力扣225.用队列实现栈
1、使用一个队列
class MyStack {
Queue<Integer> queue;
public MyStack() {
queue = new LinkedList<Integer>();
}
public void push(int x) {
int n = queue.size();
queue.offer(x);
for (int i = 0; i < n; i++) {
queue.offer(queue.poll());
}
}
public int pop() {
return queue.poll();
}
public int top() {
return queue.peek();
}
public boolean empty() {
return queue.isEmpty();
}
}
运行截图: 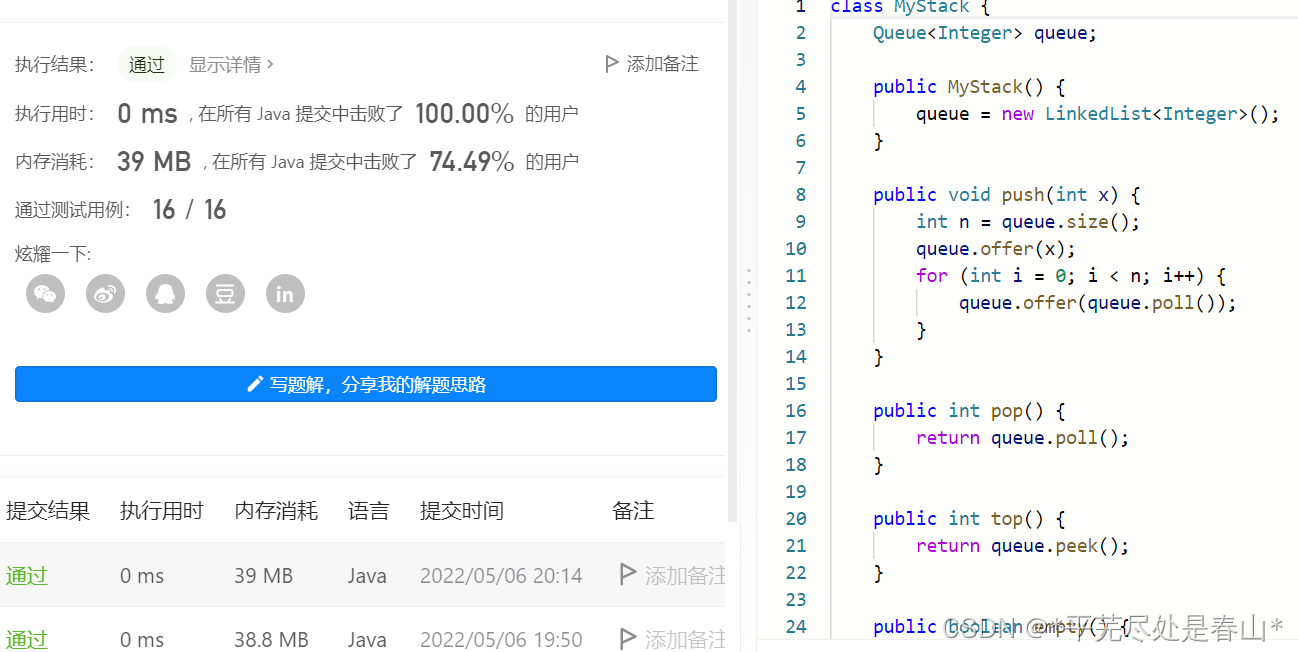
2、使用两个队列
public class MyStack {
private Queue<Integer> queue1=new LinkedList<>();
private Queue<Integer> queue2=new LinkedList<>();
public void push(int x) {
queue2.offer(x);
while(! queue1.isEmpty()){
queue2.offer(queue1.poll());
}
Queue<Integer> tmp=queue1;
queue1=queue2;
queue2=tmp;
}
public int pop() {
return queue1.poll();
}
public int top() {
return queue1.peek();
}
public boolean empty() {
return queue1.isEmpty();
}
}
运行截图: 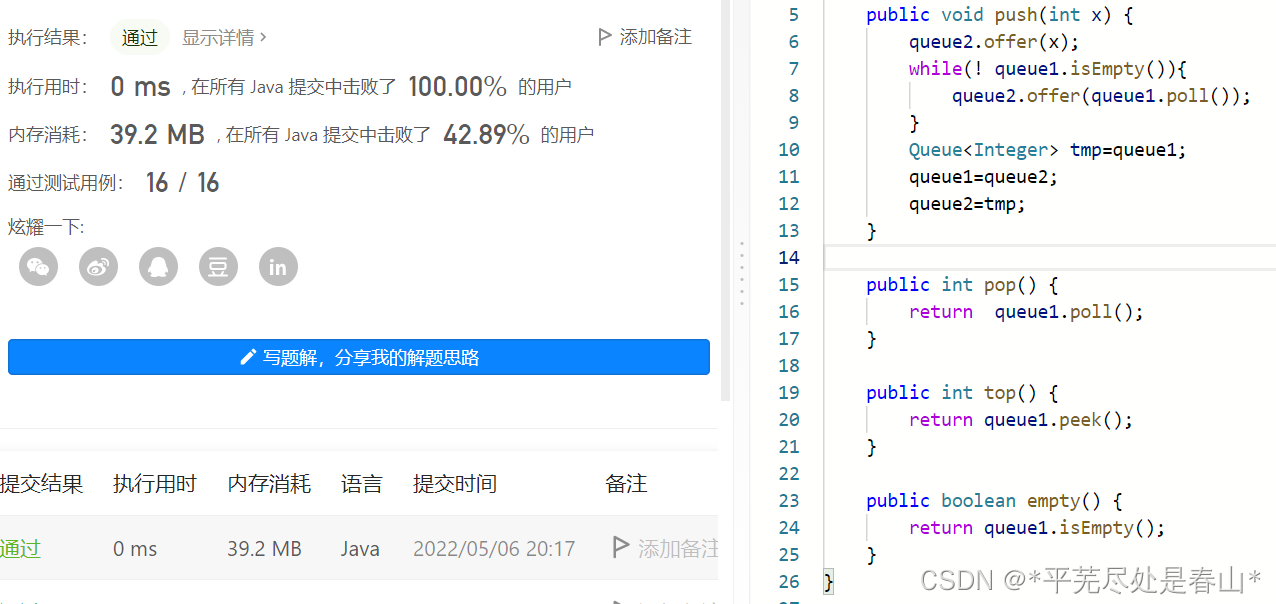
力扣232.用栈实现队列(使用两个栈)
public class MyQueue {
private Stack<Integer> s1;
private Stack<Integer> s2;
public MyQueue(){
s1=new Stack<>();
s2=new Stack<>();
}
public void push(int x){
if(s1.isEmpty()){
s1.push(x);
}else{
while(! s1.isEmpty()){
s2.push(s1.pop());
}
s1.push(x);
while(! s2.isEmpty()){
s1.push(s2.pop());
}
}
}
public int pop(){
return s1.pop();
}
public int peek(){
return s1.peek();
}
public boolean empty(){
return s1.isEmpty();
}
}
运行截图: 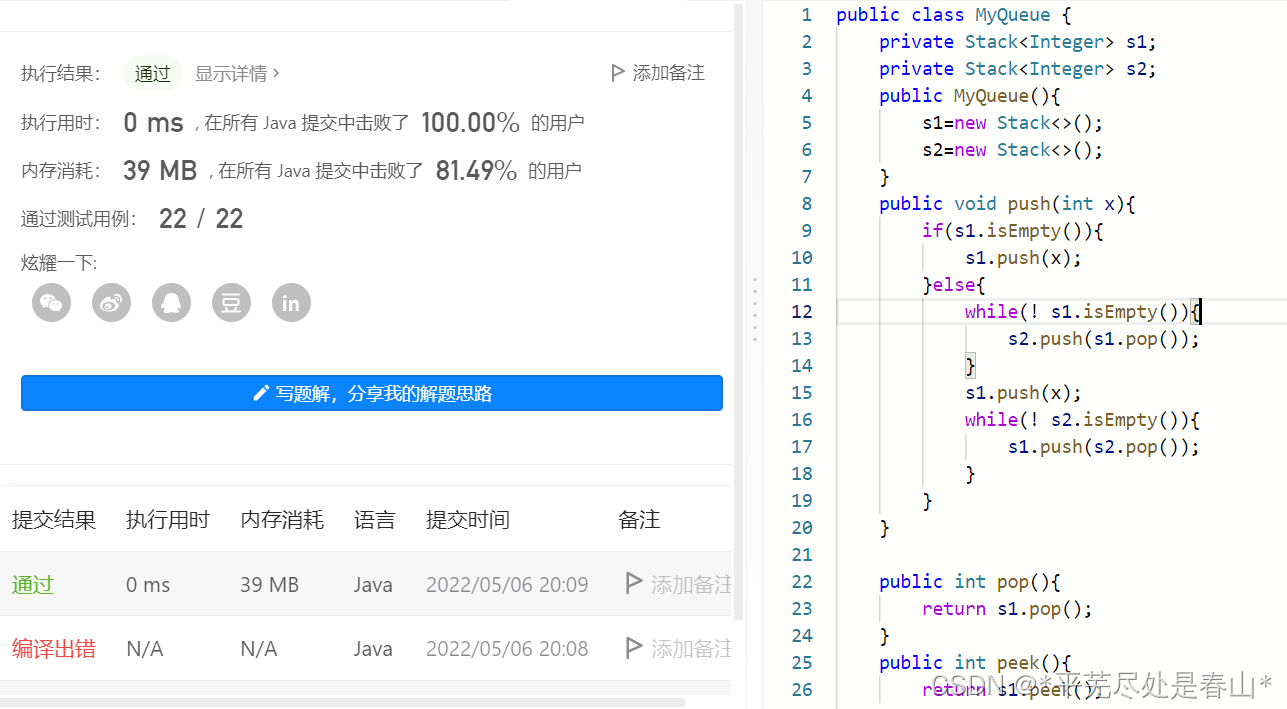
|