剑指offer 63.股票的最大利润 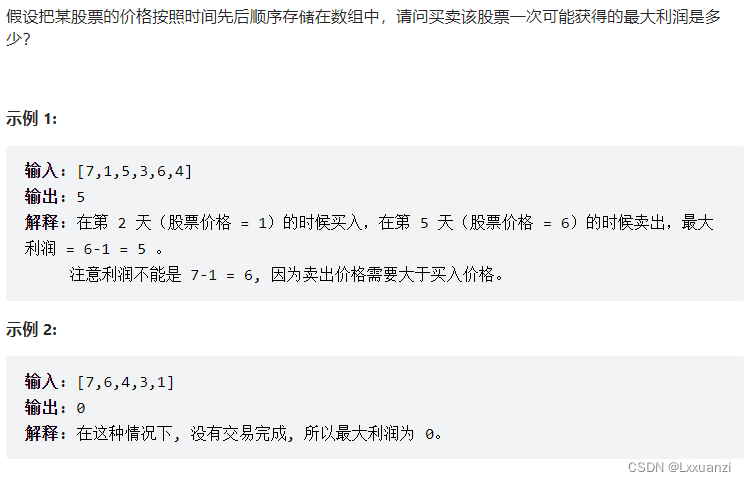
class Solution {
public int maxProfit(int[] prices) {
int buy = Integer.MAX_VALUE, maxProfit = 0;
for(int price : prices){
buy = Math.min(price, buy);
maxProfit = Math.max(maxProfit, price - buy);
}
return maxProfit;
}
}
剑指offer 42.连续子数组的最大和 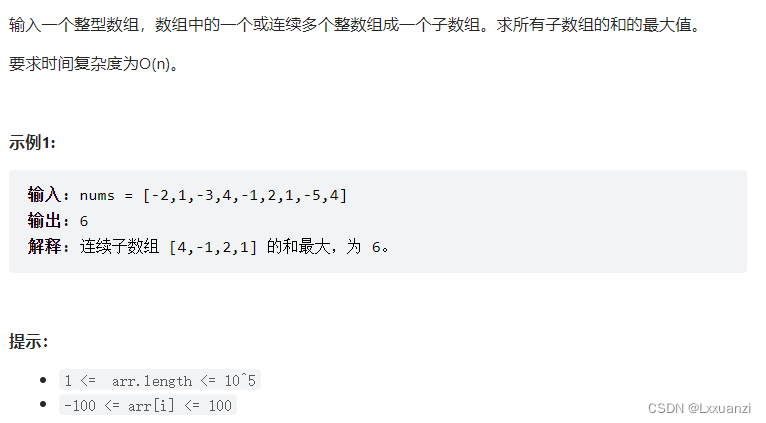
class Solution {
public int maxSubArray(int[] nums) {
int res = nums[0];
for(int i = 1; i < nums.length; i++){
nums[i] += Math.max(nums[i - 1], 0);
res = Math.max(nums[i], res);
}
return res;
}
}
剑指offer 47.礼物的最大价值 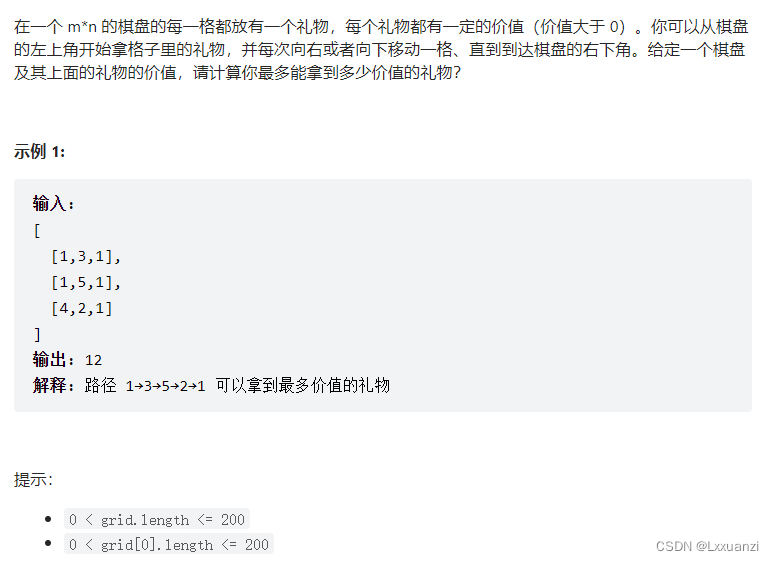
class Solution {
public int maxValue(int[][] grid) {
int m = grid.length, n = grid[0].length;
for(int i = 0; i < m; i++){
for(int j = 0; j < n; j++){
if(i == 0 && j == 0) continue;
if(i == 0) grid[i][j] += grid[i][j - 1];
else if(j == 0) grid[i][j] += grid[i - 1][j];
else grid[i][j] += Math.max(grid[i - 1][j], grid[i][j - 1]);
}
}
return grid[m - 1][n - 1];
}
}
剑指offer 46.把数字翻译成字符串 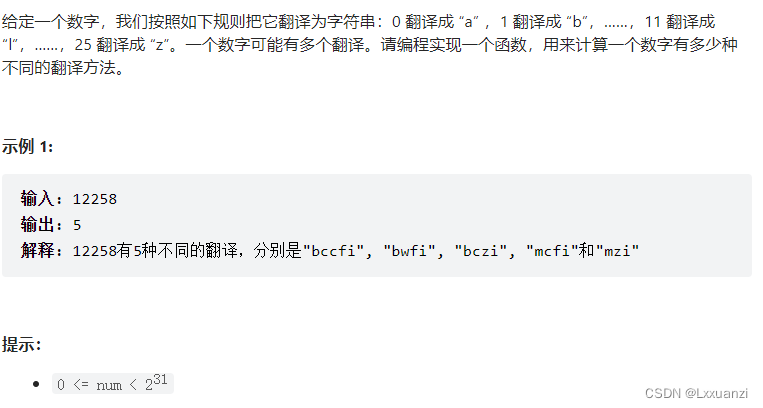
解题思路:
compareTo()方法
tmp.compareTo("10") : 如果tmp > 10 , 则返回一个大于0 的值
tmp.compareTo("25") : 如果tmp < 25 , 则返回一个小于0 的值
tmp.compareTo("10") >= 0 && tmp.compareTo("25") <= 0 : 说明tmp 的值在[10, 25] 区间之内
class Solution {
public int translateNum(int num) {
String str = String.valueOf(num);
int[] dp = new int[str.length() + 1];
dp[0] = dp[1] = 1;
for(int i = 2; i <= str.length(); i++){
String tmp = str.substring(i - 2, i);
if(tmp.compareTo("10") >= 0 && tmp.compareTo("25") <= 0){
dp[i] = dp[i - 2] + dp[i - 1];
}else{
dp[i] = dp[i - 1];
}
}
return dp[str.length()];
}
}
|