1. 剑指 Offer 32 - I. 从上到下打印二叉树
原题链接
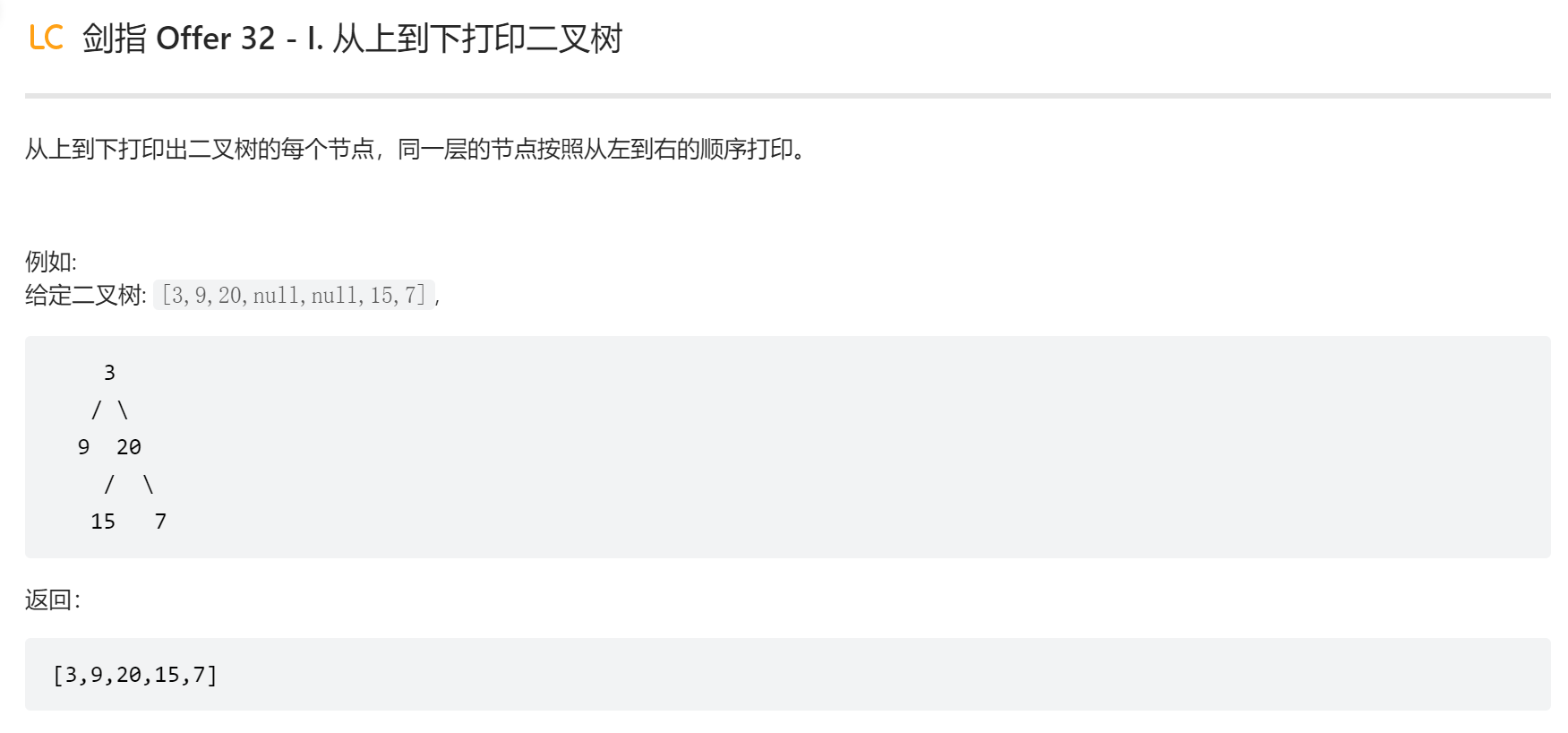 二叉树的广度优先遍历
var levelOrder = function(root) {
if(!root) return [];
const q = [root];
const res = [];
while(q.length){
let node = q.shift();
res.push(node.val);
node.left && q.push(node.left);
node.right && q.push(node.right);
}
return res;
}
2. 剑指 Offer 32 - II. 从上到下打印二叉树 II
原题链接 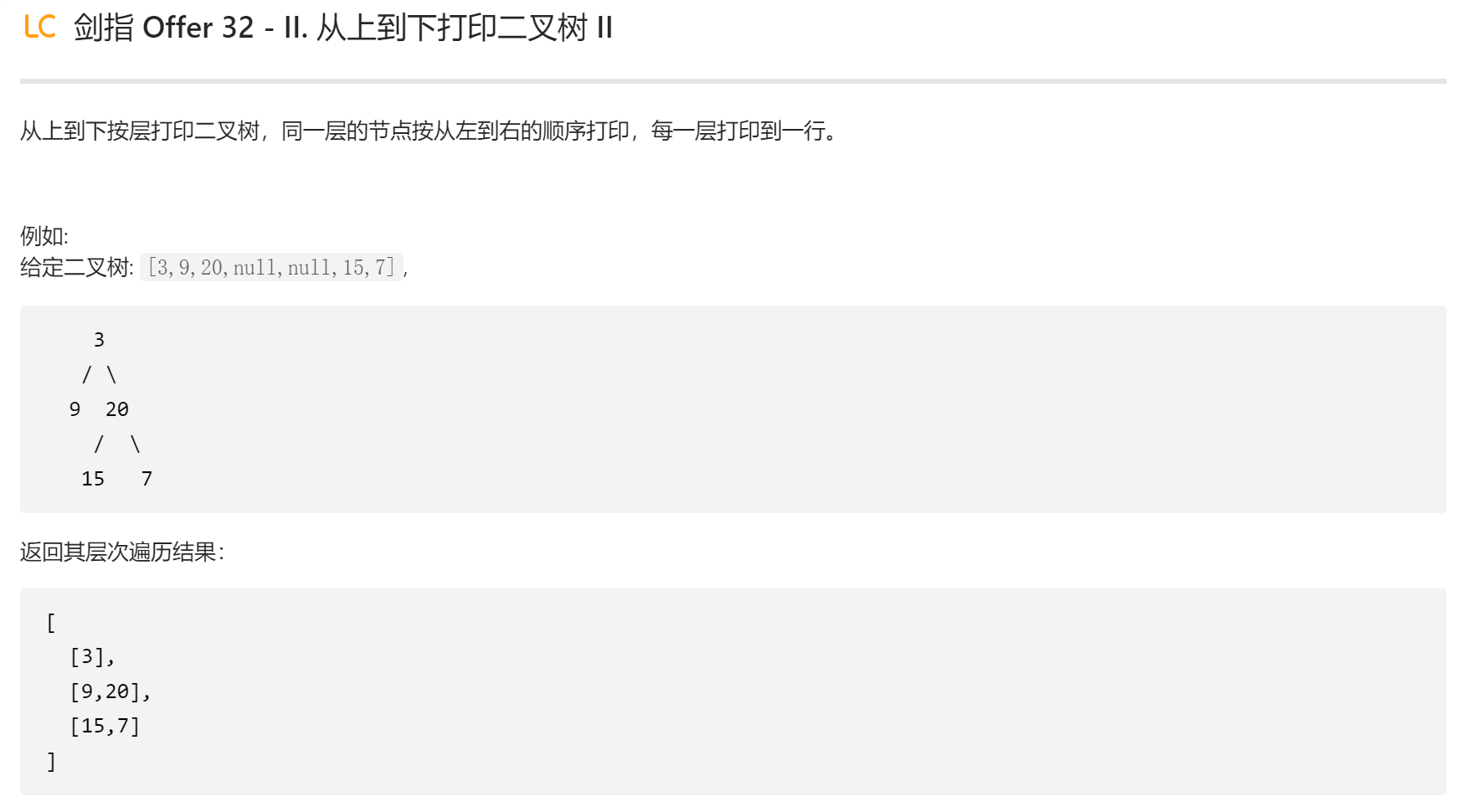 二叉树的层序遍历、双重循环
var levelOrder = function(root) {
if(!root) return [];
const q = [root];
const res = [];
while(q.length){
const tmp = [];
for(let i = q.length; i>0; i--){
let node = q.shift();
tmp.push(node.val);
node.left && q.push(node.left);
node.right && q.push(node.right);
}
res.push(tmp);
}
return res;
}
3. 剑指 Offer 32 - III. 从上到下打印二叉树 III
原题链接 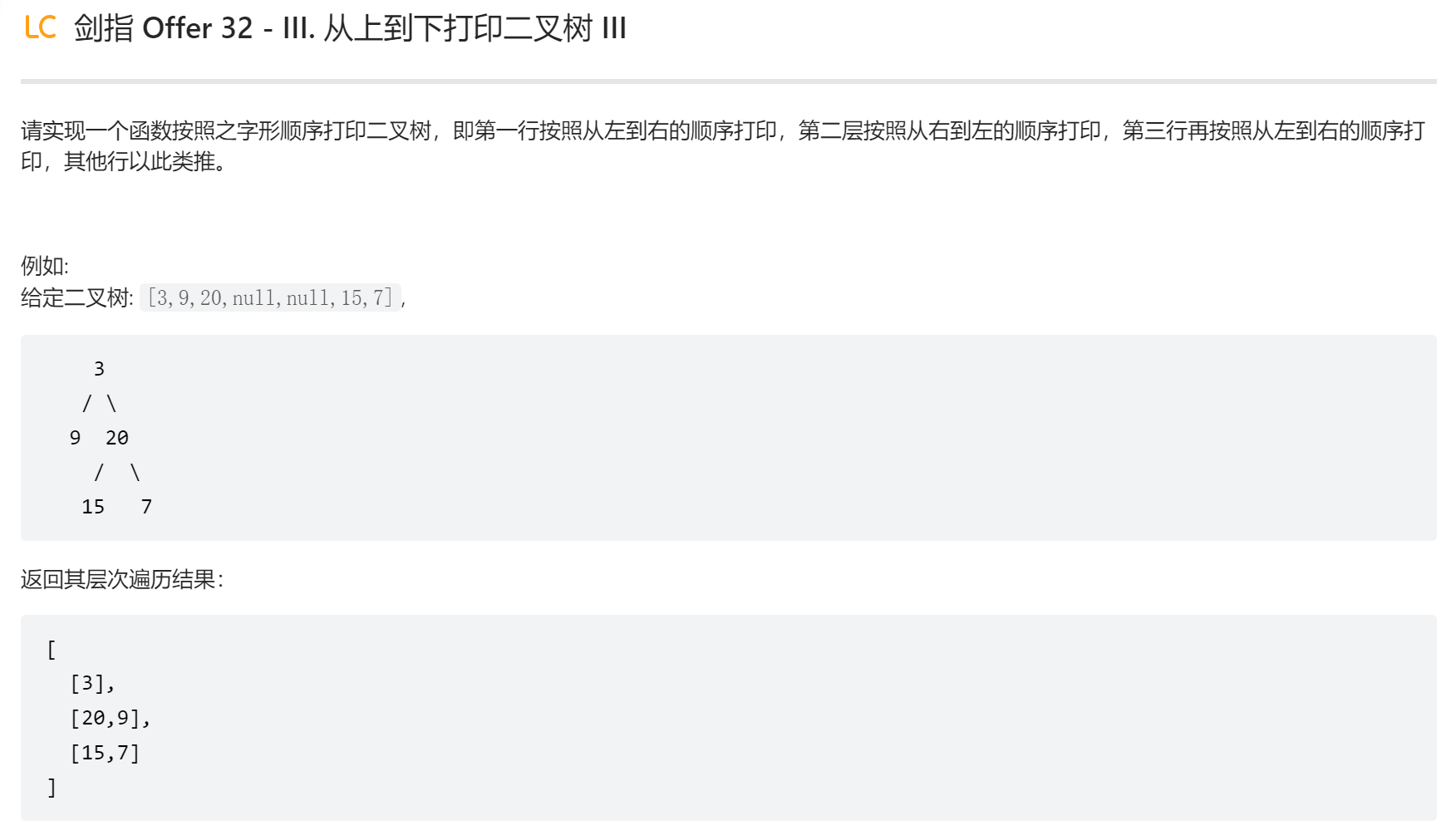 要求打印顺序交替变化
var levelOrder = function(root) {
if(!root) return [];
const q = [root];
const res = [];
let flag = 0;
while(q.length){
const tmp = [];
for(let i=q.length; i>0; i--){
let node = q.shift();
if(flag%2===0)tmp.push(node.val);
else tmp.unshift(node.val);
node.left && q.push(node.left);
node.right && q.push(node.right);
}
flag++;
res.push(tmp);
}
return res;
}
4. 剑指 Offer 34. 二叉树中和为某一值的路径
原题链接
 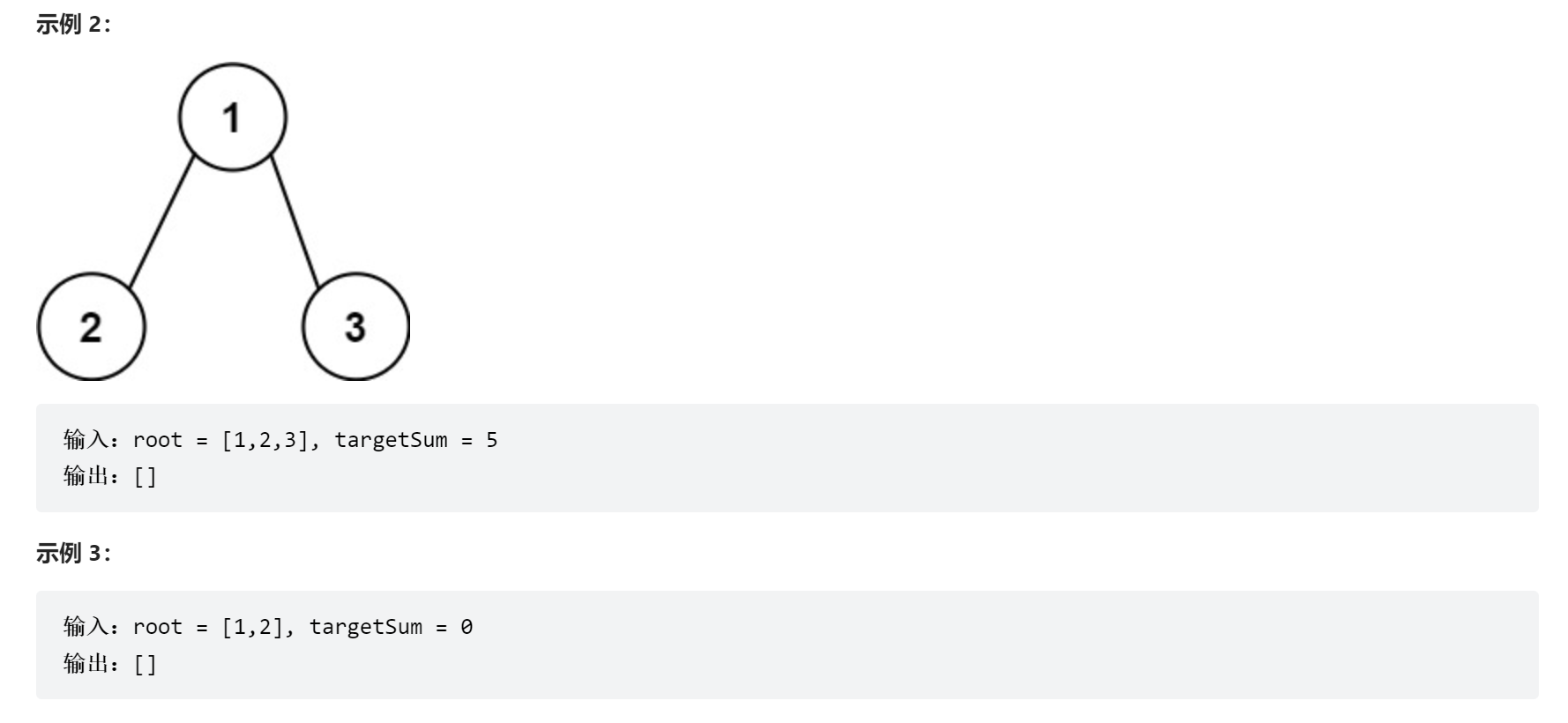
回溯(做选择,做完选择后回复做选择前的状态)
var pathSum = function(root, target) {
let res = [];
const backtrack = (root, path, sum)=>{
if(!root) return null;
path.push(root.val);
sum += root.val;
if(root.left == null && root.right == null && sum == target){
res.push(path.slice());
}
backtrack(root.left, path, sum);
backtrack(root.right, path, sum);
sum -= root.val;
path.pop();
}
backtrack(root, [], 0);
return res;
}
5. 剑指 Offer 36. 二叉搜索树与双向链表
原题链接
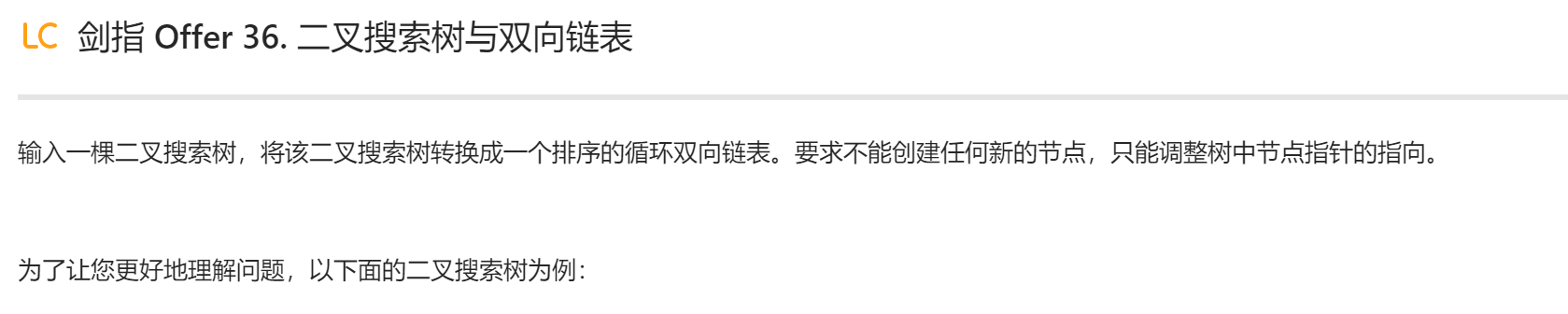 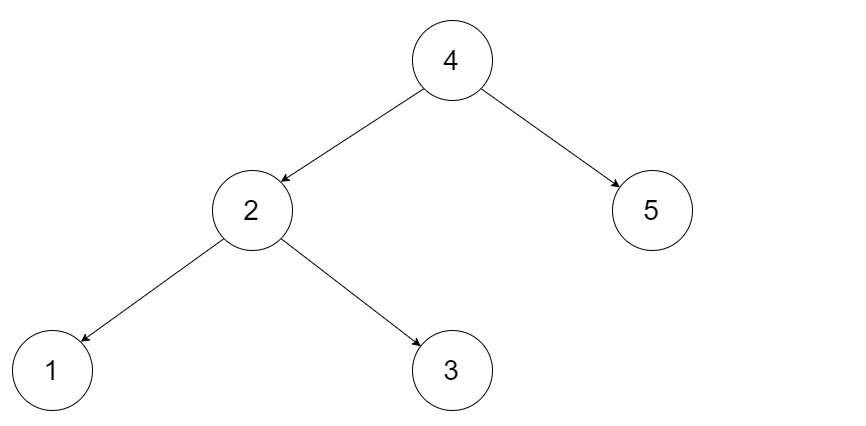 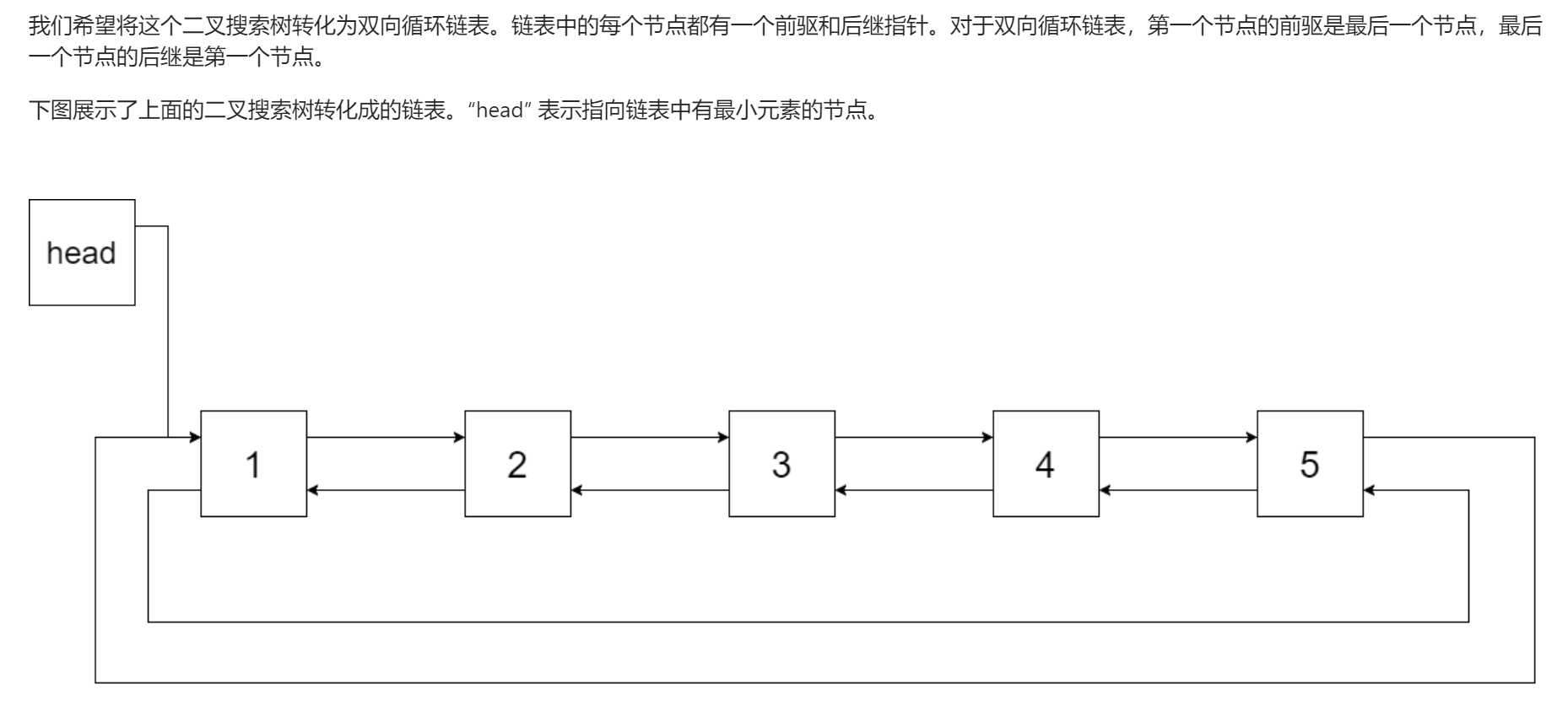 
二叉搜索树的性质:左节点 < 当前节点 < 右节点
var treeToDoublyList = function(root) {
if(!root) return null;
let head = null;
let pre = null;
const inorder = (node)=>{
if(!node) return;
inorder(node.left);
if(pre){
pre.right = node;
node.left = pre;
}else{
head = node;
}
pre = node;
inorder(node.right);
}
inorder(root);
pre.right = head;
head.left = pre;
return head;
}
|