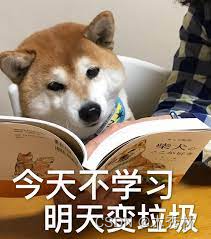
题目地址:
https://leetcode.cn/problems/route-between-nodes-lcci/
节点间通路。给定有向图,设计一个算法,找出两个节点之间是否存在一条路径。
示例1:
输入:n = 3, graph = [[0, 1], [0, 2], [1, 2], [1, 2]], start = 0, target = 2 输出:true 示例2:
输入:n = 5, graph = [[0, 1], [0, 2], [0, 4], [0, 4], [0, 1], [1, 3], [1, 4], [1, 3], [2, 3], [3, 4]], start = 0, target = 4 输出 true 提示:
节点数量n在[0, 1e5]范围内。 节点编号大于等于 0 小于 n。 图中可能存在自环和平行边。
来源:力扣(LeetCode) 链接:https://leetcode.cn/problems/route-between-nodes-lcci 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
第一种 转领接表+BFS 方式
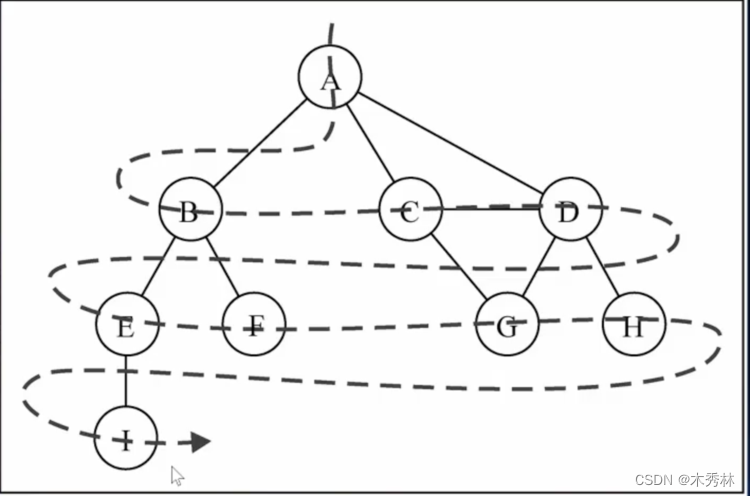
public static boolean findWhetherExistsPath(int n, int[][] graph, int start, int target) {
List<Integer>[] adj = new ArrayList[n];
for (int[] edge : graph) {
int from = edge[0];
int to = edge[1];
if (adj[from] == null) {
adj[from] = new ArrayList<>();
}
adj[from].add(to);
}
Queue<Integer> que = new LinkedList<>();
boolean[] visited = new boolean[n];
que.add(start);
visited[start] = true;
while (!que.isEmpty()) {
int node = que.poll();
if (adj[node] == null) {
continue;
}
for (Integer nex : adj[node]) {
if (nex == target) {
return true;
}
if (visited[nex]) {
continue;
}
que.add(nex);
visited[nex] = true;
}
}
return false;
}
第二种 DFS 遍历 + 递归
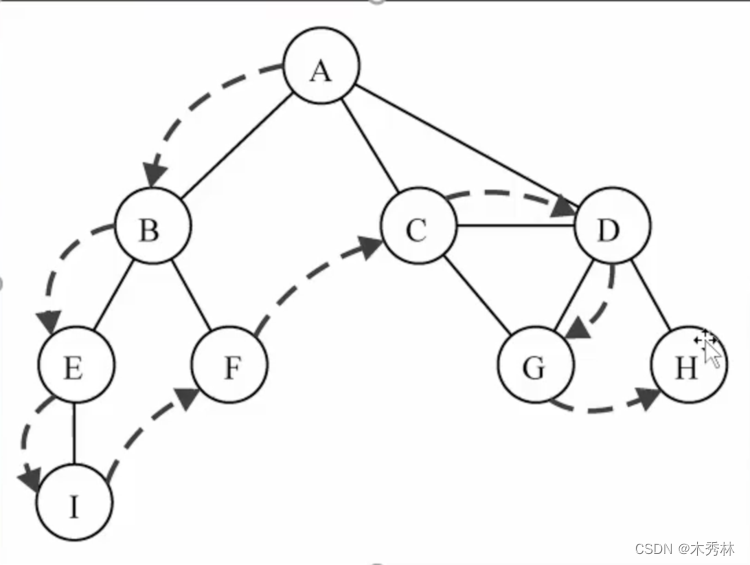
public static boolean findWhetherExistsPath(int n, int[][] graph, int start, int target) {
boolean[] visited = new boolean[graph.length];
return dfs(visited, graph, start, target);
}
private static boolean dfs(boolean[] visited, int[][] graph, int start, int target) {
for (int i = 0; i < graph.length; ++i) {
if (visited[i]) {
continue;
}
if (graph[i][0] == start && graph[i][1] == target) {
return true;
}
visited[i] = true;
if (graph[i][1] == target && dfs(visited, graph, start, graph[i][0])) {
return true;
}
visited[i] = false;
}
return false;
}
有兴趣 我们一起每日一题,有疑问也欢迎评论区交流
|