前言
问题描述:
请你实现一个链表。 操作: insert x y:将y加入链表,插入在第一个值为x的结点之前。若链表中不存在值为x的结点,则插入在链表末尾。保证x,y为int型整数。 delete x:删除链表中第一个值为x的结点。若不存在值为x的结点,则不删除。
输入描述:
第一行输入一个整数n (1≤n≤10^4),表示操作次数。 接下来的n行,每行一个字符串,表示一个操作。保证操作是题目描述中的一种。
输出描述:
输出一行,将链表中所有结点的值按顺序输出。若链表为空,输出"NULL"(不含引号)。
举例:
5
insert 0 1
insert 0 3
insert 1 2
insert 3 4
delete 4
2 1 3
解法思路:
代码结果:
#include<stdio.h>
#include<stdlib.h>
typedef struct Node
{
int data;
struct Node* next;
}Node;
Node* initList()
{
Node* list=(Node*)malloc(sizeof(Node));
list->data=0;
list->next=NULL;
return list;
}
void tailINSERT(Node* list,int x,int y)
{
Node* node = (Node*)malloc(sizeof(Node));
node->data = y;
Node* pre=list;
Node* current=list->next;
while(current)
{
if(current->data==x)
{
node->next= current;
pre->next=node;
list->data++;
return;
}
pre = current;
current = current->next;
}
node->next = NULL;
pre->next=node;
list->data++;
}
void delete(Node* list,int data)
{
Node* pre=list;
Node* current=list->next;
while(current)
{
if(current->data==data)
{
pre->next=current->next;
free(current);
list->data--;
return;
}
pre = current;
current = current->next;
}
}
void printList(Node* list)
{
list=list->next;
if(list==NULL)
{
printf("NULL");
}
while(list)
{
printf("%d ",list->data);
list=list->next;
}
}
int main()
{
Node* list= initList();
int n, x, y;
char s[10];
scanf("%d", &n);
while(n--)
{
scanf("%s", s);
if (strcmp(s, "insert") == 0)
{
scanf("%d%d", &x, &y);
tailINSERT(list, x, y);
}
else if (strcmp(s, "delete") == 0)
{
scanf("%d", &x);
delete(list, x);
}
}
printList(list);
system("pause");
}
重点难点:
本题结合文中所附的链表章节并不难,易错的在输出数据时需要带空格。
结束语
- 以上就是C数据结构栈实现链表及其重点的内容。可以在牛客尝试刷几道单链表题目来练习实践。牛客网刷题(点击可以跳转),可以尝试注册使用。
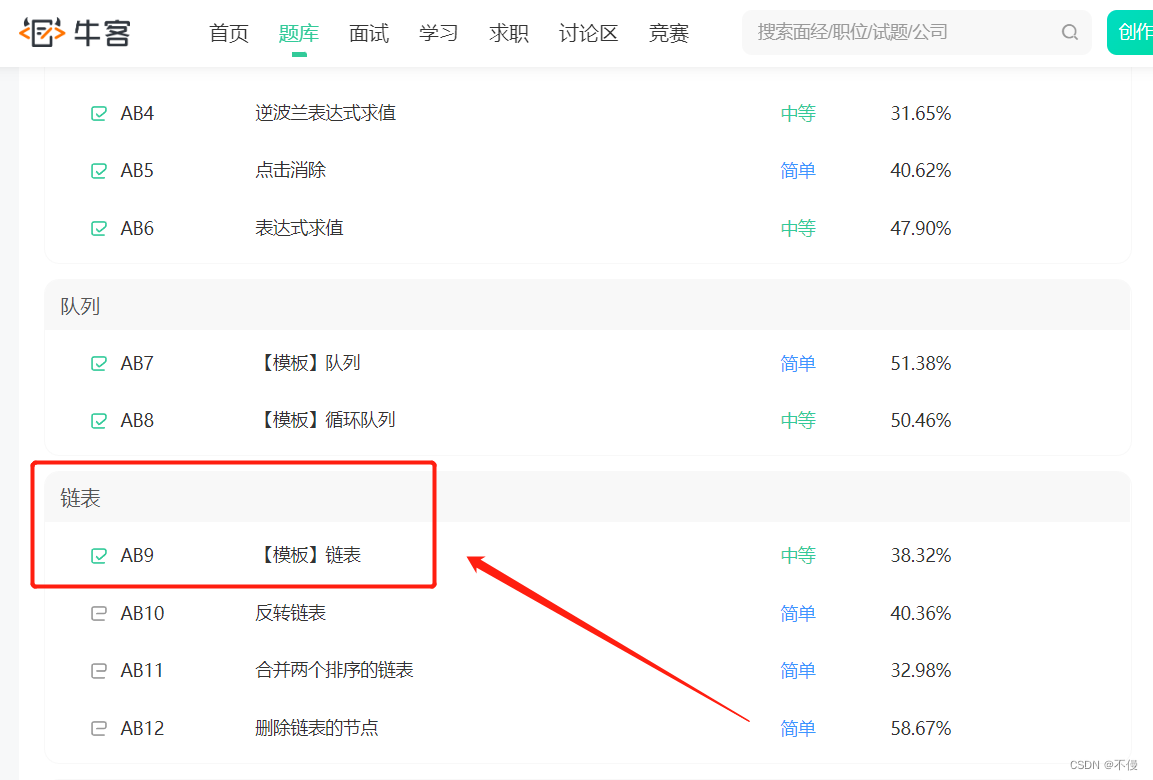
|