《数据结构与算法》 青岛大学-王卓 队列(顺序循环存储)C++
- 数据类型:队列(也是属于线性表)
- 存储方式:顺序循环存储
- 常用名称:顺序循环队列
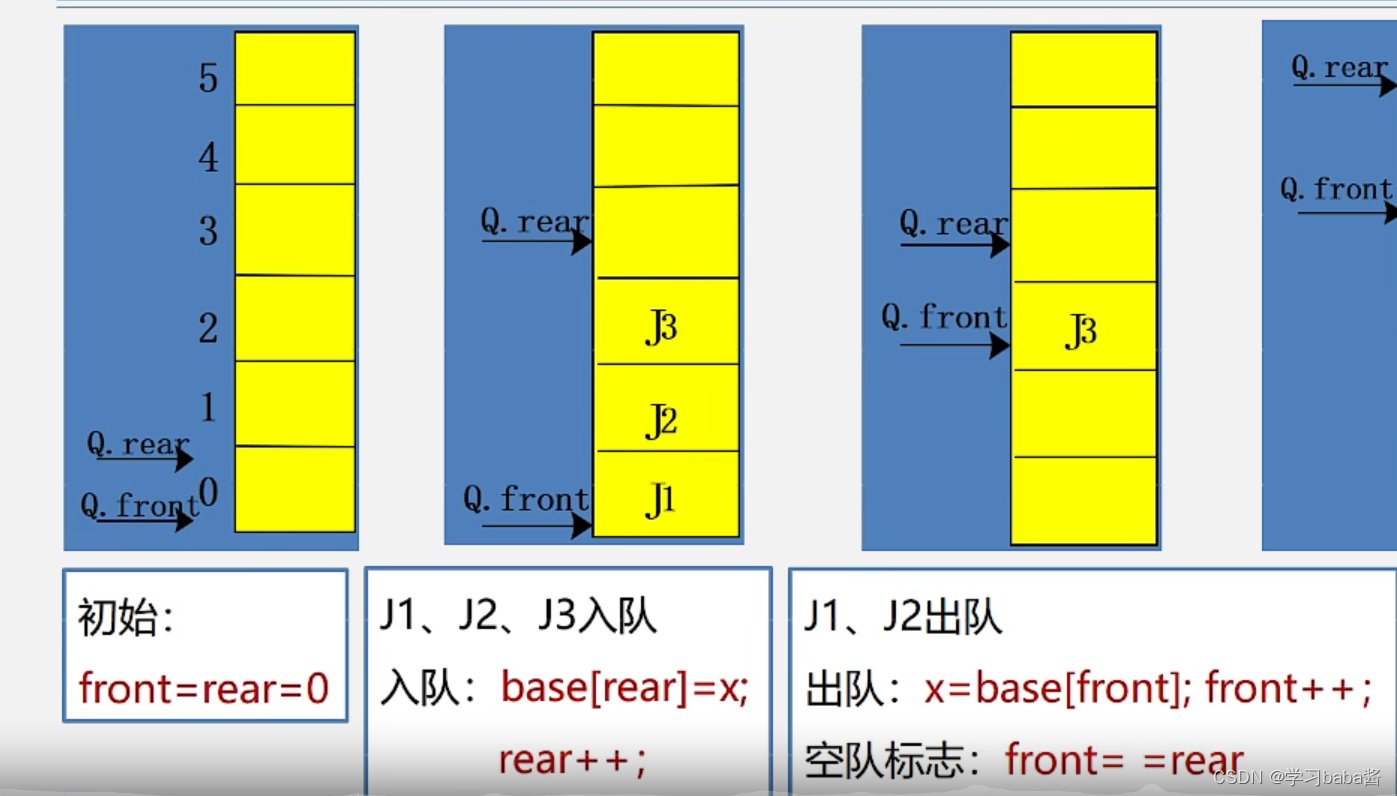 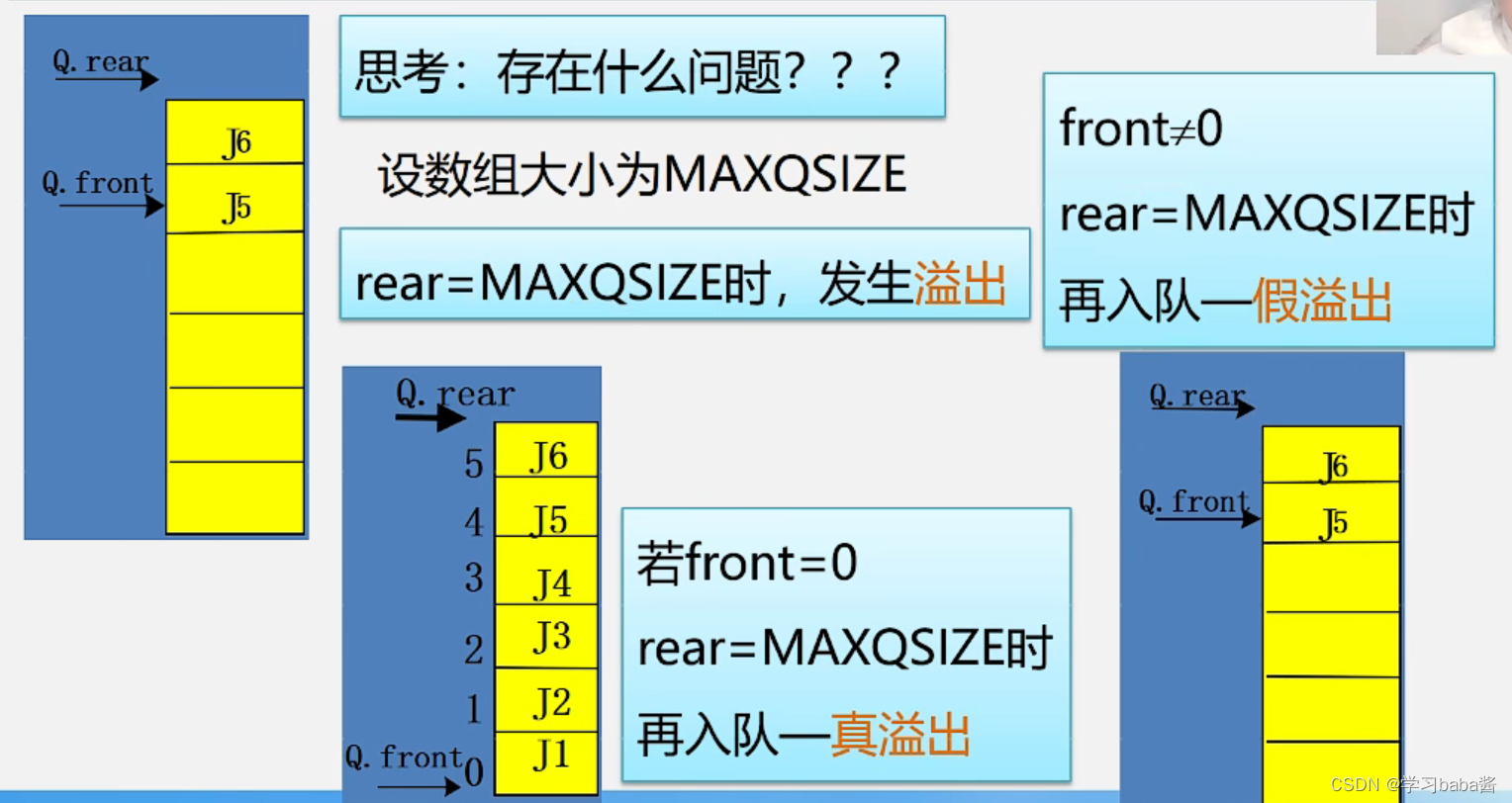 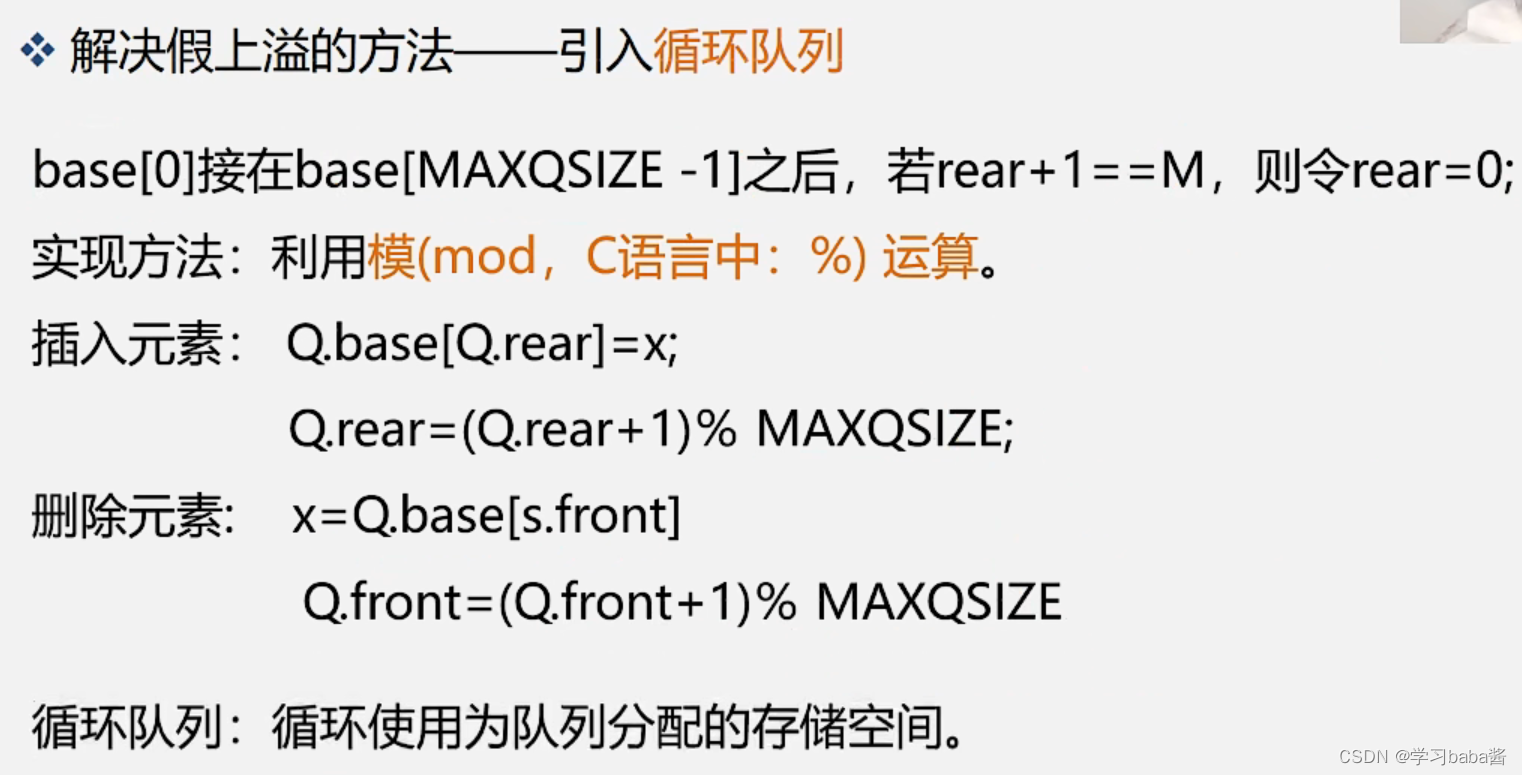 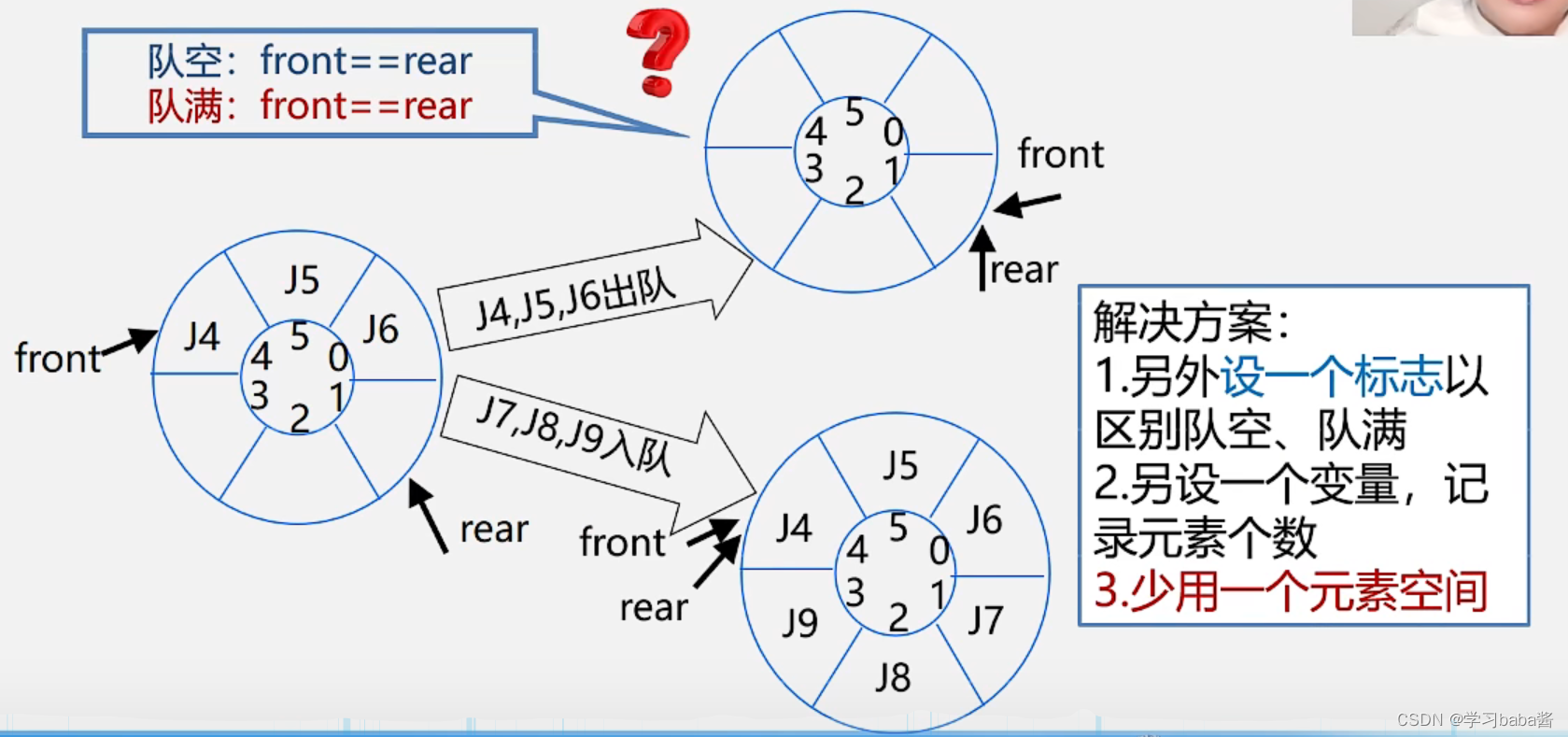 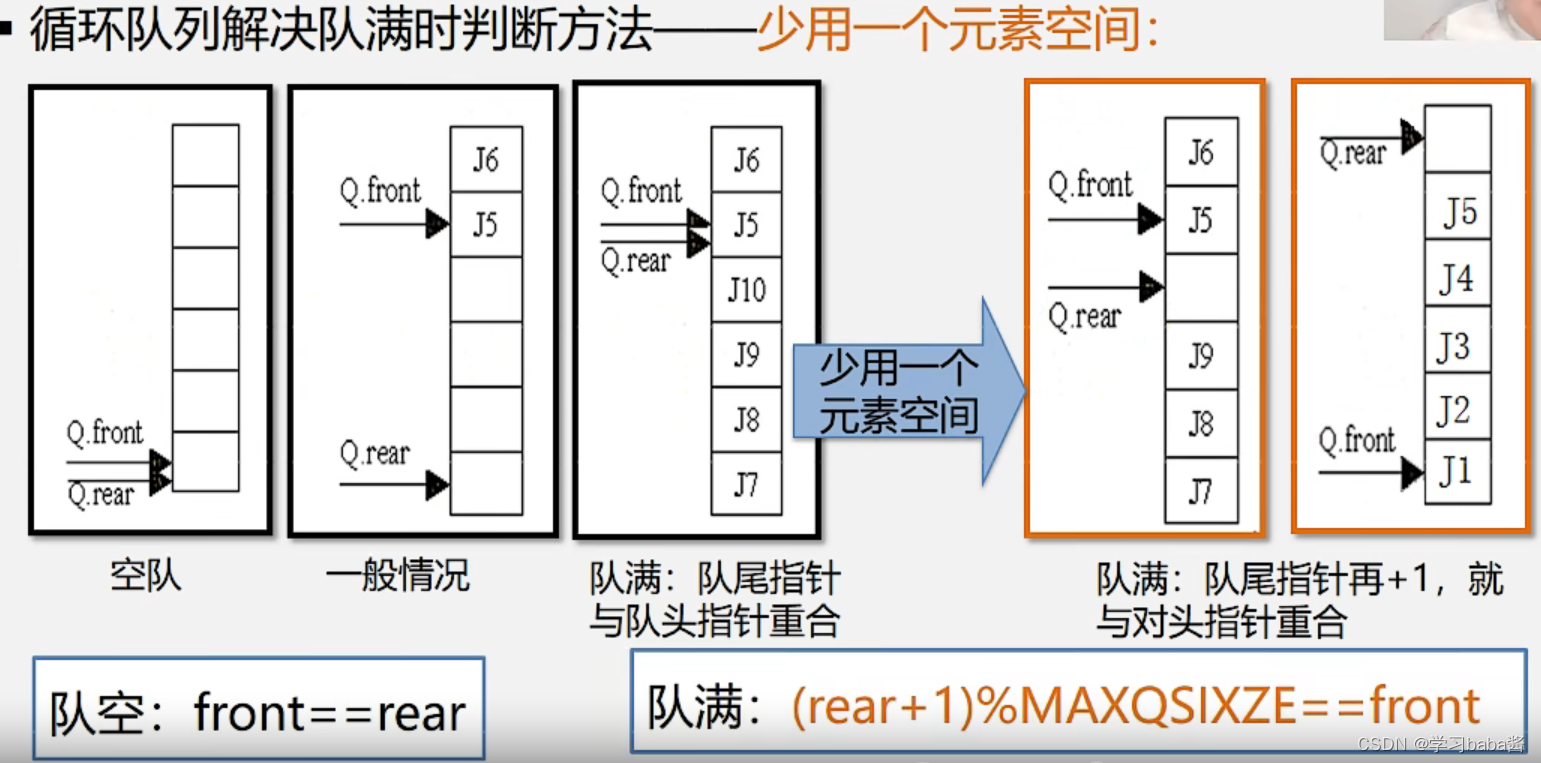
(注:图片截取自《数据结构与算法》-青岛大学王卓bilibili视频)
#include<iostream>
using namespace std;
#define MAX_SIZE 100
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
#define OVERFLOW -2
typedef int Status;
typedef int QElemType;
typedef struct
{
QElemType* base;
int front;
int rear;
}SqQueue;
Status InitQueue(SqQueue& Q)
{
Q.base = new QElemType[MAX_SIZE];
if (!Q.base) exit(OVERFLOW);
Q.front = Q.rear = 0;
return OK;
}
int QueueLength(SqQueue Q)
{
return (Q.rear - Q.front + MAX_SIZE) % MAX_SIZE;
}
Status EnQueue(SqQueue& Q, QElemType e)
{
if ((Q.rear + 1) % MAX_SIZE == Q.front) return ERROR;
Q.base[Q.rear] = e;
Q.rear = (Q.rear + 1) % MAX_SIZE;
return OK;
}
Status DeQueue(SqQueue& Q, QElemType& e)
{
if (Q.front == Q.rear) return ERROR;
e = Q.base[Q.front];
Q.front = (Q.front + 1) % MAX_SIZE;
return OK;
}
QElemType GetHead(SqQueue Q)
{
if (Q.front != Q.rear)
return Q.base[Q.front];
}
int main()
{
SqQueue q;
InitQueue(q);
EnQueue(q, 20);
EnQueue(q, 80);
EnQueue(q, -120);
EnQueue(q, 50);
int e;
int len = QueueLength(q);
for (size_t i = 0; i < len; i++)
{
DeQueue(q, e);
cout << e << endl;
}
return 0;
}
|