简单的使用(int float64 string):
package main
import (
"fmt"
"sort"
)
func testEazy() {
a := []int{5, 4, 3, 2, 1}
fmt.Printf("a: %v\n", a)
sort.Ints(a)
fmt.Printf("a: %v\n", a)
f := []float64{1.5, 1.2, 1.3, 1.6, 1.5}
fmt.Printf("f: %v\n", f)
sort.Float64s(f)
fmt.Printf("f: %v\n", f)
s := []string{"yisongjie", "wutianjun", "lizilong"}
fmt.Printf("s: %v\n", s)
sort.Strings(s)
fmt.Printf("s: %v\n", s)
s2 := []string{"张三", "李四", "王五"}
fmt.Printf("s2: %v\n", s2)
sort.Strings(s2)
fmt.Printf("s2: %v\n", s2)
}
func main() {
testEazy()
// test_hard()
}
复杂的使用(结构体,二维):
1. 重写Interface
1.1重写Len()
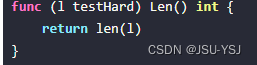
1.2重写Swap(i,j int)
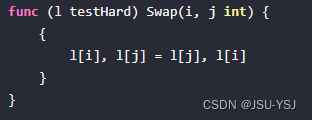
1.3重写排序规则
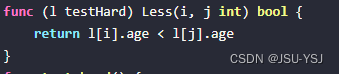
package main
import (
"fmt"
"sort"
)
type Interface interface {
Len() int // Len方法返回集合中的元素个数
Less(i, j int) bool // i>j,该方法返回索引i的元素是否比索引j的元素小、
Swap(i, j int) // 交换i, j的值
}
type People struct {
Name string
age int
}
type testHard []People
// 重写
func (l testHard) Len() int {
return len(l)
}
func (l testHard) Swap(i, j int) {
{
l[i], l[j] = l[j], l[i]
}
}
func (l testHard) Less(i, j int) bool {
return l[i].age < l[j].age
}
func test_hard() {
ls := testHard{
{Name: "张三", age: 20},
{Name: "李四", age: 23},
{Name: "王五", age: 22},
}
fmt.Printf("ls: %v\n", ls)
sort.Sort(ls)
fmt.Printf("ls: %v\n", ls)
}
func main() {
//testEazy()
test_hard()
}
|