Question
1823. 找出游戏的获胜者 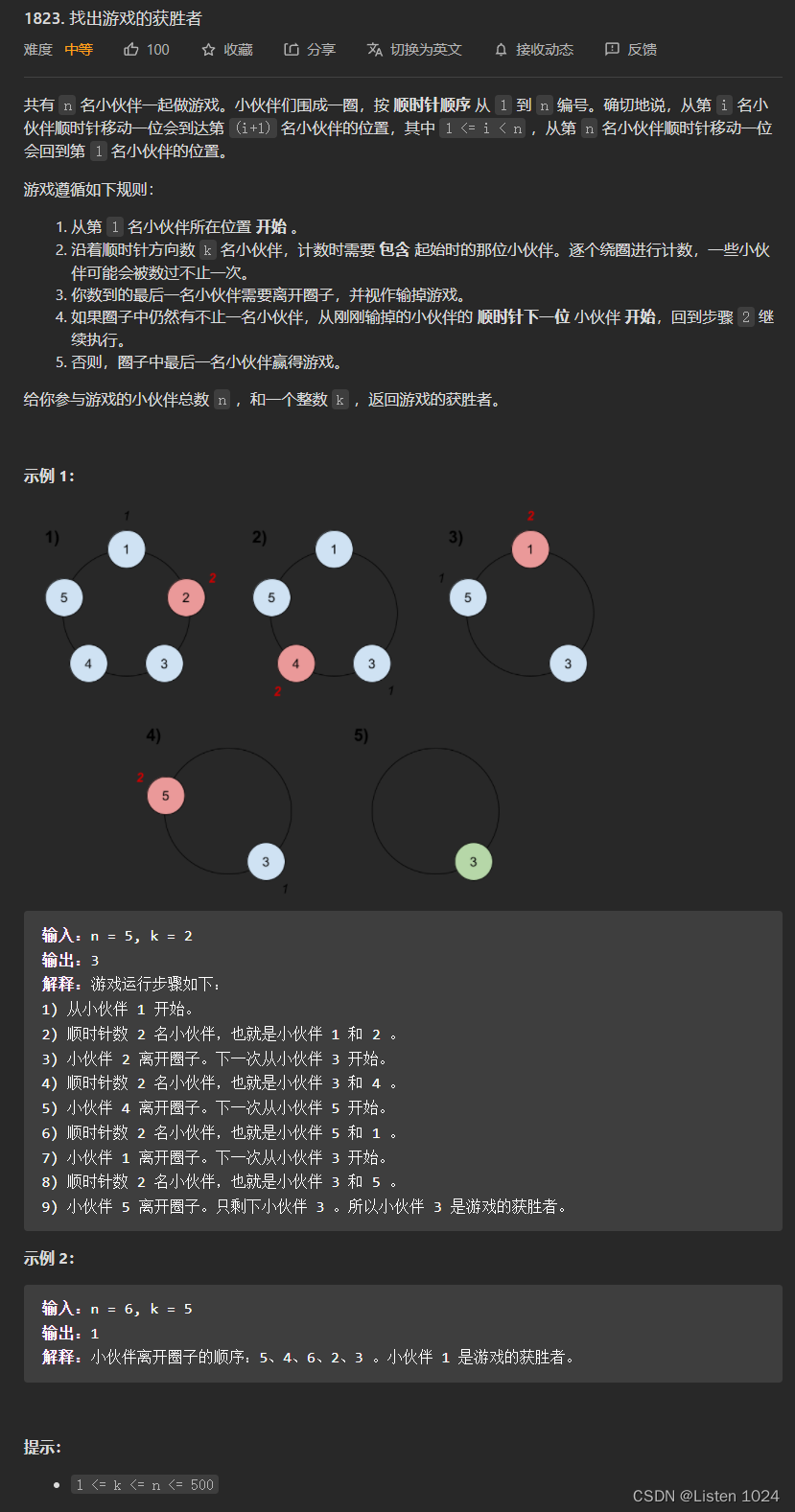
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/find-the-winner-of-the-circular-game/
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
Ideas
1、Answer( Java )
解法思路:约瑟夫环问题数学解法
??著名的约瑟夫环问题,是有 数学解法 的
👍反推的公式 (当前 index + m) % 上一轮剩余数字的个数
约瑟夫环问题数学解法详见如下题解链接
https://blog.csdn.net/Listen_BX/article/details/124572508
Code
class Solution4 {
public int findTheWinner(int n, int k) {
int res = 0;
for (int i = 2; i <= n; i++) {
res = (res + k) % i;
}
return res + 1;
}
}
https://leetcode-cn.com/problems/yuan-quan-zhong-zui-hou-sheng-xia-de-shu-zi-lcof/solution/javajie-jue-yue-se-fu-huan-wen-ti-gao-su-ni-wei-sh/
2、Answer( Java )
解法思路:简单模拟( 暴力解法 )
👍需要注意的是索引的更新是进行 取模运算 而不是简单相减
Code
class Solution {
public int findTheWinner(int n, int k) {
int res = 0;
ArrayList<Integer> list = new ArrayList<>();
for (int i = 1; i <= n; i++) {
list.add(i);
}
int index = 0;
while (list.size() > 1) {
index = (index + k - 1) % list.size();
list.remove(index);
}
res = list.get(0);
return res;
}
}
class Solution {
public int findTheWinner(int n, int k) {
int res = 0;
ArrayDeque<Integer> deque = new ArrayDeque<>();
for (int i = 1; i <= n; i++) {
deque.offer(i);
}
while (deque.size() > 1) {
for (int i = 1; i < k; i++) {
deque.offer(deque.poll());
}
deque.poll();
}
res = deque.peek();
return res;
}
}
3、Answer( Java )
解法思路:数学 + 递归
https://leetcode-cn.com/problems/find-the-winner-of-the-circular-game/solution/zhao-chu-you-xi-de-huo-sheng-zhe-by-leet-w2jd/
Code
class Solution {
public int findTheWinner(int n, int k) {
if (n == 1) {
return 1;
}
return (k + findTheWinner(n - 1, k) - 1) % n + 1;
}
}
|