学习内容
力扣算法:
24、两两交换链表中的节点
19、删除链表的倒数n个节点
07、链表相交
142、环形链表||
具体内容
24、两两交换链表中的节点
题目地址
解题
class Solution {
public ListNode swapPairs(ListNode head) {
ListNode listnode = new ListNode(-1,head);
ListNode pre = listnode;
while(pre.next != null && pre.next.next != null){
ListNode cur = pre.next;
ListNode cur1 = pre.next.next.next;
pre.next = pre.next.next;
pre.next.next = cur;
cur.next = cur1;
cur = cur.next.next;
}
return listnode.next;
}
}
代码中需要注意的地方
1、 while循环体的判断语句为何这样写
pre.next != null 判断链表个数为偶数, pre.next.next != null判断链表个数为奇数
2、在翻转指针时要多画图理解
cur虚拟头节点一定是指向要交换的两个节点的前一个节点 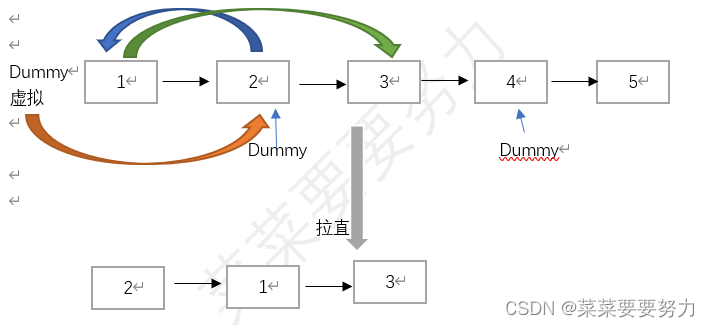
3、为什么while循环体是用&& 而不是||
结合图像判断,pre.next != null表示链表长度是单数,pre.next.next != null代表链表长度是双数,可结合上图来看
19、删除链表的倒数n个节点
题目地址
解题
快慢指针
class Solution {
public ListNode removeNthFromEnd(ListNode head, int n) {
ListNode listnode = new ListNode(-1,head);
ListNode fast = listnode;
ListNode slow = listnode;
while(n-- >= 0{
fast = fast.next;
}
while(fast != null){
fast = fast.next;
slow = slow.next;
}
slow.next = slow.next.next;
return listnode.next;
}
}
代码中需要注意的地方
1、为什么求倒数第n个节点时,快指针走n+1步之后,slow紧跟后面直到快指针等于null,慢指针指向的便是我们所求的值?
双指针的经典应用,如果要删除倒数第n个节点,让fast移动n步,然后让fast和slow同时移动,直到fast指向链表末尾。删掉slow所指向的节点就可以了。
07、链表相交
解题
题目地址
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
ListNode curA = headA;
ListNode curB = headB;
int lenA = 0;
int lenB = 0;
while(curA != null){
lenA++;
curA = curA.next;
}
while(curB != null){
lenB++;
curB = curB.next;
}
curA = headA;
curB = headB;
if(lenB > lenA){
int temp = lenA;
lenA = lenB;
lenB = temp;
ListNode list = curA;
curA = curB;
curB = list;
}
int pre = lenA - lenB;
while(pre-- > 0){
curA = curA.next;
}
while(curA != null){
if(curA == curB){
return curA;
}
curA = curA.next;
curB = curB.next;
}
return null;
}
}
解答代码存在的疑问
1、解题步骤
求两条长度不同链表相交的相同元素,由于长度不同,因此为了统一规则,可以先找出各自的链表长度,求长度差n,而较长链表在n个元素之前都不会与短链表相交,因此先让长链表遍历到n的位置,这样长短链表属于同一起点,便可一直往下走
142、环形链表||
解题
题目地址
public class Solution {
public ListNode detectCycle(ListNode head) {
ListNode fast = head;
ListNode slow = head;
while(fast != null && fast.next != null){
fast = fast.next.next;
slow = slow.next;
while (index1 != index2) {
index1 = index1.next;
index2 = index2.next;
}
return index2;
}
}
}
解答代码存在的疑问
1、当求出了快慢指针的相遇位置时,为什么还要再用while循环求?
求出了快慢指针的相遇位置,只知道了fast在哪,还要求入口
|