Example005
题目
Q 是一个队列,S 是一个空栈,实现将队列中的元素逆置的算法。
分析
主要考查对队列和栈的特性与操作。由于对队列的一系列操作不可能将其中的元素全部逆置,而栈可以将入栈的元素逆序提取出来,因此我们可以让队列中的元素逐个出队列,一一入栈;全部入栈后再逐个入栈,一一入队列。
图解
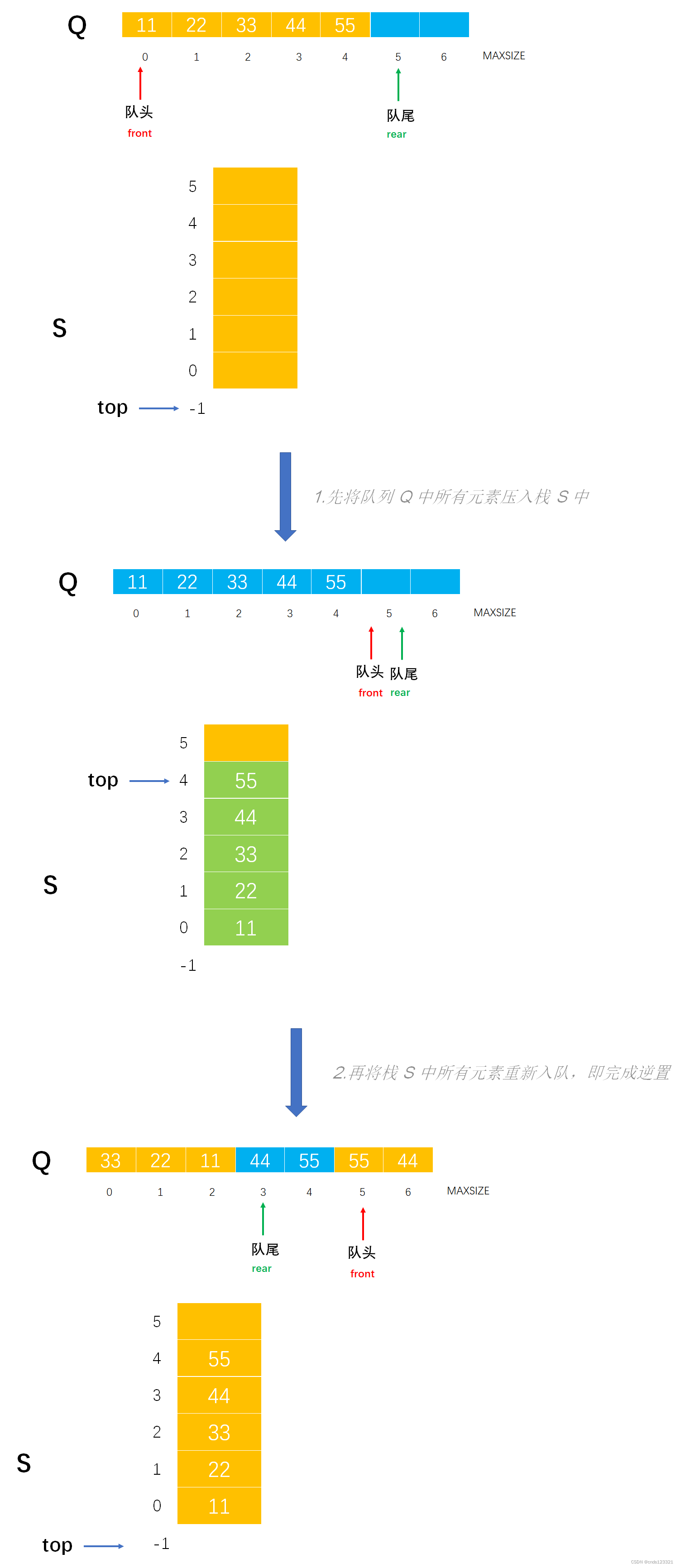
C实现
核心代码:
void inversionQueue(SeqQueue *Q, SeqStack *S) {
while (!isEmptyQueue(*Q)) {
int ele;
deQueue(Q, &ele);
push(S, ele);
}
while (!isEmptyStack(*S)) {
int top;
pop(S, &top);
enQueue(Q, top);
}
}
完整代码:
#include<stdio.h>
#define QUEUE_MAXSIZE 10
typedef struct {
int data[QUEUE_MAXSIZE];
int front;
int rear;
} SeqQueue;
void initQueue(SeqQueue *queue) {
queue->front = 0;
queue->rear = 0;
}
int isEmptyQueue(SeqQueue queue) {
if (queue.front == queue.rear) {
return 1;
} else {
return 0;
}
}
int enQueue(SeqQueue *queue, int ele) {
if (queue->rear == QUEUE_MAXSIZE) {
return 0;
}
queue->data[queue->rear] = ele;
queue->rear++;
return 1;
}
int deQueue(SeqQueue *queue, int *ele) {
if (queue->front == queue->rear) {
return 0;
}
*ele = queue->data[queue->front];
queue->front++;
return 1;
}
void printQueue(SeqQueue queue) {
printf("[");
for (int i = queue.front; i < queue.rear; i++) {
printf("%d", queue.data[i]);
if (i != queue.rear - 1) {
printf(", ");
}
}
printf("]\n");
}
#define STACK_MAXSIZE 100
typedef struct {
int data[STACK_MAXSIZE];
int top;
} SeqStack;
void initStack(SeqStack *stack) {
stack->top = -1;
}
int isEmptyStack(SeqStack stack) {
if (stack.top == -1) {
return 1;
} else {
return 0;
}
}
int push(SeqStack *stack, int ele) {
if (stack->top == STACK_MAXSIZE - 1) {
return 0;
}
stack->top++;
stack->data[stack->top] = ele;
return 1;
}
int pop(SeqStack *stack, int *ele) {
if (stack->top == -1) {
return 0;
}
*ele = stack->data[stack->top];
stack->top--;
return 1;
}
void inversionQueue(SeqQueue *Q, SeqStack *S) {
while (!isEmptyQueue(*Q)) {
int ele;
deQueue(Q, &ele);
push(S, ele);
}
while (!isEmptyStack(*S)) {
int top;
pop(S, &top);
enQueue(Q, top);
}
}
int main() {
SeqQueue queue;
initQueue(&queue);
enQueue(&queue, 11);
enQueue(&queue, 22);
enQueue(&queue, 33);
enQueue(&queue, 44);
enQueue(&queue, 55);
SeqStack stack;
initStack(&stack);
printQueue(queue);
inversionQueue(&queue, &stack);
printQueue(queue);
}
执行结果:
[11, 22, 33, 44, 55]
[55, 44, 33, 22, 11]
Java实现
核心代码:
public static void inversionQueue(CircularQueue Q, SeqStack S) throws Exception {
while (!Q.isEmpty()) {
int ele;
ele = Q.deQueue();
S.push(ele);
}
while (!S.isEmpty()) {
int top;
top = S.pop();
Q.enQueue(top);
}
}
完整代码:
public class Test {
public static void main(String[] args) throws Exception {
CircularQueue queue = new CircularQueue();
queue.init();
queue.enQueue(11);
queue.enQueue(22);
queue.enQueue(33);
queue.enQueue(44);
queue.enQueue(55);
SeqStack stack = new SeqStack();
stack.init();
queue.print();
inversionQueue(queue, stack);
queue.print();
}
public static void inversionQueue(CircularQueue Q, SeqStack S) throws Exception {
while (!Q.isEmpty()) {
int ele;
ele = Q.deQueue();
S.push(ele);
}
while (!S.isEmpty()) {
int top;
top = S.pop();
Q.enQueue(top);
}
}
}
CircularQueue :
public class CircularQueue {
private final int MAXSIZE = 7;
private Queue queue;
public void init() {
queue = new Queue();
queue.data = new int[MAXSIZE];
queue.front = 0;
queue.rear = 0;
}
public boolean isEmpty() {
return queue.rear == queue.front;
}
public boolean isFull() {
return (queue.rear + 1) % MAXSIZE == queue.front;
}
public void enQueue(int ele) throws Exception {
if (isFull()) {
throw new Exception("队列已满不能入队!");
}
queue.data[queue.rear] = ele;
queue.rear = (queue.rear + 1) % MAXSIZE;
}
public int deQueue() throws Exception {
if (isEmpty()) {
throw new Exception("队列为空不能出队!");
}
int front = queue.data[queue.front];
queue.front = (queue.front + 1) % MAXSIZE;
return front;
}
public int size() {
return (queue.rear - queue.front + MAXSIZE) % MAXSIZE;
}
public int getFront() throws Exception {
if (isEmpty()) {
throw new Exception("队空不能获取队头元素!");
}
return queue.data[queue.front];
}
public int getRear() throws Exception {
if (isEmpty()) {
throw new Exception("队空不能获取队尾元素!");
}
return queue.data[(queue.rear - 1 + MAXSIZE) % MAXSIZE];
}
public void clear() {
queue.front = 0;
queue.rear = 0;
}
public void print() {
System.out.print("[");
int front = queue.front;
while (front != queue.rear) {
System.out.print(queue.data[front]);
if (front != (queue.rear - 1 + MAXSIZE) % MAXSIZE) {
System.out.print(", ");
}
front = (front + 1) % MAXSIZE;
}
System.out.print("]\n");
}
}
class Queue {
int[] data;
int front;
int rear;
}
SeqStack :
public class SeqStack {
private final int MAXSIZE = 100;
private Stack stack;
public void init() {
stack = new Stack();
stack.data = new int[MAXSIZE];
stack.top = -1;
}
public boolean isEmpty() {
return stack.top == -1;
}
public void push(int ele) throws Exception {
if (stack.top == MAXSIZE - 1) {
throw new Exception("栈已满,不能再插入!");
}
stack.top++;
stack.data[stack.top] = ele;
}
public int pop() throws Exception {
if (stack.top == -1) {
throw new Exception("栈为空,不能出栈元素!");
}
int result = stack.data[stack.top];
stack.top--;
return result;
}
public int getTop() throws Exception {
if (stack.top == -1) {
throw new Exception("栈为空,不能获取栈顶元素!");
}
return stack.data[stack.top];
}
public int size() {
return stack.top + 1;
}
public void print() {
System.out.print("[");
for (int i = stack.top; i >= 0; i--) {
if (i != stack.top) {
System.out.print(", ");
}
System.out.print(stack.data[i]);
}
System.out.print("]\n");
}
public void clear() {
stack.top = -1;
}
}
class Stack {
int[] data;
int top;
}
执行结果:
[11, 22, 33, 44, 55]
[55, 44, 33, 22, 11]
|