数据结构-单链表
前段时间跟着尚硅谷的视频学习,自己截图做了一些学习笔记,现在简单整理出来供大家参考,同时也方便自己日后复习查询,如果有存在疏漏,欢迎大家批评指正。文末将会附上视频链接。
链表的特点
- 链表是以节点的方式来存储的,链式存储
- 每一个节点包含data域,next域:指向下一地址
- 各个节点之间不一定是连续存储
- 分为带头节点的链表和没有头节点的链表,按实际需求来定
单链表逻辑结构
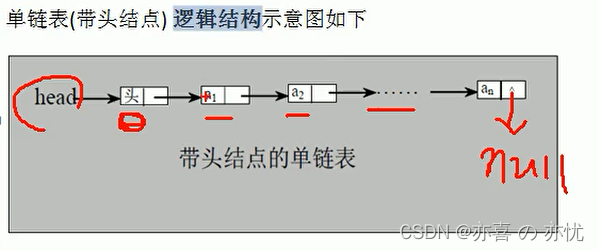
添加-单链表实现
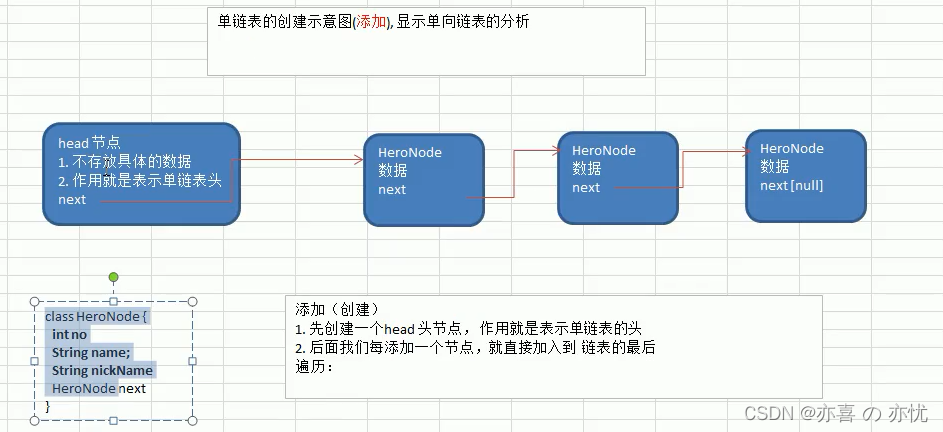
package com.main.linkedlist;
public class SingleLinkedListDemo {
public static void main(String[] args) {
HeroNode hero1=new HeroNode(1,"宋江","及时雨");
HeroNode hero2=new HeroNode(2,"卢俊义","玉麒麟");
HeroNode hero3=new HeroNode(3,"吴用","智多星");
HeroNode hero4=new HeroNode(4,"林冲","豹子头");
SingleLinkedList singleLinkedList=new SingleLinkedList();
singleLinkedList.add(hero1);
singleLinkedList.add(hero2);
singleLinkedList.add(hero3);
singleLinkedList.add(hero4);
singleLinkedList.list();
}
}
class SingleLinkedList{
private HeroNode head = new HeroNode(0,"","");
public void add(HeroNode heroNode){
HeroNode temp=head;
while (true){
if (temp.next==null){
break;
}
temp=temp.next;
}
temp.next=heroNode;
}
public void list(){
if(head.next==null){
System.out.println("链表为空");
return;
}
HeroNode temp=head.next;
while (true){
if (temp==null){
break;
}
System.out.println(temp);
temp=temp.next;
}
}
}
class HeroNode{
public int no;
public String name;
public String nickname;
public HeroNode next;
public HeroNode(int no,String name,String nickname){
this.no=no;
this.name=name;
this.nickname=nickname;
}
@Override
public String toString() {
return "HeroNode{" +
"no=" + no +
", name='" + name + '\'' +
", nickname='" + nickname +
'}';
}
}
代码优化,按照编号顺序添加节点
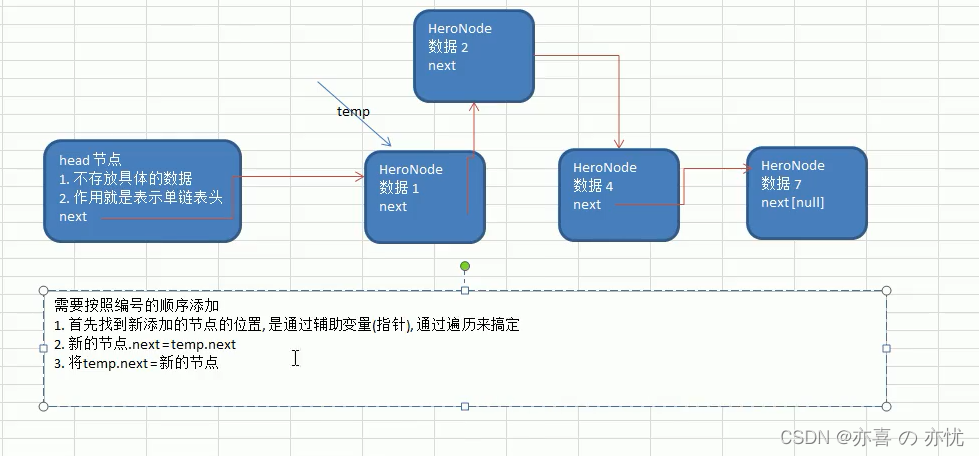
public void addByOrder(HeroNode heroNode){
HeroNode temp=head;
boolean flag=false;
while (true){
if (temp.next==null){
break;
}
if (temp.next.no>heroNode.no){
break;
} else if (temp.next.no==heroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag){
System.out.printf("准备插入的英雄编号%d已经存在,不能添加",heroNode.no);
}else {
heroNode.next=temp.next;
temp.next=heroNode;
}
}
修改-单链表实现
-
单链表代码实现
-
先找到该节点,通过遍历链表 -
创建一个临时节点存储修改后的值 -
将临时节点的值,赋给找到的待修改的节点
public void update(HeroNode newHeroNode){
if (head.next==null){
System.out.println("链表为空");
return;
}
HeroNode temp = head.next;
boolean flag=false;
while (true){
if (temp==null){
break;
}
if (temp.no == newHeroNode.no){
flag=true;
break;
}
temp=temp.next;
}
if (flag){
temp.name=newHeroNode.name;
temp.nickname=newHeroNode.nickname;
}else {
System.out.printf("没有找到英雄编号%d的节点,不能修改",newHeroNode.no);
}
}
删除-单链表实现
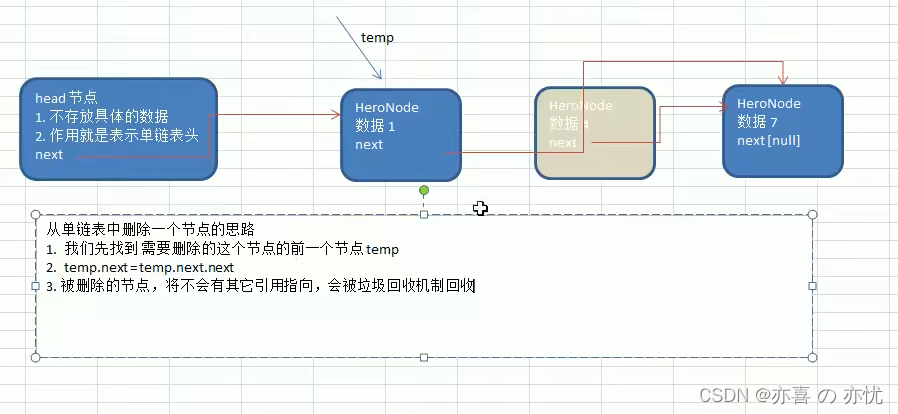
public void del(int no){
HeroNode temp=head;
boolean flag=false;
while (true){
if (temp.next==null){
break;
}
if (temp.next.no==no){
flag=true;
break;
}
temp=temp.next;
}
if(flag){
temp.next=temp.next.next;
}else {
System.out.printf("要删除的%d节点不存在\n",no);
}
}
```
#### 获取单链表的节点个数
* 代码实现
```java
public int getLength(HeroNode head){
if (head.next==null){
return 0;
}
int length=0;
HeroNode cur=head.next;
while (cur!=null){
length++;
cur=cur.next;
}
return length;
}
查找单链表中的倒数第K个节点
-
编写一个方法,接收head节点,同时接收一个index -
index表示倒数第index个节点 -
先把链表从头到尾遍历,得到链表的总长getLength -
得到size后,我们从链表的第一个开始遍历(size-index)个, -
找到返回该节点,否则返回空 -
代码实现
public HeroNode findLastIndexNode(HeroNode head, int index){
if (head.next==null){
return null;
}
int size=getLength(head);
if (index<=0||index>size){
return null;
}
HeroNode cur=head.next;
for (int i=0;i<size-index;i++){
cur=cur.next;
}
return cur;
}
单链表的反转
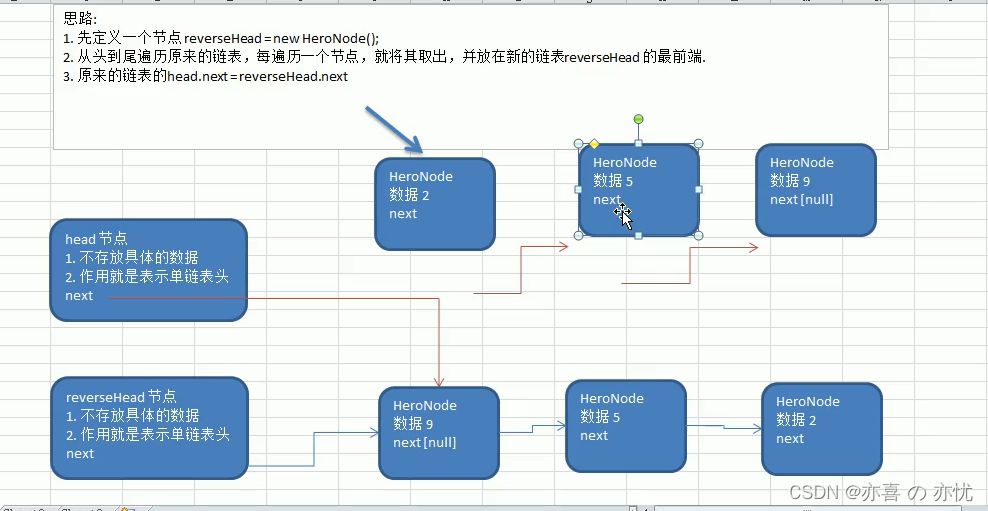
在此附上尚硅谷的教学视频: 【尚硅谷】数据结构与算法(Java数据结构与算法)
|