LC 206 反转链表 给你单链表的头节点 head ,请你反转链表,并返回反转后的链表 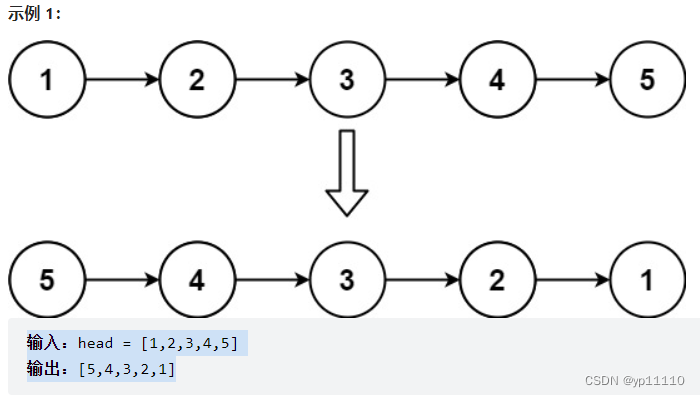 这道题题的难点在于 如何移动指针的同时,将当前元素的next指向前驱元素;下面先介绍双指针写法
public ListNode reverseList(ListNode head) {
ListNode pre =null;
ListNode cur = head;
while(cur!=null){
ListNode temp =cur.next;
cur.next=pre;
pre= cur;
cur =temp;
}
return pre;
}
当要移动某个指针时候,先考虑当前指针指向的位置在之后的遍历中还需不需要用到,如果需要,直接拿个变量记住它就行了 在双指针的移动过程中,这里先移动pre,如果先移动cur到cur.next的话也可以,只不过要用个临时变量先把cur当前位置记录下来。
递归写法:
public ListNode reverseList(ListNode head) {
return reverse(head,null);
}
public static ListNode reverse(ListNode cur,ListNode pre){
if(cur==null) return pre;
ListNode temp = cur.next;
cur.next = pre;
return reverse(temp,cur);
}
递归我是这样理解的:一个reverse(cur,pre);相当于将当前cur的next指向pre,在其方法内部,有个临时变量temp记录了cur.next ,所以在回调reverse()函数时候,参数填充情况就变成了reverse(temp,cur);
LC.707 实现包括获取指定位置的元素,按下标插入新增节点,头插法增加节点,尾插法增加节点,以及删除指定位置的节点 方法在内的单链表
没什么好说的,需要注意的地方就是,将链表头节点定义为虚拟头节点,这个节点中的元素并不算入链表中
class ListNode {
int val;
ListNode next;
ListNode(){}
ListNode(int val) {
this.val=val;
}
}
class MyLinkedList {
int size;
ListNode head;
public MyLinkedList() {
size = 0;
head = new ListNode();
}
public int get(int index) {
if (index < 0 || index >= size) {
return -1;
}
ListNode currentNode = head;
for (int i = 0; i <= index; i++) {
currentNode = currentNode.next;
}
return currentNode.val;
}
public void addAtHead(int val) {
addAtIndex(0, val);
}
public void addAtTail(int val) {
addAtIndex(size, val);
}
public void addAtIndex(int index, int val) {
if (index > size) {
return;
}
if (index < 0) {
index = 0;
}
size++;
ListNode pred = head;
for (int i = 0; i < index; i++) {
pred = pred.next;
}
ListNode toAdd = new ListNode(val);
toAdd.next = pred.next;
pred.next = toAdd;
}
public void deleteAtIndex(int index) {
if (index < 0 || index >= size) {
return;
}
size--;
if (index == 0) {
head = head.next;
return;
}
ListNode pred = head;
for (int i = 0; i < index ; i++) {
pred = pred.next;
}
pred.next = pred.next.next;
}
}
LC.203 移除链表中的元素 给你一个链表的头节点 head 和一个整数 val ,请你删除链表中所有满足 Node.val == val 的节点,并返回 新的头节点 。
示例 1: 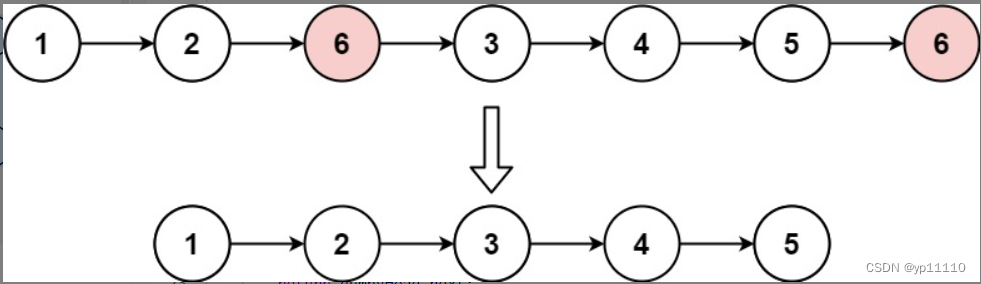
输入:head = [1,2,6,3,4,5,6], val = 6 输出:[1,2,3,4,5]
定义虚拟头节点:那么无论是哪个位置的元素都可以正常移除,不定义虚拟头节点,直接将cur初始化为head,就需要考虑要删除的元素在0位置的情况,特殊处理。 定义虚拟头节点
class Solution {
public ListNode removeElements(ListNode head, int val) {
ListNode dumpyHead = new ListNode();
dumpyHead.next = head;
ListNode cur=dumpyHead;
while(cur!=null&&cur.next!=null){
if(cur.next.val==val){
cur.next=cur.next.next;
}else{
cur = cur.next;
}
}
return dumpyHead.next;
}
}
不定义虚拟头节点
class Solution {
public ListNode removeElements(ListNode head, int val) {
while(head!=null&&head.val==val){
head=head.next;
}
ListNode cur=head;
while(cur!=null&&cur.next!=null){
if(cur.next.val==val){
cur.next=cur.next.next;
}else{
cur = cur.next;
}
}
return head;
}
}
|