马踏棋盘算法
- 图的深度优先DFS
- 回溯
- 八皇后问题、小老鼠找迷宫问题
一、介绍
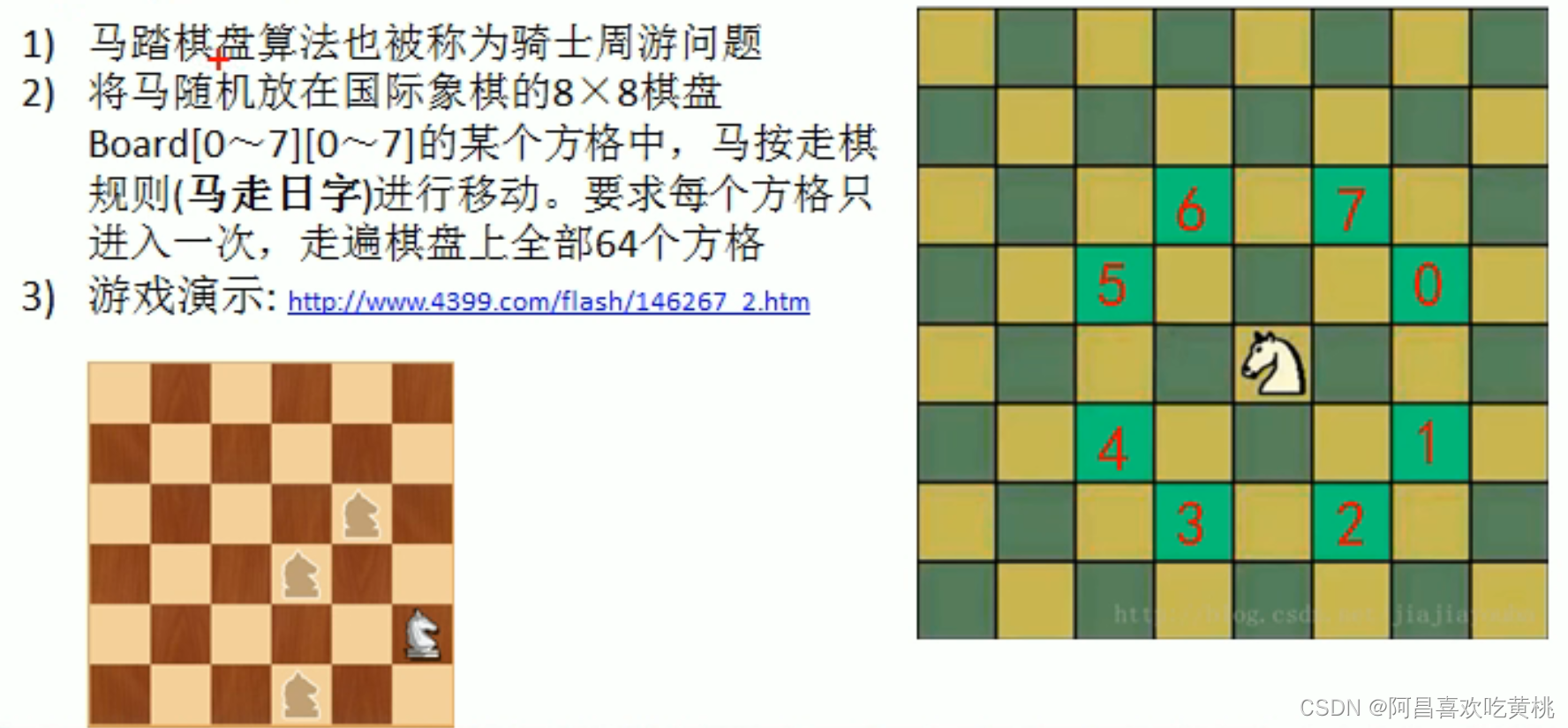
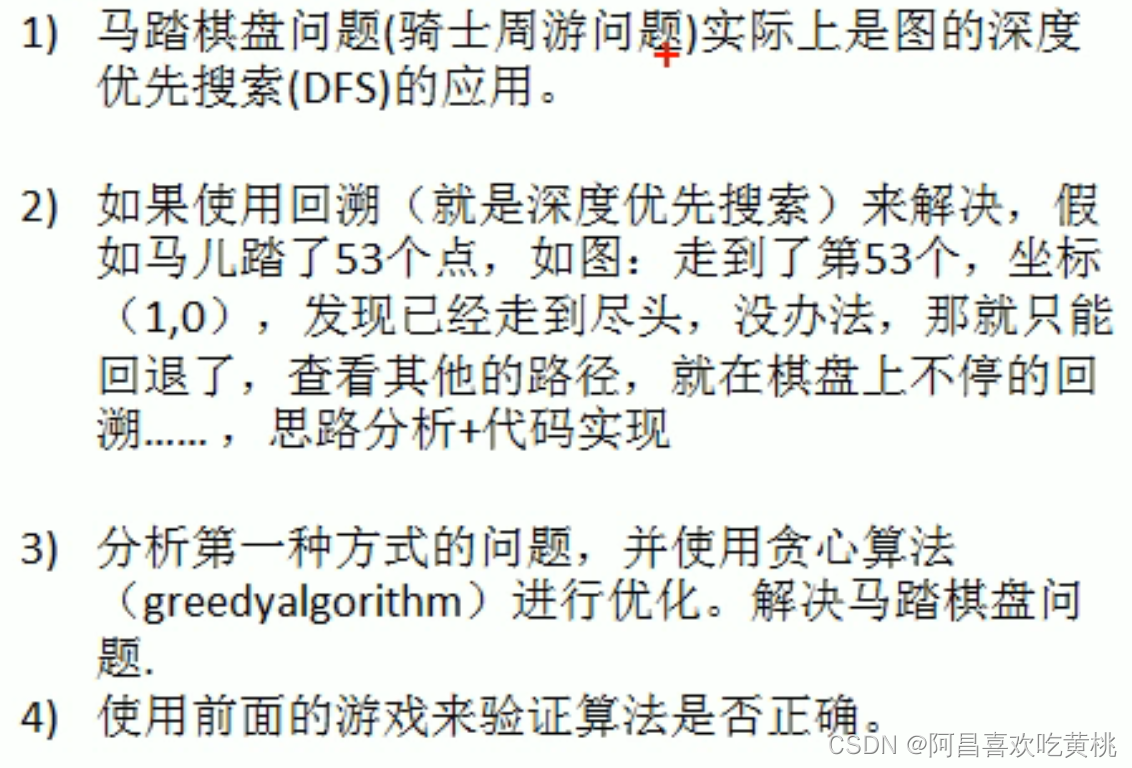
二、思路分析
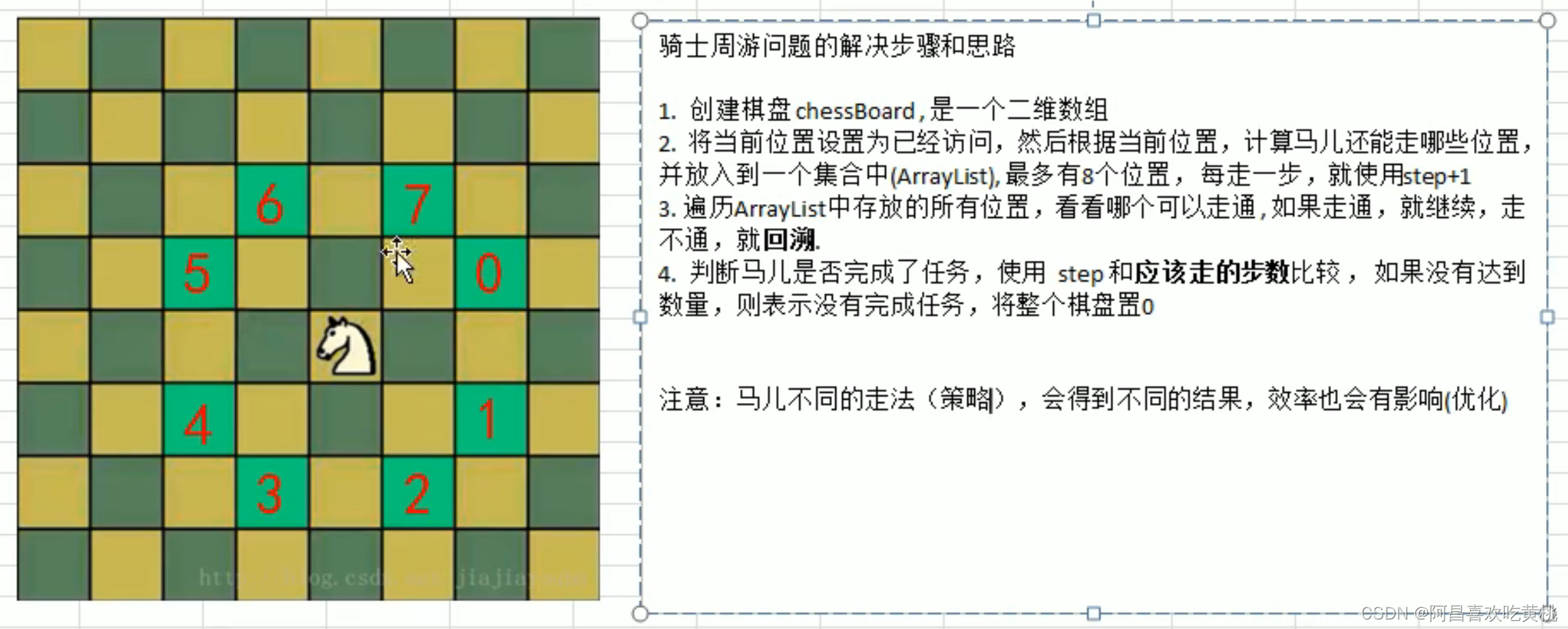
三、代码实现
package com.achang.algorithm;
import java.awt.*;
import java.util.ArrayList;
import java.util.Arrays;
class HorseChessBoard {
public static void main(String[] args) {
x = 6;
y = 6;
int row = 2;
int column = 4;
int[][] chessboard = new int[x][y];
visited = new boolean[x * y];
long startTime = System.currentTimeMillis();
traversalChessboard(chessboard, row - 1, column - 1, 1);
System.out.println(("程序运行了:" + (System.currentTimeMillis() - startTime) + "ms"));
for (int[] ints : chessboard) {
System.out.println(Arrays.toString(ints));
}
}
private static boolean[] visited;
private static boolean finished;
private static int x;
private static int y;
public static void traversalChessboard(int[][] chessBoard, int row, int column, int step) {
chessBoard[row][column] = step;
visited[row * x + column] = true;
ArrayList<Point> ps = next(new Point(column, row));
while (!ps.isEmpty()) {
Point p = ps.remove(0);
if (!visited[p.y * x + p.x]) {
traversalChessboard(chessBoard, p.y, p.x, step + 1);
}
}
if (step < (x * y) && !finished) {
chessBoard[row][column] = 0;
visited[row * x + column] = false;
} else {
finished = true;
}
}
public static ArrayList<Point> next(Point curPoint) {
ArrayList<Point> points = new ArrayList<>();
Point point = new Point();
if ((point.x = curPoint.x - 2) >= 0 && (point.y = curPoint.y - 1) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 1) >= 0 && (point.y = curPoint.y - 2) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 1) < x && (point.y = curPoint.y - 2) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 2) < x && (point.y = curPoint.y - 1) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 2) < x && (point.y = curPoint.y + 1) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 1) < x && (point.y = curPoint.y + 2) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 1) >= 0 && (point.y = curPoint.y + 2) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 2) >= 0 && (point.y = curPoint.y + 1) < y) {
points.add(new Point(point));
}
return points;
}
}
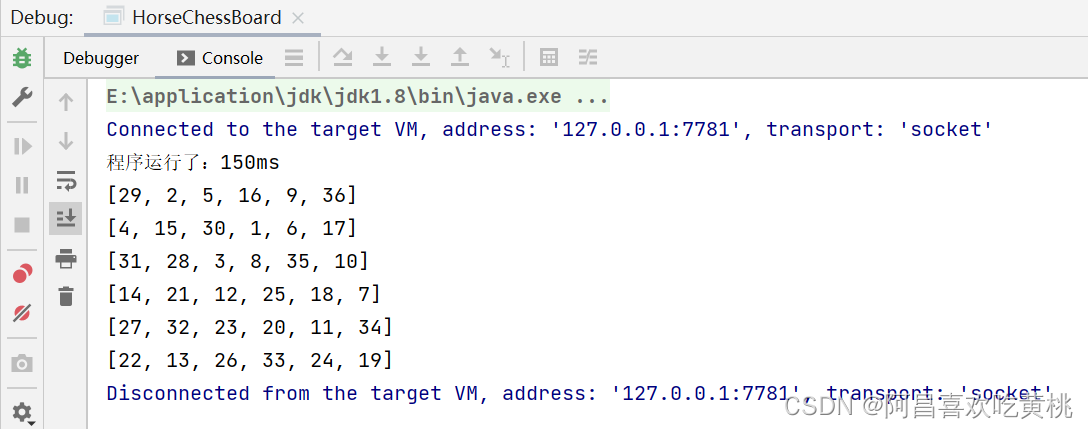
当我们将棋盘设置为8*8以后,会发现这个算法会大幅度降低速度!!!
四、贪心算法优化方案
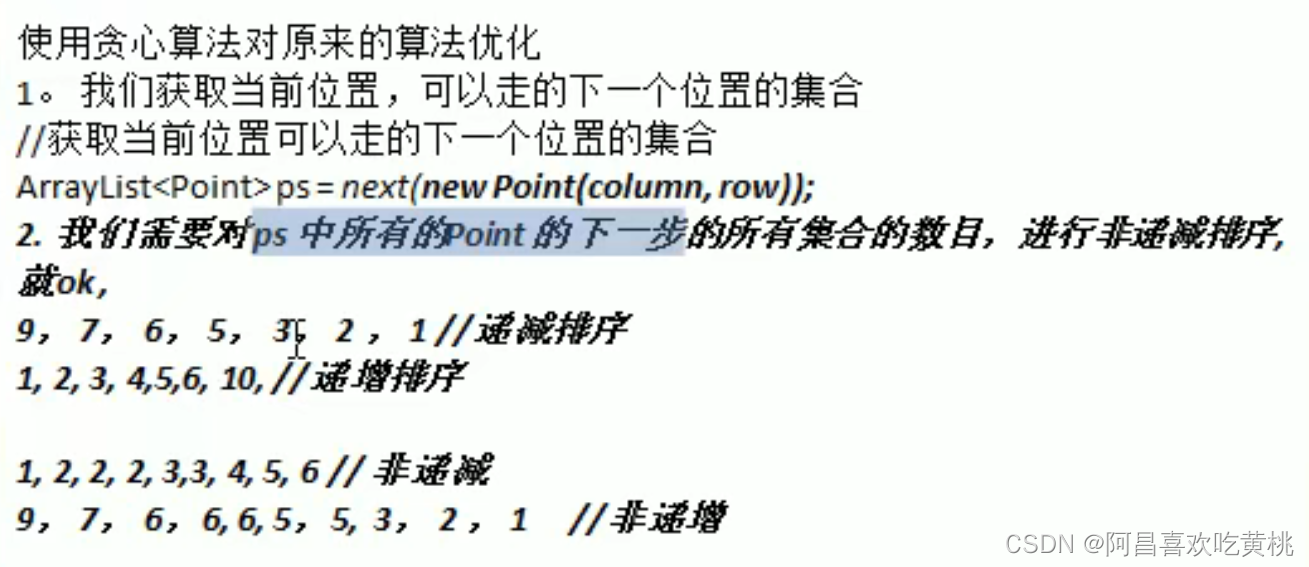
package com.achang.algorithm;
import java.awt.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
class HorseChessBoard {
public static void main(String[] args) {
x = 6;
y = 6;
int row = 2;
int column = 4;
int[][] chessboard = new int[x][y];
visited = new boolean[x * y];
long startTime = System.currentTimeMillis();
traversalChessboard(chessboard, row - 1, column - 1, 1);
System.out.println(("程序运行了:" + (System.currentTimeMillis() - startTime) + "ms"));
for (int[] ints : chessboard) {
System.out.println(Arrays.toString(ints));
}
}
private static boolean[] visited;
private static boolean finished;
private static int x;
private static int y;
public static void traversalChessboard(int[][] chessBoard, int row, int column, int step) {
chessBoard[row][column] = step;
visited[row * x + column] = true;
ArrayList<Point> ps = next(new Point(column, row));
sort(ps);
while (!ps.isEmpty()) {
Point p = ps.remove(0);
if (!visited[p.y * x + p.x]) {
traversalChessboard(chessBoard, p.y, p.x, step + 1);
}
}
if (step < (x * y) && !finished) {
chessBoard[row][column] = 0;
visited[row * x + column] = false;
} else {
finished = true;
}
}
public static ArrayList<Point> next(Point curPoint) {
ArrayList<Point> points = new ArrayList<>();
Point point = new Point();
if ((point.x = curPoint.x - 2) >= 0 && (point.y = curPoint.y - 1) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 1) >= 0 && (point.y = curPoint.y - 2) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 1) < x && (point.y = curPoint.y - 2) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 2) < x && (point.y = curPoint.y - 1) >= 0) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 2) < x && (point.y = curPoint.y + 1) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x + 1) < x && (point.y = curPoint.y + 2) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 1) >= 0 && (point.y = curPoint.y + 2) < y) {
points.add(new Point(point));
}
if ((point.x = curPoint.x - 2) >= 0 && (point.y = curPoint.y + 1) < y) {
points.add(new Point(point));
}
return points;
}
public static void sort(ArrayList<Point> ps){
ps.sort(new Comparator<Point>() {
@Override
public int compare(Point o1, Point o2) {
ArrayList<Point> next = next(o1);
ArrayList<Point> next1 = next(o1);
if (next.size() < next1.size()){
return -1;
}else if (next.size() == next1.size()){
return 0;
}else {
return 1;
}
}
});
}
}
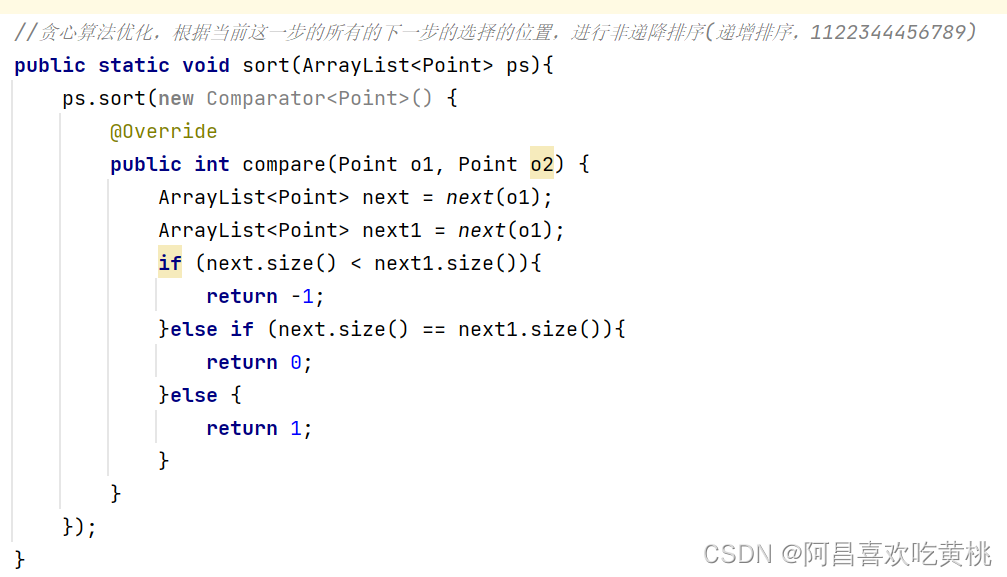
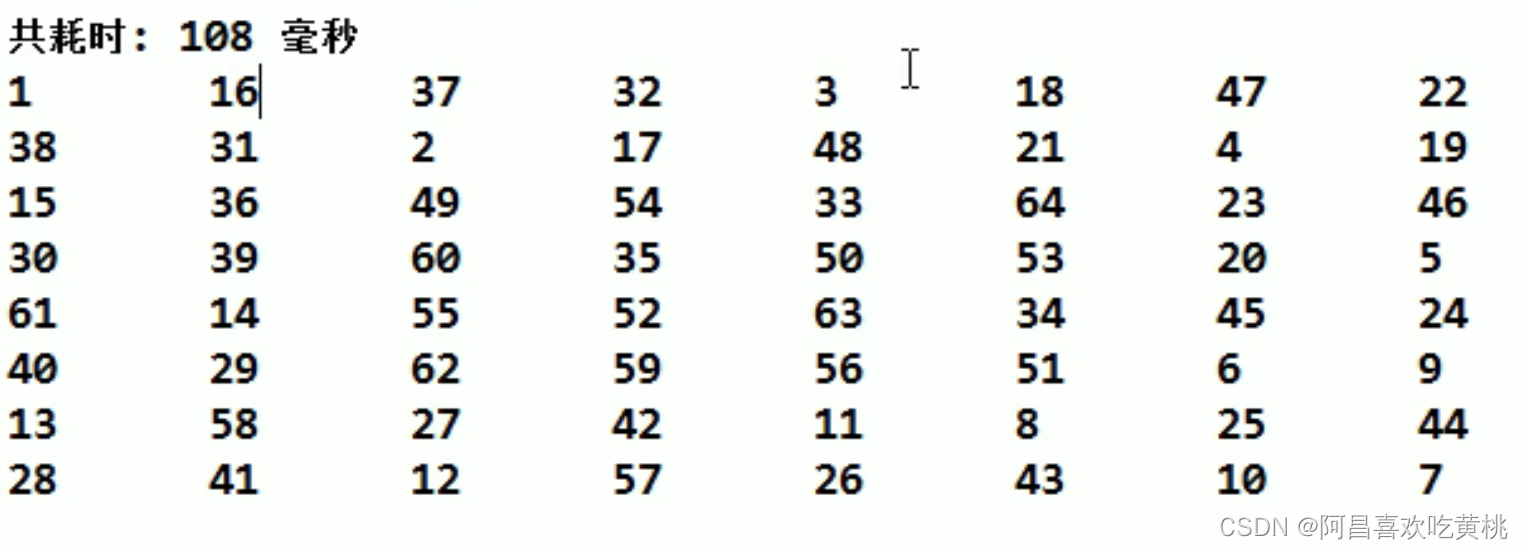
|