题目链接 https://leetcode.cn/problems/aMhZSa 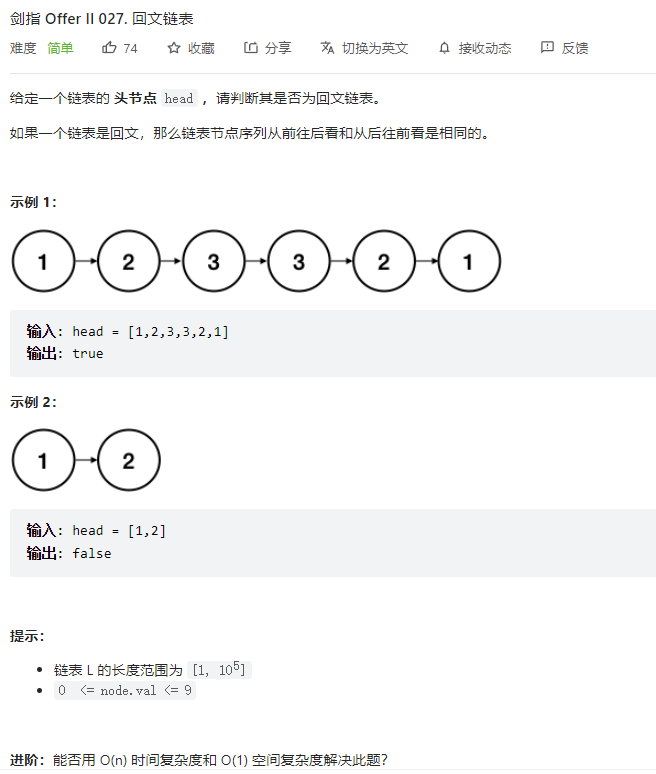
一、空间复杂度为O(n)
借助栈,先把所有的链表元素压栈,之后依次出栈和链表元素比较,因为栈是先进后出,所以出栈顺序和入栈顺序是完全相反的,当如果是回文串时,反过来比也是一样的,如果不一样,则不是回文串。
public static boolean isPalindrome1(Node head) {
Stack<Node> stack = new Stack<>();
Node cur = head;
while (cur != null) {
stack.push(cur);
cur = cur.next;
}
while (head != null) {
if (stack.pop().value != head.value) {
return false;
} else {
head = head.next;
}
}
return true;
}
二、空间复杂度O(n/2)
显然,刚刚那个方式的后半段是重复操作。 可以利用双指针法,直接从中间才开始添加元素比
public static boolean isPalindrome2(Node head) {
if (head == null || head.next == null) {
return true;
}
Node right = head.next;
Node cur = head;
while (cur.next != null && cur.next.next != null) {
right = right.next;
cur = cur.next.next;
}
Stack<Node> stack = new Stack<>();
while (right != null) {
stack.push(right);
right = right.next;
}
while (!stack.isEmpty()) {
if (stack.pop().value != head.value) {
return false;
}
head = head.next;
}
return true;
}
三、空间复杂度O(1)
这个就比较麻烦点 先把 1->2->3->4->5 变成 1->2->3<-4<-5 最后再变回 1->2->3->4->5
public static boolean isPalindrome(Node head) {
if (head == null || head.next == null) {
return true;
}
Node slow = head;
Node fast = head;
while (fast.next != null && fast.next.next != null) {
slow = slow.next;
fast = fast.next.next;
}
fast = slow.next;
slow.next = null;
Node node = null;
while (fast != null) {
node = fast.next;
fast.next = slow;
slow = fast;
fast = node;
}
node = slow;
fast = head;
boolean res = true;
while (fast != null && slow != null) {
if (fast.value != slow.value) {
res = false;
break;
}
fast = fast.next;
slow = slow.next;
}
slow = node.next;
node.next = null;
while (slow != null) {
fast = slow.next;
slow.next = node;
node = slow;
slow = fast;
}
return res;
}
|