lt.24. 两两交换链表中的节点
[案例需求] 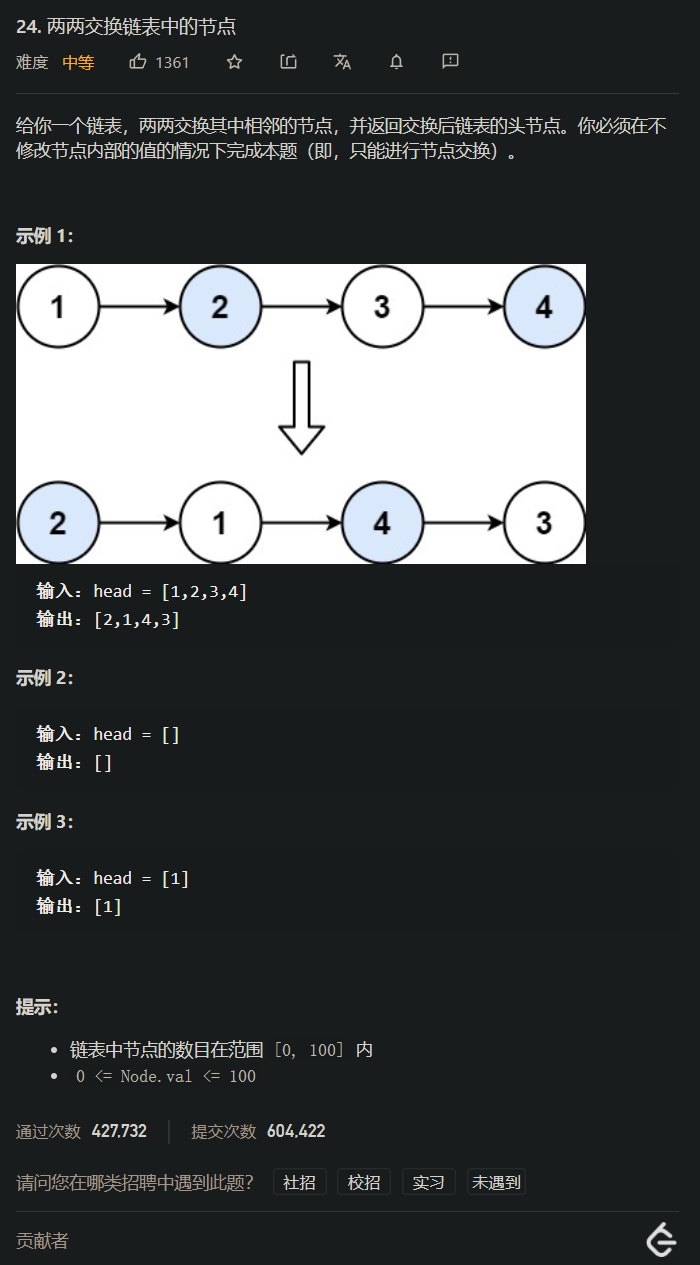
[思路分析一, 迭代法]
- 艹, 自己半年独立写的一道题, 再写一次过不了了还. 蛋疼!
[代码实现]
class Solution {
public ListNode swapPairs(ListNode head) {
ListNode dummyNode = new ListNode(-1);
dummyNode.next = head;
ListNode temp = dummyNode;
while(temp.next != null && temp.next.next != null){
ListNode cur_left = temp.next;
ListNode cur_right = temp.next.next;
temp.next = cur_right;
cur_left.next = cur_right.next;
cur_right.next = cur_left;
temp = cur_left;
}
return dummyNode.next;
}
}
[思路分析二, 递归法] 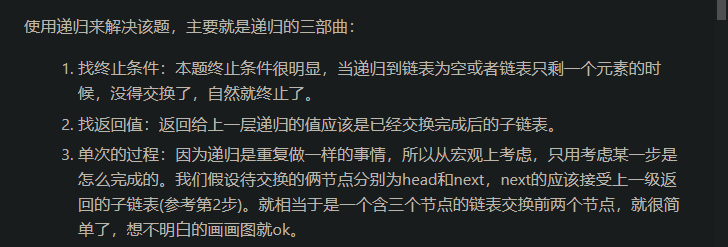
class Solution {
public ListNode swapPairs(ListNode head) {
if(head == null || head.next == null)return head;
ListNode next = head.next;
head.next = next.next;
next.next = head;
head.next = swapPairs(head.next);
return next;
}
}
lt.61. 旋转链表
[案例需求] 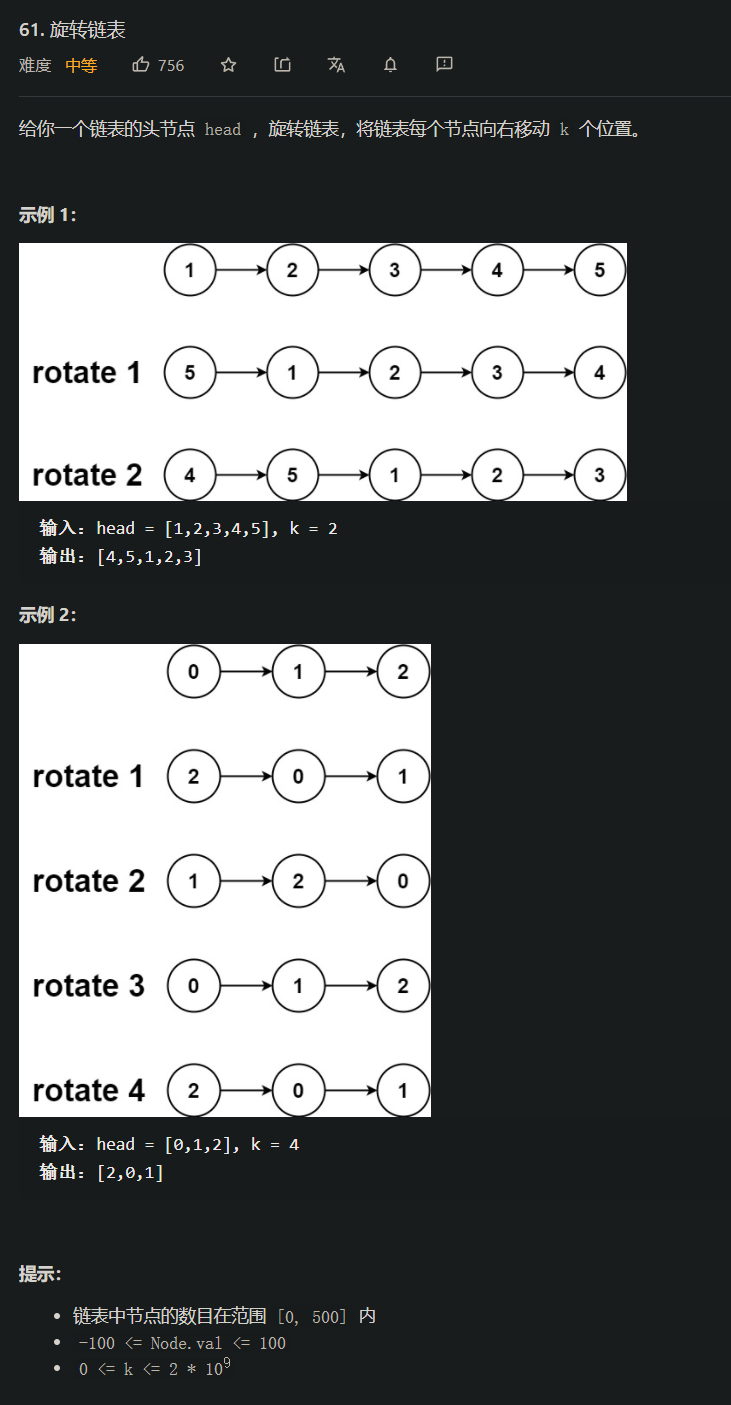
[思路分析]
[代码实现]
class Solution {
public ListNode rotateRight(ListNode head, int k) {
if(head == null) return head;
ListNode lenNode= head;
int count = 1;
while(lenNode.next != null){
count++;
lenNode = lenNode.next;
}
if(k % count == 0) return head;
int j = count - (k % count);
ListNode preNode = head;
while(j > 1){
preNode = preNode.next;
j--;
}
lenNode.next = head;
head = preNode.next;
preNode.next = null;
return head;
}
}
|