数据结构与算法
数据结构是计算机中存储、组织数据的方式
算法通俗理解就是解决问题的方法/步骤逻辑
定义:
1.一个有限的指令集,每条指令的描述不依赖与语言
2.接受一些输入(也可能不需要输入),产生输出
3.一定在有限步骤之后终止
数据结构的实现,离不开算法
数组是一种线性结构,可以在数组的任意位置插入和删除数据 为了实现某些功能,必须对这种任意性加以限制 栈和队列就是常见的受限的线性结构
栈
后进先出 LIFO 类似于子弹夹
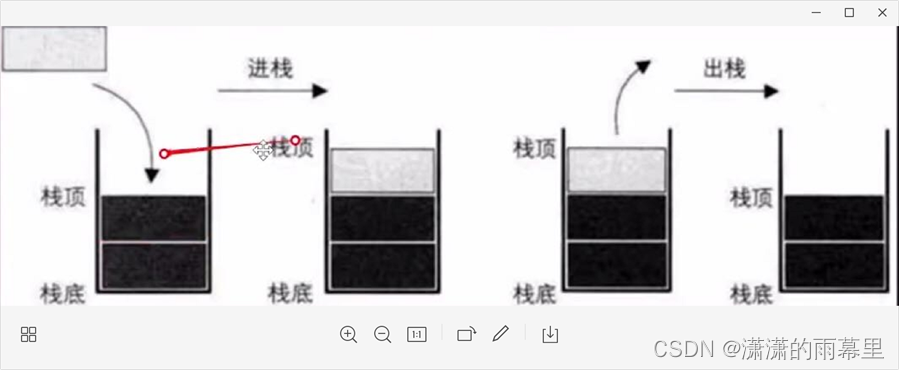
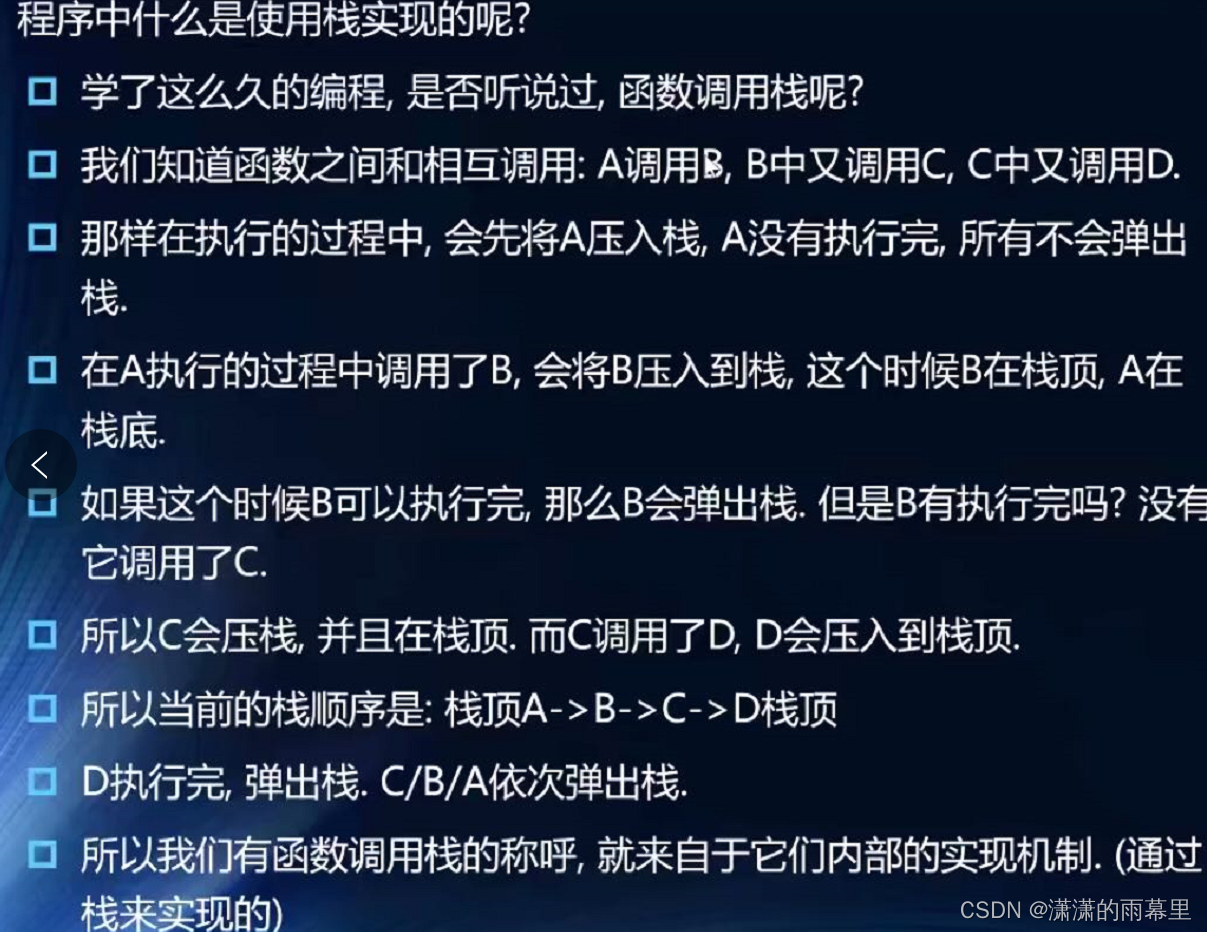
所以递归容易出现栈溢出
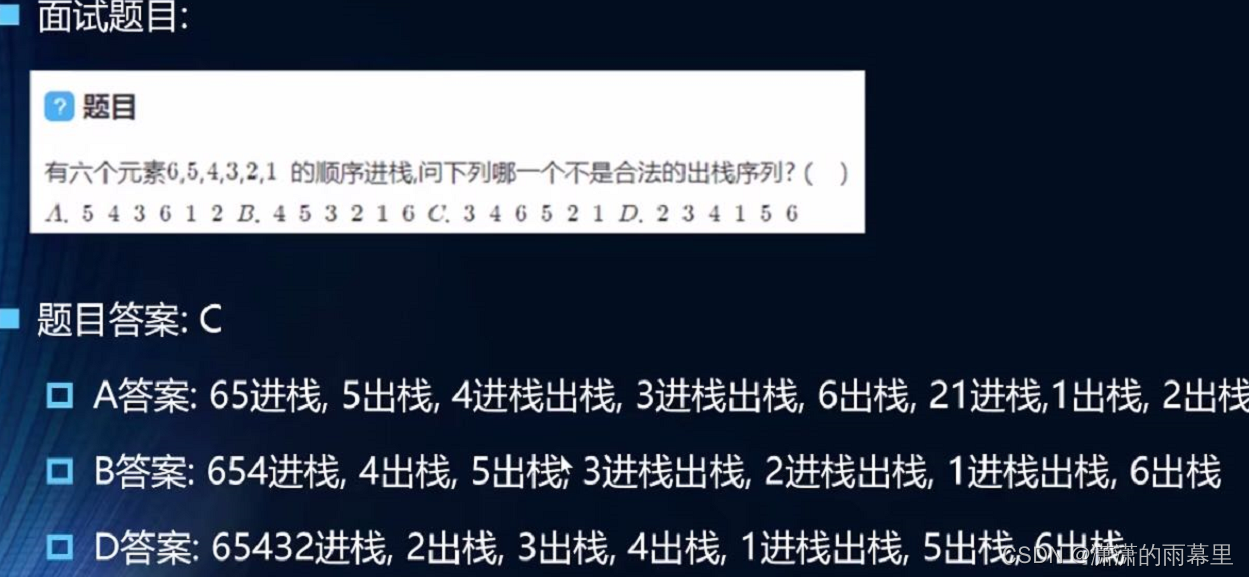
栈结构的实现
1.基于数据
2.基于链表
基于数据实现栈结构 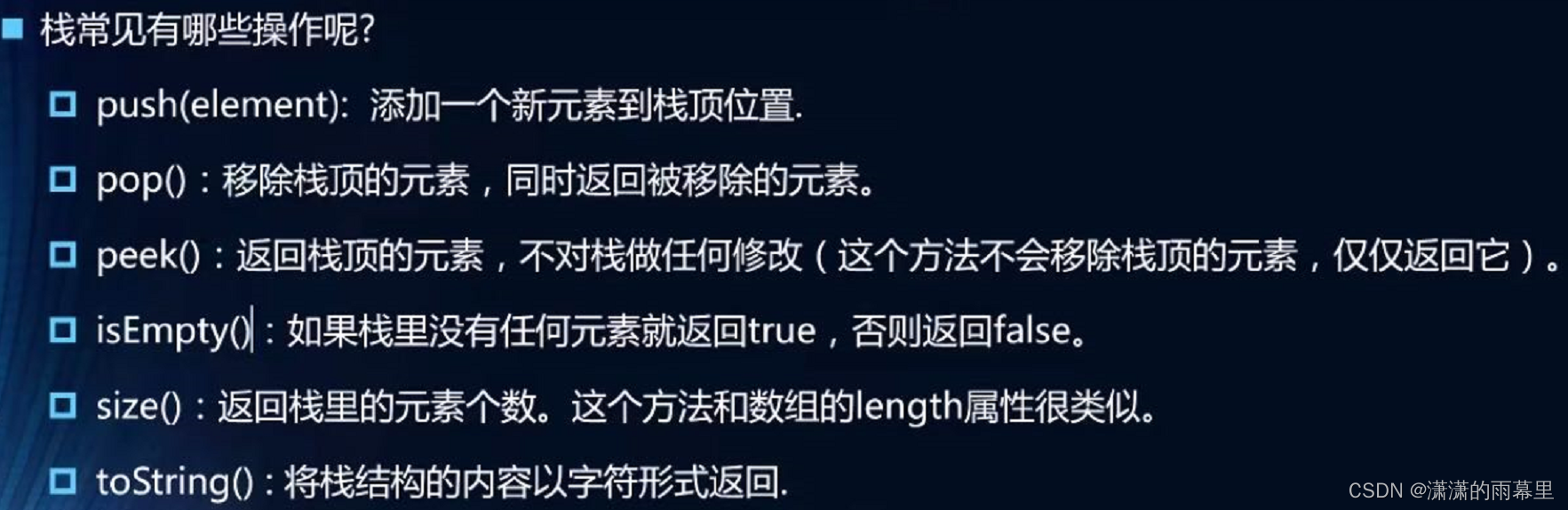
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>itjc8</title>
</head>
<body>
<script>
function Stack() {
var items = []
this.push = function (element) {
items.push(element)
}
this.pop = function () {
return items.pop()
}
this.peek = function () {
return items[items.length - 1]
}
this.isEmpty = function () {
return items.length == 0
}
this.size = function () {
return items.length
}
}
function dec2bin(decNumer) {
var stack = new Stack()
var remainder;
while (decNumer > 0) {
remainder = decNumer % 2
decNumer = Math.floor(decNumer / 2)
stack.push(remainder)
}
var binayriStrng = ""
while (!stack.isEmpty()) {
binayriStrng += stack.pop()
}
return binayriStrng
}
alert(dec2bin(10))
alert(dec2bin(233))
alert(dec2bin(1000))
</script>
</body>
</html>
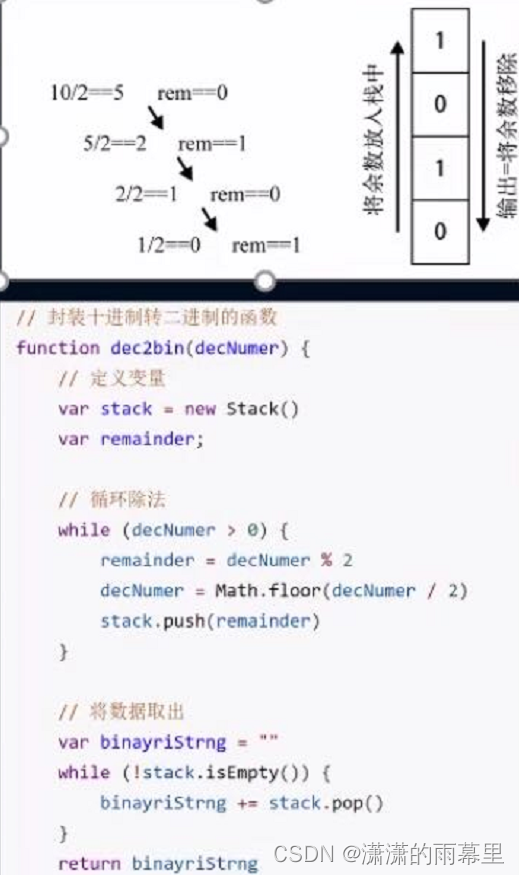
|