完整源代码及文件在资源链接:
C++实现通讯录管理系统(OOP类,链表,文件读取等操作实现通讯录的基本功能) 下载后可直接运行使用
分析
1.需求分析
需求分析 1、具有联系人基本信息的添加、修改、删除、显示信息和查询功能的通讯录管理系统。 2、联系人数据:姓名,性别,索引、电话号码和QQ账号,电子邮件。 3、可对记录中的姓名和电话号码和QQ账号进行修改。 4、可增加和删除联系人。 5、可显示所有的联系人信息。 6、可按人名或电话号码进行查询联系人基本信息或联系人是否存在。
2.设计
1.添加联系人。 2.查询联系人 3.修改联系人 4.删除联系人 5.显示联系人基本信息 6.保存联系人基本信息到文件
3.抽象类型定义
class Info
{
public:
Info();
friend class AddressBook;
#ifdef _VC2010_VER_
friend ofstream & operator << (ofstream &,const Info &);
friend ifstream & operator >> (ifstream &, Info &);
#endif
private:
Info(const Info &);
const Info &operator=(const Info &);
char name[20];
char gender[5];
char phone[50];
char qq[20];
char email[50];
int index;
Info * next;
};
通讯录管理系统功能介绍
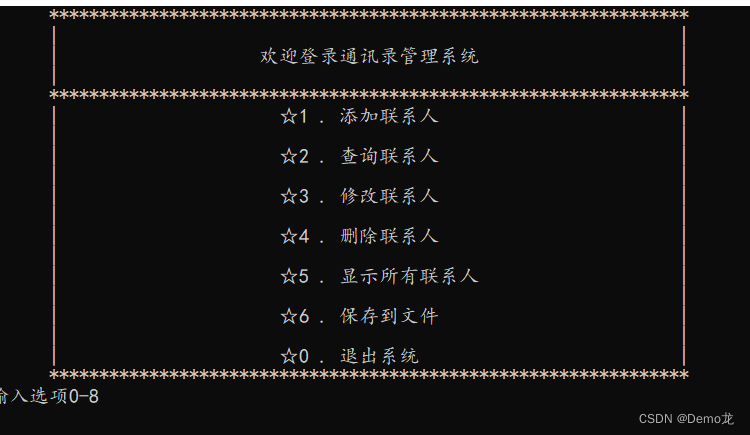
1.添加联系人
利用链表存储数据,以链式存储结构存储通讯录数据。 使用链表存储数据,方便删除和修改,提高了代码灵活性
void AddressBook::Add()
{
cout << "请输入联系人信息: " <<endl;
Info * temp = new Info;
cout << "姓名( 1 ~ " << sizeof(temp->name) - 1 << " 字符): " << ends;
cin >> temp->name;
cout << "性别( 男 女 ): " << ends;
cin >> temp->gender;
cout << "电话( 1 ~ " << sizeof(temp->phone) - 1 << " 字符): " << ends;
cin >> temp->phone;
cout << "QQ( 1 ~ " << sizeof(temp->qq) - 1 << " 字符): " << ends;
cin >> temp->qq;
cout << "email( 0 ~ " << sizeof(temp->email) - 1 << " 字符): " << ends;
cin >> temp->email;
AddBase(temp);
}
2.查询联系人
输入基本信息后进入文件函数中,打开并读取文件,从而查询联系人
void AddressBook::Query()
{
cout << " 请选择查询方式 " << endl;
cout << " 0. 按名字 " << endl;
cout << " 1. 电话 " << endl;
int choose = 0;
cin >> choose;
char str[50] = {0};
switch (choose)
{
case 0:
cout << "请输入名字: " << ends;
cin >> str;
break;
case 1:
cout << "请输入电话: " << ends;
cin >> str;
break;
default:
cout << "没有该选项" << endl;
return;
}
Info * result = head;
ShowInfoTitle();
int index = 0;
do
{
result = QueryBase(result, choose, str);
if (result!= NULL)
{
result->index = index;
ShowInfo(result);
index++;
result = result->next;
}
} while (result != NULL);
}
3.修改联系人
输入要修改的联系人基本信息,查找是否存在后,进行修改(此处的修改是利用DelBase(index)函数删除原有的,利用Add()函数添加新的联系人),这就是使用链表的好处。
void AddressBook::Modify()
{
cout << "请选择要修改的联系人(输入序号): " << endl;
int count = ShowAllInfo();
int index = 0;
cin >> index;
if (index < 0 || index >= count)
{
cout << "选择无效, 没有该序号的联系人" << endl;
return;
}
DelBase(index);
Add();
}
4.删除联系人
先判断联系人是否存在,若存在,利用DelBase(index);进行链表的删除操作
void AddressBook::Del()
{
cout << "请选择要删除的联系人(输入序号): " << endl;
int count = ShowAllInfo();
int index = 0;
cin >> index;
if (index < 0 || index >= count)
{
cout << "选择无效, 没有该序号的联系人" << endl;
return;
}
DelBase(index);
}
5.显示所有联系人
void AddressBook::ShowAll()
{
ShowAllInfo();
}
6.保存到文件
将通讯录中的数据保存在data.txt文件中 1.可读取 2.可写入
void AddressBook::Load()
{
ifstream ifile(".\\data.txt");
if (!ifile)
{
cout << "打开文件失败" << endl;
return;
}
while (!ifile.eof())
{
Info * temp = new Info;
#ifdef _VC2010_VER_
ifile >> *temp;
#else
ifile >> temp->name >> temp->gender >> temp->phone >> temp->qq >> temp->email;
#endif
if (ifile)
{
AddBase(temp);
}
}
ifile.close();
}
void AddressBook::Save()
{
ofstream ofile(".\\data.txt", ios::trunc);
if (!ofile)
{
cout << "保存失败" << endl;
return;
}
Info * temp = head;
while (temp != NULL)
{
#ifdef _VC2010_VER_
ofile <<* temp<<endl;
#else
ofile << temp->name << " " << temp->gender << " " << temp->phone << " " << temp->qq << " " << temp->email << " " << endl;
#endif
temp = temp->next;
}
ofile.close();
}
0.退出系统
其他副函数
1.按照序号删除
进行链表的删除操作。
void AddressBook::DelBase(int index)
{
Info * temp = head;
Info ** parent = &head;
while (temp != NULL)
{
if (temp->index == index)
{
*parent = temp->next;
delete temp;
return;
}
parent = &temp->next;
temp = temp->next;
}
}
2.输出联系人信息
void AddressBook::ShowInfoTitle()
{
cout << "序号 姓名 性别 电话 QQ email" << endl;
}
void AddressBook::ShowInfo(const Info * pInfo)
{
cout << setiosflags(ios::left)
<< setw(14) << pInfo->index
<< setw(14) << pInfo->name
<< setw(14) << pInfo->gender
<< setw(14) << pInfo->phone
<< setw(14) << pInfo->qq
<< setw(14) << pInfo->email << endl;
}
3.输出序号
int AddressBook::ShowAllInfo()
{
int index = 0;
Info * temp = head;
ShowInfoTitle();
while (temp)
{
temp->index = index;
index++;
ShowInfo(temp);
temp = temp->next;
}
return index;
}
4.主菜单函数
int menu()
{
system("cls");
cout<<" ****************************************************************"<<endl;
cout<<" | |"<<endl;
cout<<" | 欢迎登录通讯录管理系统 |"<<endl;
cout<<" | |"<<endl;
cout<<" ****************************************************************"<<endl;
cout<<" | ☆1 . 添加联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆2 . 查询联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆3 . 修改联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆4 . 删除联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆5 . 显示所有联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆6 . 保存到文件 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆0 . 退出系统 |"<<endl;
cout<<" ****************************************************************"<<endl;
int m = 0;
do
{
cout << "请输入选项0-8\n";
cin >> m;
} while( m < 0 || m > 8 );
return m;
}
main函数
#include "addressmain.h"
int main()
{
int m = 0;
AddressBook book;
book.Load();
do
{
m = menu();
switch (m)
{
case 0:
exit();
break;
case 1:
book.Add();
break;
case 2:
book.Query();
break;
case 3:
book.Modify();
break;
case 4:
book.Del();
break;
case 5:
book.ShowAll();
break;
case 6:
book.Save();
break;
default:
cout << "暂不支持该选项\n" << endl;
}
cout << "回车继续..." << endl;
getch();
} while (m > 0);
book.Save();
return 0;
}
void exit();
int menu();
end
完整源代码及文件在资源链接: C++实现通讯录管理系统(OOP类,链表,文件读取等操作实现通讯录的基本功能) 下载后可直接运行使用
完整方法代码
Info::Info()
{
memset(name, 0, sizeof(name));
memset(gender,0, sizeof(gender));
memset(phone, 0, sizeof(phone));
memset(qq, 0, sizeof(qq));
memset(email, 0, sizeof(email));
index = 0;
next = NULL;
}
#ifdef _VC2010_VER_
ofstream & operator << (ofstream & ofs,const Info & c)
{
ofs << c.name << " "<< c.gender << " " << c.phone << " " << c.qq << " " << c.email << " ";
return ofs;
}
ifstream & operator >> (ifstream & ifs,Info & c)
{
ifs >> c.name >> c.gender >> c.phone >> c.qq >> c.email;
return ifs;
}
#endif
AddressBook::AddressBook()
{
head = NULL;
}
AddressBook::~AddressBook()
{
Info * temp = head;
while (head != NULL)
{
temp = head;
head = head->next;
delete temp;
}
}
void AddressBook::Load()
{
ifstream ifile(".\\data.txt");
if (!ifile)
{
cout << "打开文件失败" << endl;
return;
}
while (!ifile.eof())
{
Info * temp = new Info;
#ifdef _VC2010_VER_
ifile >> *temp;
#else
ifile >> temp->name >> temp->gender >> temp->phone >> temp->qq >> temp->email;
#endif
if (ifile)
{
AddBase(temp);
}
}
ifile.close();
}
void AddressBook::Save()
{
ofstream ofile(".\\data.txt", ios::trunc);
if (!ofile)
{
cout << "保存失败" << endl;
return;
}
Info * temp = head;
while (temp != NULL)
{
#ifdef _VC2010_VER_
ofile <<* temp<<endl;
#else
ofile << temp->name << " " << temp->gender << " " << temp->phone << " " << temp->qq << " " << temp->email << " " << endl;
#endif
temp = temp->next;
}
ofile.close();
}
void AddressBook::Add()
{
cout << "请输入联系人信息: " <<endl;
Info * temp = new Info;
cout << "姓名( 1 ~ " << sizeof(temp->name) - 1 << " 字符): " << ends;
cin >> temp->name;
cout << "性别( 男 女 ): " << ends;
cin >> temp->gender;
cout << "电话( 1 ~ " << sizeof(temp->phone) - 1 << " 字符): " << ends;
cin >> temp->phone;
cout << "QQ( 1 ~ " << sizeof(temp->qq) - 1 << " 字符): " << ends;
cin >> temp->qq;
cout << "email( 0 ~ " << sizeof(temp->email) - 1 << " 字符): " << ends;
cin >> temp->email;
AddBase(temp);
}
void AddressBook::Query()
{
cout << " 请选择查询方式 " << endl;
cout << " 0. 按名字 " << endl;
cout << " 1. 电话 " << endl;
int choose = 0;
cin >> choose;
char str[50] = {0};
switch (choose)
{
case 0:
cout << "请输入名字: " << ends;
cin >> str;
break;
case 1:
cout << "请输入电话: " << ends;
cin >> str;
break;
default:
cout << "没有该选项" << endl;
return;
}
Info * result = head;
ShowInfoTitle();
int index = 0;
do
{
result = QueryBase(result, choose, str);
if (result!= NULL)
{
result->index = index;
ShowInfo(result);
index++;
result = result->next;
}
} while (result != NULL);
}
void AddressBook::Modify()
{
cout << "请选择要修改的联系人(输入序号): " << endl;
int count = ShowAllInfo();
int index = 0;
cin >> index;
if (index < 0 || index >= count)
{
cout << "选择无效, 没有该序号的联系人" << endl;
return;
}
DelBase(index);
Add();
}
void AddressBook::Del()
{
cout << "请选择要删除的联系人(输入序号): " << endl;
int count = ShowAllInfo();
int index = 0;
cin >> index;
if (index < 0 || index >= count)
{
cout << "选择无效, 没有该序号的联系人" << endl;
return;
}
DelBase(index);
}
void AddressBook::ShowAll()
{
ShowAllInfo();
}
void AddressBook::AddBase(Info * pInfo)
{
Info ** parent = &head;
Info * temp = head;
while (temp)
{
if (strcmp(temp->name, pInfo->name) >= 0)
{
break;
}
parent = &temp->next;
temp = temp->next;
}
pInfo->next = temp;
*parent = pInfo;
}
Info * AddressBook::QueryBase(Info * start, int choose, const char * str)
{
while (start != NULL)
{
switch (choose)
{
case 0:
if (strcmp(start->name, str) == 0)
{
return start;
}else{
start=start->next;
continue;
}
break;
case 1:
if (strcmp(start->phone, str) == 0)
{
return start;
}else{
start=start->next;
continue;
}
break;
default:
break;
}
return NULL;
}
return start;
}
void AddressBook::DelBase(int index)
{
Info * temp = head;
Info ** parent = &head;
while (temp != NULL)
{
if (temp->index == index)
{
*parent = temp->next;
delete temp;
return;
}
parent = &temp->next;
temp = temp->next;
}
}
void AddressBook::ShowInfoTitle()
{
cout << "序号 姓名 性别 电话 QQ email" << endl;
}
void AddressBook::ShowInfo(const Info * pInfo)
{
cout << setiosflags(ios::left)
<< setw(14) << pInfo->index
<< setw(14) << pInfo->name
<< setw(14) << pInfo->gender
<< setw(14) << pInfo->phone
<< setw(14) << pInfo->qq
<< setw(14) << pInfo->email << endl;
}
int AddressBook::ShowAllInfo()
{
int index = 0;
Info * temp = head;
ShowInfoTitle();
while (temp)
{
temp->index = index;
index++;
ShowInfo(temp);
temp = temp->next;
}
return index;
}
void exit()
{
cout << " ****************************************************************"<<endl;
cout << " ******************* 谢谢使用 *****************"<<endl;
cout << " ****************************************************************"<<endl;
}
int menu()
{
system("cls");
cout<<" ****************************************************************"<<endl;
cout<<" | |"<<endl;
cout<<" | 欢迎登录通讯录管理系统 |"<<endl;
cout<<" | |"<<endl;
cout<<" ****************************************************************"<<endl;
cout<<" | ☆1 . 添加联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆2 . 查询联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆3 . 修改联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆4 . 删除联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆5 . 显示所有联系人 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆6 . 保存到文件 |"<<endl;
cout<<" | |"<<endl;
cout<<" | ☆0 . 退出系统 |"<<endl;
cout<<" ****************************************************************"<<endl;
int m = 0;
do
{
cout << "请输入选项0-8\n";
cin >> m;
} while( m < 0 || m > 8 );
return m;
}
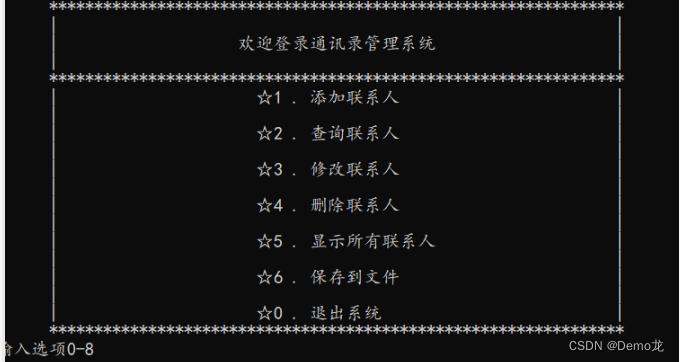
|