前言: 本文重点实现双向循环链表功能的实现以及讲解 喜欢的老铁留下您宝贵的三连!
双向循环链表图样

创建新节点
ListNode* ListBuyNode(LTDataType x)
{
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode == NULL)
{
perror("malloc fail");
exit(-1);
}
newNode->data = x;
newNode->next = NULL;
newNode->prev = NULL;
return newNode;
}
初始化链表
ListNode* ListInit(ListNode* phead)
{
ListNode* newNode=ListBuyNode(-1);
newNode->next = newNode;
newNode->prev = newNode;
return newNode;
}
链表的打印
void ListPrint(ListNode* phead)
{
ListNode* cur = phead->next;
while (cur != phead)
{
printf("%d ", cur->data);
cur = cur->next;
}
printf("\n");
}
头插步骤样例和代码
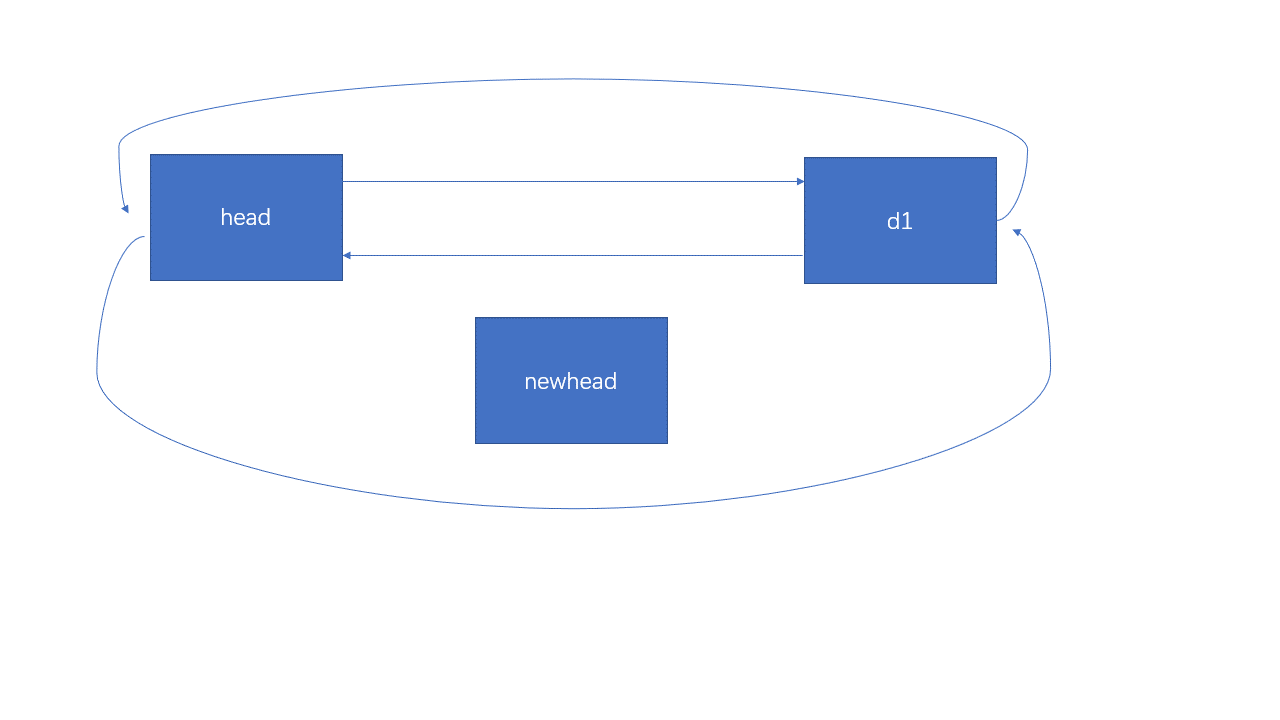 代码:
void ListPushFront(ListNode* phead, LTDataType x)
{
assert(phead);
ListNode* newNode = ListBuyNode(x);
ListNode* cur = phead->next;
phead->next = newNode;
newNode->next = cur;
cur->prev = newNode;
newNode->prev = phead;
}
头删步骤样例和代码
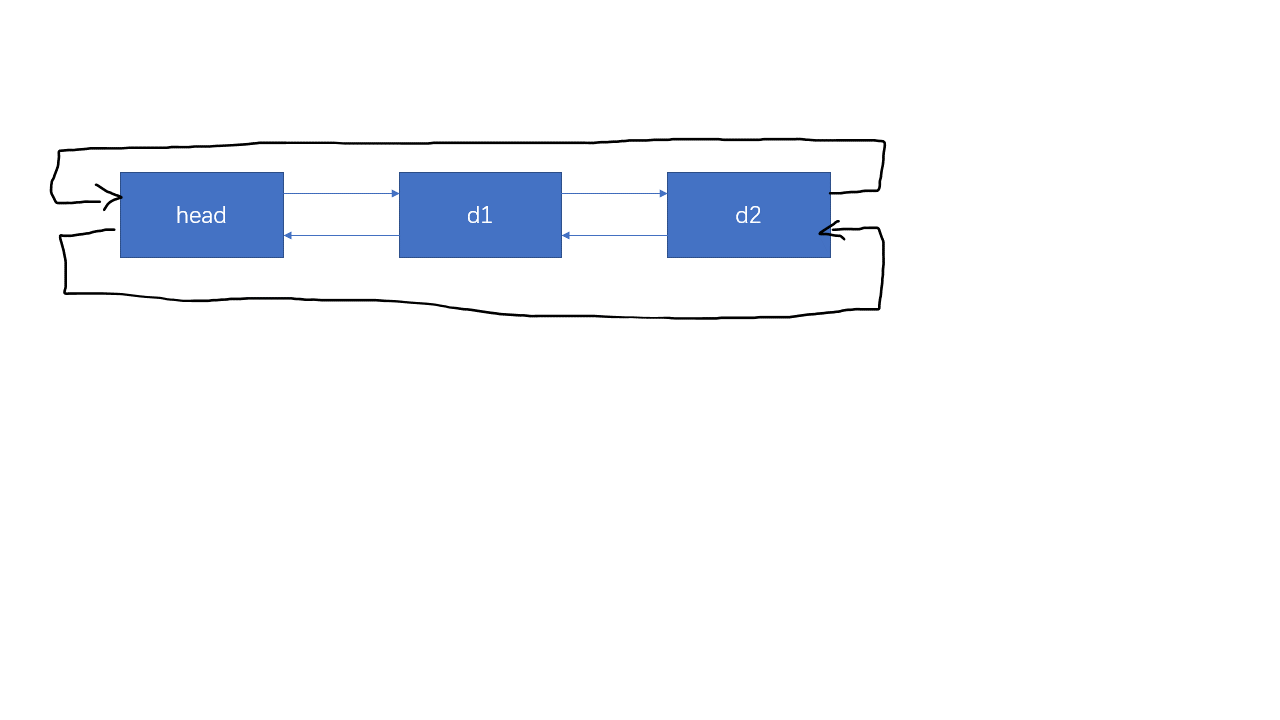 代码:
void ListPopFront(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListNode* cur = phead->next;
phead->next = cur->next;
cur->next->prev = phead;
free(cur);
}
尾插步骤样例和代码
通过head->prev找到tail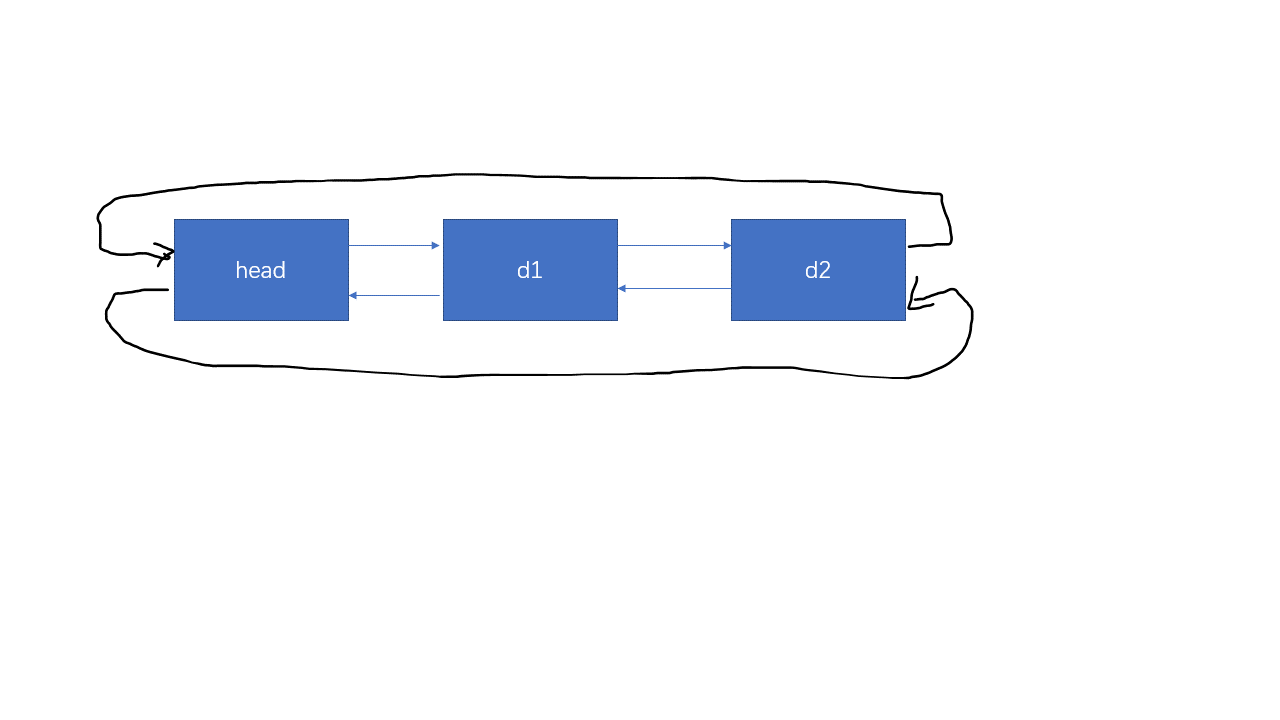 代码:
void ListPushBack(ListNode* phead, LTDataType x)
{
assert(phead);
ListNode* newNode = ListBuyNode(x);
ListNode* tail = phead->prev;
tail->next = newNode;
newNode->prev = tail;
newNode->next = phead;
phead->prev = newNode;
}
尾删步骤样例和代码
通过head->prev找到tail,通过tail->prev找到prev 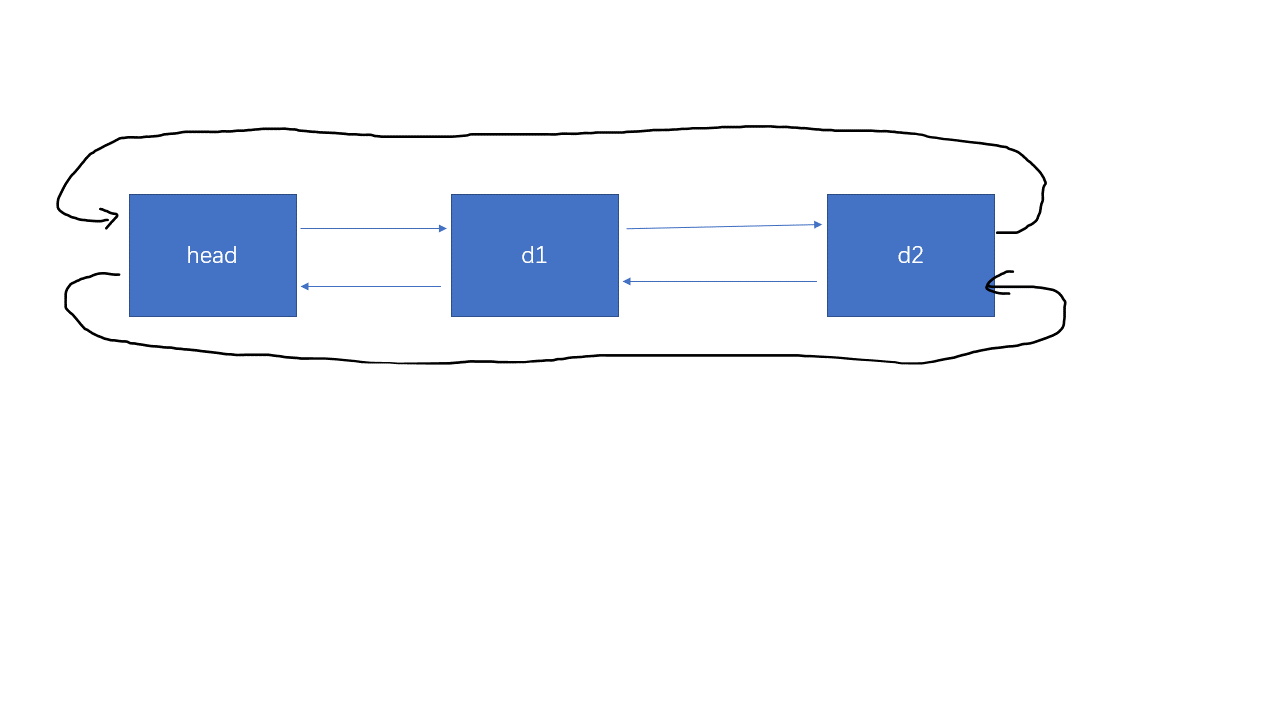 代码:
void ListPopBack(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListNode* tail = phead->prev;
tail->prev->next = phead;
phead->prev = tail->prev;
free(tail);
}
在pos的前面进行插入代码
弄懂了头插和尾插,这就很简单就能实现了,直接看代码吧!!
代码:
void ListInsert(ListNode* pos, LTDataType x)
{
assert(pos);
ListNode* newNode = ListBuyNode(x);
ListNode* cur = pos->prev;
cur->next = newNode;
newNode->next = pos;
pos->prev = newNode;
newNode->prev = cur;
}
查找pos位置
ListNode* ListFind(ListNode* phead, LTDataType x)
{
assert(phead);
ListNode* cur = phead->next;
while (cur != phead)
{
if (cur->data == x)
return cur;
cur = cur->next;
}
return NULL;
}
删除pos位置的节点代码
void ListErase(ListNode* pos)
{
assert(pos);
ListNode* prev = pos->prev;
ListNode* next = pos->next;
prev->next = next;
next->prev = prev;
free(pos);
}
重点(面试官让你十分钟写成链表的秘诀)
头插的优化
void ListPushFront(ListNode* phead, LTDataType x)
{
assert(phead);
ListInsert(phead->next, x);
}
头删的优化
void ListPopFront(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListErase(phead->next);
}
尾插的优化
void ListPushBack(ListNode* phead, LTDataType x)
{
assert(phead);
ListInsert(phead, x);
}
尾删的优化
void ListPopBack(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListErase(phead->prev);
}
链表销毁
void ListDestory(ListNode* phead)
{
ListNode* cur = phead->next;
while (cur != phead)
{
ListNode* next = cur->next;
free(cur);
cur = next;
}
free(phead);
}
完整代码:
头文件
#pragma once
#include<stdio.h>
#include<assert.h>
#include<stdlib.h>
typedef int LTDataType;
typedef struct ListNode
{
LTDataType data;
struct ListNode* next;
struct ListNode* prev;
}ListNode;
ListNode* ListBuyNode(LTDataType x);
ListNode* ListInit(ListNode* phead);
void ListDestory(ListNode* phead);
void ListPrint(ListNode* phead);
void ListPushBack(ListNode* phead, LTDataType x);
void ListPopBack(ListNode* phead);
void ListPushFront(ListNode* phead, LTDataType x);
void ListPopFront(ListNode* phead);
ListNode* ListFind(ListNode* phead, LTDataType x);
void ListInsert(ListNode* pos, LTDataType x);
void ListErase(ListNode* pos);
函数
#define _CRT_SECURE_NO_WARNINGS
#include"ListNode.h"
ListNode* ListBuyNode(LTDataType x)
{
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode == NULL)
{
perror("malloc fail");
exit(-1);
}
newNode->data = x;
newNode->next = NULL;
newNode->prev = NULL;
return newNode;
}
ListNode* ListInit(ListNode* phead)
{
ListNode* newNode=ListBuyNode(-1);
newNode->next = newNode;
newNode->prev = newNode;
return newNode;
}
void ListPrint(ListNode* phead)
{
ListNode* cur = phead->next;
while (cur != phead)
{
printf("%d ", cur->data);
cur = cur->next;
}
printf("\n");
}
void ListPushBack(ListNode* phead, LTDataType x)
{
assert(phead);
ListInsert(phead, x);
}
void ListPopBack(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListErase(phead->prev);
}
void ListPushFront(ListNode* phead, LTDataType x)
{
assert(phead);
ListInsert(phead->next, x);
}
void ListPopFront(ListNode* phead)
{
assert(phead);
assert(phead->next != phead);
ListErase(phead->next);
}
ListNode* ListFind(ListNode* phead, LTDataType x)
{
assert(phead);
ListNode* cur = phead->next;
while (cur != phead)
{
if (cur->data == x)
return cur;
cur = cur->next;
}
return NULL;
}
void ListInsert(ListNode* pos, LTDataType x)
{
assert(pos);
ListNode* newNode = ListBuyNode(x);
ListNode* cur = pos->prev;
cur->next = newNode;
newNode->next = pos;
pos->prev = newNode;
newNode->prev = cur;
}
void ListErase(ListNode* pos)
{
assert(pos);
ListNode* prev = pos->prev;
ListNode* next = pos->next;
prev->next = next;
next->prev = prev;
free(pos);
}
void ListDestory(ListNode* phead)
{
ListNode* cur = phead->next;
while (cur != phead)
{
ListNode* next = cur->next;
free(cur);
cur = next;
}
free(phead);
}
|