- 简介
数据驱动概念:数据和操作分离 关键字驱动概念:将重复操作的函数和方法封装,其他人员调用该方法或函数皆可。 POM核心四层:基类、页面对象类、测试用例类、测试数据类 基类(base):主要用于提供常用的函数、为页面对象类进行服务(appium常用函数一般是元素定位、输入、点击、滑动等) 页面对象类(pageObject):提取所有可被自动化执行的页面,类中包含页面的核心元素与页面的核心流程 测试用例类(Cases):测试代码部分,用于拼接各类页面对象、实现最终的测试流程 测试数据类(Data):用于提取所有在实际测试过程中需要的应用数据内容 - 分层介绍:
- 代码实现:
'''Base包下的BasicOperation.py'''
from time import sleep
class BasicOperation:
def __init__(self,driver):
self.driver=driver
def locator(self,loc):
return self.driver.find_element(*loc)
def input_content(self,loc,text):
self.locator(loc).send_keys(text)
def click_operation(self,loc):
self.locator(loc).click()
def swipe(self,start_x,start_y,end_x,end_y,duration=0):
window_size=self.driver.get_window_size()
x=window_size['width']
y=window_size['height']
self.driver.swipe(start_x=x*start_x,start_y=y*start_y,end_x=x*end_x,end_y=y*end_y,duration=duration)
def wait_time(self,time):
sleep(time)
def quit_(self):
self.driver.quit()
'''Config包下的File_Read.py'''
import os
from configparser import ConfigParser
import yaml
class ReadFile:
def __init__(self,fileName=None):
if fileName:
self.FilePathName=fileName
else:
current_path=os.path.dirname(os.path.dirname(__file__))
self.FilePathName=current_path+'/Data/config.ini'
def ReadYaml(self):
try:
with open(self.FilePathName,mode='r',encoding='utf-8')as f:
data=yaml.load(f,Loader=yaml.FullLoader)
except IOError as e:
print("文件读取失败",e)
finally:
f.close()
return data
def WriteYaml(self,datas):
try:
with open(self.FilePathName,mode='w',encoding='utf-8') as f:
data=yaml.dump(datas,f,allow_unicode=True)
except IOError as e:
print('文件读取失败',e)
finally:
f.close()
def ReadConfig_param(self,title,param):
self.cf=MyConfigParser()
self.cf.read(self.FilePathName,encoding='utf-8')
value=self.cf.get(title,param)
return value
def ReadConfigItem(self,title):
self.cf= MyConfigParser()
self.cf.read(self.FilePathName,encoding='utf-8')
datas=dict(self.cf.items(title))
return datas
class MyConfigParser(ConfigParser):
def optionxform(self, optionstr):
return optionstr
'''PageObject包下的LoginPage.py'''
from appium.webdriver.common.mobileby import MobileBy
from Base.BasicOperation import BasicOperation
class LoginPage(BasicOperation):
loginBounced=(MobileBy.ID,'bt_to_login')
agreementAgree=(MobileBy.ID,'tv_agree')
phoneInputBox=(MobileBy.ID,'et_phone')
passwordInputBox=(MobileBy.ID,'et_pwd_one')
checkBox=(MobileBy.ID,'cb_login')
loginButton=(MobileBy.ID,'bt_login')
def login(self,phone,password):
self.wait_time(15)
self.click_operation(self.loginBounced)
self.wait_time(15)
self.click_operation(self.agreementAgree)
self.wait_time(5)
self.input_content(self.phoneInputBox,phone)
self.input_content(self.passwordInputBox,password)
if self.locator(self.checkBox).get_attribute('checked')=='false':
self.click_operation(self.checkBox)
self.click_operation(self.loginButton)
self.wait_time(5)
'''测试用例'''
import pytest
from Config.File_Read import ReadFile
from PageObject.LoginPage import LoginPage
from appium import webdriver
class TestLogin:
def setup(self):
data=ReadFile("../Data/appDesiredCaps.yaml").ReadYaml()
driver=webdriver.Remote('http://127.0.0.1:4723/wd/hub',data['desiredcaps'])
self.login_page=LoginPage(driver)
@pytest.mark.parametrize('phone,pwd',[('1234569','123456cs'),('12345619','123456')])
def test_01_login(self,phone,pwd):
self.login_page.login(phone,pwd)
if __name__ == '__main__':
pytest.main(['-vs','./test_login.py'])
保存的ini文件内容: 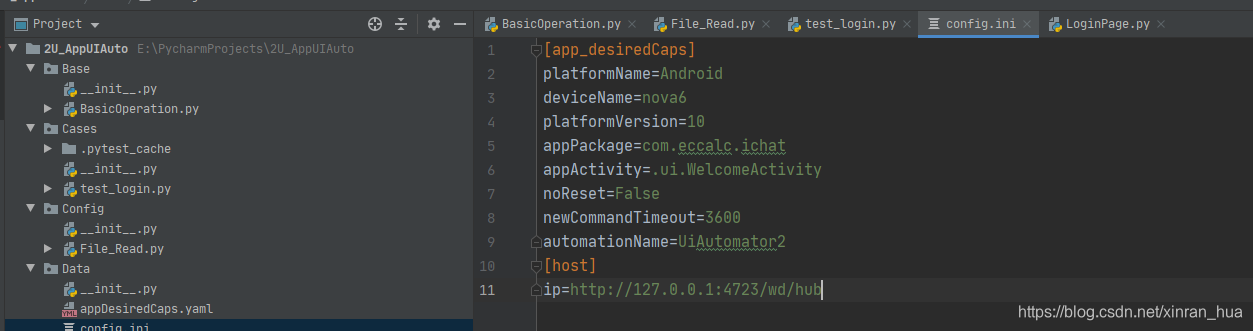 保存的yaml文件内容: 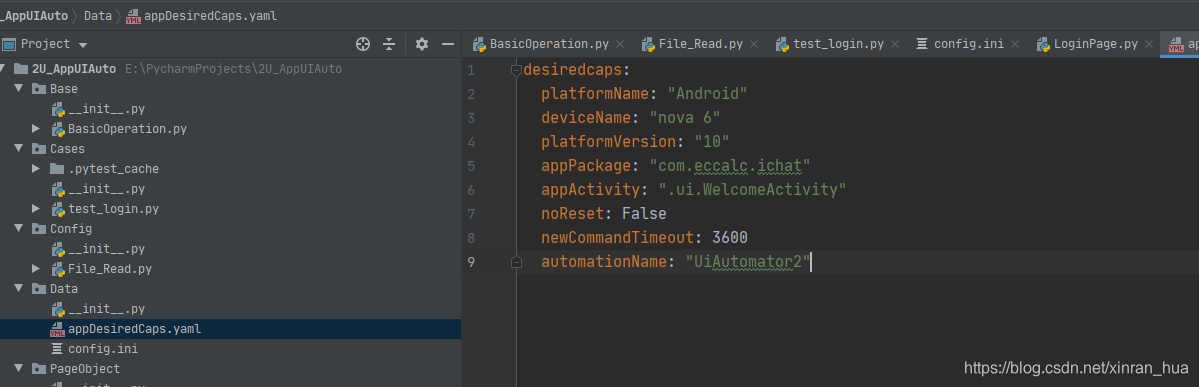
|