testng在maven项目中的使用
pom.xml
<dependencies>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<forkCount>0</forkCount>
<testFailureIgnore>true</testFailureIgnore>
<suiteXmlFiles>
<suiteXmlFiles>testng.xml</suiteXmlFiles>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
com.yineng.aos.testng.TestngGroups
package com.yineng.aos.testng;
import org.testng.annotations.Test;
public class TestngGroups {
@Test(groups = {"group1", "group2"})
public void testMethod1() {
System.out.println("group1 group2 testMethod1");
}
@Test(groups = {"group1", "group2"})
public void testMethod2() {
System.out.println("group1 group2 testMethod2");
}
@Test(groups = {"group1"})
public void testMethod3() {
System.out.println("group1 testMethod3");
}
@Test(groups = {"group2"})
public void testMethod4() {
System.out.println("group2 testMethod4");
}
}
testng.xml
<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite1">
<test name="login">
<groups>
<run>
<inlude name="group2" />
</run>
</groups>
<classes>
<class name="com.yineng.aos.testng.TestngGroups" />
</classes>
</test>
</suite>
idea中,可以双击执行:
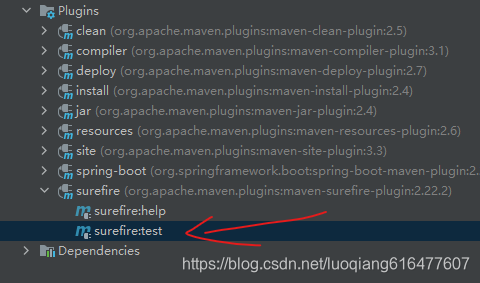
命令行输入命令执行:
mvn test
testng.xml的配置说明
<test>元素是<suite>的子元素,用以定义一个测试用例。定义测试用例可以通过<classes>或<packages>。
<!ELEMENT test (method-selectors?,parameter*,groups?,packages?,classes?) >
1). <classes>表示以测试类的方式定义测试用例,粒度较小。
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite1" verbose="1" >
<test name="Regression1">
<classes>
<class name="com.yineng.aos.testng.TestngGroups"/>
<class name="com.yineng.aos.testng.TestngGroups2"/>
</classes>
</test>
</suite>
2). <packages>表示以测试类所在的包的方式定义测试用例,包中的所有测试类都被涉及,粒度较大。
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite1" verbose="1" >
<test name="Regression1" >
<packages>
<package name="com.yineng.aos.testng" />
</packages>
</test>
</suite>
3). <groups>元素
我们知道,<suite>中可以定义一个全局的<groups>。而这里<test>元素中也可以定义一个自己的<groups>,其中定义的组仅对当前所在的测试用例可见。示例如下:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<test name="login">
<groups>
<run>
<exclude name="group2" />
<include name="group1" />
</run>
</groups>
<classes>
...
</classes>
</test>
注意:在testng.xml配置文件中,<suite>中可定义多个<test>,<test>的执行顺序默认按照在<suite>中出现先后顺序。也可提供<test>的preserve-order='false'改变默认顺序。
3. <methods>
具体到测试类的某个方法,对于<classes>中的一个<class>,可以提供<methods>设置测试方法。示例如下:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<test name="login">
<classes>
<class name="com.yineng.aos.testng.TestngGroups">
<methods>
<include name="testMethod1" />
<include name="testMethod2" />
</methods>
</class>
<class name="com.yineng.aos.testng.TestngGroups2" />
</classes>
</test>
可通过指定<suiteXmlFiles>属性来运行指定的testng.xml
(将< suiteXmlFile>的value值设置为引用properties更灵活。执行命令时就可指定testng.xml 例如:mvn clean test -DsuiteXmlFile=src/test/resources/xml/a.xml)
<properties>
<suiteXmlFile>testng.xml</suiteXmlFile>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<encoding>UTF-8</encoding>
<suiteXmlFiles>
<suiteXmlFile>${suiteXmlFile}</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
mvn clean test -DsuiteXmlFile=src/test/resources/xml/a.xml
更多surefire plugin的使用方法可以通过下面的两种方法查看:
mvn help:describe -Dplugin=org.apache.maven.plugins:maven-surefire-plugin:2.22.2 -Ddetail
mvn surefire:help -Ddetail=true -Dgoal=test
|