1.List接口
(1)List接口是Collection接口的子接口,看源码: 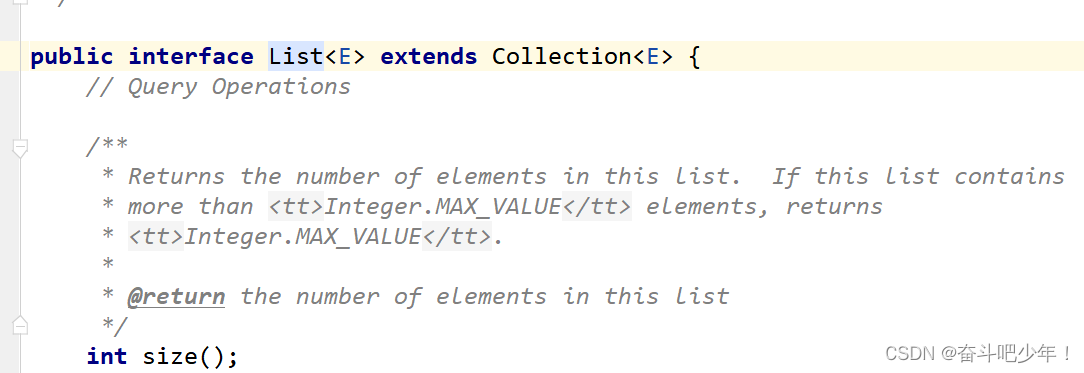 (2)List集合类特点
- List集合类中的元素有序(即添加顺序和取出顺序一致)、且可重复
- List集合中的每一个元素都有其对应的顺序索引,即支持索引。
- List容器中的元素都对应一个整数型的序号,记载其在容器中的位置,可以根据序号存取容器中的元素。
- JDK API中List接口的实现类
下图仅列出List接口的部分实现类,因为太多了: 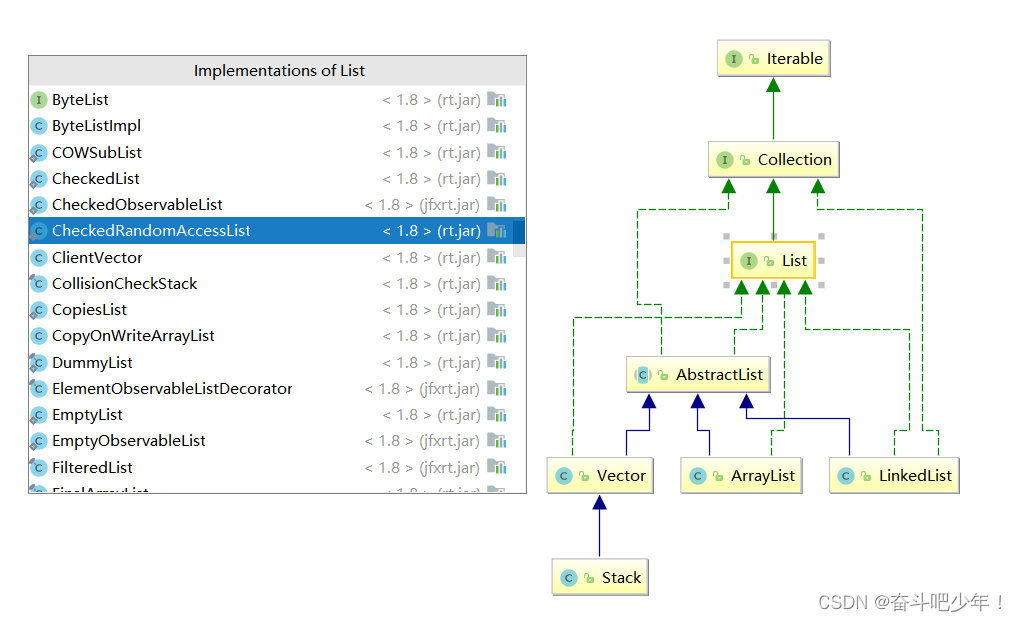
2.List接口常用方法
以下方法来自List接口源码:
public interface List<E> extends Collection<E> {
int size();
boolean isEmpty();
boolean contains(Object o);
Iterator<E> iterator();
Object[] toArray();
<T> T[] toArray(T[] a);
boolean remove(Object o);
boolean containsAll(Collection<?> c);
boolean addAll(Collection<? extends E> c);
boolean addAll(int index, Collection<? extends E> c);
boolean removeAll(Collection<?> c);
boolean retainAll(Collection<?> c);
default void replaceAll(UnaryOperator<E> operator) {
Objects.requireNonNull(operator);
final ListIterator<E> li = this.listIterator();
while (li.hasNext()) {
li.set(operator.apply(li.next()));
}
}
default void sort(Comparator<? super E> c) {
Object[] a = this.toArray();
Arrays.sort(a, (Comparator) c);
ListIterator<E> i = this.listIterator();
for (Object e : a) {
i.next();
i.set((E) e);
}
}
void clear();
boolean equals(Object o);
int hashCode();
E get(int index);
E set(int index, E element);
void add(int index, E element);
E remove(int index);
int indexOf(Object o);
}
重点讲述方法:
* @param a the array into which the elements of this list are to
* be stored, if it is big enough; otherwise, a new array of the
* same runtime type is allocated for this purpose.
* @return an array containing the elements of this list
* @throws ArrayStoreException if the runtime type of the specified array
* is not a supertype of the runtime type of every element in
* this list
* @throws NullPointerException if the specified array is null
*/
<T> T[] toArray(T[] a);
<T> T[] toArray(T[] a);:实现链表到数组的转换,这里的a指定的存储数组
如果a的长度小于当前列表大小,则不进行转换 如果a的长度大于当前列表大小,多余的位置的一个会用null标记 注意:throws ArrayStoreException 如果指定数组的运行时类型 不是每个元素的运行时类型的超类型,保证指定的数组类型为链表元素的超类型。
List<Integer> list3 = new ArrayList<>();
list3.add(1);
list3.add(2);
list3.add(3);
Integer[] objects = new Integer[]{8, 8,8};
list3.toArray(objects);
System.out.println(Arrays.asList(objects));
3.练习
上述方法测试(部分),剩余内容可以自己去测:
package Collection.list;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ListTest {
public static void main(String[] args) {
List list=new ArrayList();
list.add(1);
list.add(2);
list.add("背景");
list.add(new Cat("小欢",3));
System.out.println("当前集合大小:"+list.size());
System.out.println("当前集合是否为空:"+list.isEmpty());
System.out.println("是否包含2:"+list.contains(2));
System.out.println("迭代器遍历集合:");
Iterator iterator = list.iterator();
while (iterator.hasNext()){
Object next = iterator.next();
System.out.println(next);
}
System.out.println("集合转化为数组:");
Object[] array = list.toArray();
for (Object o : array) {
System.out.println(o);
}
System.out.println("remove的使用:");
Iterator iterator1 = list.iterator();
while (iterator1.hasNext()){
System.out.println(iterator1.next());
}
System.out.println("containsAll:判断当前集合是否包含指定的集合元素:");
ArrayList list1 = new ArrayList();
list1.add(1);
list1.add(2);
System.out.println(list.containsAll(list1));
System.out.println("addAll(Collection<? extends E> c)添加集合,该集合可以是E或E的子类");
list.addAll(list1);
iterator=list.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
System.out.println("boolean addAll(int index, Collection<? extends E> c)");
list.addAll(1,list1);
iterator=list.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
System.out.println("boolean removeAll(Collection<?> c):");
System.out.println("boolean retainAll(Collection<?> c):");
list.retainAll(list1);
iterator=list.iterator();
while (iterator.hasNext()){
System.out.println(iterator.next());
}
System.out.println("clear:清空列表");
list.clear();
System.out.println(list);
int i = list.hashCode();
System.out.println(i);
}
}
class Cat{
public String name;
public int age;
public Cat(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Cat{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
|