Java数组03 拓展
冒泡排序
package com.Ym.array;
?
import java.util.Arrays;
?
public class ArrayDemo07 {
? ?public static void main(String[] args) {
? ? ? ?int[] a = {1,3,456,452,13,125,102,745,23,124,23,8};
? ? ? ?int[] sort = sort(a);
? ? ? ?//调用完我们自己写的排序方法以后,返回一个排序后的数组
? ? ? ?System.out.println(Arrays.toString(sort));
? }
? ?
? ?//冒泡排序
? ?//1. 比较数组中,两个相邻的元素,如果第一个数比第二个数大,我们就交换他们的位置。
? ?//2. 每一次比较,都会产生出一个最大,或者最小的数字。
? ?//3. 下一轮则可以少一次排序
? ?//4. 依次循环,知道结束
? ?
? ?public static int[] sort(int[] array){
? ? ? ?//临时变量
? ? ? ?int temp = 0;
? ? ? ?boolean flag = false;//优化
? ? ? ?//外层循环,判断我们这个要走多少次
? ? ? ?for (int i = 0; i < array.length-1; i++) {
? ? ? ? ? ?//内层循环,比价判断俩个数,如果第一个数比第二个数大,则交换位置
? ? ? ? ? ?//j = length-1-i的意思是他每进行一次外部循环时就可以确定一个数,内部循环就相应的减少一次
? ? ? ? ? ?for (int j = 0; j < array.length-1-i ; j++) {
? ? ? ? ? ? ? ?if (array[j+1] < array[j]){
? ? ? ? ? ? ? ? ? ?temp = array[j];
? ? ? ? ? ? ? ? ? ?array[j] = array[j+1];
? ? ? ? ? ? ? ? ? ?array[j+1] = temp;
? ? ? ? ? ? ? ? ? ?flag = true//优化
? ? ? ? ? ? ? }
? ? ? ? ? } ? ? ? ?
? ? ? ? ? ?if(flag == false){
? ? ? ? ? ? ? ?break;
? ? ? ? ? }//优化
? ? ? }
? ? ? ?return array;
? }
}
稀疏数组
-
记录数组一共有几行几列,有多少个不同值 -
把具有不同值的元素和行列及值记录在一个小规模的数组中,从而缩小程序的规模
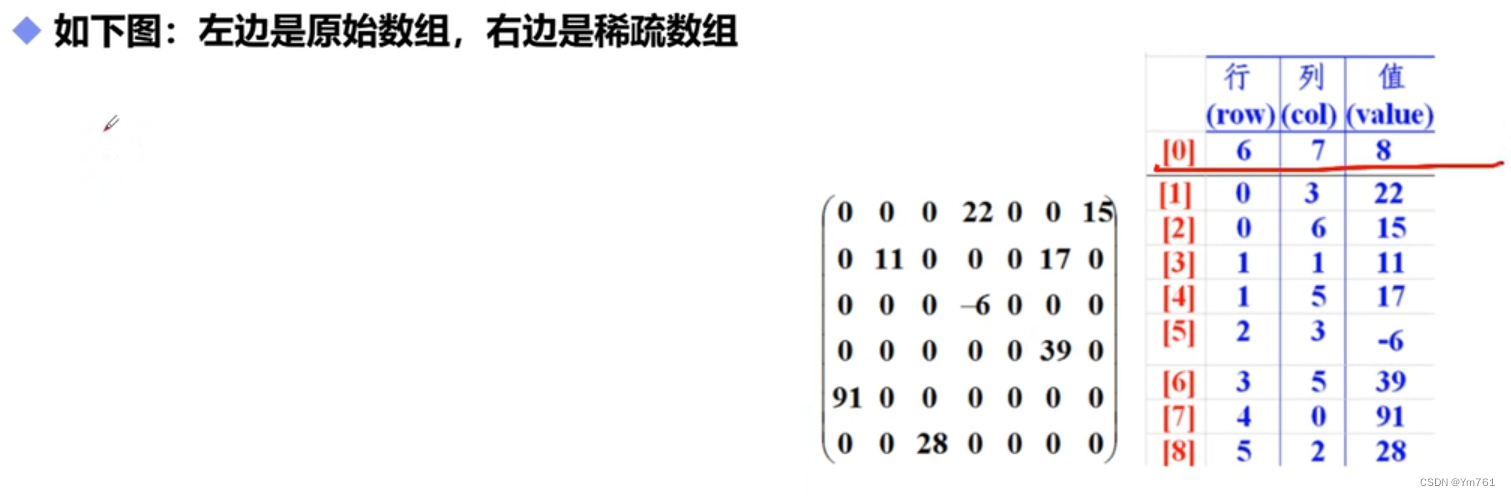
?
增强For循环的注:
-
增强For循环与普通For循环的区别:
-
增强For循环的特点:书写简介,但只能获取集合元素,不能对集合进行操作 -
书写格式:for(数据类型变量名:被遍历的集合或者数组{执行语句}) -
增强for循环使用示例
public class ArrayTestDemo05 {
? ?public static void main(String[] args) {
? ? ? ?int[][] array1 = new int[11][11];
? ? ? ?array1[1][2] = 1;
? ? ? ?array1[2][3] = 2;
?
? ? ? ?System.out.println("输出最原始的数组:");
? ? ? ?//增强for循环输出二维数组
? ? ? ?for (int[] ints : array1) {
? ? ? ? ? ?for (int anInt : ints) {
? ? ? ? ? ? ? ?System.out.print(anInt + "\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
?
? ? ? ?System.out.println("=======================");
? ? ? ?//普通for循环输出二维数组
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array1[i][j] + "\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
稀疏数组与还原稀疏数组
//转换用稀疏数组
? ? ? ?//1. 获取有效值的个数
? ? ? ?int value = 0;
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?if (array1[i][j] != 0){
? ? ? ? ? ? ? ? ? ?value++;
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
? ? ? ?System.out.println("有效值的个数:" + value);
?
? ? ? ?//2. 创建一个稀疏数组的数组
? ? ? ?//错误形式int[][] array2 = new int[11][11];
? ? ? ?int[][] array2 = new int[value + 1][3];
? ? ? ?array2[0][0] = 11;
? ? ? ?array2[0][1] = 11;
? ? ? ?array2[0][2] = value;//有效值的个数
?
? ? ? ?//3. 遍历二维数组,将非零的值,存放在稀疏数组之中
? ? ? ?int count = 0;
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?if (array1[i][j] != 0){
? ? ? ? ? ? ? ? ? ?count++;
? ? ? ? ? ? ? ? ? ?array2[count][0] = i;
? ? ? ? ? ? ? ? ? ?array2[count][1] = j;
? ? ? ? ? ? ? ? ? ?array2[count][2] = array1[i][j];
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
? ? ? ?System.out.println("稀疏数组");
? ? ? ?for (int i = 0; i < array2.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array2[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array2[i][j] + "\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
System.out.println("===========================");
? ? ? ?System.out.println("还原稀疏数组");
? ? ? ?//1. 读取稀疏数组
? ? ? ?int[][] array3 = new int[array2[0][0]][array2[0][1]];
?
? ? ? ?//2. 给其中的元素还原它的值
? ? ? ?for (int i = 1; i < array2.length; i++) {
? ? ? ? ? ?array3[array2[i][0]][array2[i][1]] = array2[i][2];
? ? ? }
?
? ? ? ?//3. 打印
? ? ? ?System.out.println("还原的数组为:");
? ? ? ?for (int[] ints : array3) {
? ? ? ? ? ?for (int anInt : ints) {
? ? ? ? ? ? ? ?System.out.print(anInt + "\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
? }
2022.9.19 星期一
? 昨天白天满课,晚上我跑去健身了哈哈, 虽然体重没有降下来,但是感觉运动起来的强度有所提升了,感觉继续坚持下来就好,就像健身房的标题:Make time more powerful!
? 今天本来想一个早上搞定数组的学习的,但是早上实在是太困了,莫名其妙的,加上这个最后两节课实在是太难了哈哈,就学了一天,而且掌握的程度也不高,需要自己回去打打,现在继续看文献把,准备好明天的组会!
? 加油加油
|