You are given the?head ?of a linked list, and an integer?k .
Return?the head of the linked list after?swapping?the values of the?kth ?node from the beginning and the?kth ?node from the end (the list is?1-indexed).
Example 1:
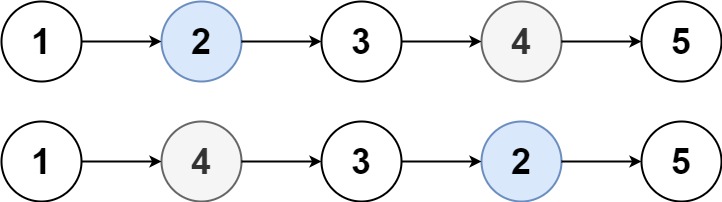
Input: head = [1,2,3,4,5], k = 2
Output: [1,4,3,2,5]
Example 2:
Input: head = [7,9,6,6,7,8,3,0,9,5], k = 5
Output: [7,9,6,6,8,7,3,0,9,5]
Constraints:
- The number of nodes in the list is?
n . 1 <= k <= n <= 105 0 <= Node.val <= 100
题目链接:https://leetcode.com/problems/swapping-nodes-in-a-linked-list/
题目大意:将链表从左往右和从右往左的第k个节点的值交换
题目分析:快指针走k步后记录当且节点,然后快慢指针同时走,快指针走到最后时慢指针位置即为倒序第k个
2ms,时间击败100%
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode swapNodes(ListNode head, int k) {
int cnt = 1;
ListNode cur = head, fir = null;
while (cur != null) {
if (cnt == k) {
fir = cur;
break;
}
cur = cur.next;
cnt++;
}
ListNode slow = head;
while (cur.next != null) {
cur = cur.next;
slow = slow.next;
}
int tmp = fir.val;
fir.val = slow.val;
slow.val = tmp;
return head;
}
}
|