冒泡排序
什么是冒泡排序
冒泡排序(Bubble Sort),是计算机科学领域简单的排序算法
重复的访问每一个元素,依次相邻的两个元素进行比较大小,进行交换位置,
为什么叫冒泡排序:
- 越小的元素会经过交换位置来浮到数组的顶端,(升序或降序的排序),类似水中气泡慢慢浮现到水面
升序
@Test
public void testBubbling() {
int[] a = {1, 47, 4, 36, 8, 2, 90, 2, 3};
int aLength = a.length;
System.out.println("未排序前的数组"+Arrays.toString(a));
for (int i = 1; i < aLength; i++) {
for (int j = 0; j < aLength - i; j++) {
if (a[j] > a[j + 1]) {
int stemp = a[j];
a[j] = a[j + 1];
a[j + 1] = stemp;
}
}
}
System.out.println("排序后的结果"+Arrays.toString(a));
}
运行结果
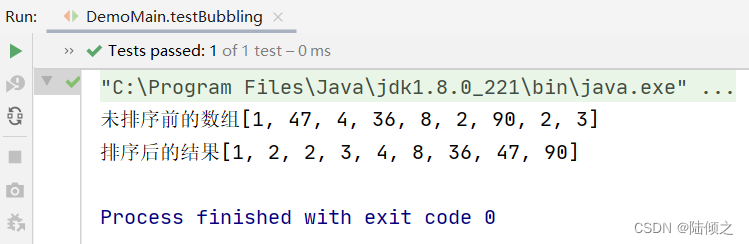
分析
- 依次对相邻两个元素做比较
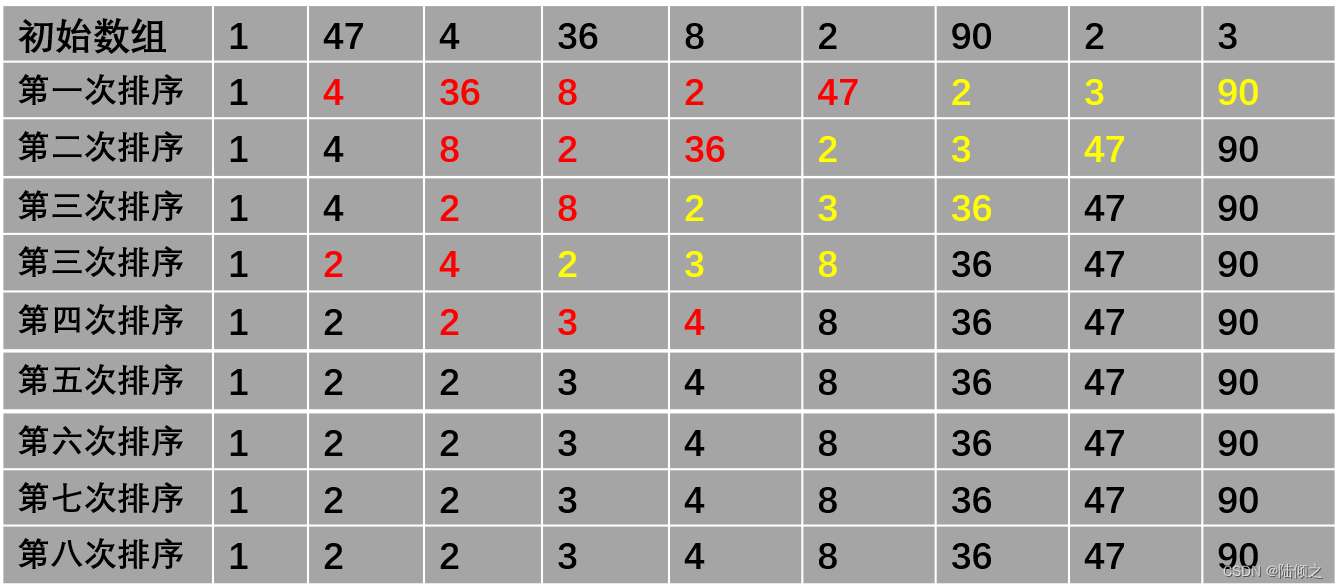
降序
@Test
public void testBubbling() {
int[] a = {1, 47, 4, 36, 8, 2, 90, 2, 3};
int aLength = a.length;
System.out.println("未排序前的数组"+Arrays.toString(a));
for (int i = 1; i < aLength; i++) {
for (int j = 0; j < aLength - i; j++) {
if (a[j] < a[j + 1]) {
int stemp = a[j+1];
a[j+1] = a[j];
a[j] = stemp;
}
}
}
System.out.println("排序后的结果"+Arrays.toString(a));
}
运行结果
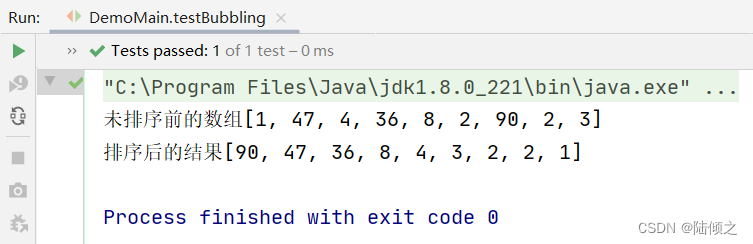
冒泡排序工具类
方法 | 返回类型 | 作用 | 参数说明 | 访问权限 |
---|
ascengSort(byte[] a) | byte[] | 对byte数组升序排序 | 传入byte数组 | 公开 | ascengSort(byte[] a,int fromIndex,int toIndex) | byte[] | 对byte数组具体索引升序排序 | 传入byte数组,开始索引,结束索引 | 公开 | ascengSort(short[] a) | short[] | 对short数组升序排序 | 传入short数组 | 公开 | ascengSort(short[] a,int fromIndex,int toIndex) | short[] | 对short数组具体索引升序排序 | 传入short数组,开始索引,结束索引 | 公开 | ascengSort(int[] a) | int[] | 对int数组升序排序 | 传入int数组 | 公开 | ascengSort(int[] a,int fromIndex,int toIndex) | int[] | 对int数组具体索引升序排序 | 传入int数组,开始索引,结束索引 | 公开 | ascengSort(long[] a) | long[] | 对long数组升序排序 | 传入long数组 | 公开 | ascengSort(long[] a,int fromIndex,int toIndex) | long[] | 对long数组具体索引升序排序 | 传入long数组,开始索引,结束索引 | 公开 | ascengSort(double[] a) | double[] | 对double数组升序排序 | 传入double数组 | 公开 | ascengSort(double[] a,int fromIndex,int toIndex) | double[] | 对double数具体索引升序排序 | 传入double数组,开始索引,结束索引 | 公开 | ascengSort(float[] a) | float[] | 对float数组升序排序 | 传入float数组 | 公开 | ascengSort(float[] a,int fromIndex,int toIndex) | float[] | 对float数组具体索引升序排序 | 传入float数组,开始索引,结束索引 | 公开 | ascengSort(char[] a) | char[] | 对char数组升序排序 | 传入char数组 | 公开 | ascengSort(char[] a,int fromIndex,int toIndex) | char[] | 对char数组具体索引升序排序 | 传入char数组,开始索引,结束索引 | 公开 | dropSort(byte[] a) | byte[] | 对byte数组降序排序 | 传入byte数组 | 公开 | dropSort(byte[] a, int fromIndex, int toIndex) | byte[] | 对byte数组具体索引降序排序 | 传入byte数组,开始索引,结束索引 | 公开 | dropSort(short[] a) | short[] | 对short数组降序排序 | 传入short数组 | 公开 | dropSort(short[] a, int fromIndex, int toIndex) | short[] | 对short数组具体索引降序排序 | 传入short数组,开始索引,结束索引 | 公开 | dropSort(int[] a) | int[] | 对int数组降序排序 | 传入int数组 | 公开 | dropSort(int[] a, int fromIndex, int toIndex) | int[] | 对int数组具体索引降序排序 | 传入int数组,开始索引,结束索引 | 公开 | dropSort(long[] a) | long[] | 对long数组降序排序 | 传入long数组 | 公开 | dropSort(long[] a, int fromIndex, int toIndex) | long[] | 对long数组具体索引降序排序 | 传入long数组,开始索引,结束索引 | 公开 | dropSort(double[] a) | double[] | 对double数组降序排序 | 传入double数组 | 公开 | dropSort(double[] a, int fromIndex, int toIndex) | double[] | 对double数组具体索引降序排序 | 传入double数组,开始索引,结束索引 | 公开 | dropSort(float[] a) | float[] | 对float数组降序排序 | 传入float数组 | 公开 | dropSort(float[] a, int fromIndex, int toIndex) | float[] | 对float数组具体索引降序排序 | 传入float数组,开始索引,结束索引 | 公开 | dropSort(char[] a) | char[] | 对char数组降序排序 | 传入char数组 | 公开 | dropSort(char[] a, int fromIndex, int toIndex) | char[] | 对char数组具体索引降序排序 | 传入char数组,开始索引,结束索引 | 公开 | rangeCheck(int arrayLength, int fromIndex, int toIndex) | void | 索引范围比较 | 数组长度,开始索引,结束索引 | 私有 |
package com.sin.demo.utli;
public class BubbleSortUtlis {
public byte[] ascengSort(byte[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
byte cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public byte[] ascengSort(byte[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
byte cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public short[] ascengSort(short[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
short cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public short[] ascengSort(short[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
short cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public int[] ascengSort(int[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
int cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public int[] ascengSort(int[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
int cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public long[] ascengSort(long[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
long cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public long[] ascengSort(long[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
long cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public double[] ascengSort(double[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
double cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public double[] ascengSort(double[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
double cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public float[] ascengSort(float[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
float cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public float[] ascengSort(float[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
float cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public char[] ascengSort(char[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] > a[j + 1]) {
char cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public char[] ascengSort(char[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] > a[j + 1]) {
char cache = a[j];
a[j] = a[j + 1];
a[j + 1] = cache;
}
}
}
return a;
}
public byte[] dropSort(byte[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
byte cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public byte[] dropSort(byte[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
byte cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public short[] dropSort(short[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
short cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public short[] dropSort(short[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
short cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public int[] dropSort(int[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
int cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public int[] dropSort(int[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
int cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public long[] dropSort(long[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
long cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public long[] dropSort(long[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
long cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public double[] dropSort(double[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
double cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public double[] dropSort(double[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
double cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public float[] dropSort(float[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
float cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public float[] dropSort(float[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
float cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public char[] dropSort(char[] a) {
int aLength = a.length;
int i, j;
for (i = 1; i < aLength; i++) {
for (j = 0; j < aLength - 1; j++) {
if (a[j] < a[j + 1]) {
char cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
public char[] dropSort(char[] a, int fromIndex, int toIndex) {
int aLength = a.length;
this.rangeCheck(aLength, fromIndex, toIndex);
int cycles = fromIndex - toIndex;
int i, j;
for (i = cycles; i < aLength; i++) {
for (j = fromIndex; j < toIndex; j++) {
if (a[j] < a[j + 1]) {
char cache = a[j + 1];
a[j + 1] = a[j];
a[j] = cache;
}
}
}
return a;
}
private void rangeCheck(int arrayLength, int fromIndex, int toIndex) {
if (fromIndex > toIndex) {
throw new IllegalArgumentException(
"开始索引(" + fromIndex + ") > 结束索引(" + toIndex + ")");
}
if (fromIndex < 0) {
throw new ArrayIndexOutOfBoundsException(fromIndex);
}
if (toIndex > arrayLength) {
throw new ArrayIndexOutOfBoundsException(toIndex);
}
}
}
BubbleSortUtlis工具类测试
BubbleSortUtlis bubbleSortUtlis = new BubbleSortUtlis();
int类型数组升序排序
@Test
public void testIntAscengSort() {
int[] a = {2,5,3,8,1,9,23,11,6};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.ascengSort(a);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
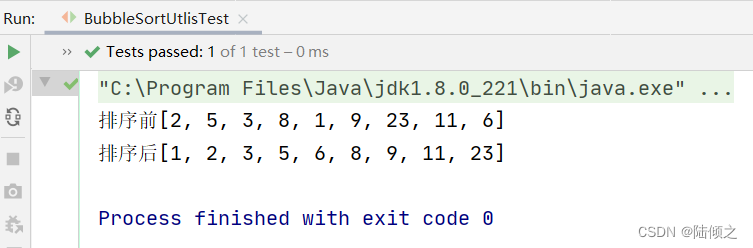
int类型数组对具体元素升序排序
@Test
public void testIntAscengSort() {
int[] a = {2,5,3,8,1,9,23,11,6};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.ascengSort(a,3,7);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
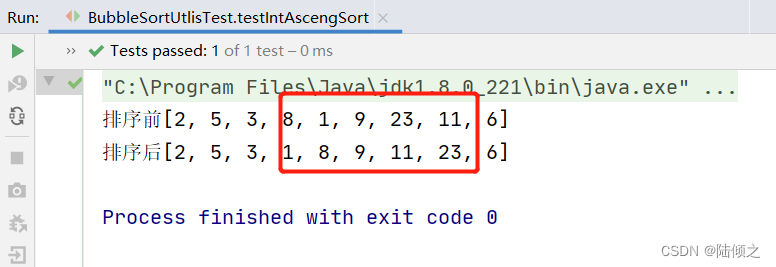
int类型降序排序
@Test
public void testIntAscengSort() {
int[] a = {2,5,3,8,1,9,23,11,6};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.dropSort(a);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
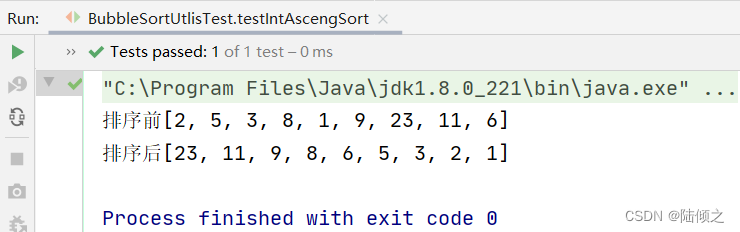
int类型对具体元素降序排序
@Test
public void testIntAscengSort() {
int[] a = {2,5,3,8,1,9,23,11,6};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.dropSort(a,2,5);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
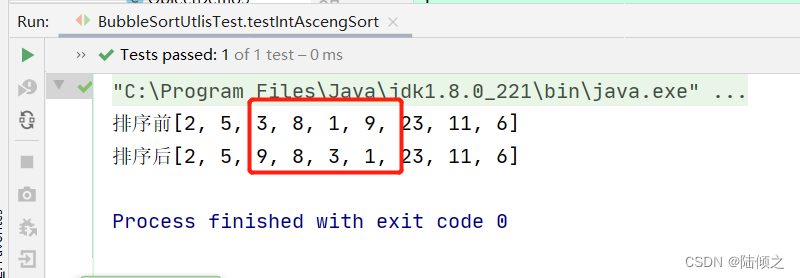
char类型数组升序排序
@Test
public void testCharAscengSort() {
char[] a = {'a', 'v', 'd', 'e', 'f', 'c', 'b'};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.ascengSort(a);
System.out.println("排序后"+Arrays.toString(a));
}
结束结果 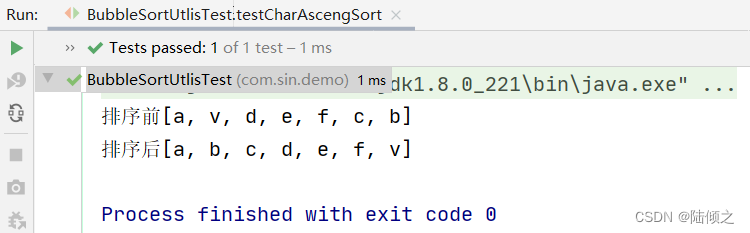
char类型数组对具体元素升序排序
@Test
public void testCharAscengSort() {
char[] a = {'a', 'v', 'd', 'e', 'f', 'c', 'b'};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.ascengSort(a,2,5);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
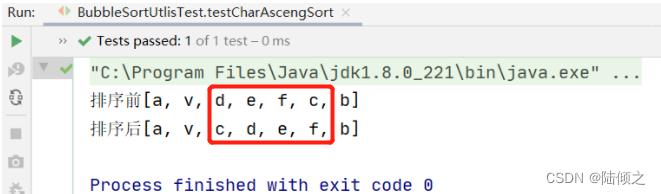
char类型数组降序排序
@Test
public void testCharAscengSort() {
char[] a = {'a', 'v', 'd', 'e', 'f', 'c', 'b'};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.dropSort(a);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
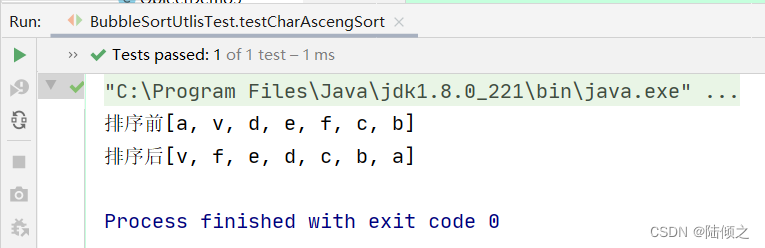
char类型数组对具体元素降序排序
@Test
public void testCharAscengSort() {
char[] a = {'a', 'v', 'd', 'e', 'f', 'c', 'b'};
System.out.println("排序前"+Arrays.toString(a));
bubbleSortUtlis.dropSort(a,2,4);
System.out.println("排序后"+Arrays.toString(a));
}
测试结果
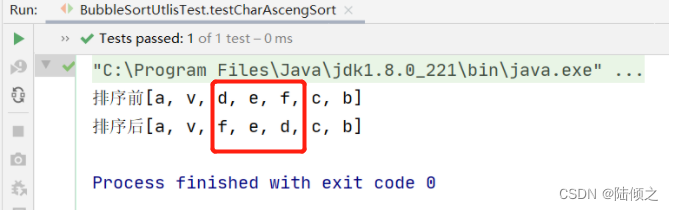
|