内容: 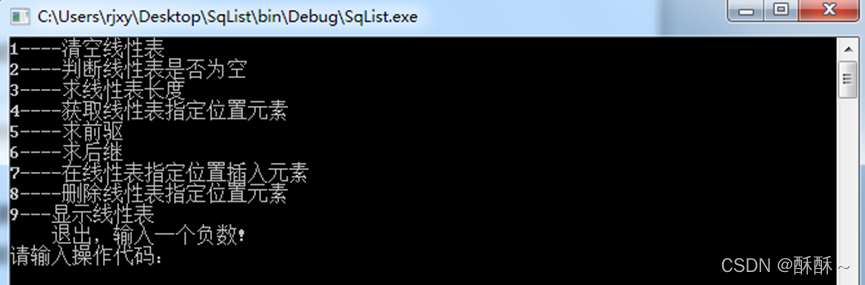
实现思路: ① 对数据进行定义 ② 在主函数中定义顺序表 ③ 分别编写顺序表的初始化、清空、判断是否为空、获取长度、获取指定位置元素、求前驱、求后继、元素定位、插入元素、删除指定位置元素、显示等操作函数 ④ 初始化顺序表 ⑤ 根据指令选项判断需要执行的指令 ⑥ 接受退出指令退出 ⑦ 程序退出
程序代码:
#include <iostream>
using namespace std;
const int size=20;
typedef int ElemType;
typedef struct
{
ElemType *elem;
int length;
}SqList;
int InitList(SqList *L)
{
L->elem=new ElemType[size];
if(!L->elem)
return 0;
L->length=0;
return 1;
}
void DestoryList(SqList *L)
{
L->length=0;
}
bool ListEmpty(SqList L)
{
if(L.length==0)
return true;
else
return false;
}
int ListLength(SqList L)
{
return L.length;
}
int GetElem(SqList L,int i,ElemType *e)
{
if(i<1||i>L.length)
return 0;
*e=L.elem[i-1];
return 1;
}
int PriorElem(SqList L,ElemType cur_e,ElemType *pre_er)
{
int flag=0;
for(int i=0;i<L.length;i++)
{
if(L.elem[i]==cur_e&&i!=0)
{
*pre_er=L.elem[i-1];
flag=1;
}
}
if(flag==1)
return 1;
else
return 0;
}
int NextElem(SqList L,ElemType cur_e,ElemType *next_e)
{
int flag=0;
for(int i=0;i<L.length;i++)
{
if(L.elem[i]==cur_e&&i!=L.length-1)
{
*next_e=L.elem[i-1];
flag=1;
}
}
if(flag==1)
return 1;
else
return 0;
}
int ListInsert(SqList *L,int i,ElemType e)
{
if(i<1 || i>L->length+1 || L->length==size)
return 0;
for(int j=L->length-1;j>=i-1;j--)
{
L->elem[j+1]=L->elem[j];
}
L->elem[i-1]=e;
L->length++;
return 1;
}
int ListDelete(SqList *L,int i)
{
if(i<1 || i>L->length)
return 0;
for(int j=i;j<L->length;j++)
{
L->elem[j-1]=L->elem[j];
}
L->length--;
return 1;
}
void ShowList(SqList L)
{
for(int i=0;i<L.length;i++)
cout<<L.elem[i]<<" ";
cout<<endl;
}
int main()
{
cout<<"1----清空线性表"<<endl;
cout<<"2----判断线性表是否为空"<<endl;
cout<<"3----求线性表的长度"<<endl;
cout<<"4----获取线性表指定位置的元素"<<endl;
cout<<"5----求前驱"<<endl;
cout<<"6----求后继"<<endl;
cout<<"7----在线性表指定位置插入元素"<<endl;
cout<<"8----删除线性表指定位置元素"<<endl;
cout<<"9----显示线性表"<<endl;
cout<<" 退出,输入一个负数!"<<endl;
SqList L;
int flag=InitList(&L);
if(flag==0)
cout<<"线性表初始化失败!"<<endl;
int IP;
while(1)
{
cout<<endl<<endl;
cout<<"====================================="<<endl;
cout<<"请输入操作码:"<<endl;
cin>>IP;
if(IP<0)
break;
else if(IP==1)
{
DestoryList(&L);
cout<<"线性表清空成功"<<endl;
}
else if(IP==2)
{
if(ListEmpty(L))
{
cout<<"线性表为空"<<endl;
}
else
cout<<"线性表不为空"<<endl;
}
else if(IP==3)
{
cout<<"线性表的长度为:"<<ListLength(L)<<endl;
}
else if(IP==4)
{
cout<<"请输入你想要获取的元素的位置:"<<endl;
int i;
ElemType e;
cin>>i;
int Flag=GetElem(L,i,&e);
if(Flag)
cout<<"线性表第"<<i<<"个元素为"<<e<<endl;
else
cout<<"输入元素的位置错误,查找失败!"<<endl;
}
else if(IP==5)
{
cout<<"请输入需要求前驱的元素:"<<endl;
ElemType cur_e;
cin>>cur_e;
ElemType pre_e;
int Flag=PriorElem(L,cur_e,&pre_e);
if(Flag)
cout<<"元素"<<cur_e<<"的前驱为"<<pre_e<<endl;
else
cout<<"元素"<<cur_e<<"不在线性表中或者不存在前驱!"<<endl;
}
else if(IP==6)
{
cout<<"请输入需要求后继的元素:"<<endl;
ElemType cur_e;
cin>>cur_e;
ElemType next_e;
int Flag=NextElem(L,cur_e,&next_e);
if(Flag)
cout<<"元素"<<cur_e<<"的前驱为"<<next_e<<endl;
else
cout<<"元素"<<cur_e<<"不在线性表中或者不存在前驱!"<<endl;
}
else if(IP==7)
{
cout<<"请输入要插入的数据的位置和值,以空格隔开:"<<endl;
int i;
ElemType e;
cin>>i>>e;
int Flag=ListInsert(&L,i,e);
if(Flag)
cout<<"插入成功!"<<endl;
else
cout<<"插入失败,输入的位置不合法!"<<endl;
}
else if(IP==8)
{
cout<<"请输入要删除元素的位置:"<<endl;
int i;
cin>>i;
int Flag=ListDelete(&L,i);
if(Flag)
cout<<"删除成功!"<<endl;
else
cout<<"删除失败,输入的元素位置不合法!"<<endl;
}
else if(IP==9)
{
cout<<"线性表的元素为:";
ShowList(L);
}
}
return 0;
}
|