1. 删除链表中的节点
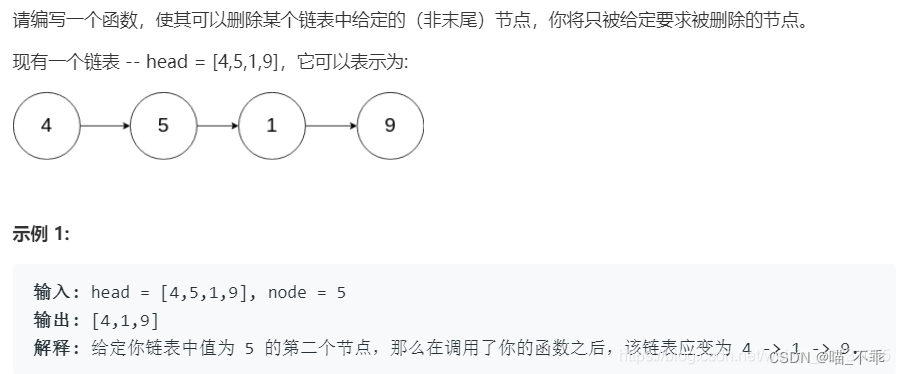  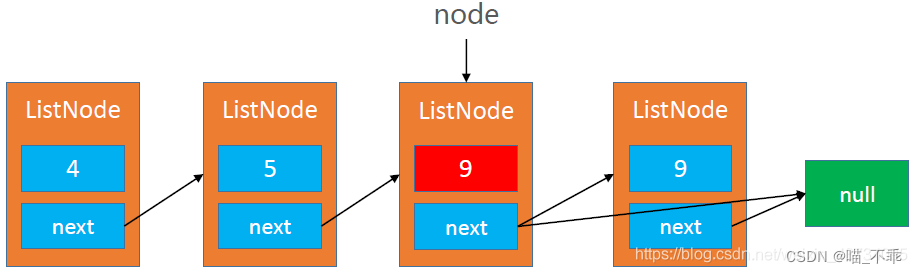
class Solution {
public void deleteNode(ListNode node) {
node.val = node.next.val;
node.next = node.next.next;
}
}
2. 反转一个链表(非递归解法)
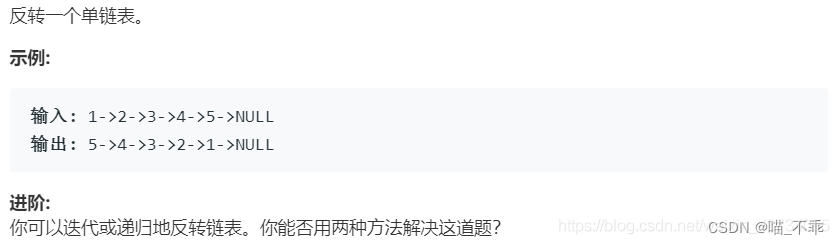
class Solution {
public ListNode reverseList(ListNode head) {
ListNode newHead = null;
while (head != null) {
ListNode tmp = head.next;
head.next = newHead;
newHead = head;
head = tmp;
}
return newHead;
}
}
3. 判断一个链表是否有环(快慢指针)
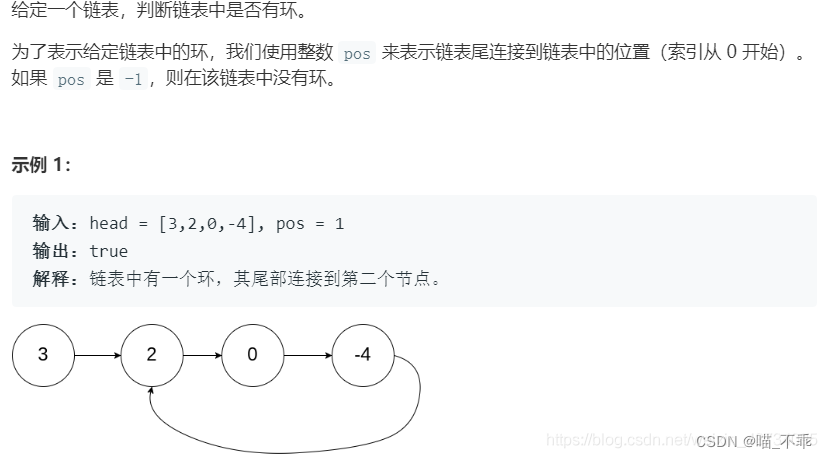 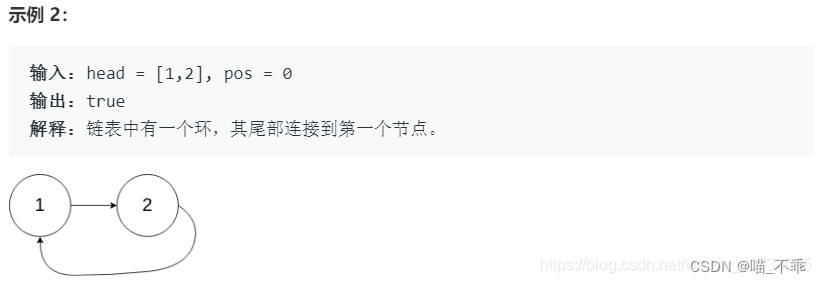 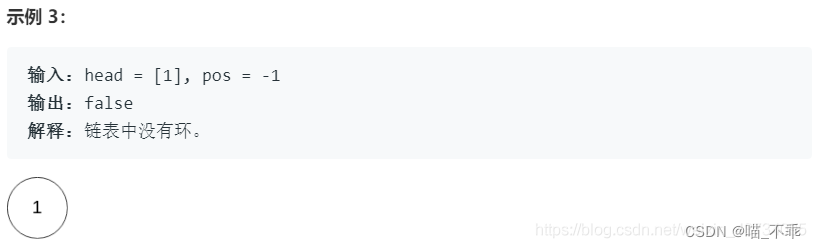 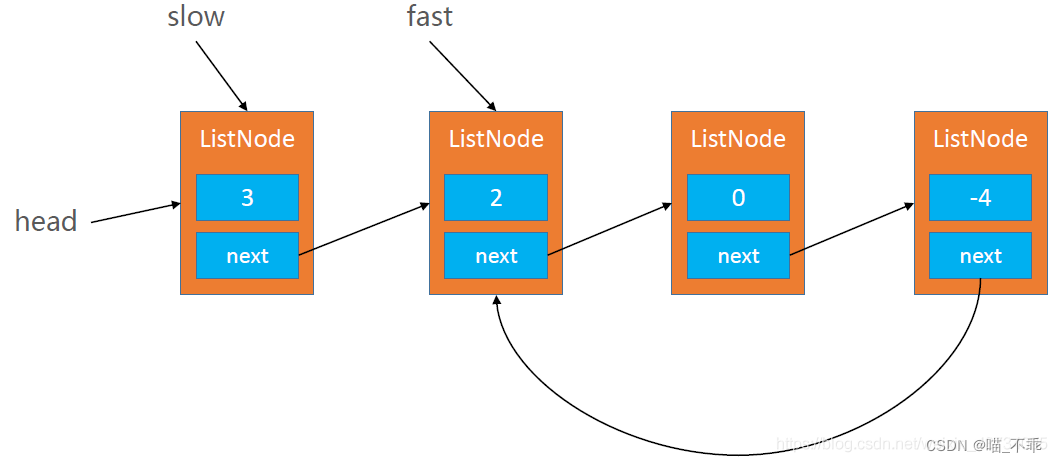
public class Solution {
public boolean hasCycle(ListNode head) {
if(head == null || head.next == null) return false;
ListNode slow = head;
ListNode fast = head.next;
while (fast != null && fast.next != null) {
if (slow.val == fast.val) return true;
slow = slow.next;
fast = fast.next.next;
}
return false;
}
}
慢指针每次移动一格,快指针每次移动两格,在有环的链表里,他们一定会相遇 1、当快指针就在慢指针后面,那么下一次慢指针移动一位,快指针移动两位,相遇 2、当快指针和慢指针差一个位置,那么下一次慢指针移动一位,快指针移动两位,他们会变成第一种情况 3、当快指针和慢指针差两个位置,那么下一次慢指针移动一位,快指针移动两位,他们会变成第二种情况 我知道你也在纠结为什么会没有快指针跳过慢指针他们没有相遇的这种情况发生,但是这种情况只会发生在他们相遇后的下一次移动 原因:其实从上面的三步不难看出:快指针是一格一格追赶慢指针的,即他们的距离是…4->3->2->1->0这样缩短的。所以一定会相遇 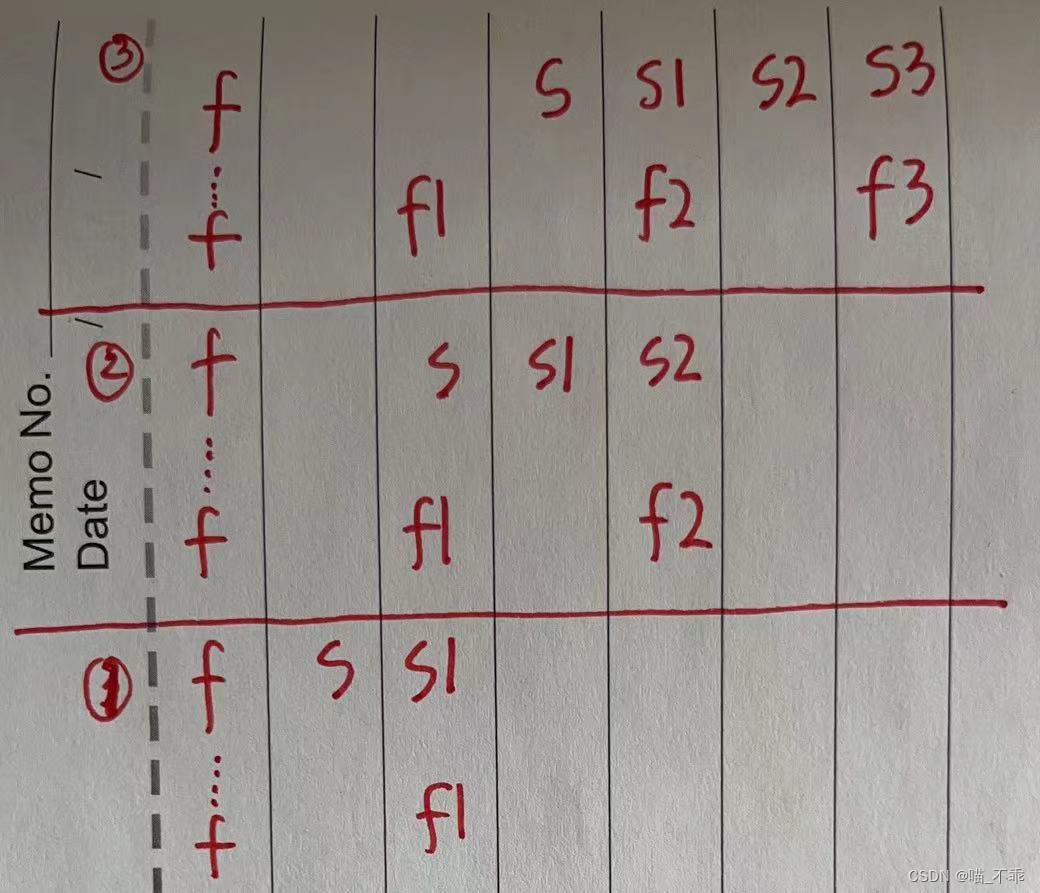
4. 获取单链表的节点个数
public static int getLinkListNodeLength(Node head){
if(head == null){
return 0;
}
int length = 0;
Node currentNode = head;
while(currentNode != null){
length++;
currentNode = currentNode.next;
}
return length;
}
5. 查询单链表中倒数第K个节点
static Node getLastIndex(Node first, int k) {
if (first == null) {
return null;
}
int length = getLinkListNodeLength(first);
if (k < 0 || k > length) {
return null;
}
Node cur = first;
for (int i = 0; i < length - k; i++) {
cur = cur.next;
}
return cur;
}
6. 逆序打印单向链表(栈)
LinkedList linkedList = new LinkedList<Integer>();
linkedList.add(0,0);
linkedList.add(1,1);
linkedList.add(2,2);
linkedList.add(3,3);
Stack<Integer> stacks = new Stack<>();
LinkedList.Node cur = linkedList.getFirst();
while (cur != null) {
stacks.push((Integer) cur.element);
cur = cur.next;
}
while (stacks.size() > 0) {
System.out.println(stacks.pop());
}

7. 约瑟夫环问题

1. 构建环形链表并遍历
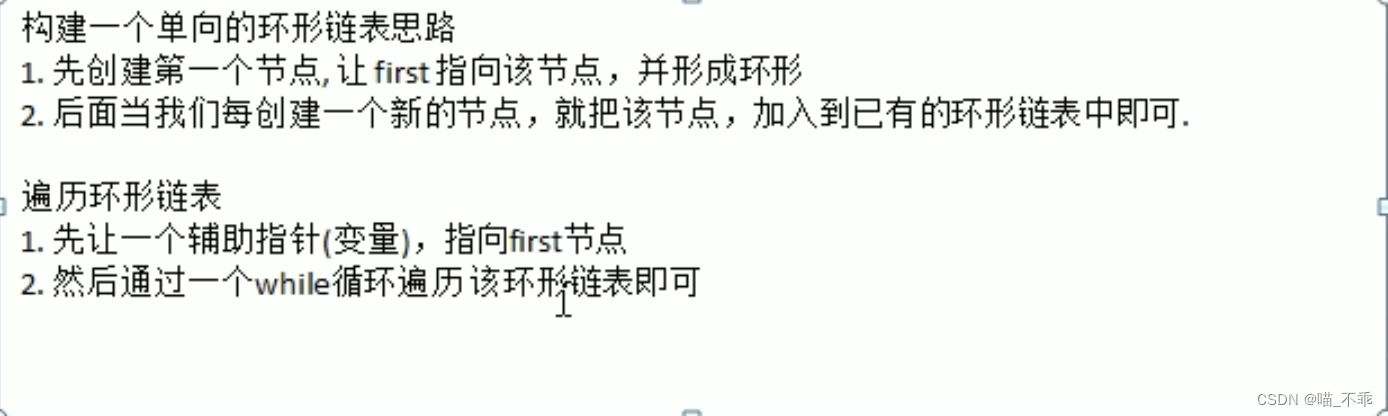
2. 出圈
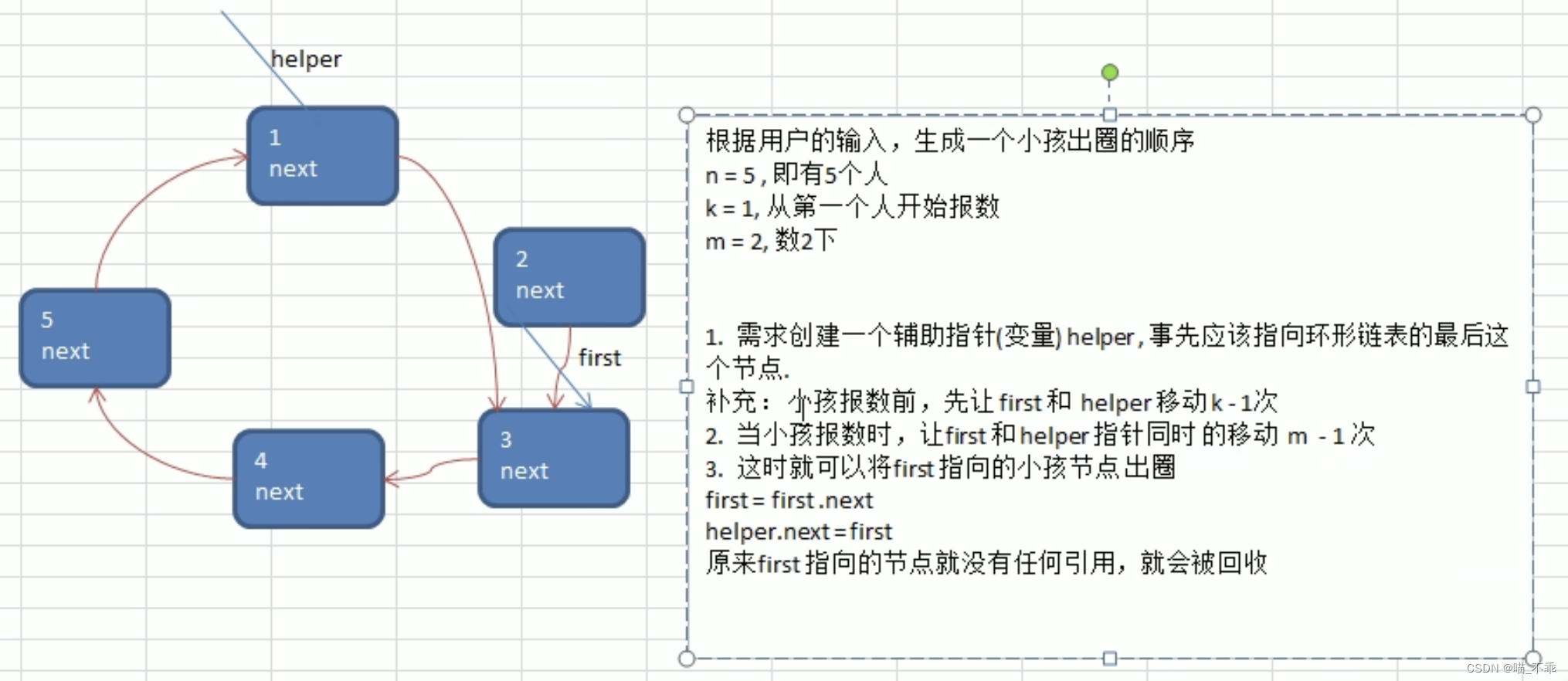
public class JosepfuDemo {
public static void main(String[] args) {
CircleSingleLinkedList circleSingleLinkedList = new CircleSingleLinkedList();
circleSingleLinkedList.addBoy(5);
circleSingleLinkedList.show();
circleSingleLinkedList.countBoy(1, 2, 5);
}
}
class CircleSingleLinkedList {
private Boy first;
void addBoy(int nums) {
Boy cur = null;
for (int i = 1; i <= nums; i++) {
Boy boy = new Boy(i);
if (i == 1) {
first = boy;
first.setNext(first);
cur = boy;
} else {
cur.setNext(boy);
boy.setNext(first);
cur = boy;
}
}
}
void countBoy(int startNo, int countNum, int nums) {
if (first == null || startNo < 1 || startNo > nums) {
System.out.println("参数输入有误, 请重新输入");
return;
}
Boy helper = first;
while (true) {
if (helper.getNext() == first) {
break;
}
helper = helper.getNext();
}
for (int i = 0; i < startNo - 1; i++) {
helper = helper.getNext();
first = first.getNext();
}
while (true) {
if (first == helper) {
System.out.println("最后一个小孩:" + first.getNo());
break;
}
for (int i = 0; i < countNum - 1; i++) {
helper = helper.getNext();
first = first.getNext();
}
System.out.println("出圈:" + first.getNo());
first = first.getNext();
helper.setNext(first);
}
}
void show() {
Boy cur = first;
while (true) {
System.out.println(cur.getNo());
if (cur.getNext() == first) {
break;
}
cur = cur.getNext();
}
}
}
class Boy {
private int no;
private Boy next;
public Boy(int no) {
this.no = no;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public Boy getNext() {
return next;
}
public void setNext(Boy next) {
this.next = next;
}
}

问题1-3参考:https://luzhenyu.blog.csdn.net/article/details/104609375 问题4参考:获取单链表的节点个数:https://blog.csdn.net/jcm666666/article/details/52278471 快慢指针为什么一定会相遇:https://blog.csdn.net/Leslie5205912/article/details/89386769 约瑟夫环问题:https://www.bilibili.com/video/BV1E4411H73v?p=28&vd_source=b901ef0e9ed712b24882863596eab0ca 单向链表反转讲解(真的一看就懂):https://www.bilibili.com/video/BV1Ai4y157mn/?spm_id_from=333.337.search-card.all.click&vd_source=b901ef0e9ed712b24882863596eab0ca
|