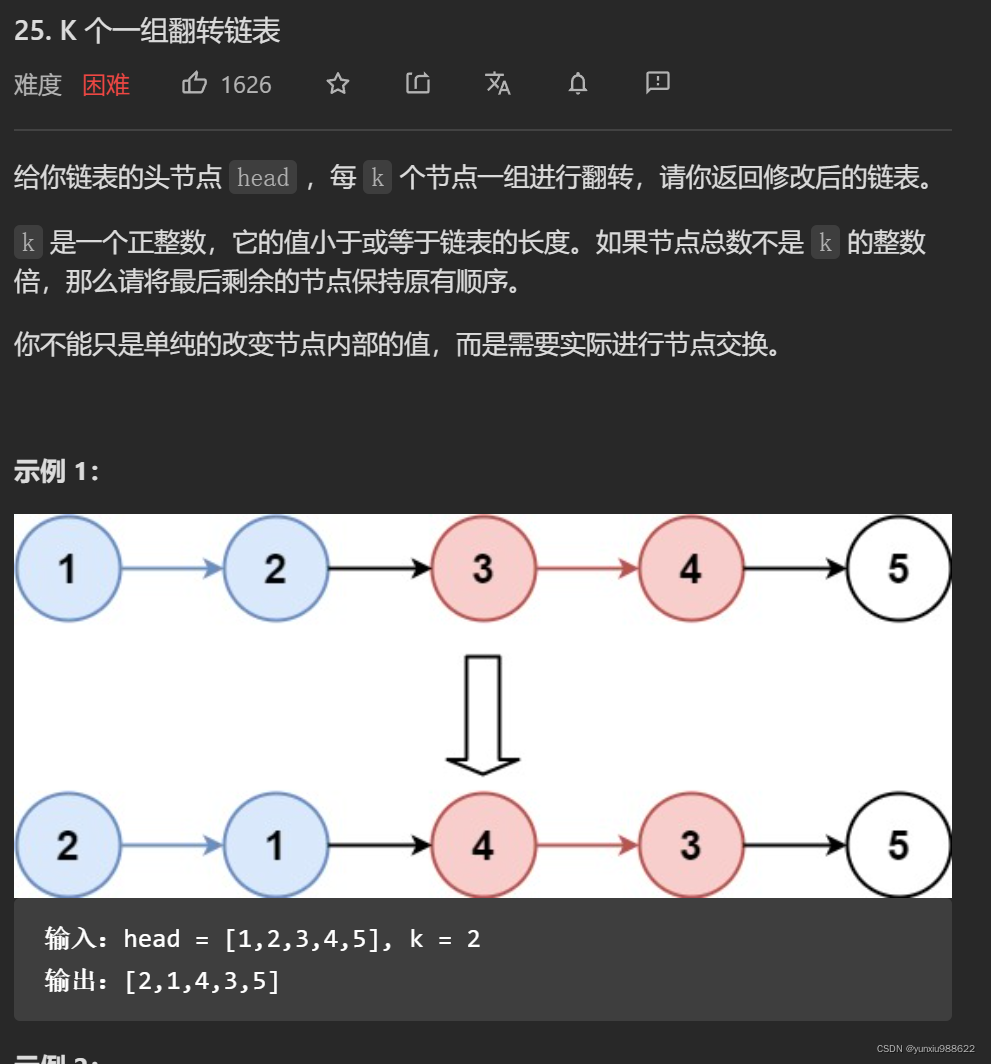 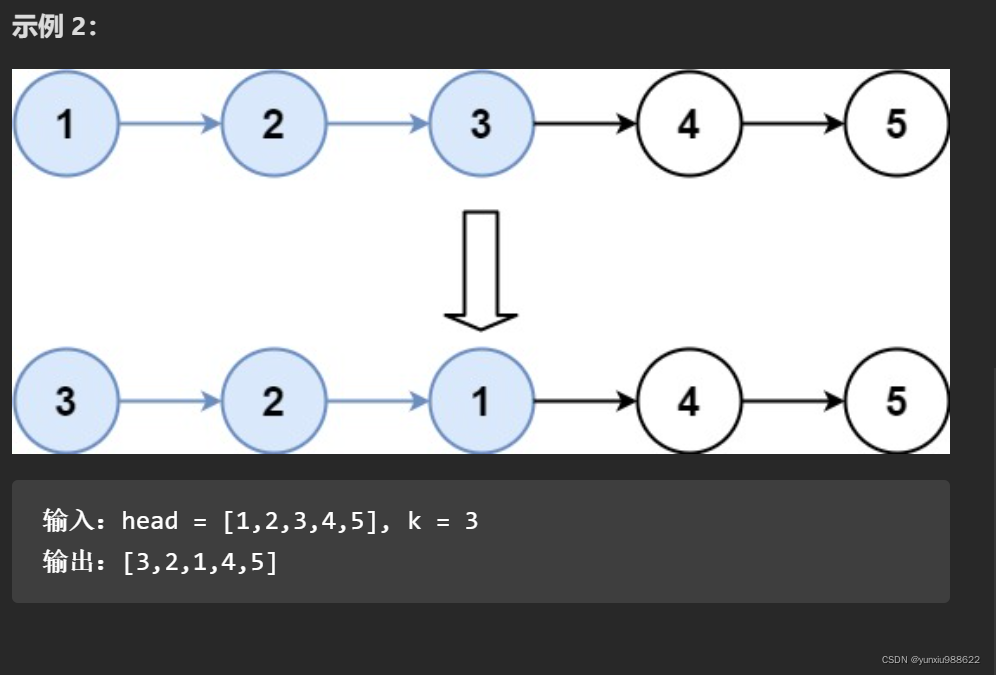 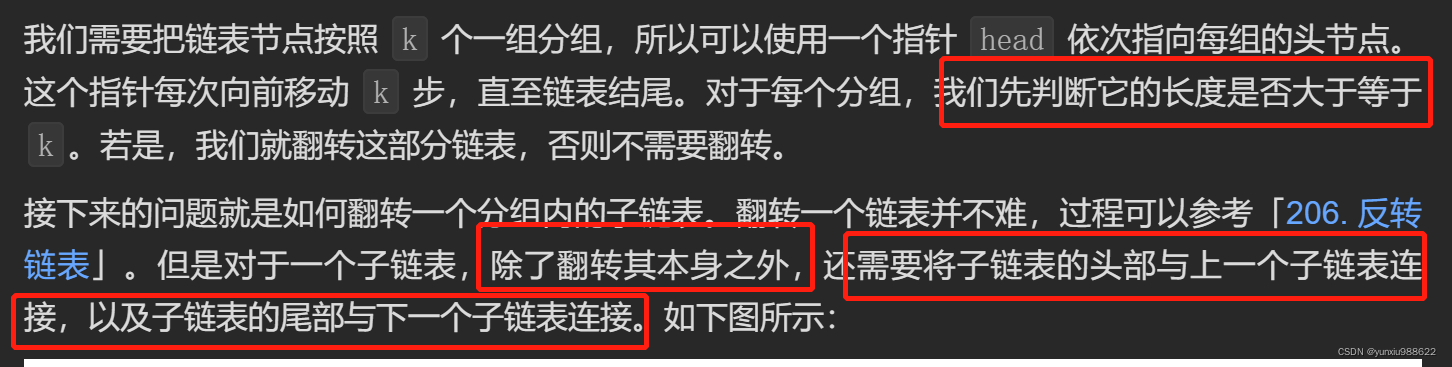
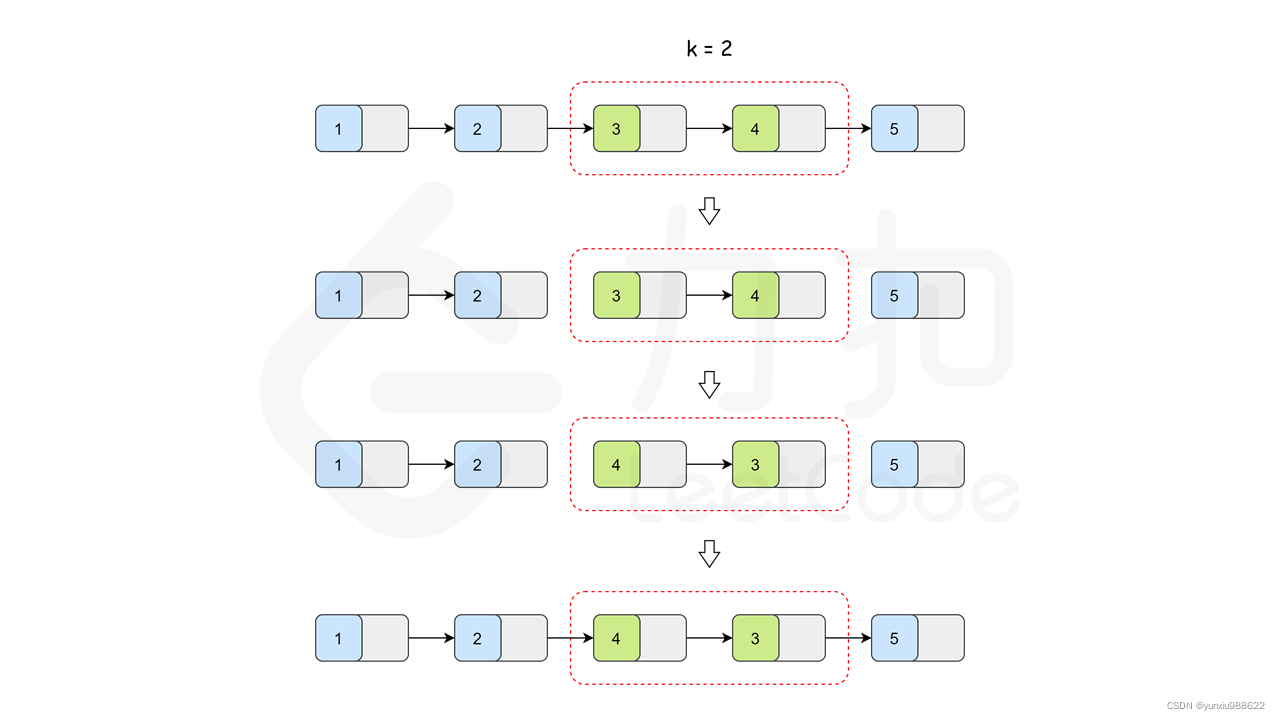 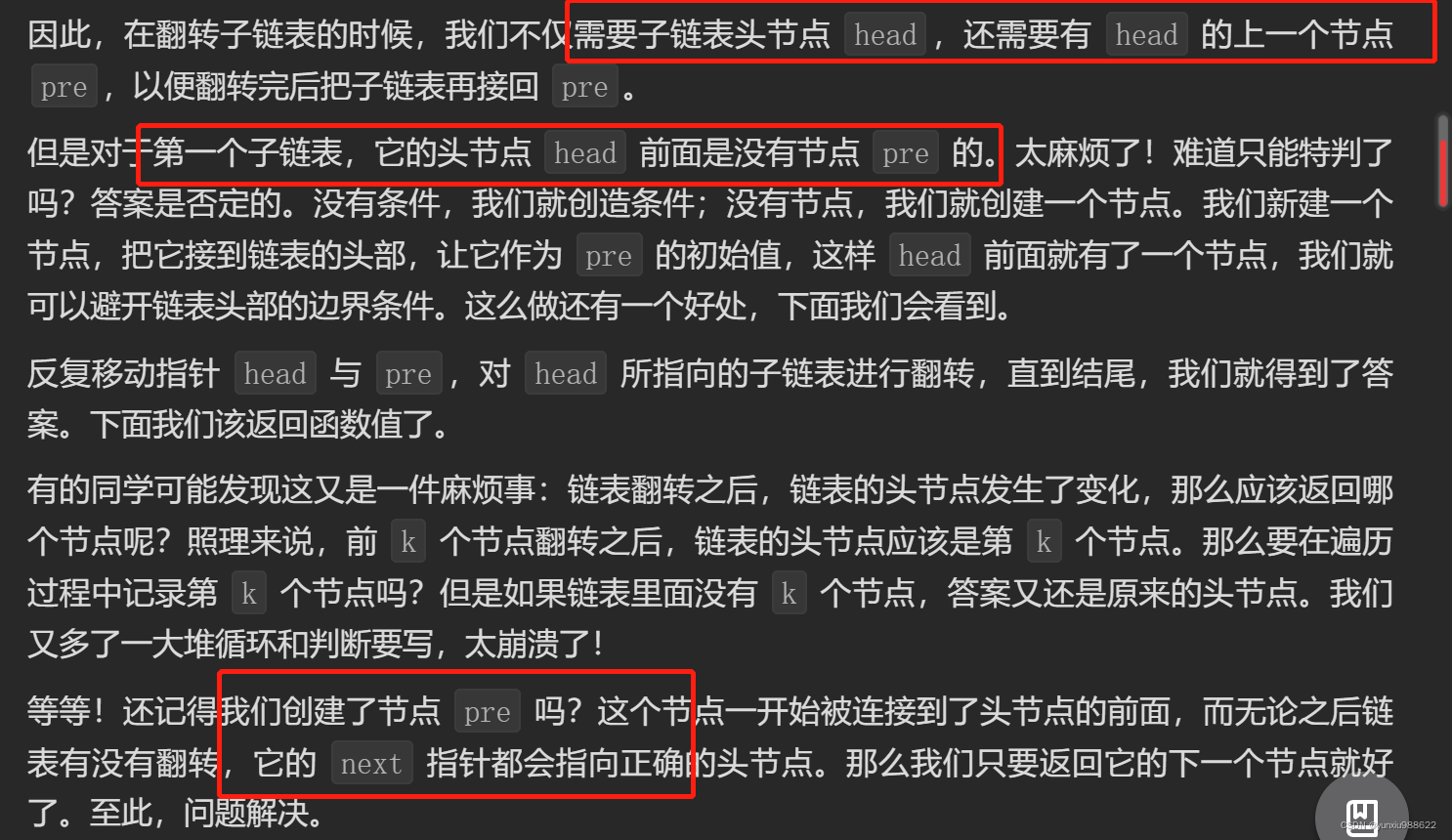 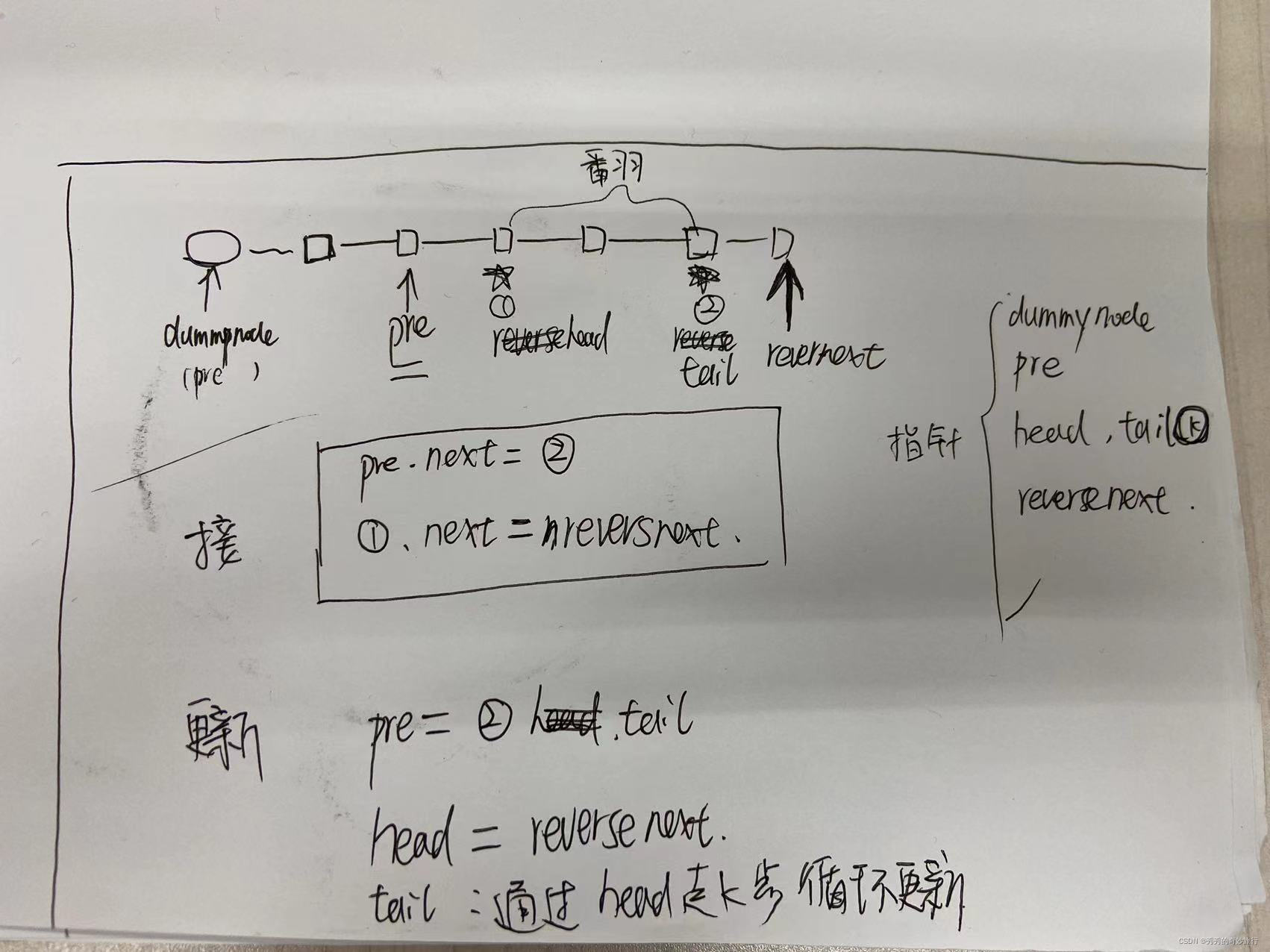
class Solution {
public ListNode reverseKGroup(ListNode head, int k) {
ListNode dummynode=new ListNode(0);
dummynode.next=head;
ListNode pre=dummynode;
ListNode tail=pre;
while(head!=null){
for(int i=0;i<k;i++){
tail=tail.next;
if(tail==null){
return dummynode.next;
}
}
ListNode reversenext=tail.next;
ListNode[] reverse=subreverse(head,tail);
head=reverse[0];
tail=reverse[1];
pre.next=head;
tail.next=reversenext;
pre=tail;
head=tail.next;
}
return dummynode.next;
}
ListNode[] subreverse(ListNode head,ListNode tail){
ListNode pre =null;
ListNode cur=head;
tail.next=null;
while(cur!=null){
ListNode temp=cur.next;
cur.next=pre;
pre=cur;
cur=temp;
}
return new ListNode[]{tail,head};
}
}
|