单元测试
Junit介绍
Junit是一个Java语言的单元测试框架,简单理解为可以用取代Java的(部分)main方法。Junit属于第三方工具,需导入jar包后使用。
Junit基本使用
public class Demo01Junit {
@Test
public void show01(){
System.out.println("show01方法!");
System.out.println("show01方法!");
System.out.println("show01方法!");
System.out.println("show01方法!");
System.out.println("show01方法!");
}
@Test
public void show02(){
System.out.println("show02方法!");
}
@Test
public void show03(){
System.out.println("show03方法!");
}
}
Junit注意事项
public class Demo02Junit {
public void show01(){
System.out.println("show01方法!");
}
@Test
protected void show02(){
System.out.println("show02方法!");
}
@Test
public void show03(int a){
System.out.println("show03方法!"+a);
}
@Test
public String show04(){
System.out.println("show01方法!");
return "aaaa";
}
@Test
public static void show05(){
System.out.println("show05方法!");
}
}
Junit相关注解
public class Demo03Junit {
@Test
public void show01(){
System.out.println("show01方法");
}
@Test
public void show02(){
System.out.println("show02方法");
}
@Test
public void show03(){
System.out.println("show03方法");
}
@Before
public void before(){
System.out.println("before方法");
}
@After
public void after(){
System.out.println("after方法");
}
@BeforeClass
public static void beforeClass(){
System.out.println("beforeClass方法");
}
@AfterClass
public static void afterClass(){
System.out.println("afterClass方法");
}
}
BIO、NIO、AIO概述
1).BIO:Block(阻塞的) IO。【同步、阻塞】 2).NIO:Non-Block(非阻塞的(同步)IO——JDK1.4开始的。【同步、非阻塞】 3).AIO:Asynchronous(异步-非阻塞)IO——JDK1.7开始【异步、非阻塞】
阻塞和非阻塞,同步和异步的概念
举个例子,比如我们去照相馆拍照,拍完照片之后,商家说需要30分钟左右才能洗出来照片
-
同步+阻塞 这个时候如果我们一直在店里面啥都不干,一直等待商家面前等待它洗完照片,这个过程就叫同步阻塞。 -
同步+非阻塞 当然大部分人很少这么干,更多的是大家拿起手机开始看电视,看一会就会问老板洗完没,老板说没洗完,然后我们接着看,再过一会接着问(轮询),直到照片洗完,这个过程就叫同步非阻塞。 -
异步+阻塞 因为店里生意太好了,越来越多的人过来拍,店里面快没地方坐了,老板说你把你手机号留下,我一会洗好了就打电话告诉你过来取,然后你去外面找了一个长凳开始躺着睡觉等待老板打电话,啥不都干,这个过程就叫异步阻塞。 -
异步+非阻塞 当然实际情况是,大家可能会直接先去逛街或者吃饭做其他的活动,同时等待老板打电话,这样以来两不耽误,这个过程就叫异步非阻塞。
总结
从上面的描述中我们其实能够看到阻塞和非阻塞通常是指客户端在发出请求后,在服务端处理这个请求的过程中,客户端本身是否直接挂起等待结果(阻塞),还是继续做其他的任务(非阻塞)。 而异步和同步,则是对于请求结果的获取是客户端主动等待获取(同步),还是由服务端来通知消息结果(异步)。 从这一点来看同步和阻塞其实描述的两个不同角度的事情,阻塞和非阻塞指的一个是客户端等待消息处理时的本身的状态,是挂起还是继续干别的。同步和异步指的对于消息结果的获取是客户端主动获取,还是由服务端间接推送。
阻塞:等待结果
非阻塞:可以做别的事情
同步:主动获取结果
异步:等待服务器通知结果
NIO之所以是同步,是因为它的accept/read/write方法的内核I/O操作都会阻塞当前线程 首先,我们要先了解一下NIO的三个主要组成部分:Buffer(缓冲区)、Channel(通道)、Selector(选择器)
Buffer类(缓冲区)概述
- java.nio.Buffer(抽象类):用于特定原始类型(基本类型)的数据的容器。后期在会用Channel进行通信时,底层全部使用Buffer。
- 它的几个子类:
1.ByteBuffer:里面可以封装一个byte[]数组。【重点掌握】 2.ShortBuffer:里面可以封装一个short[]数组。 3.CharBuffer:里面可以封装一个char[]数组 4.IntBuffer:里面可以封装一个int[]数组。 5.LongBuffer:里面可以封装一个long[]数组。 6.FloatBuffer:里面可以封装一个float[]数组。 7.DoubleBuffer:里面可以封装一个double[]数组。
创建ByteBuffer
- 没有构造方法可以创建ByteBuffer,可以通过它的一些“静态方法”获取ByteBuffer对象。
- 常用三个静态方法: new byte[10]; 默认值 0,0,0…0
- public static ByteBuffer allocate(int capacity):使用一个“容量”来创建一个“间接字节缓存区”——程序的“堆”空间中创建。
- public static ByteBuffer allocateDirect(int capacity):使用一个“容量”来创建一个“直接字节缓存区”——系统内存。 {1,2,3,4,5}
- public static ByteBuffer wrap(byte[] byteArray):使用一个“byte[]数组”创建一个“间接字节缓存区”。
public class Demo01ByteBuffer {
public static void main(String[] args) {
ByteBuffer buffer1 = ByteBuffer.allocate(100);
ByteBuffer buffer2 = ByteBuffer.allocateDirect(100);
ByteBuffer buffer3 = ByteBuffer.wrap("我爱java".getBytes());
}
}
向ByteBuffer添加数据
- public ByteBuffer put(byte b):向当前可用位置添加数据。
- public ByteBuffer put(byte[] byteArray):向当前可用位置添加一个byte[]数组
- public ByteBuffer put(byte[] byteArray,int offset,int len):添加一个byte[]数组的一部分
- byte[] array()获取此缓冲区的 byte 数组
public class Demo02ByteBuffer {
public static void main(String[] args) {
ByteBuffer buffer = ByteBuffer.allocate(10);
System.out.println(Arrays.toString(buffer.array()));
buffer.put((byte)1);
buffer.put((byte)2);
System.out.println(Arrays.toString(buffer.array()));
byte[] bytes = {10,20,30,40,50};
buffer.put(bytes);
System.out.println(Arrays.toString(buffer.array()));
buffer.put(bytes,3,2);
System.out.println(Arrays.toString(buffer.array()));
buffer.put(9,(byte)88);
System.out.println(Arrays.toString(buffer.array()));
}
}
容量-capacity
- Buffer的容量(capacity)是指:Buffer所能够包含的元素的最大数量。定义了Buffer后,容量是不可变的。
public class Demo03capacity {
public static void main(String[] args) {
ByteBuffer buffer1 = ByteBuffer.allocate(10);
System.out.println("容量:"+buffer1.capacity());
ByteBuffer buffer2 = ByteBuffer.wrap("你好".getBytes());
System.out.println("容量:"+buffer2.capacity());
}
}
限制 -limit
- 限制:limit:表示如果设置“限制为某一个位置,那么此位置后的位置将不可用”。
- 有两个相关方法:
- public int limit():获取此缓冲区的限制。
- public Buffer limit(int newLimit):设置此缓冲区的限制。
public class Demo04Limit {
public static void main(String[] args) {
ByteBuffer buf = ByteBuffer.allocate(10);
System.out.println("容量:" + buf.capacity() + " 限制:" + buf.limit());
buf.limit(3);
buf.put((byte)0);
buf.put((byte)1);
buf.put((byte)2);
System.out.println("容量:" + buf.capacity() + " 限制:" + buf.limit());
}
}
位置 -position
- 位置position是指:当前可写入的索引。位置不能小于0,并且不能大于"限制"。
- 有两个相关方法:
- public int position():获取当前可写入位置索引。
- public Buffer position(int p):更改当前可写入位置索引。
public class Demo05Position {
public static void main(String[] args) {
ByteBuffer buf = ByteBuffer.allocate(10);
System.out.println("初始容量:" + buf.capacity() +
" 初始限制:" + buf.limit() +
" 当前位置:" + buf.position());
buf.put((byte) 10);
buf.put((byte) 20);
buf.put((byte) 30);
System.out.println("当前容量:" + buf.capacity() +
" 初始限制:" + buf.limit() +
" 当前位置:" + buf.position());
System.out.println(Arrays.toString(buf.array()));
buf.position(1);
buf.put((byte) 2);
buf.put((byte) 3);
System.out.println(Arrays.toString(buf.array()));
buf.limit(3);
buf.put((byte) 4);
}
}
标记 -mark
- 标记mark是指:当调用缓冲区的reset()方法时,会将缓冲区的position位置重置为该索引。不能为0,不能大于position。
- 相关方法:
- public Buffer mark():设置此缓冲区的标记为当前的position位置。
public class Demo06mark {
public static void main(String[] args) {
ByteBuffer buffer = ByteBuffer.allocate(10);
buffer.put((byte)0);
buffer.mark();
buffer.put((byte)1);
buffer.put((byte)2);
System.out.println("position:"+buffer.position());
System.out.println(Arrays.toString(buffer.array()));
buffer.reset();
System.out.println("position:"+buffer.position());
buffer.put((byte)100);
System.out.println(Arrays.toString(buffer.array()));
}
}
其他方法
- public int remaining():获取position与limit之间的元素数。
- public boolean isReadOnly():获取当前缓冲区是否只读。
- public boolean isDirect():获取当前缓冲区是否为直接缓冲区。
- public Buffer clear():还原缓冲区的状态。
- 将position设置为:0
- 将限制limit设置为容量capacity;
- 丢弃标记mark。
- public Buffer flip():缩小limit的范围。 获取读取的有效数据0到position之间的数据
- 将limit设置为当前position位置;
- 将当前position位置设置为0;
- 丢弃标记。
Channel(通道)
概述
1).java.nio.channels.Channel(接口):用于 I/O 操作的连接。
- 表示:通道。
- 可以是“文件通道-FileChannel”、“网络通道-SocketChannel和ServerSockecChannel”。
- 它类似于IO流,但比IO流更强大。read(byte[]) write(byte[])
- IO流是“单向”的,Channel是“双向的”。
2).Channel全部使用Buffer实现读、写。read(ByteBuffer) write(ByteBuffer)
FileChannel类的基本使用(重点)
- java.nio.channels.FileChannel (抽象类):用于读、写文件的通道。
- FileChannel是抽象类,我们可以通过FileInputStream和FileOutputStream的getChannel()方法方便的获取一个它的子类对象。
public class Demo01FileChannel {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("c:\\1.jpg");
FileOutputStream fos = new FileOutputStream("d:\\1.jpg");
FileChannel fisChannel = fis.getChannel();
FileChannel fosChannel = fos.getChannel();
ByteBuffer buffer = ByteBuffer.allocate(1024);
int len = 0;
while ((len=fisChannel.read(buffer))!=-1){
buffer.flip();/mit设置为当前position位置,position位置设置为0
fosChannel.write(buffer);
buffer.clear();
}
fosChannel.close();
fisChannel.close();
fos.close();
fis.close();
}
}
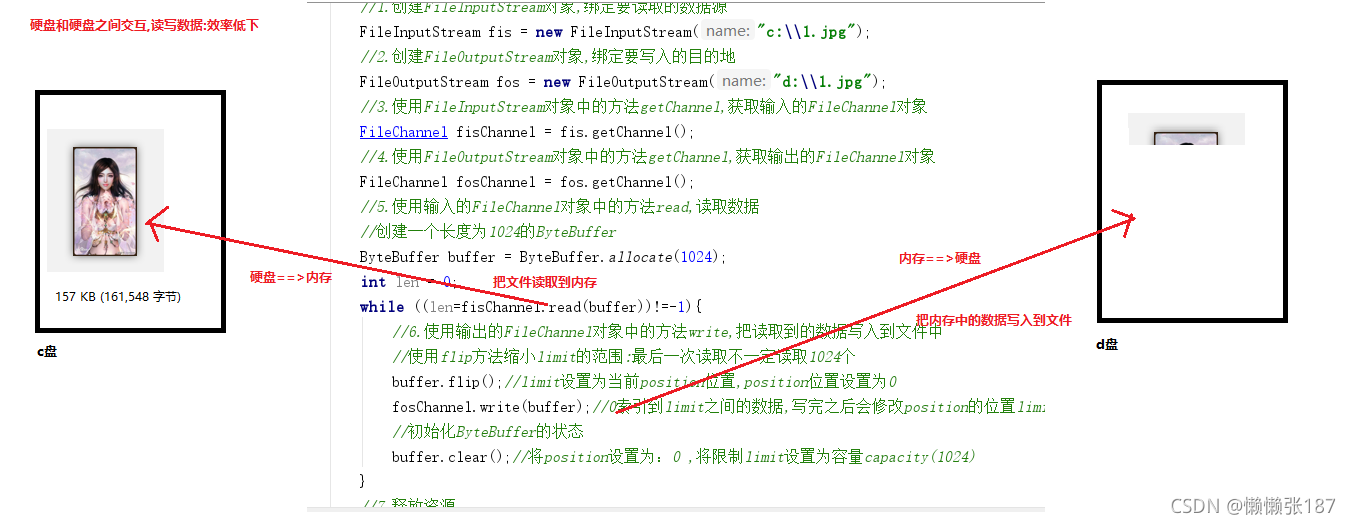
FileChannle结合MappedByteBuffer实现高效读写(重点)
- java.io.RandomAccessFile类
获取FileChannel需要使用RandomAccessFile类,可以创建流对象的同时设置读写模式
java.io.RandomAccessFile类,可以设置读、写模式的IO流类
构造方法:
RandomAccessFile(String name, String mode)
参数:
String name:要读取的数据源,或者写入的目的地
String mode:设置流的读写模式
"r":只读,必须是小写
"rw":读写,必须是小写
成员方法:
FileChannel getChannel() 返回与此文件关联的唯一 FileChannel 对象。
- 使用FileChannel类中的方法map得到MappedByteBuffer
- MappedByteBuffer map(FileChannel.MapMode mode, long position, long size) 将此通道的文件区域直接映射到内存中。
参数:
FileChannel.MapMode mode:设置读写的模式
READ_ONLY:只读映射模式。
READ_WRITE:读取/写入映射模式。
long position:文件中的位置,映射区域从此位置开始,一般都是从0开始
size - 要映射的区域大小,就是要复制文件的大小,单位字节
- java.nio.MappedByteBuffer 类
java.nio.MappedByteBuffer:它可以创建“直接缓存区”,将文件的磁盘数据映射到内存。
注意:它最大可以映射:Integer.MAX_VALUE个字节(2G)左右。
eg:磁盘和内存实时映射 硬盘(abc) 内存(abc) 内存修改为(ab) 磁盘也跟着修改(ab)
MappedByteBuffer中的方法:
byte get(int index) 获取缓冲区中指定索引处的字节
ByteBuffer put(int index, byte b) 把字节写入到指定的索引处
public class Demo02MappedByteBuffer {
public static void main(String[] args) throws IOException {
long s = System.currentTimeMillis();
RandomAccessFile inRAF = new RandomAccessFile("c:\\748m.rar","r");
RandomAccessFile outRAF = new RandomAccessFile("d:\\748m.rar","rw");
FileChannel inRAFChannel = inRAF.getChannel();
FileChannel outRAFChannel = outRAF.getChannel();
long size = inRAFChannel.size();
System.out.println(size);
MappedByteBuffer inMap = inRAFChannel.map(FileChannel.MapMode.READ_ONLY, 0, size);
MappedByteBuffer outMap = outRAFChannel.map(FileChannel.MapMode.READ_WRITE, 0, size);
for (int i = 0; i < size; i++) {
byte b = inMap.get(i);
outMap.put(i,b);
}
outRAF.close();
inRAF.close();
long e = System.currentTimeMillis();
System.out.println("复制文件共耗时:"+(e-s)+"毫秒!");
}
}
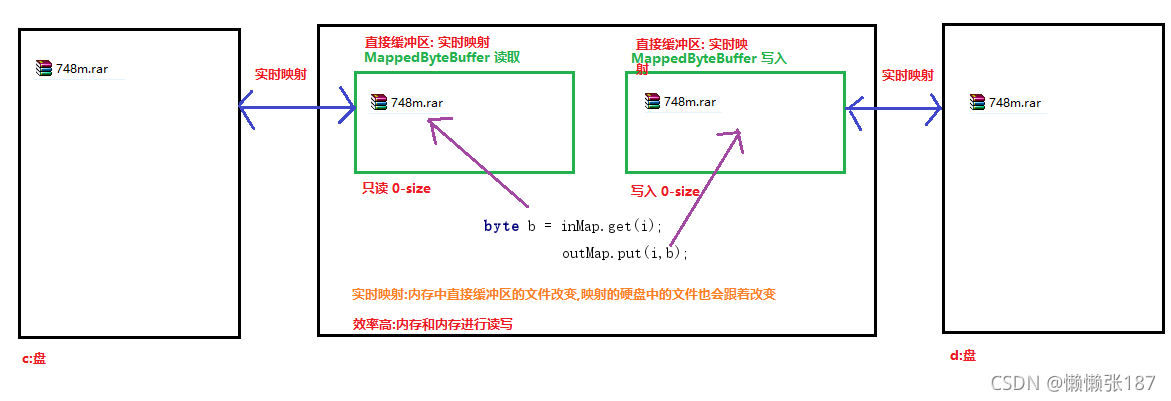
- 代码实现 :复制2g以上的文件
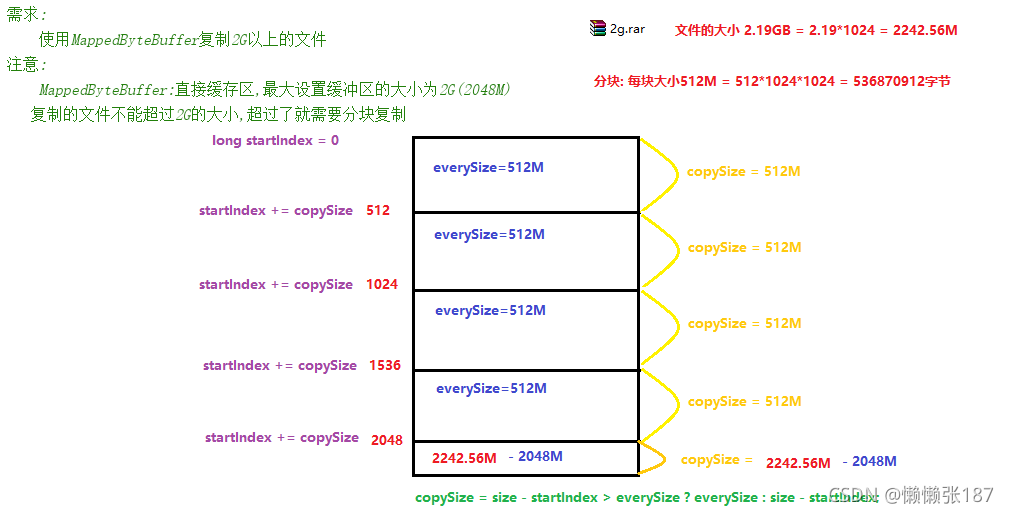
public class Demo03MappedByteBuffer {
public static void main(String[] args) throws IOException {
long s = System.currentTimeMillis();
RandomAccessFile inRAF = new RandomAccessFile("c:\\2g.rar","r");
RandomAccessFile outRAF = new RandomAccessFile("d:\\2g.rar","rw");
FileChannel inRAFChannel = inRAF.getChannel();
FileChannel outRAFChannel = outRAF.getChannel();
long size = inRAFChannel.size();
long count = 1;
long startIndex = 0;
long copySize = size;
long everSize = 512*1024*1024;
if(size>everSize){
count = size%everSize==0 ? size/everSize : size/everSize+1;
copySize = everSize;
}
for (int i = 0; i < count; i++) {
MappedByteBuffer inMap = inRAFChannel.map(FileChannel.MapMode.READ_ONLY, startIndex, copySize);
MappedByteBuffer outMap = outRAFChannel.map(FileChannel.MapMode.READ_WRITE, startIndex, copySize);
System.out.println("每块文件的开始索引:"+startIndex);
System.out.println("每次复制每块文件的大小:"+copySize);
System.out.println("-------------------------------------------");
for (int j = 0; j < copySize; j++) {
byte b = inMap.get(j);
outMap.put(j,b);
}
startIndex += copySize;
copySize = size-startIndex > everSize ? everSize : size-startIndex;
}
inRAFChannel.close();
outRAFChannel.close();
long e = System.currentTimeMillis();
System.out.println("复制文件共耗时:"+(e-s)+"毫秒!");
}
}
|