一、哈希概念
顺序结构以及平衡树中,元素关键码与其存储位置之间没有对应的关系,因此在查找一个元素时,必须要经过关键码的多次比较。顺序查找时间复杂度为O(N),平衡树中为树的高度,即O(logN),搜索的效率取决于搜索过程中元素的比较次数。
理想的搜索方法:可以不经过任何比较,一次直接从表中得到要搜索的元素。 如果构造一种存储结构,通过某种函数(hashFunc)使元素的存储位置与它的关键码之间能够建立一一映射的关系,那么在查找时通过该函数可以很快找到该元素。
向该结构中插入元素(根据待插入元素的关键码,以此来计算出该元素的存储位置并按此位置进行存放)搜索元素(对元素的关键码进行同样的计算,把求得的函数值当做元素的存储位置,在结构中按此位置取元素比较,若关键码相等,则搜索成功)该方式即为 哈希(散列) 方法。
哈希方法中使用的转换函数称为哈希(散列)函数,构造出来的结构叫做哈希表(Hash Table)或称为散列表
例如有一个数组arr[] = { 1,7,6,4,5,9 }; 将哈希函数设置为:hash(key)= key % capacity; 其中capacity为存储元素底层空间的总大小 在这里我们设置capacity为10
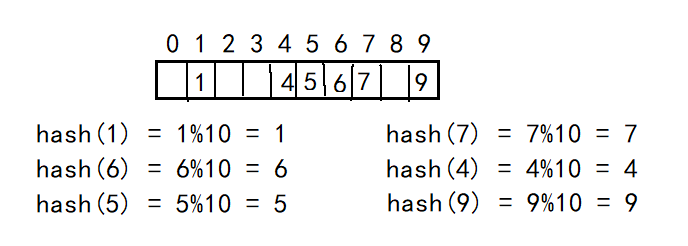
如果在插入一个元素44会出现什么问题?—— hash(44) = 44 % 10 = 4这样子计算出相同的地址了,就出现问题了——哈希冲突
二、哈希冲突
对于两个数据元素的关键字和 (Ki != Kj),有 Ki != Kj,但有:Hash(Ki) == Hash(Kj),即:不同关键字通过相同哈希数计算出相同的哈希地址,该种现象称为哈希冲突或哈希碰撞。 把具有不同关键码而具有相同哈希地址的数据元素称为“同义词”。
发生了哈希冲突如何解决? 解决哈希冲突的两种常见方法是:闭散列和开散列
三、哈希冲突解决
3.1 闭散列
闭散列:也叫开放定址法,当发生哈希冲突时,如果哈希表未被装满,说明在哈希表中必然还有空位置,那么可以把key存放到冲突位置中的“下一个” 空位置中去。那如何寻找下一个空位置呢?
3.1.1 线性探测
线性探测:从发生冲突的位置开始,依次向后探测,直到寻找到下一个空位置为止。
enum Status
{
EXIST,
EMPTY,
DELETE
};
template <class K, class V>
struct HashDate
{
pair<K, V> _kv;
Status _status;
};
template<class K, class V>
class HashTable
{
public:
bool Insert(const pair<K, V>& kv)
{
size_t start = kv.first % _tables.size();
size_t i = 0;
size_t index = start + i;
while (_tables[index]._status == EXIST)
{
i++;
index = start + i;
index %= _table.size();
}
_talbes[index]._kv = kv;
_talbes[index]._status = EXIST;
++_n;
}
private:
vector<HashDate<K, V>> _tables;
size_t _n = 0;
};
但是这样子也有一定程度的缺陷: 
线性探测优点:实现非常简单, 线性探测缺点:一旦发生哈希冲突,所有的冲突连在一起,容易产生数据“堆积”,即:不同关键码占据了可利用的空位置,使得寻找某关键码的位置需要许多次比较,导致搜索效率降低。如何缓解呢?
3.1.2 二次探测
在线性探测中,我们使用的方法是:start + i (i = 0,1,2,…) 改进为二次探测后,我们使用的方法是:start + i ^ 2 (i = 0,1,2,…) 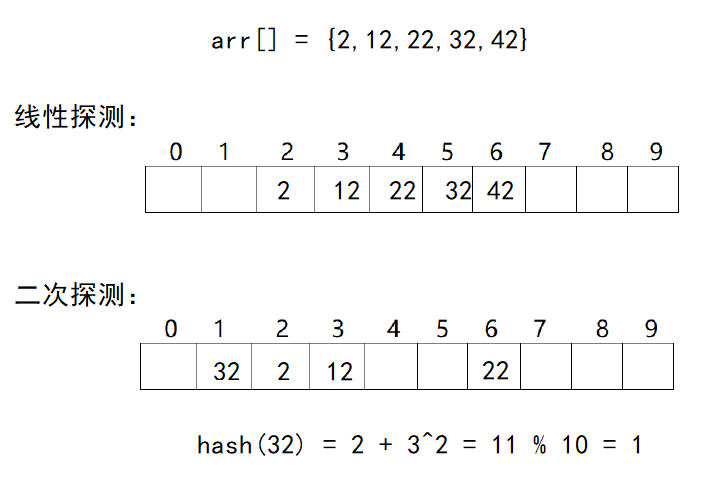
哈希表什么情况下进行扩容?如何扩容? 散列表的载荷因子定义为:α = 填入表中元素个数 / 散列表的长度 α是散列表装满程度的标志因子。由于表长是定值,α与"填入表中元素个数 "成正比,因此α越大,表明填入表中的元素越多,产生冲突的可能性就越大;α越小,表明填入表中的元素越少,产生冲突的可能性就越小。实际上散列表的平均查找长度是载荷因子α的函数,只是不同处理冲突的方法有不同的函数。
对于开放定址法,载荷因子是特别重要因素,应严格限制在0.7 - 0.8以下,超过0.8查表时的CPU缓存不命中(cache missing)按照指数曲线上升。超过后要扩容散列表
bool Insert(const pair<K, V>& kv)
{
if (_tables.size() == 0 || _n * 10 / _tables.size() >= 7)
{
size_t newSize = _tables.size() == 0 ? 10 : _tables.size() * 2;
vector<HashDate<K, V>> newTables;
newTables.resize(newSize);
for (size_t i = 0; i < _tables.size(); ++i)
{
}
_tables.swap(newTables);
}
size_t start = kv.first % _tables.size();
size_t i = 0;
size_t index = start + i;
while (_tables[index]._status == EXIST)
{
i++;
index = start + i * i;
index %= _tables.size();
}
_talbes[index]._kv = kv;
_talbes[index]._status = EXIST;
++_n;
}
上述代码十分复杂,因此进行一些改进:
template<class K, class V>
class HashTable
{
public:
bool Insert(const pair<K, V>& kv)
{
if (_tables.size() == 0 || _n * 10 / _tables.size() >= 7)
{
size_t newSize = _tables.size() == 0 ? 10 : _tables.size() * 2;
HashTable<K, V> newHT;
newHT._tables.resize(newSize);
for (size_t i = 0; i < _tables.size(); ++i)
{
if (_tables[i]._status == EXIST)
{
newHT.Insert(_tables[i]._kv);
}
}
_tables.swap(newHT._tables);
}
size_t start = kv.first % _tables.size();
size_t i = 0;
size_t index = start + i;
while (_tables[index]._status == EXIST)
{
i++;
index = start + i * i;
index %= _tables.size();
}
_tables[index]._kv = kv;
_tables[index]._status = EXIST;
++_n;
return true;
}
private:
vector<HashDate<K, V>> _tables;
size_t _n = 0;
};
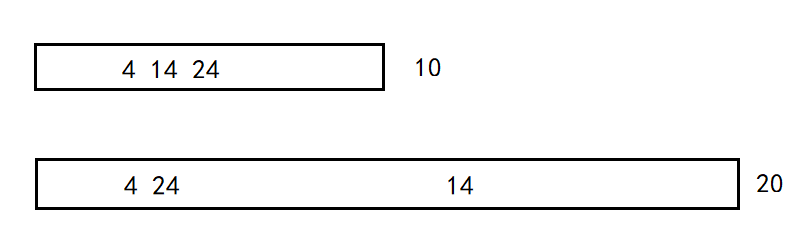 哈希表扩容的时候,我们可以直接复用insert,直接将数据拷贝下来
在以上的功能中,我们再加入find函数和erase函数,因此哈希实现如下:
#pragma once
#include <vector>
#include <iostream>
using namespace std;
enum Status
{
EXIST,
EMPTY,
DELETE
};
template <class K, class V>
struct HashData
{
pair<K, V> _kv;
Status _status = EMPTY;
};
template <class K>
struct Hash
{
size_t operator()(const K& key)
{
return key;
}
};
struct HashStr
{
size_t operator()(const string& s)
{
size_t value = 0;
for (auto ch : s)
{
value *= 31;
value += ch;
}
return value;
}
};
template<class K, class V, class HashFunc = Hash<K>>
class HashTable
{
public:
bool Erase(const K& key)
{
HashData<K, V>* ret = Find(key);
if (ret == nullptr)
{
return false;
}
else
{
--_n;
ret->_status = DELETE;
return true;
}
}
HashData<K, V>* Find(const K& key)
{
if (_tables.size() == 0)
{
return nullptr;
}
HashFunc hf;
size_t start = hf(key) % _tables.size();
size_t i = 0;
size_t index = start;
while (_tables[index]._status != EMPTY)
{
if (_tables[index]._kv.first == key && _tables[index]._status == EXIST)
{
return &_tables[index];
}
i++;
index = start + i;
index %= _tables.size();
}
return nullptr;
}
bool Insert(const pair<K, V>& kv)
{
HashData<K, V>* ret = Find(kv.first);
if (ret != nullptr)
{
return false;
}
if (_tables.size() == 0 || _n * 10 / _tables.size() >= 7)
{
size_t newSize = _tables.size() == 0 ? 10 : _tables.size() * 2;
HashTable<K, V, HashFunc> newHT;
newHT._tables.resize(newSize);
for (size_t i = 0; i < _tables.size(); ++i)
{
if (_tables[i]._status == EXIST)
{
newHT.Insert(_tables[i]._kv);
}
}
_tables.swap(newHT._tables);
}
HashFunc hf;
size_t start = hf(kv.first) % _tables.size();
size_t i = 0;
size_t index = start;
while (_tables[index]._status == EXIST)
{
i++;
index = start + i;
index %= _tables.size();
}
_tables[index]._kv = kv;
_tables[index]._status = EXIST;
++_n;
return true;
}
private:
vector<HashData<K, V>> _tables;
size_t _n = 0;
};
void TestHashTable()
{
HashTable<int, int, Hash<int>> ht;
int a[] = { 2, 12, 22, 32, 42, 52, 62 };
for (auto e : a)
{
ht.Insert(make_pair(e, e));
}
ht.Insert(make_pair(72, 72));
ht.Insert(make_pair(32, 32));
cout << ht.Find(12) << endl;
ht.Erase(12);
cout << ht.Find(12) << endl;
}
void TestHashTable2()
{
HashStr hs;
cout << hs("sort") << endl;
cout << hs("srot") << endl;
cout << hs("insert") << endl;
HashTable<string, string, HashStr> ht;
ht.Insert(make_pair("string", "字符串"));
}
闭散列:(开放定址法,在开放的空间中找)问题是,不同位置冲突数据会相互影响,相邻的位置冲突会争抢位置,互相影响效率,所以我们进行了一种优化思路——开散列/拉链法/哈希桶
3.2 开散列
开散列法又叫链地址法(拉链法、哈希桶),首先对关键码集合用散列函数计算散列地址,具有相同地址的关键码归于同一子集合,每一个子集合称为一个桶,各个桶中的元素通过一个单链表链接起来,各链表的头结点存储在哈希表中。 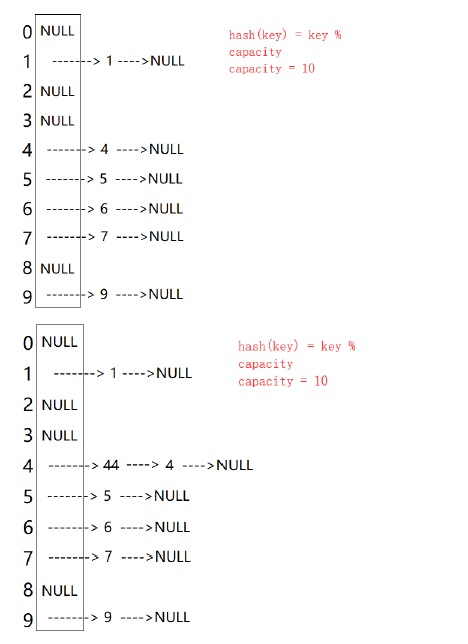
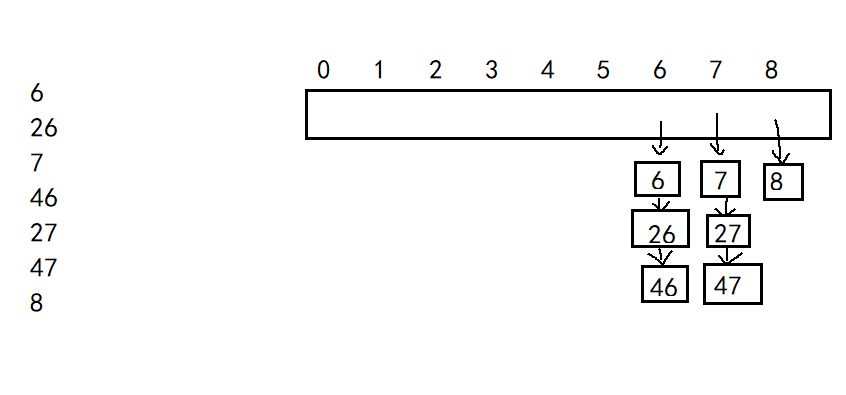
相邻位置冲突,不会争抢位置,不会互相影响
插入元素分析: 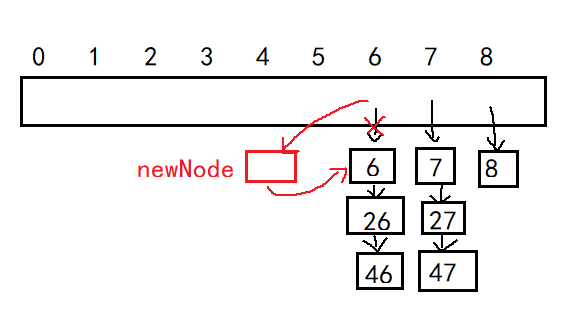
极端场景分析:
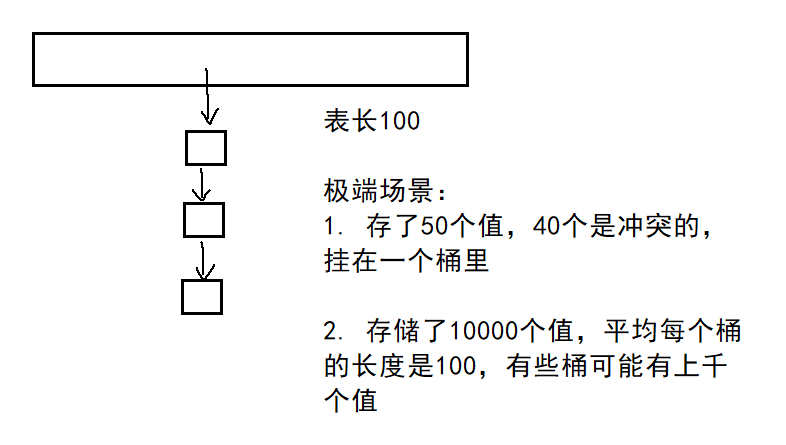 我们采用控制负载因子处理这种问题:负载因子到了就需要扩容,扩容后需要重新映射
极端场景防止一个桶位置发生冲突太多,链表太长:当一个桶长度超过一定值以后,转化为红黑树
在这里,如果涉及到哈希表的扩容,我们不能像上述那样的方法直接复用insert,因为此时这里是链表,涉及到释放旧空间等等,所以不如直接把链表连接至新表。
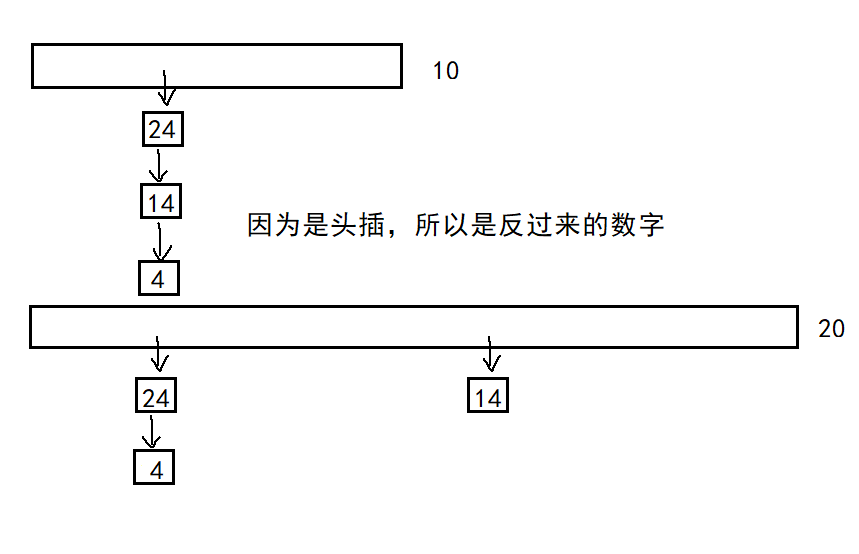 代码实现如下:
namespace LinkHash
{
template <class K, class V>
struct HashNode
{
pair<K, V> _kv;
HashNode<K, V>* _next;
HashNode(const pair<K, V>& kv)
:_kv(kv)
,_next(nullptr)
{}
};
template <class K>
struct Hash
{
size_t operator()(const K& key)
{
return key;
}
};
struct HashStr
{
size_t operator()(const string& s)
{
size_t value = 0;
for (auto ch : s)
{
value *= 31;
value += ch;
}
return value;
}
};
template <class K, class V, class HashFunc = Hash<K>>
class HashTable
{
typedef HashNode<K, V> Node;
public:
bool Erase(const K& key)
{
if (_tables.empty())
{
return false;
}
HashFunc hf;
size_t index = hf(key) % _tables.size();
Node* cur = _tables[index];
Node* prev = nullptr;
while (cur)
{
if (cur->_kv.first == key)
{
if (prev == nullptr)
{
_tables[index] = cur->_next;
}
else
{
prev->_next = cur->_next;
}
--_n;
delete cur;
return true;
}
else
{
prev = cur;
cur = cur->_next;
}
}
return false;
}
Node* Find(const K& key)
{
if (_tables.empty())
{
return nullptr;
}
HashFunc hf;
size_t index = hf(key) % _tables.size();
Node* cur = _tables[index];
while (cur)
{
if (cur->_kv.first == key)
{
return cur;
}
else
{
cur = cur->_next;
}
}
return nullptr;
}
bool Insert(const pair<K, V>& kv)
{
HashFunc hf;
Node* ret = Find(kv.first);
if (ret)
{
return false;
}
if (_n == _tables.size())
{
size_t newSize = _tables.size() == 0 ? 10 : 2 * _tables.size();
vector<Node*> newTables;
newTables.resize(newSize);
for (size_t i = 0; i < _tables.size(); ++i)
{
if (_tables[i])
{
Node* cur = _tables[i];
while (cur)
{
Node* next = cur->_next;
size_t index = hf(cur->_kv.first) % newTables.size();
cur->_next = newTables[index];
newTables[i] = cur;
cur = next;
}
}
_tables[i] = nullptr;
}
_tables.swap(newTables);
}
size_t index = hf(kv.first) % _tables.size();
Node* newnode = new Node(kv);
newnode->_next = _tables[index];
_tables[index] = newnode;
++_n;
return true;
}
private:
vector<Node*> _tables;
size_t _n = 0;
};
void TestHashTable()
{
int a[] = { 4, 24, 14, 7, 37, 27, 57, 67, 34, 14, 54 };
HashTable<int, int> ht;
for (auto e : a)
{
ht.Insert(make_pair(e, e));
}
}
}
|