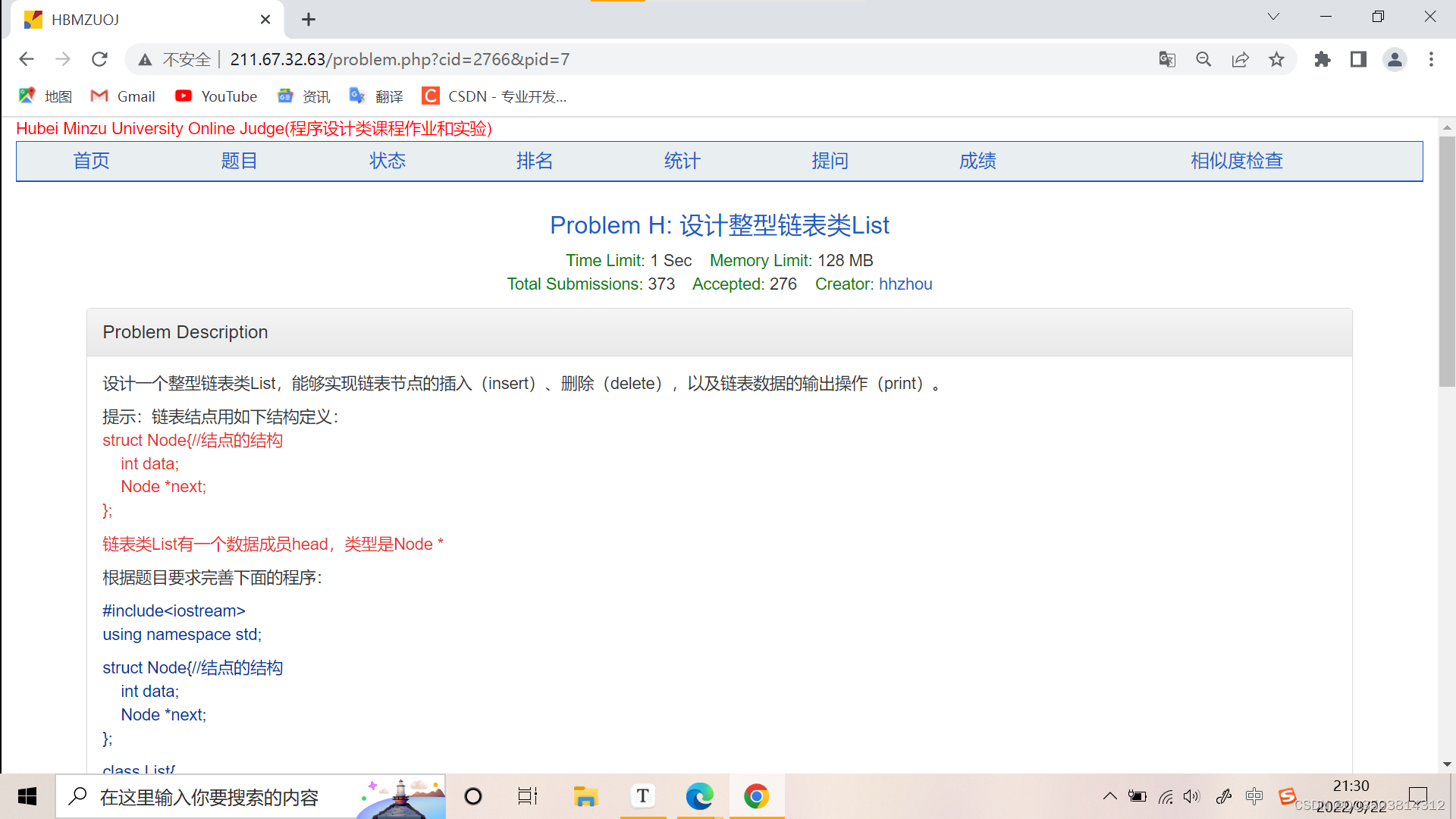
?
Problem Description
设计一个整型链表类List,能够实现链表节点的插入(insert)、删除(delete),以及链表数据的输出操作(print)。
提示:链表结点用如下结构定义: struct Node{//结点的结构 ????int data; ????Node *next; };
链表类List有一个数据成员head,类型是Node *
根据题目要求完善下面的程序:
#include<iostream> using namespace std;
struct Node{//结点的结构 ????int data; ????Node *next; };
class List{ private: ????Node* head; public:
//你的代码将被嵌在这里
int main() { ????List list;//定义一个空链表list ????list.Listinsert(0,10);//在第0个结点的后面插入值为10的新结点,也即在链表头部插入新的结点 ????list.Listinsert(0,66); ????list.Listinsert(1,292);//在第1个结点的后面插入值为10的新结点 ????list.Listdelete(66);//删除链表中第一个值为66的结点 ????list.Listinsert(2,-2);//在第2个结点的后面插入值为-2的新结点 ????list.Listinsert(1,3);//在第1个结点的后面插入值为3的新结点 ????list.Listprint();//从头到尾输出链表结点的值,每个输出值占一行 ????return 0; }
Sample Output
292
3
10
-2
?
#include <iostream>
#include <string>
using namespace std;
class Salary {
private:
double Wage, Subsidy, Rent, WaterFee, ElecFee;//基本工资Wage,岗位工资Subsidy,房租Rent,水费WaterFee,电费ElecFee
public:
Salary(double i1, double i2 = 0, double i3 = 0, double i4 = 0, double i5 = 0) {//初始化工资数据的各分项
Wage = i1;
Subsidy = i2;
Rent = i3;
WaterFee = i4;
ElecFee = i5;
}
Salary() {//初始化工资的各分项数据为0
Wage = Subsidy = Rent = WaterFee = ElecFee = 0;
}
void setWage(double f) { Wage = f; }
double getWage() { return Wage; }
void setSubsidy(double f) { Subsidy = f; }
double getSubsidy() { return Subsidy; }
void setRent(double f) { Rent = f; }
double getRent() { return Rent; }
void setWaterFee(double f) { WaterFee = f; }
double getWaterFee() { return WaterFee; }
void setElecFee(double f) { ElecFee = f; }
double getElecFee() { return ElecFee; }
double RealSalary() {//计算实发工资,实发工资=Wage+Subsidy-Rent-WaterFee-ElecFee
return Wage + Subsidy - Rent - WaterFee - ElecFee;
}
};
//你的代码将被嵌在这里
int m_Num = 0;
class Worker : public Salary
{
public:
Worker(string name, int age, string dept)
{
m_name = name;
m_age = age;
m_Dept = dept;
m_Num++;
}
Worker()
{
m_Num++;
}
int getNum()
{
return m_Num;
}
void setName(string name)
{
m_name = name;
}
string getName()
{
return m_name;
}
string m_name;
int m_age;
string m_Dept;
Salary m_salary;
};
int main() {
Worker w1("John", 30, "design");
Worker w2;
cout << "the total num is: " << w1.getNum() << endl;
w2.setName("Linda");
cout << "in w2 the name is: " << w2.getName() << endl;
return 0;
}
|