题目:
给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。
示例 1:
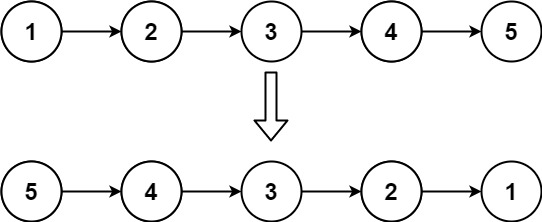
输入:head = [1,2,3,4,5]
输出:[5,4,3,2,1]
示例 2:
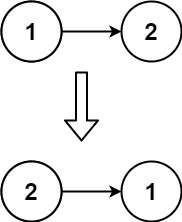
输入:head = [1,2]
输出:[2,1]
示例 3:
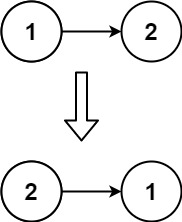
输入:head = []
输出:[]
方法一:迭代
- 定义两个指针 pre 和 cur,pre 指向 null,cur 指向 head。
- 遍历链表,每次让 cur 的 next 指向 pre,实现一次局部反转,局部反转完成之后,pre 和 cur 同时往前移动一个位置
循环上述过程,直至 cur 到达链表尾部。
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-VVqWviH2-1650870894068)(imgs/206.反转链表/image-20220425142205837.png)]
class Solution {
public ListNode reverseList(ListNode head) {
ListNode pre = null;
ListNode cur = head;
ListNode next;
while(cur != null){
next = cur.next;
cur.next = pre;
pre = cur;
cur = next;
}
return pre;
}
}
复杂度分析
- 时间复杂度O(n):其中 n 是链表的长度。需要遍历链表一次。
- 空间复杂度O(1)。
方法二:递归
[动画演示+多种解法 206. 反转链表 - 反转链表 - 力扣(LeetCode) (leetcode-cn.com)](https://leetcode-cn.com/problems/reverse-linked-list/solution/fan-zhuan-lian-biao-shuang-zhi-zhen-di-gui-yao-mo-/)
里面的幻灯片动画讲解不错
- 使用递归函数,一直递归到链表的最后一个结点,该结点就是反转后的头结点,记作 ret
- 此后,每次函数在返回的过程中,让当前结点的下一个结点的 next 指针指向当前节点
- 同时让当前结点的 next 指针指向 NULL ,从而实现从链表尾部开始的局部反转
- 当递归函数全部出栈后,链表反转完成
class Solution {
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode ret = reverseList(head.next);
head.next.next = head;
head.next = null;
return ret;
}
}
复杂度分析
- 时间复杂度O(n):其中 n 是链表的长度。需要对链表的每个节点进行反转操作。
- 空间复杂度O(n):其中 n 是链表的长度。空间复杂度主要取决于递归调用的栈空间,最多为 n 层。
|