定义于头文件 <list>
template< ?class T, ?class Allocator = std::allocator<T> > class list; ? ?(1) ? ?
namespace pmr { template <class T>
?
using list = std::list<T, std::pmr::polymorphic_allocator<T>>;} (2) ? ?(C++17 起)
std::list 是支持常数时间从容器任何位置插入和移除元素的容器。不支持快速随机访问。它通常实现为双向链表。与 std::forward_list 相比,此容器提供双向迭代但在空间上效率稍低。
在 list 内或在数个 list 间添加、移除和移动元素不会非法化迭代器或引用。迭代器仅在对应元素被删除时非法化。
std::list 满足容器 (Container) 、具分配器容器 (AllocatorAwareContainer) 、序列容器 (SequenceContainer) 及可逆容器 (ReversibleContainer) 的要求。
合并二个已排序列表
std::list<T,Allocator>::merge
void merge( list& other ); | (1) | (C++11 起) | void merge( list&& other ); | template <class Compare> void merge( list& other, Compare comp ); | (2) | (C++11 起) | template <class Compare> void merge( list&& other, Compare comp ); |
归并二个已排序链表为一个。链表应以升序排序。
不复制元素。操作后容器 other 变为空。若 other 与 *this 指代同一对象则函数不做任何事。若 get_allocator() != other.get_allocator() ,则行为未定义。没有引用和迭代器变得非法,除了被移动元素的迭代器现在指代到 *this 中,而非到 other 中,第一版本用 operator< 比较元素,第二版本用给定的比较函数 comp 。
此操作是稳定的:对于二个链表中的等价元素,来自 *this 的元素始终前驱来自 other 的元素,而且 *this 和 other 的等价元素顺序不更改。
参数
other | - | 要交换的另一容器 | comp | - | 比较函数对象(即满足比较 (Compare) 概念的对象),若第一参数小于(即先序于)第二参数则返回 ?true 。 比较函数的签名应等价于如下: ?bool cmp(const Type1 &a, const Type2 &b); 虽然签名不必有 const & ,函数也不能修改传递给它的对象,而且必须接受(可为 const 的)类型 Type1 与 Type2 的值,无关乎值类别(从而不允许 Type1 & ,亦不允许 Type1 ,除非 Type1 的移动等价于复制 (C++11 起))。 类型 Type1 与 Type2 必须使得 list<T,Allocator>::const_iterator 类型的对象能在解引用后隐式转换到这两个类型。 ? |
返回值
(无)
异常
若抛出异常,则此函数无效果(强异常保证),除非异常来自比较函数。
复杂度
至多 std::distance(begin(), end()) + std::distance(other.begin(), other.end()) - 1 次比较。
调用示例
#include <iostream>
#include <list>
std::ostream& operator<<(std::ostream& ostr, const std::list<int>& list)
{
for (auto &i : list)
{
ostr << " " << i;
}
return ostr;
}
int main()
{
std::list<int> list1 = { 5, 9, 0, 1, 3 };
std::list<int> list2 = { 8, 7, 2, 6, 4 };
list1.sort();
list2.sort();
std::cout << "list1: " << list1 << "\n";
std::cout << "list2: " << list2 << "\n";
list1.merge(list2);
std::cout << "merged: " << list1 << "\n\n";
auto func_com = [](int a, int b)
{
return a > b;
};
std::list<int> list3 = { 5, 9, 0, 1, 3 };
std::list<int> list4 = { 8, 7, 2, 6, 4 };
list3.sort();
list4.sort();
std::cout << "list3: " << list3 << "\n";
std::cout << "list4: " << list4 << "\n";
list4.merge(list3, func_com);
std::cout << "merged: " << list4 << "\n\n";
}
?输出
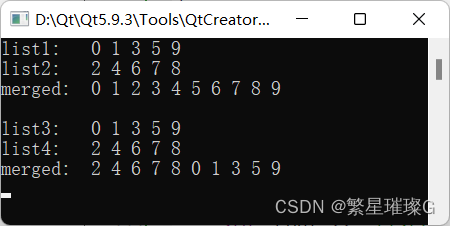
将该链表的所有元素的顺序反转
std::list<T,Allocator>::reverse
void reverse(); | (C++11 前) | void reverse() noexcept; | (C++11 起) |
逆转容器中的元素顺序。不非法化任何引用或迭代器。
参数
(无)
返回值
(无)
复杂度
与容器大小成线性
?调用示例
#include <iostream>
#include <list>
std::ostream& operator<<(std::ostream& ostr, const std::list<int>& list)
{
for (auto &i : list)
{
ostr << " " << i;
}
return ostr;
}
int main()
{
std::list<int> list = { 8, 7, 5, 9, 0, 1, 3, 2, 6, 4 };
std::cout << "before: " << list << "\n";
list.sort();
std::cout << "ascending: " << list << "\n";
list.reverse();
std::cout << "descending: " << list << "\n";
}
输出
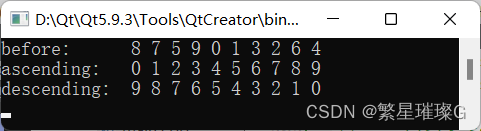
?
|