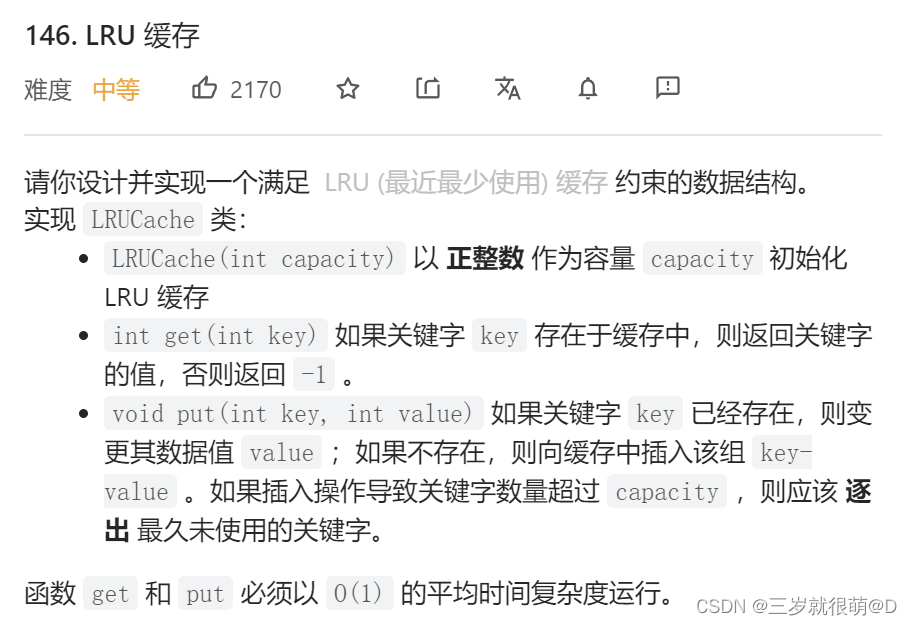 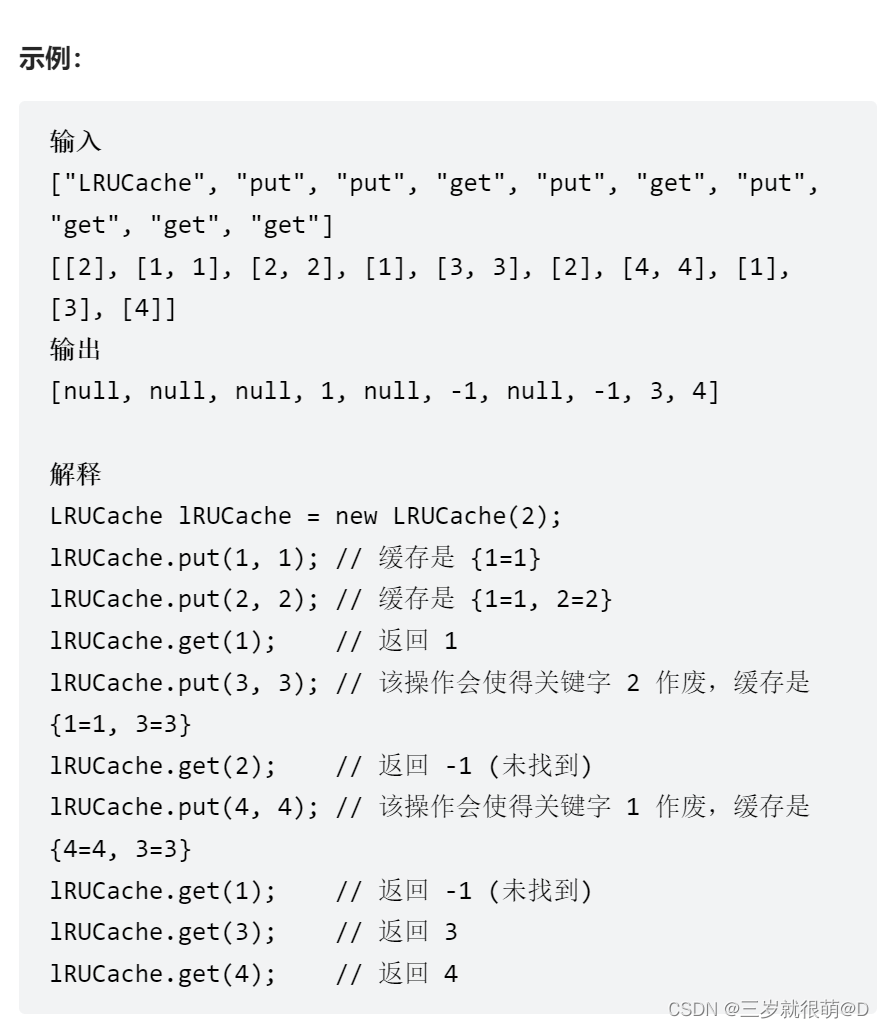 LRU讲的很不错https://leetcode.cn/problems/lru-cache/solution/lru-ce-lue-xiang-jie-he-shi-xian-by-labuladong/
哈希双链表
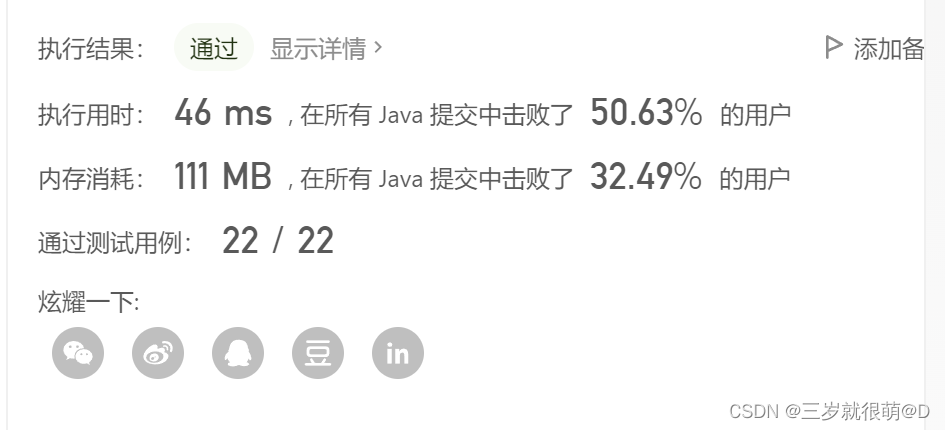
class LRUCache {
class Node{
int key;
int val;
Node prev;
Node next;
public Node(int key,int val){
this.key = key;
this.val = val;
}
}
class DoubleList{
private Node head;
private Node tail;
private int size;
public DoubleList(){
this.head = new Node(0,0);
this.tail = new Node(0,0);
head.next = tail;
tail.prev = head;
size = 0;
}
public void addLast(Node x){
x.prev = tail.prev;
x.next = tail;
tail.prev.next = x;
tail.prev = x;
size += 1;
}
public void remove(Node x){
x.prev.next = x.next;
x.next.prev = x.prev;
size -= 1;
}
public int getSize(){
return size;
}
public Node removeFirst(){
if(head.next == tail)
return null;
Node first = head.next;
remove(first);
return first;
}
}
private Map<Integer,Node> map;
private DoubleList cache;
private int cap;
public LRUCache(int capacity) {
this.map = new HashMap<>();
this.cache = new DoubleList();
this.cap = capacity;
}
public void makeRecently(int key){
Node x = map.get(key);
cache.remove(x);
cache.addLast(x);
}
public int get(int key) {
if(map.containsKey(key) == false)
return -1;
makeRecently(key);
return map.get(key).val;
}
public void put(int key, int value) {
if(map.containsKey(key) == true){
Node node = map.get(key);
node.val = value;
makeRecently(key);
return ;
}
if(cache.getSize() == cap){
Node first = cache.removeFirst();
map.remove(first.key);
}
Node x = new Node(key,value);
map.put(key,x);
cache.addLast(x);
}
}
|