
cout << str[0].substr(str[0].size() - len) << endl;
}
return 0;
}
|