leetcode 1019. 链表中的下一个更大节点
一、题目
原题链接:1019. 链表中的下一个更大节点
1.题目描述
给定一个长度为 n 的链表 head
对于列表中的每个节点,查找下一个 更大节点 的值。也就是说,对于每个节点,找到它旁边的第一个节点的值,这个节点的值 严格大于 它的值。
返回一个整数数组 answer ,其中 answer[i] 是第 i 个节点( 从1开始 )的下一个更大的节点的值。如果第 i 个节点没有下一个更大的节点,设置 answer[i] = 0 。
示例 1:
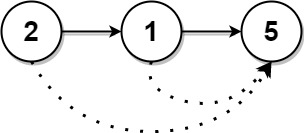
输入:head = [2,1,5] 输出:[5,5,0]
示例 2:
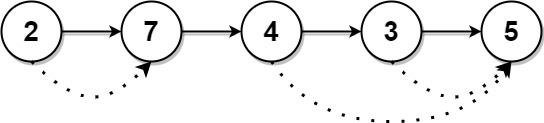
输入:head = [2,7,4,3,5] 输出:[7,0,5,5,0]
提示:
- 链表中节点数为
n 1 <= n <= 10^4 1 <= Node.val <= 10^9
2.基础框架
C++基础框架代码如下:
vector<int> nextLargerNodes(ListNode* head) {
}
3.解题思路
-
维护一个单调递增的队列,即栈底向栈顶的方向,从大到小。 -
栈存储的数据类型为pair<int, int> ,其中first 为结点值val,second 为结点索引。 -
遍历链表结点,当head->val 大于栈顶的值,则进行退栈,将退栈的栈顶元素所在res 数组中的下标,也就是res[st.top().second] 设置为head->val 元素,直到head->val 不大于栈顶值则停止从栈顶退栈。
脑补:我head->val 你们的大王来啦,比我小的栈顶元素,都给我滚出栈,外面将会有res 收留你们,只要以你们的索引st.top().second 进驻,报上我的名字head->val ,别人就知道你的大王是谁啦:res[st.top().second] = head->val 。
-
如果head->val 小于栈顶元素,即满足单调递增栈,将head->val 入栈。 -
当链表结点遍历往后,栈中可能还存在元素,它们对应res中的位置应该设为0,因为栈是单调递增的,后面没有再比它的值大的情况。
-
实现代码: vector<int> nextLargerNodes(ListNode* head) {
stack<pair<int, int> > st;
vector<int> res(10001, 0);
int i = 0;
while (head)
{
while (!st.empty() && head->val > st.top().first)
{
int index = st.top().second;
st.pop();
res[index] = head->val;
}
st.push({head->val, i});
head = head->next;
++i;
}
while (!st.empty())
{
int index = st.top().second;
st.pop();
res[index] = 0;
}
res.resize(i);
return res;
}
4.知识点
? 单调栈
|