普利姆算法
一、问题引出
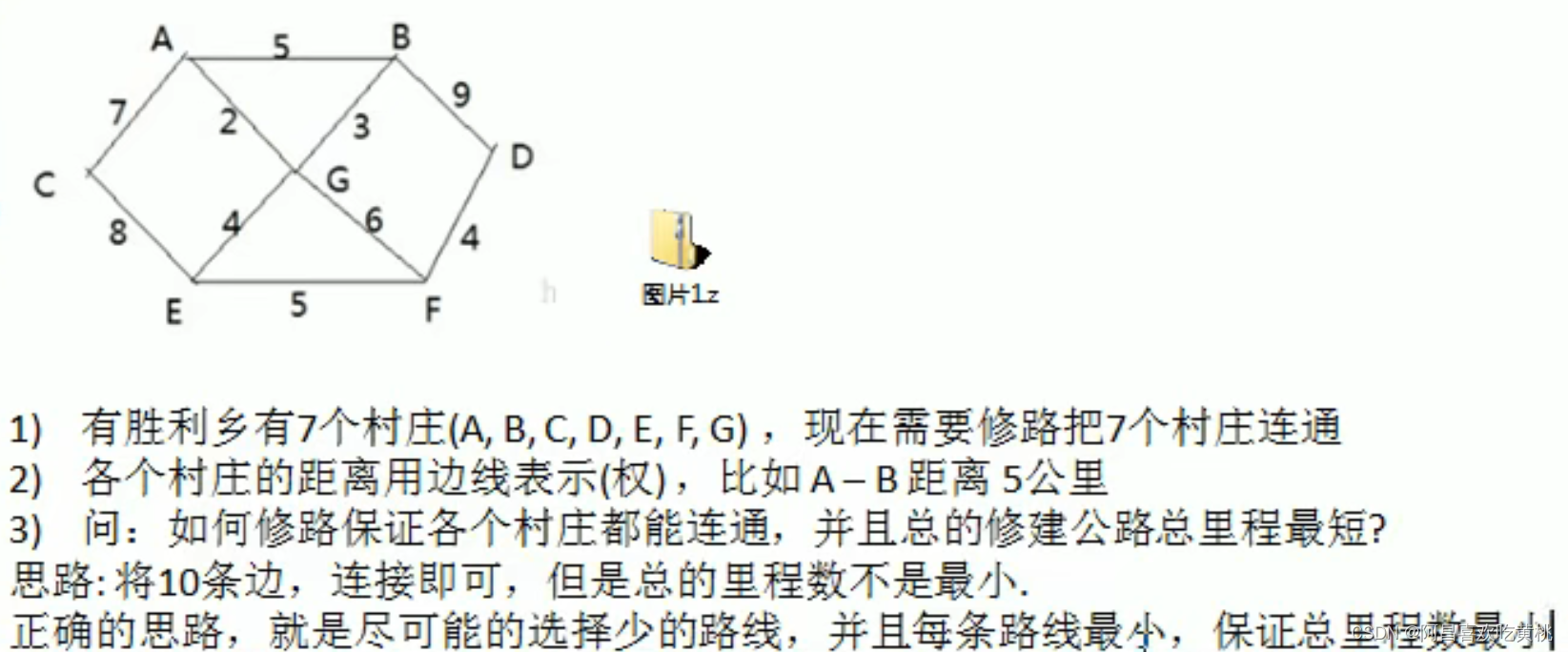
二、最小生成树
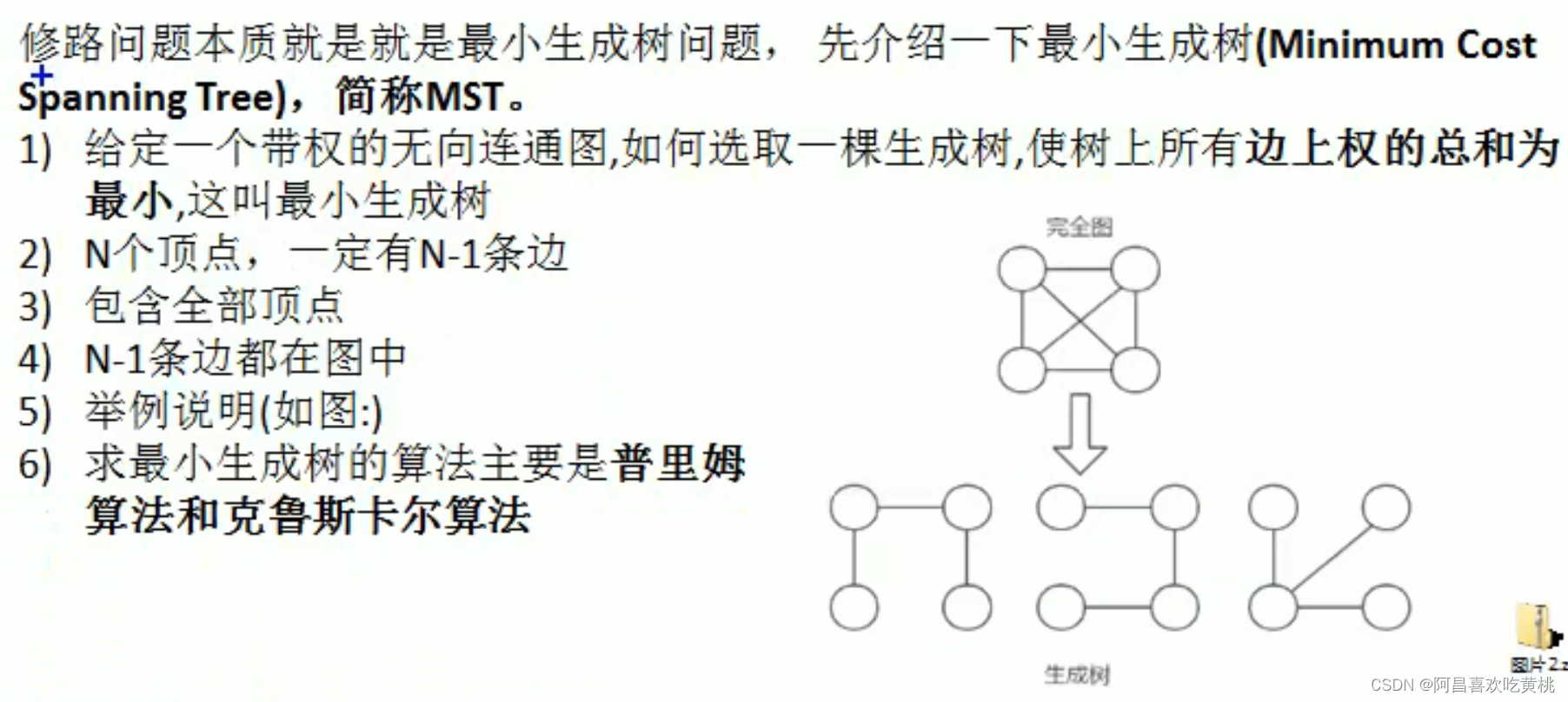
三、普利姆算法介绍
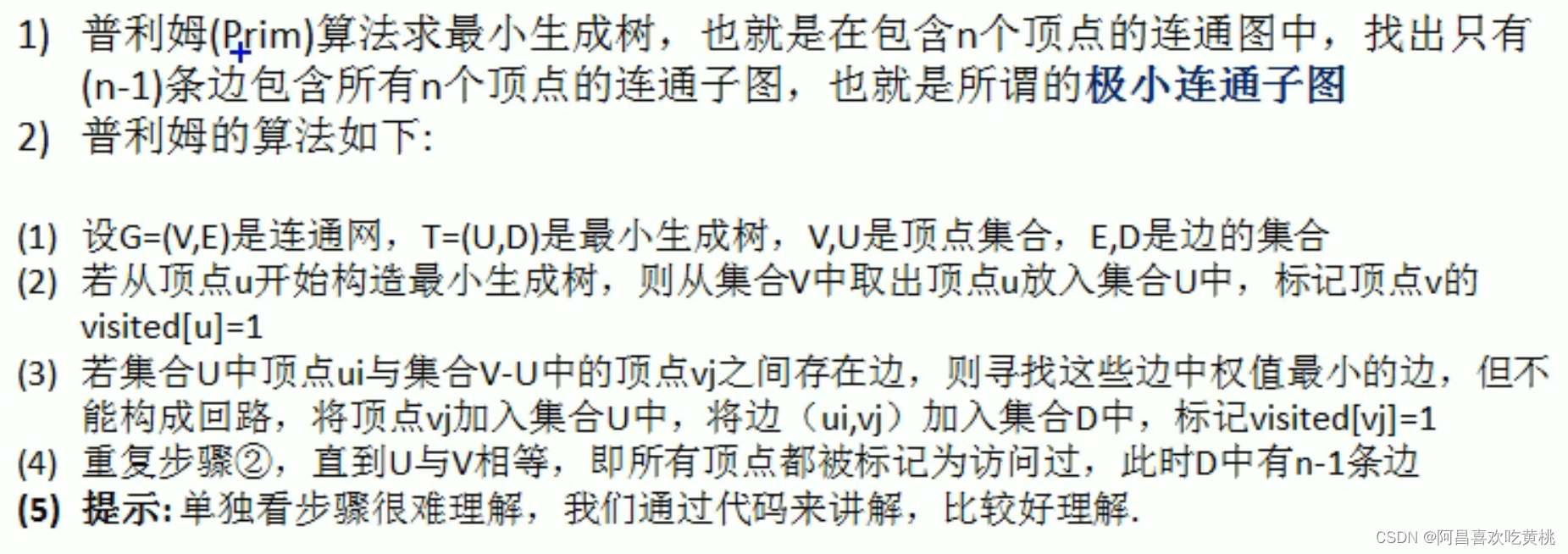
四、图解分析
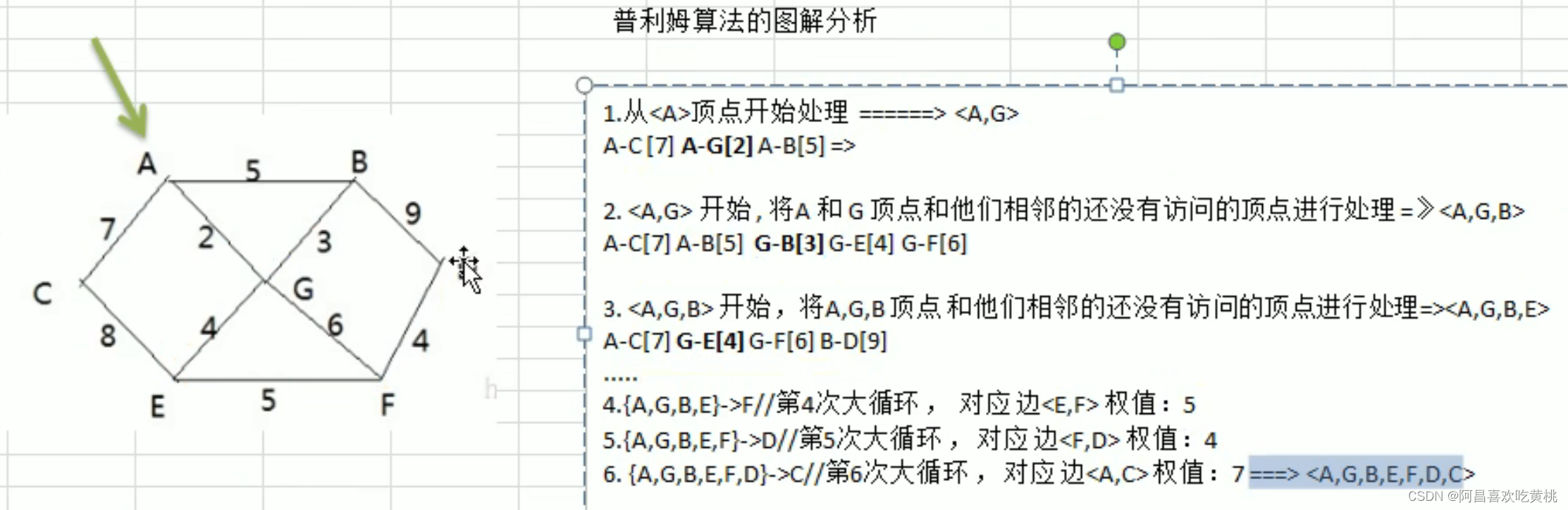
五、代码实现
package com.achang.algorithm;
import java.util.Arrays;
public class PrimAlgorithm {
public static void main(String[] args) {
char[] data = {'a','b','c','d','e','f','g'};
int[][] weight = new int[][]{
{10000,5,7,10000,10000,10000,2},
{5,10000,10000,9,10000,10000,3},
{7,10000,10000,10000,8,10000,10000},
{10000,9,10000,10000,10000,4,10000},
{10000,10000,8,10000,10000,5,4},
{10000,10000,10000,4,5,10000,6},
{2,3,10000,10000,4,6,10000},
};
Graph graph = new Graph(data.length);
MinTree minTree = new MinTree();
minTree.createGraph(graph,
data.length,
data,
weight);
minTree.list(graph);
minTree.prim(graph,0);
}
}
class MinTree{
public void createGraph(Graph graph,int verxs,char[] data,int[][] weight){
int i,j;
for (i = 0; i < verxs; i++) {
graph.data[i] = data[i];
for (j = 0; j < verxs;j++){
graph.weight[i][j] = weight[i][j];
}
}
}
public void list(Graph graph){
for (int[] ints : graph.weight) {
System.out.println(Arrays.toString(ints));
}
}
public void prim(Graph graph,int v){
int[] visited = new int[graph.verxs];
visited[v] = 1;
int h1 = -1;
int h2 = -1;
int minWeight = 10000;
for (int i = 1; i < graph.verxs; i++) {
for (int j = 0; j < graph.verxs; j++) {
for (int k = 0; k < graph.verxs; k++) {
if (visited[j]==1 && visited[k] == 0 && graph.weight[j][k] < minWeight){
minWeight = graph.weight[j][k];
h1 = j;
h2 = k;
}
}
}
System.out.println("边<"+graph.data[h1]+","+graph.data[h2]+">权值:"+minWeight);
visited[h2] = 1;
minWeight = 10000;
}
}
}
class Graph{
int verxs;
char[] data;
int[][] weight;
public Graph(int size){
this.verxs = size;
data = new char[verxs];
weight = new int[verxs][verxs];
}
}
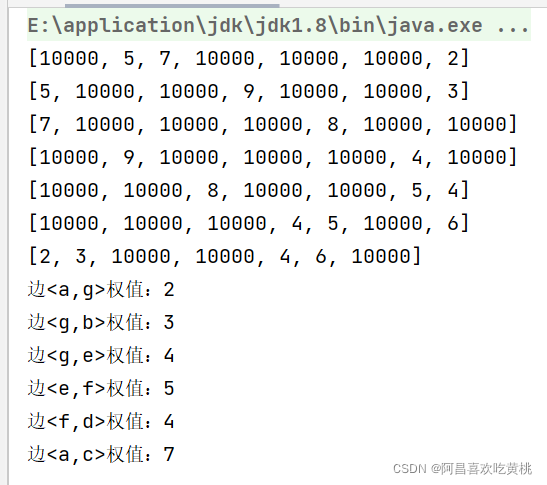
|