本期内容
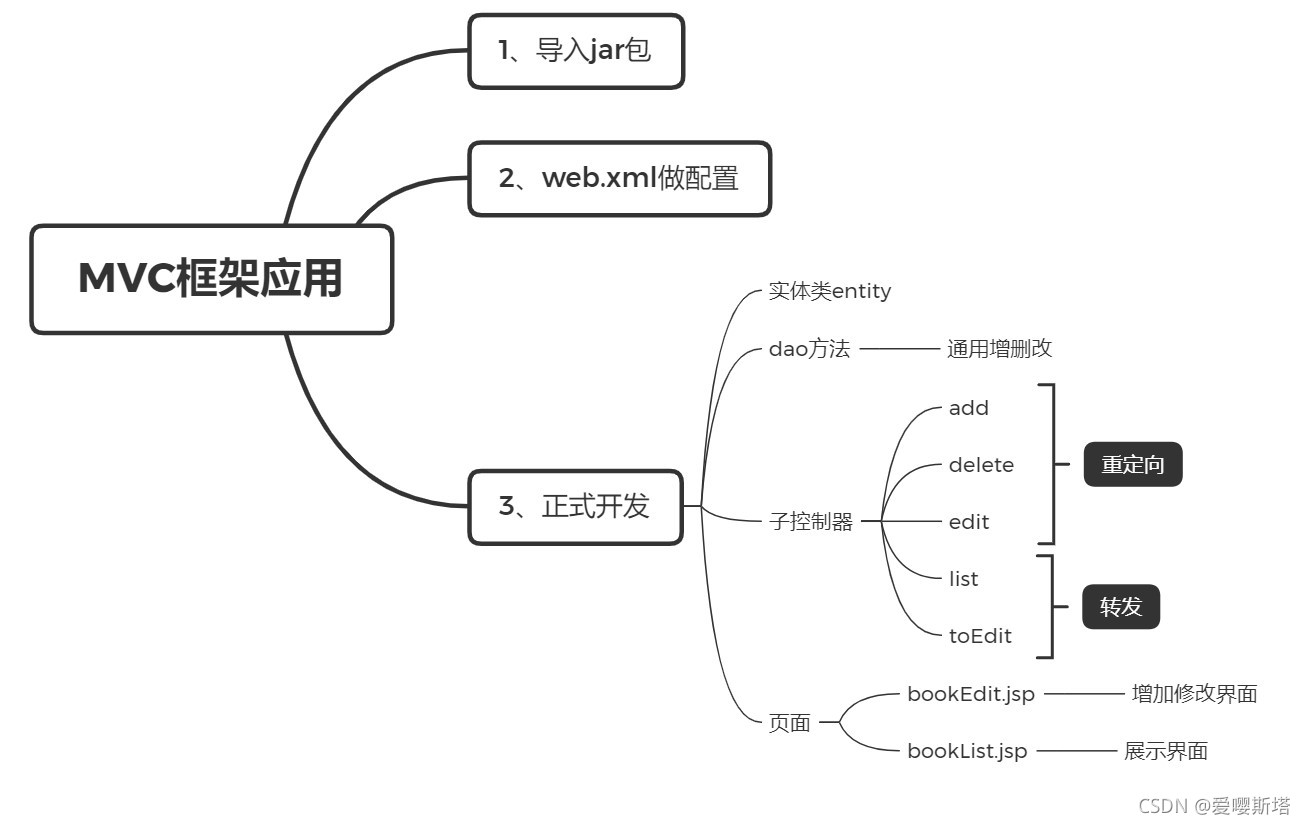
?一、导入jar包
1、如何导出自己写出的MVC框架形成jar包?
①、在文件右键以下,找到Export...
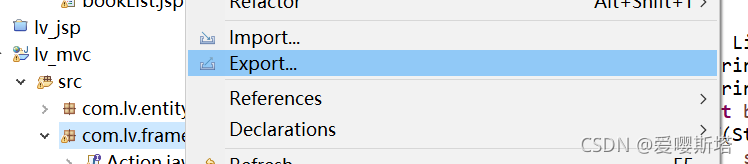
?②、点击JAR file
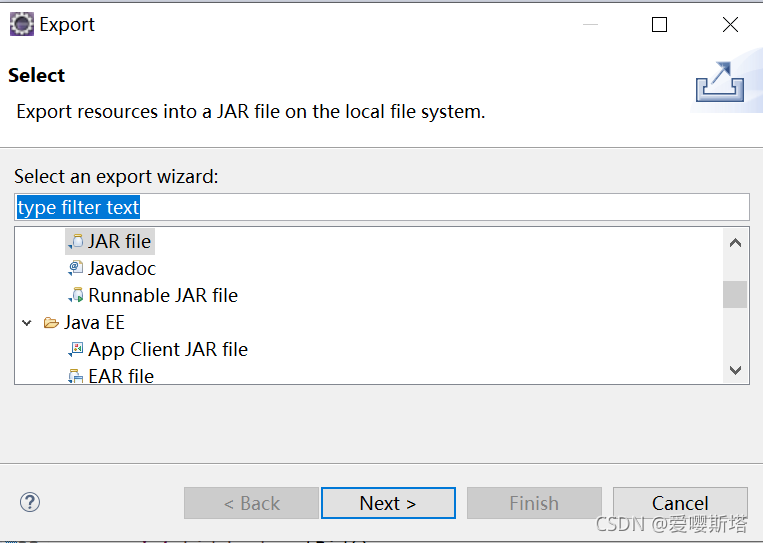
③、寻找存放地点(名字.jar),然后点击next,直到finish
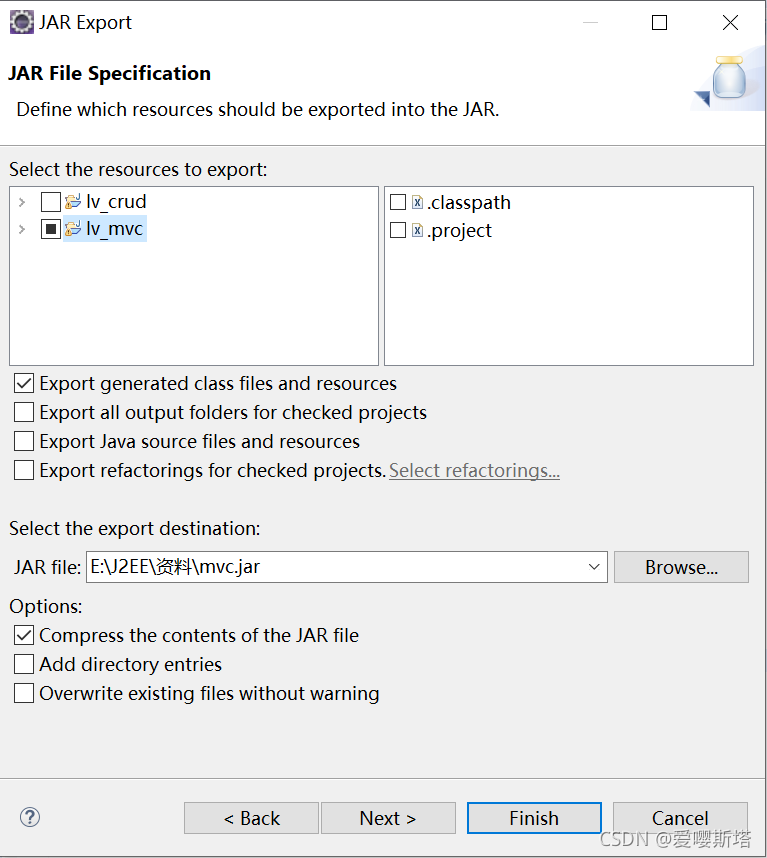
?2、就可以利用我们自己写的jar包了,导入我们需要写的文件里
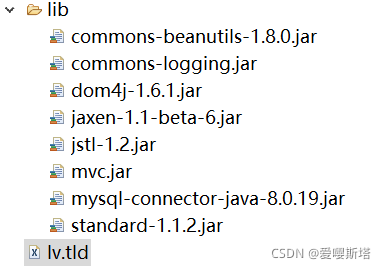
二、web.xml做配置
<?xml version="1.0" encoding="UTF-8"?> <config> ?? ?<!--? ?? ??? ?在这里每加一个配置,就相当于actions.put("/goods", new GoodsAction()); ?? ??? ?这样就解决了代码灵活性的问题 ?? ? --> ?? ?<action path="/book" type="com.lv.web.BookAction"> ?? ??? ?<forward name="list" path="/bookList.jsp" redirect="false" /> ?? ??? ?<forward name="toList" path="/book.action?methodName=list" redirect="true" /> ?? ??? ?<forward name="toEdit" path="/bookEdit.jsp" redirect="false" /> ?? ?</action> ? ? ? ? </config>
三、正式开发
实体类:Book:
所有Dao类的父类:
public class BaseDao<T> { /** ?* 通用的增删改方法 ?* ?? ?思路: ?* ?? ??? ?1、从传进来的t中读取属性值 ?* ?? ??? ?2、往预定义对象中设置了值 ?* @param sql ?* @param t ?* @throws Exception ?*/ ?? ?public void executeUpdate(String sql,T t,String[] attrs) throws Exception { //?? ??? ?String[] attrs=new String[] {"bid","bname","price"}; ?? ??? ?Connection con=DBAccess.getConnection(); ?? ??? ?PreparedStatement ps=con.prepareStatement(sql); ?? ??? ?for (int i = 0; i < attrs.length; i++) { ?? ??? ??? ?Field f = t.getClass().getDeclaredField(attrs[i]); //?? ??? ??? ?打开访问权限 //?? ??? ??? ?t-->book,f-->bid ?? ??? ??? ?f.setAccessible(true); ?? ??? ??? ?ps.setObject(i+1, f.get(t)); ?? ??? ?} ?? ??? ?ps.executeUpdate(); ?? ?} ?? ? ? ? }
Dao方法:
package com.lv.dao;
import java.util.List;
import com.lv.entity.Book; import com.lv.util.BaseDao; import com.lv.util.PageBean; import com.lv.util.StringUtils;
public class BookDao extends BaseDao<Book>{ ?? ?/** ?? ? * 增删改查的通用套路 ?? ? * 1、建立连接 ?? ? * 2、预定义对象PreparedStatement ?? ? * 3、设置占位符 ?? ? * 4、ps.executeUpdate() ?? ? * @throws Exception? ?? ? */ // ? ? ? ? ?? 增加 ?? ?public void add(Book book) throws Exception { ?? ??? ?String sql="insert into t_mvc_book values(?,?,?)"; ?? ??? ?super.executeUpdate(sql, book, new String[] {"bid","bname","price"}); ?? ?} // ? ? ? ? ?? 修改 ?? ?public void edit(Book book) throws Exception { ?? ??? ?String sql="update t_mvc_book set bname= ?,price= ? where bid= ?"; ?? ??? ?super.executeUpdate(sql, book, new String[] {"bname","price","bid"}); ?? ?} // ? ? ? ? ?? 删除 ?? ?public void delete(Book book) throws Exception { ?? ??? ?String sql="delete from t_mvc_book where bid=?"; ?? ??? ?super.executeUpdate(sql, book, new String[] {"bid"}); ?? ?} // ? ? ? ? ?? 查看 ?? ?public List<Book> list(Book book,PageBean pageBean) throws Exception { ?? ??? ?String sql="select * from t_mvc_book where 1=1"; ?? ??? ?String bname=book.getBname(); ?? ??? ?int bid=book.getBid(); ?? ??? ?if(StringUtils.isNotBlank(bname)) { ?? ??? ??? ?sql+=" and bname like '%"+bname+"%'"; ?? ??? ?} ?? ??? ?if(bid!=0) { ?? ??? ??? ?sql+=" and bid = "+bid; ?? ??? ?} ?? ??? ?return super.executeQuery(sql, Book.class, pageBean); ?? ?} ?? }
子控制器BookAction:
类需要extends ActionSupport implements ModelDriver<Book>
package com.lv.web;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.lv.dao.BookDao;
import com.lv.entity.Book;
import com.lv.framework.ActionSupport;
import com.lv.framework.ModelDriver;
import com.lv.util.PageBean;
public class BookAction extends ActionSupport implements ModelDriver<Book>{
private Book book=new Book();
private BookDao bd=new BookDao();
@Override
public Book getModel() {
// TODO Auto-generated method stub
return book;
}
// 增删改最终都要跳回查询页面
/**
* 分析增删改查一共有多少结果集的配置
* 查询:BookList.jsp 返回值:list
* 增删改确定:book.action?methodName=list 返回值:toList
* 增删改跳转对应界面:bookEdit.jsp 返回值:toEdit
* @param req
* @param resp
* @return
*/
public String add(HttpServletRequest req, HttpServletResponse resp) {
try {
bd.add(book);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "toList";
}
public String list(HttpServletRequest req, HttpServletResponse resp) {
try {
PageBean pageBean=new PageBean();
pageBean.setRequest(req);
List<Book> list = bd.list(book, pageBean);
req.setAttribute("books", list);
req.setAttribute("pageBean", pageBean);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "list";
}
public String delete(HttpServletRequest req, HttpServletResponse resp) {
try {
bd.delete(book);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "toList";
}
public String edit(HttpServletRequest req, HttpServletResponse resp) {
try {
bd.edit(book);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "toList";
}
// 跳转到新增修改页面
public String toEdit(HttpServletRequest req, HttpServletResponse resp) {
try {
// 如果是跳转修改页面,那么需要做bid条件的精准查询
if(book.getBid()!=0) {
List<Book> list=bd.list(book, null);
req.setAttribute("b", list.get(0));
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "toEdit";
}
}
主界面:
<%@ page language='java' contentType='text/html; charset=UTF-8' ?? ?pageEncoding='UTF-8'%> ?? ?<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> ?? ?<%@ taglib prefix="z" uri="http://jsp.veryedu.cn"%> <!DOCTYPE html PUBLIC '-//W3C//DTD HTML 4.01 Transitional//EN' 'http://www.w3.org/TR/html4/loose.dtd'> <html> <head> <meta http-equiv='Content-Type' content='text/html; charset=UTF-8'> <link ?? ?href='https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.0/css/bootstrap.css' ?? ?rel='stylesheet'> <script ?? ?src='https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.0/js/bootstrap.js'></script> <title>书籍列表</title> <style type='text/css'> .page-item input { ?? ?padding: 0; ?? ?width: 40px; ?? ?height: 100%; ?? ?text-align: center; ?? ?margin: 0 6px; }
.page-item input, .page-item b { ?? ?line-height: 38px; ?? ?float: left; ?? ?font-weight: 400; }
.page-item.go-input { ?? ?margin: 0 10px; } </style> </head> <body> ?? ?<form class="form-inline" ?? ??? ?action="${pageContext.request.contextPath }/book.action?methodName=list" method='post'> ?? ??? ?<div class='form-group mb-2'> ?? ??? ??? ?<input type='text' class='form-control-plaintext' name='bname' ?? ??? ??? ??? ?placeholder='请输入书籍名称'> ?? ??? ??? ??? ?<!-- 不分页 --> ?? ??? ??? ?<!-- ?? ?<input name="rows" value="false" type="hidden"> --> ?? ??? ?</div> ?? ??? ?<button type='submit' class='btn btn-primary mb-2'>查询</button> ?? ??? ?<a ?class='btn btn-primary mb-2' href="${pageContext.request.contextPath }/book.action?methodName=toEdit">>新增</a> ?? ?</form>
?? ?<table class='table table-striped bg-success'> ?? ??? ?<thead> ?? ??? ??? ?<tr> ?? ??? ??? ??? ?<th scope='col'>书籍ID</th> ?? ??? ??? ??? ?<th scope='col'>书籍名</th> ?? ??? ??? ??? ?<th scope='col'>价格</th> ?? ??? ??? ??? ?<th scope='col'>操作</th> ?? ??? ??? ?</tr> ?? ??? ?</thead> ?? ??? ?<tbody> ?? ??? ?<c:forEach var="b" items="${books }"> ?? ??? ?<tr> ?? ??? ??? ??? ?<td>${b.bid }</td> ?? ??? ??? ??? ?<td>${b.bname }</td> ?? ??? ??? ??? ?<td>${b.price }</td> ?? ??? ??? ??? ?<td> ?? ??? ??? ??? ?<a href="${pageContext.request.contextPath }/book.action?methodName=toEdit&bid=${b.bid}">>修改</a> ?? ??? ??? ??? ?<a href="${pageContext.request.contextPath }/book.action?methodName=delete&bid=${b.bid}">>删除</a>
?? ??? ??? ??? ? ?? ??? ??? ??? ?</td> ?? ??? ??? ?</tr> ?? ??? ?</c:forEach> ?? ??? ??? ?
?? ??? ?</tbody> ?? ?</table> ?? ?<!-- 这一行就相当于前面分页需求前端的几十行了 --> ?? ?<z:page pageBean="${pageBean }"></z:page> ?? ? </body> </html>
呈现效果:
?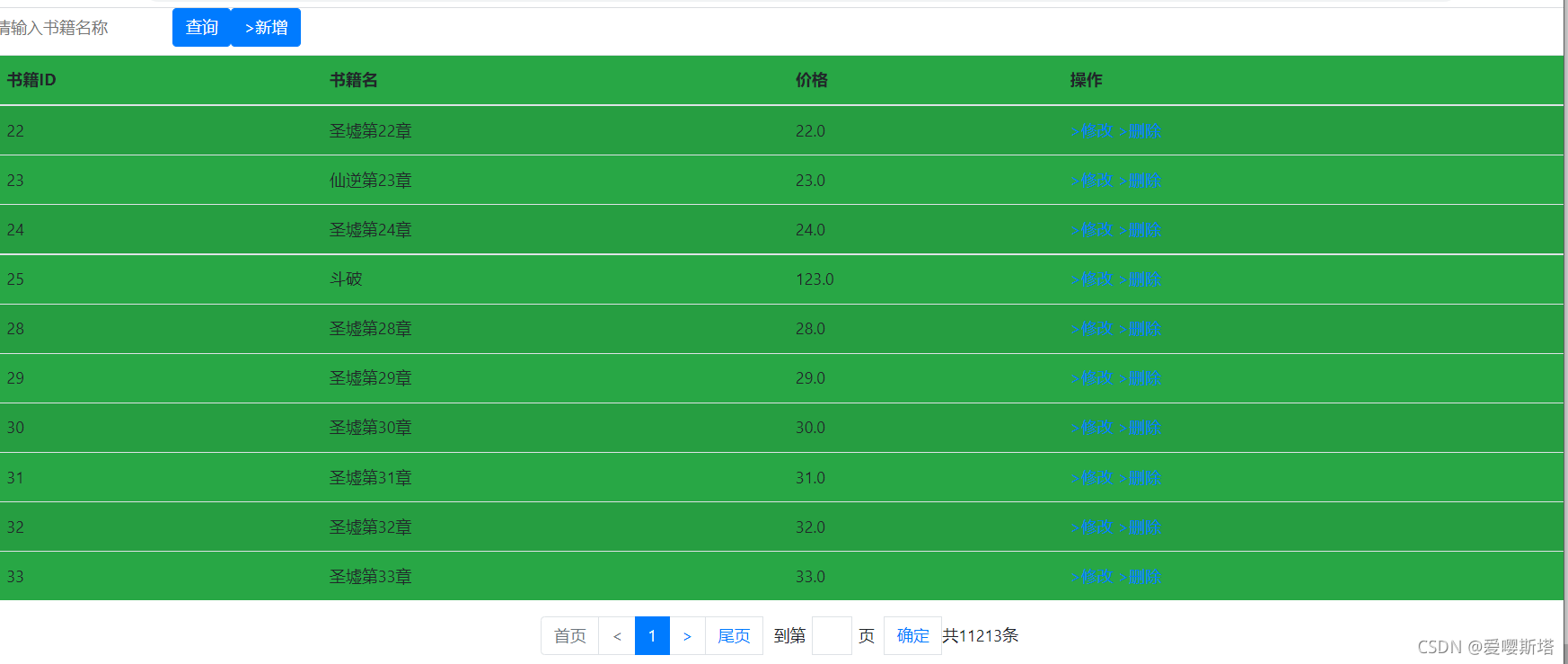
?增加与修改是同一个界面:
<%@ page language='java' contentType='text/html; charset=UTF-8' ?? ?pageEncoding='UTF-8'%> ?? ?<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> ?? ?<%@ taglib prefix="z" uri="http://jsp.veryedu.cn"%> <!DOCTYPE html PUBLIC '-//W3C//DTD HTML 4.01 Transitional//EN' 'http://www.w3.org/TR/html4/loose.dtd'> <html> <head> <meta http-equiv='Content-Type' content='text/html; charset=UTF-8'> <link ?? ?href='https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.0/css/bootstrap.css' ?? ?rel='stylesheet'> <script ?? ?src='https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.0/js/bootstrap.js'></script> <title>新增/修改书籍列表</title>
</head> <body> ?? ?<form class="form-inline" ?? ??? ?action="${pageContext.request.contextPath }/book.action?methodName=${empty b ? 'add' : 'edit'}" method="post"> ?? ??? ?书籍ID:<input type="text" name="bid" value="${b.bid }"><br> ?? ??? ?书籍名称:<input type="text" name="bname" value="${b.bname }"><br> ?? ??? ?书籍价格:<input type="text" name="price" value="${b.price }"><br> ?? ??? ?<input type="submit"> ?? ?</form>
</body> </html>
?增加效果界面:

?修改界面呈现数据:

?本期内容结束~
|