.net core 未来预测会火,随便写一个先练习熟悉一下。
现在主流前后端分离,所以后台采用webapi
层级结构大概这样,WebAPI 授权和鉴权 用JWT 先简单看一下
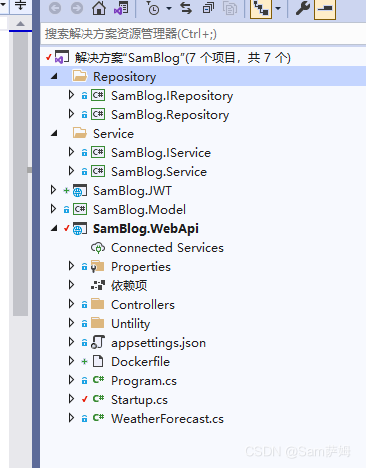
测试:
运行JWT
?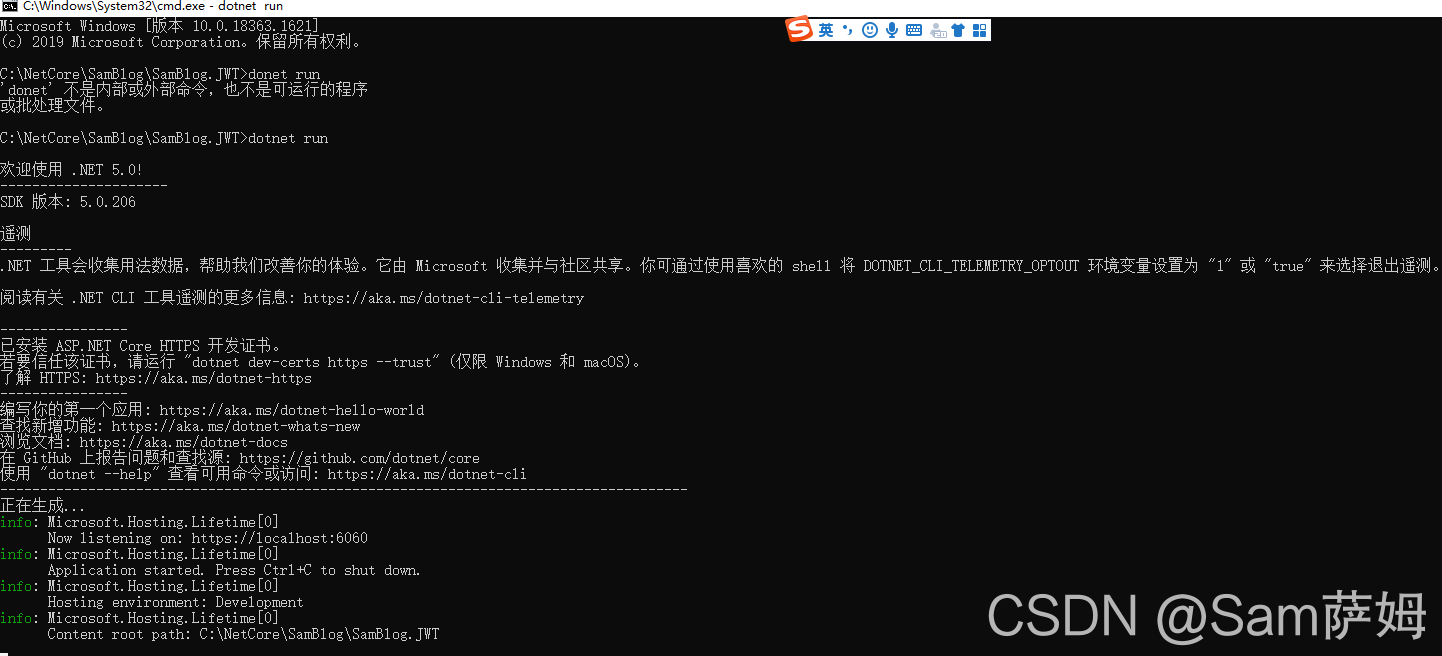
?
输入用户名密码获取token
?
运行web api
地址:http://localhost:5000/swagger/index.html
?
测试创建博客文章
?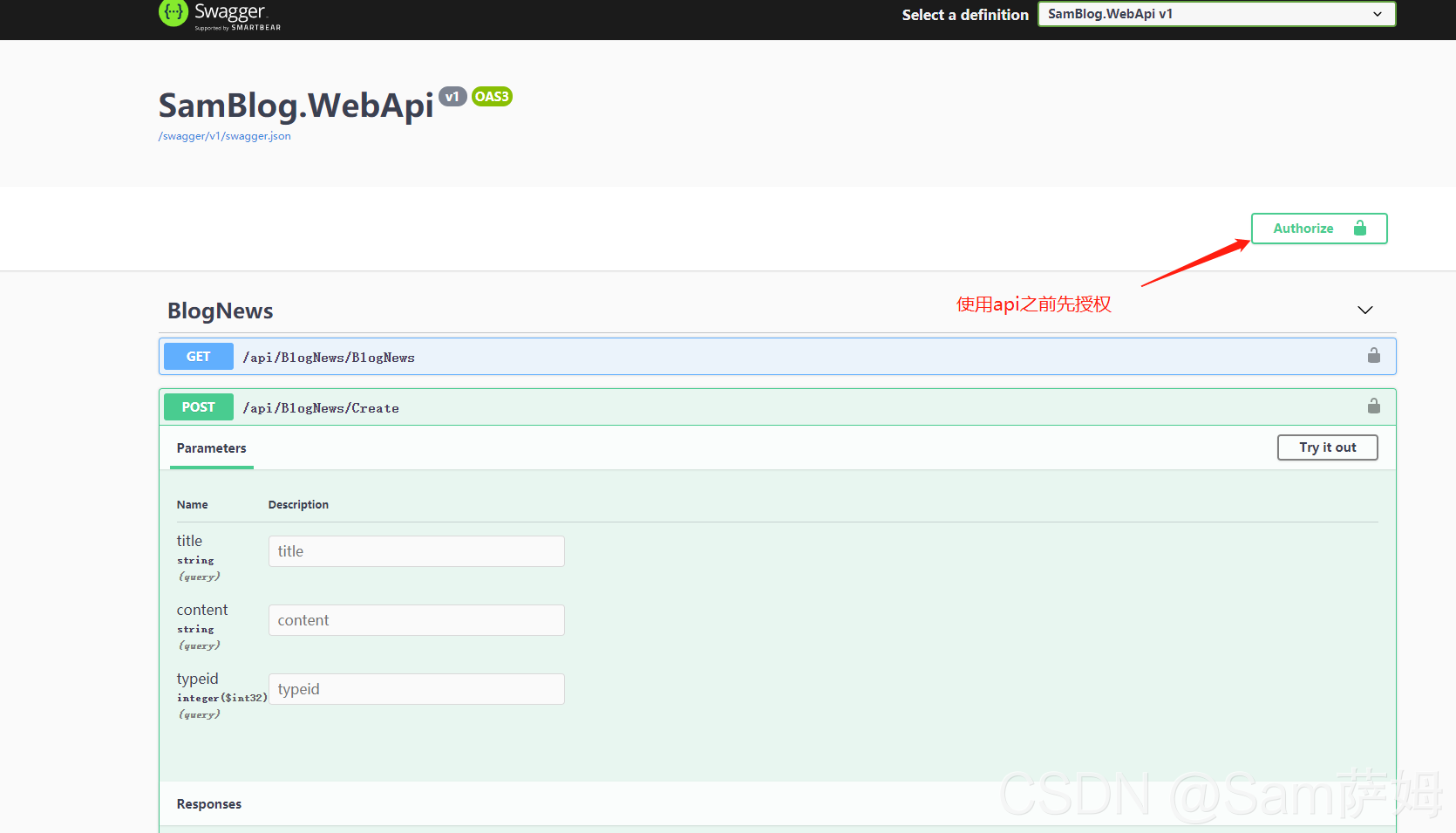
填好刚才的token
?
测试创建接口
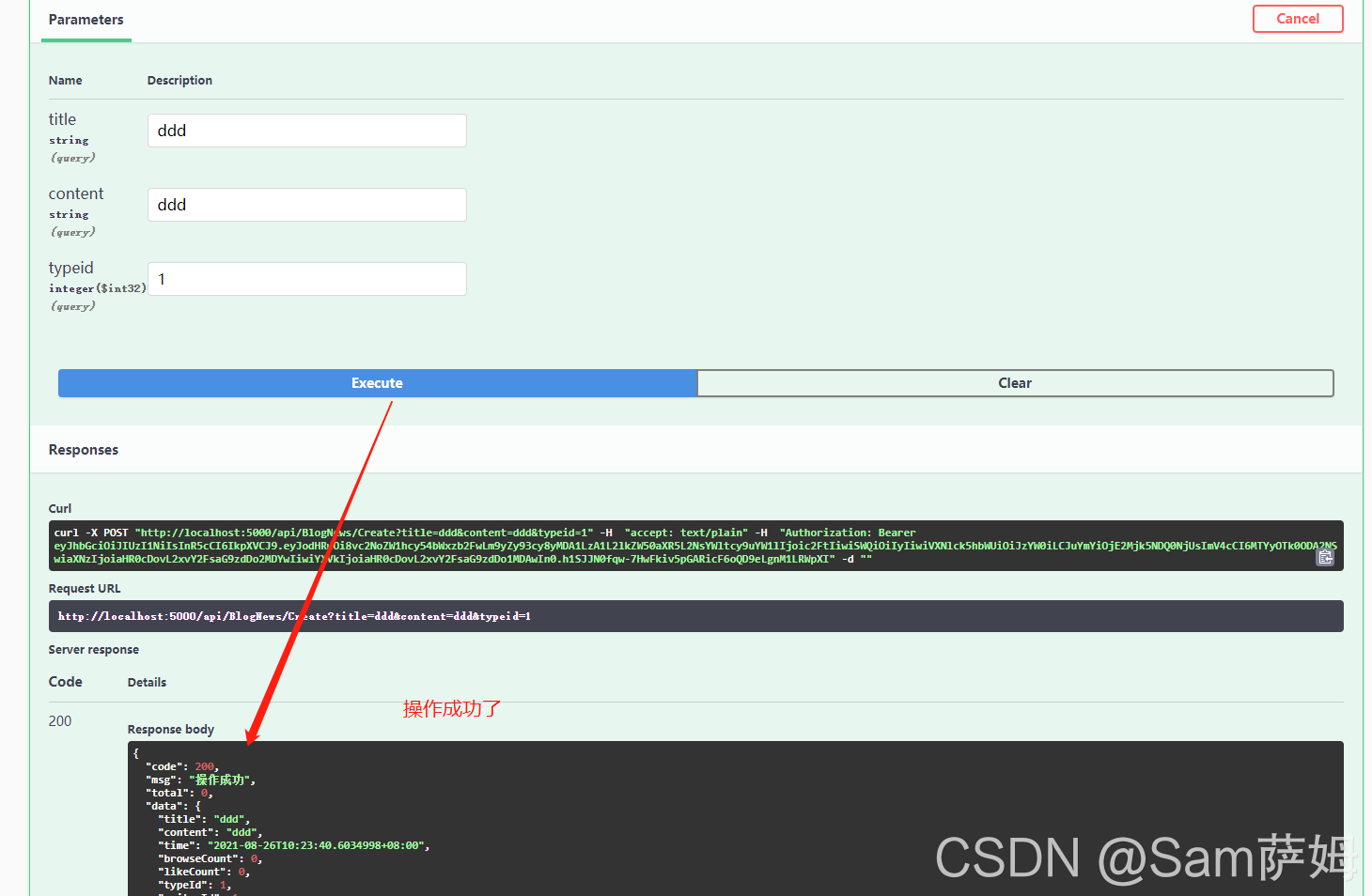
?基础仓库代码段?
using SamBlog.IRepository;
using SamBlog.Model;
using SqlSugar;
using SqlSugar.IOC;
using System;
using System.Collections.Generic;
using System.Linq.Expressions;
using System.Text;
using System.Threading.Tasks;
namespace SamBlog.Repository
{
public class BaseRepository<TEntity> : SimpleClient<TEntity>, IBaseRepository<TEntity> where TEntity : class, new()
{
public BaseRepository(ISqlSugarClient context=null):base(context)
{
base.Context = DbScoped.Sugar;
// 创建数据库
base.Context.DbMaintenance.CreateDatabase();
// 创建表
base.Context.CodeFirst.InitTables(
typeof(BlogNews),
typeof(TypeInfo),
typeof(WriterInfo)
);
}
public async Task<bool> CreateAsync(TEntity entity)
{
return await base.InsertAsync(entity);
}
public async Task<bool> DeleteAsync(int id)
{
return await base.DeleteByIdAsync(id);
}
public async Task<bool> EditAsync(TEntity entity)
{
return await base.UpdateAsync(entity);
}
//导航查询
public virtual async Task<TEntity> FindAsync(int id)
{
return await base.GetByIdAsync(id);
}
public async Task<TEntity> FindAsync(Expression<Func<TEntity, bool>> func)
{
return await base.GetSingleAsync(func);
}
public virtual async Task<List<TEntity>> QueryAsync()
{
return await base.GetListAsync();
}
public virtual async Task<List<TEntity>> QueryAsync(Expression<Func<TEntity, bool>> func)
{
return await base.GetListAsync(func);
}
public virtual async Task<List<TEntity>> QueryAsync(int page, int size, RefAsync<int> total)
{
return await base.Context.Queryable<TEntity>()
.ToPageListAsync(page, size, total);
}
public virtual async Task<List<TEntity>> QueryAsync(Expression<Func<TEntity, bool>> func, int page, int size, RefAsync<int> total)
{
return await base.Context.Queryable<TEntity>()
.Where(func)
.ToPageListAsync(page, size, total);
}
}
}
?
博客文章的增删改查接口代码段
using AutoMapper;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using SamBlog.IService;
using SamBlog.Model;
using SamBlog.Model.DTO;
using SamBlog.WebApi.Untility.ApiResult;
using SqlSugar;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace SamBlog.WebApi.Controllers
{
[Route("api/[controller]")]
[ApiController]
[Authorize]
public class BlogNewsController : ControllerBase
{
private readonly IBlogNewsService _iBlogNewsService;
public BlogNewsController(IBlogNewsService iblogNewsService)
{
this._iBlogNewsService = iblogNewsService;
}
[HttpGet("BlogNews")]
public async Task<ActionResult<ApiResult>> GetBlogNews()
{
int id = Convert.ToInt32(this.User.FindFirst("Id").Value);
var data = await _iBlogNewsService.QueryAsync(c => c.WriterId == id);
if (data == null) return ApiResultHelper.Error("没有更多的文章");
return ApiResultHelper.Success(data);
}
[HttpPost("Create")]
public async Task<ActionResult<ApiResult>> Create(string title,string content,int typeid)
{
BlogNews blogNews = new BlogNews
{
BrowseCount = 0,
Content = content,
LikeCount = 0,
Time = DateTime.Now,
Title = title,
TypeId = typeid,
WriterId=1
//WriterId = Convert.ToInt32(this.User.FindFirst("Id").Value)
};
bool result =await _iBlogNewsService.CreateAsync(blogNews);
if (!result) return ApiResultHelper.Error("添加失败,服务器发生错误");
return ApiResultHelper.Success(blogNews);
}
[HttpDelete("Delete")]
public async Task<ActionResult<ApiResult>>Delete(int id)
{
bool result = await _iBlogNewsService.DeleteAsync(id);
if(!result) return ApiResultHelper.Error("删除失败!");
return ApiResultHelper.Success(result);
}
[HttpPut("Edit")]
public async Task<ActionResult<ApiResult>> Edit(int id, string title, string content, int typeid)
{
var blogNews = await _iBlogNewsService.FindAsync(id);
if (blogNews == null) return ApiResultHelper.Error("没有找到该文章");
blogNews.Title = title;
blogNews.Content = content;
blogNews.TypeId = typeid;
bool result = await _iBlogNewsService.EditAsync(blogNews);
if (!result) return ApiResultHelper.Error("修改失败");
return ApiResultHelper.Success(blogNews);
}
[HttpGet("BlogNewsPage")]
public async Task<ApiResult> GetBlogNewsPage([FromServices] IMapper iMapper, int page, int size)
{
RefAsync<int> total = 0;
var blognews = await _iBlogNewsService.QueryAsync(page, size, total);
try
{
var blognewsDTO = iMapper.Map<List<BlogNewsDTO>>(blognews);
return ApiResultHelper.Success(blognewsDTO, total);
}
catch (Exception)
{
return ApiResultHelper.Error("AutoMapper映射错误");
}
}
}
}
总结:
.net core 写后台和传统的 其实差不太多。现在发布docker 还有点问题,微软要在2021年11月份发布新的VS IDE 到时候在试试。
?
|