题目: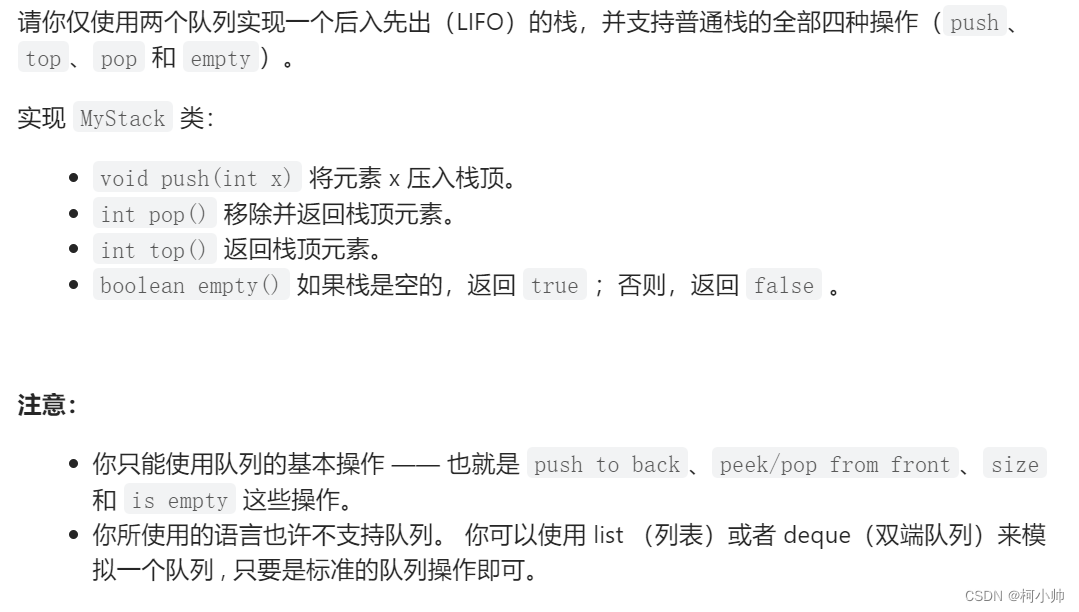
算法思想: 使用两个队列,一个作为主队列,一个作为辅助队列,这题关键主要就是入队列的时候,需要把主队列的所有已存在的元素临时存储到辅助队列之中,然后把数x入到主队列中,再将辅助队列的所有元素输出到主队列即可。
代码:
class MyStack {
Queue<Integer> q1;
Queue<Integer> q2;
public MyStack() {
q1 = new LinkedList<>();
q2 = new LinkedList<>();
}
public void push(int x) {
while(!q1.isEmpty()) {
q2.offer(q1.poll());
}
q1.offer(x);
while(!q2.isEmpty()) {
q1.offer(q2.poll());
}
}
public int pop() {
return q1.poll();
}
public int top() {
return q1.peek();
}
public boolean empty() {
if(q1.isEmpty()) {
return true;
} else {
return false;
}
}
}
|