题目:
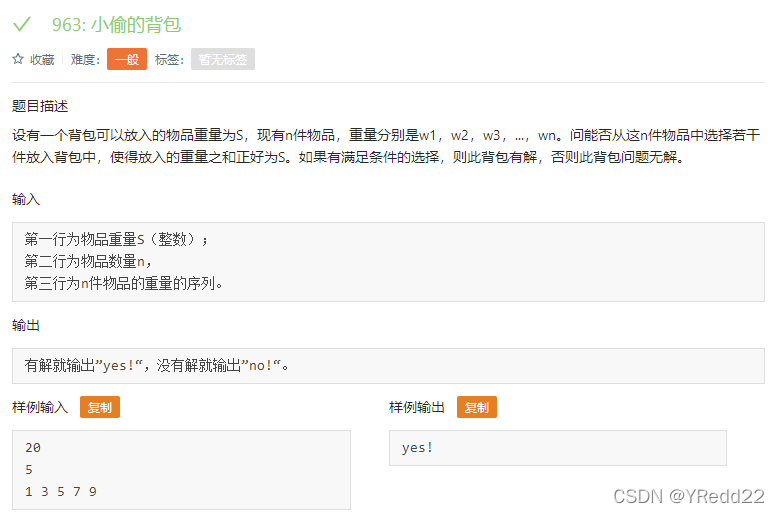
思路:
此题就是将题目所给的数据进行入栈,大家可以从题目给的例子中发现,这是一个升序序列,入栈过后最大的数就在栈顶,每一次取出来的都是栈中最大的,每一次进行相加,如果加到大于s,那么就舍弃这个栈顶元素,出栈一次,继续循环加下面的元素,直到栈为空为止。
代码: ?
#include<iostream>
using namespace std;
typedef struct Stack
{
int* data;
int top;
int capacity;
}ST;//创建栈的结构体
void STInit(ST* ps)
{
ps->data = NULL;
ps->top = 0;
ps->capacity = 0;
}//栈的初始化
bool IsEmpty(ST* ps)
{
return ps->top == 0;
}//判断栈是否为空
int STTop(ST* ps)
{
return ps->data[ps->top - 1];
}//栈顶元素
void STPush(ST* ps,int x)
{
if (ps->top == ps->capacity)
{
int newCapacity = ps->capacity == 0 ? 4 : ps->capacity * 2;
int* tmp = (int*)realloc(ps->data, sizeof(int)*newCapacity);
if (tmp == NULL)
{
exit(-1);
}
ps->data = tmp;
ps->capacity = newCapacity;
}
ps->data[ps->top] = x;
ps->top++;
}//入栈操作
void STCreate(ST* ps)
{
int n = 0;
cin >> n;
int x = 0;
while (n)
{
cin >> x;
STPush(ps, x);
n--;
}
}//栈的创建
void STPop(ST* ps)
{
ps->top--;
}//出栈操作
bool STPut(ST* ps,int s)
{
int sum = 0;//sum记录重量总和
int flag = 0;//记录是否是由于栈为空结束的循环
while (sum != s && !IsEmpty(ps))
{
sum += STTop(ps);
if (sum == s)
{
flag = 1;
return true;
}
if (sum > s)//sum比s大则减去这个元素
{
sum -= STTop(ps);
}
STPop(ps);//把栈顶元素出栈
}
if (flag == 0)//如果是因为栈为空结束的循环就返回假
{
return false;
}
}
int main()
{
ST p;
STInit(&p);
int s = 0;
cin >> s;
STCreate(&p);
if (STPut(&p, s))
{
printf("yes!");
}
else
{
printf("no!");
}
return 0;
}
|